mbed official WiflyInterface (interface for Roving Networks Wifly modules)
Dependents: Wifly_HelloWorld Websocket_Wifly_HelloWorld RPC_Wifly_HelloWorld HTTPClient_Wifly_HelloWorld ... more
Wifly.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 * @section DESCRIPTION 00019 * 00020 * Wifly RN131-C, wifi module 00021 * 00022 * Datasheet: 00023 * 00024 * http://dlnmh9ip6v2uc.cloudfront.net/datasheets/Wireless/WiFi/WiFly-RN-UM.pdf 00025 */ 00026 00027 #ifndef WIFLY_H 00028 #define WIFLY_H 00029 00030 #include "mbed.h" 00031 #include "RawSerial.h" 00032 #include "CBuffer.h" 00033 00034 #define DEFAULT_WAIT_RESP_TIMEOUT 10000 00035 00036 enum Security { 00037 NONE = 0, 00038 WEP_128 = 1, 00039 WPA = 3 00040 }; 00041 00042 enum Protocol { 00043 UDP = (1 << 0), 00044 TCP = (1 << 1) 00045 }; 00046 00047 /** 00048 * The Wifly class 00049 */ 00050 class Wifly 00051 { 00052 00053 public: 00054 /** 00055 * Constructor 00056 * 00057 * @param tx mbed pin to use for tx line of Serial interface 00058 * @param rx mbed pin to use for rx line of Serial interface 00059 * @param reset reset pin of the wifi module () 00060 * @param tcp_status connection status pin of the wifi module (GPIO 6) 00061 * @param ssid ssid of the network 00062 * @param phrase WEP or WPA key 00063 * @param sec Security type (NONE, WEP_128 or WPA) 00064 */ 00065 Wifly( PinName tx, PinName rx, PinName reset, PinName tcp_status, const char * ssid, const char * phrase, Security sec); 00066 00067 /** 00068 * Connect the wifi module to the ssid contained in the constructor. 00069 * 00070 * @return true if connected, false otherwise 00071 */ 00072 bool join(); 00073 00074 /** 00075 * Disconnect the wifly module from the access point 00076 * 00077 * @return true if successful 00078 */ 00079 bool disconnect(); 00080 00081 /** 00082 * Open a tcp connection with the specified host on the specified port 00083 * 00084 * @param host host (can be either an ip address or a name. If a name is provided, a dns request will be established) 00085 * @param port port 00086 * @return true if successful 00087 */ 00088 bool connect(const char * host, int port); 00089 00090 00091 /** 00092 * Set the protocol (UDP or TCP) 00093 * 00094 * @param p protocol 00095 * @return true if successful 00096 */ 00097 bool setProtocol(Protocol p); 00098 00099 /** 00100 * Reset the wifi module 00101 */ 00102 void reset(); 00103 00104 /** 00105 * Reboot the wifi module 00106 */ 00107 bool reboot(); 00108 00109 /** 00110 * Check if characters are available 00111 * 00112 * @return number of available characters 00113 */ 00114 int readable(); 00115 00116 /** 00117 * Check if characters are available 00118 * 00119 * @return number of available characters 00120 */ 00121 int writeable(); 00122 00123 /** 00124 * Check if a tcp link is active 00125 * 00126 * @return true if successful 00127 */ 00128 bool is_connected(); 00129 00130 /** 00131 * Read a character 00132 * 00133 * @return the character read 00134 */ 00135 char getc(); 00136 00137 /** 00138 * Flush the buffer 00139 */ 00140 void flush(); 00141 00142 /** 00143 * Write a character 00144 * 00145 * @param the character which will be written 00146 */ 00147 int putc(char c); 00148 00149 00150 /** 00151 * To enter in command mode (we can configure the module) 00152 * 00153 * @return true if successful, false otherwise 00154 */ 00155 bool cmdMode(); 00156 00157 /** 00158 * To exit the command mode 00159 * 00160 * @return true if successful, false otherwise 00161 */ 00162 bool exit(); 00163 00164 /** 00165 * Close a tcp connection 00166 * 00167 * @return true if successful 00168 */ 00169 bool close(); 00170 00171 /** 00172 * Send a string to the wifi module by serial port. This function desactivates the user interrupt handler when a character is received to analyze the response from the wifi module. 00173 * Useful to send a command to the module and wait a response. 00174 * 00175 * 00176 * @param str string to be sent 00177 * @param len string length 00178 * @param ACK string which must be acknowledge by the wifi module. If ACK == NULL, no string has to be acknoledged. (default: "NO") 00179 * @param res this field will contain the response from the wifi module, result of a command sent. This field is available only if ACK = "NO" AND res != NULL (default: NULL) 00180 * 00181 * @return true if ACK has been found in the response from the wifi module. False otherwise or if there is no response in 5s. 00182 */ 00183 int send(const char * str, int len, const char * ACK = NULL, char * res = NULL, int timeout = DEFAULT_WAIT_RESP_TIMEOUT); 00184 00185 /** 00186 * Send a command to the wify module. Check if the module is in command mode. If not enter in command mode 00187 * 00188 * @param str string to be sent 00189 * @param ACK string which must be acknowledge by the wifi module. If ACK == NULL, no string has to be acknoledged. (default: "NO") 00190 * @param res this field will contain the response from the wifi module, result of a command sent. This field is available only if ACK = "NO" AND res != NULL (default: NULL) 00191 * 00192 * @return true if successful 00193 */ 00194 bool sendCommand(const char * cmd, const char * ack = NULL, char * res = NULL, int timeout = DEFAULT_WAIT_RESP_TIMEOUT); 00195 00196 /** 00197 * Return true if the module is using dhcp 00198 * 00199 * @return true if the module is using dhcp 00200 */ 00201 bool isDHCP() { 00202 return state.dhcp; 00203 } 00204 00205 bool gethostbyname(const char * host, char * ip); 00206 00207 static Wifly * getInstance() { 00208 return inst; 00209 }; 00210 00211 protected: 00212 RawSerial wifi; 00213 DigitalOut reset_pin; 00214 DigitalIn tcp_status; 00215 char phrase[65]; 00216 char ssid[33]; 00217 const char * ip; 00218 const char * netmask; 00219 const char * gateway; 00220 int channel; 00221 CircBuffer<char> buf_wifly; 00222 00223 static Wifly * inst; 00224 00225 void attach_rx(bool null); 00226 void handler_rx(void); 00227 00228 00229 typedef struct STATE { 00230 bool associated; 00231 bool tcp; 00232 bool dhcp; 00233 Security sec; 00234 Protocol proto; 00235 bool cmd_mode; 00236 } State; 00237 00238 State state; 00239 char * getStringSecurity(); 00240 }; 00241 00242 #endif
Generated on Tue Jul 12 2022 18:24:22 by
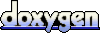