mbed official WiflyInterface (interface for Roving Networks Wifly modules)
Dependents: Wifly_HelloWorld Websocket_Wifly_HelloWorld RPC_Wifly_HelloWorld HTTPClient_Wifly_HelloWorld ... more
CBuffer.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef CIRCBUFFER_H_ 00020 #define CIRCBUFFER_H_ 00021 00022 template <class T> 00023 class CircBuffer { 00024 public: 00025 CircBuffer(int length) { 00026 write = 0; 00027 read = 0; 00028 size = length + 1; 00029 buf = (T *)malloc(size * sizeof(T)); 00030 }; 00031 00032 bool isFull() { 00033 return (((write + 1) % size) == read); 00034 }; 00035 00036 bool isEmpty() { 00037 return (read == write); 00038 }; 00039 00040 void queue(T k) { 00041 if (isFull()) { 00042 read++; 00043 read %= size; 00044 } 00045 buf[write++] = k; 00046 write %= size; 00047 } 00048 00049 void flush() { 00050 read = 0; 00051 write = 0; 00052 } 00053 00054 00055 uint32_t available() { 00056 return (write >= read) ? write - read : size - read + write; 00057 }; 00058 00059 bool dequeue(T * c) { 00060 bool empty = isEmpty(); 00061 if (!empty) { 00062 *c = buf[read++]; 00063 read %= size; 00064 } 00065 return(!empty); 00066 }; 00067 00068 private: 00069 volatile uint32_t write; 00070 volatile uint32_t read; 00071 uint32_t size; 00072 T * buf; 00073 }; 00074 00075 #endif
Generated on Tue Jul 12 2022 18:24:21 by
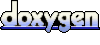