USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
WANDongle.cpp
00001 /* Copyright (c) 2010-2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "USBHostConf.h" 00020 00021 #ifdef USBHOST_3GMODULE 00022 00023 #include "dbg.h" 00024 #include <stdint.h> 00025 #include "rtos.h" 00026 00027 #include "WANDongle.h" 00028 #include "WANDongleInitializer.h" 00029 00030 WANDongle::WANDongle() : m_pInitializer(NULL), m_serialCount(0), m_totalInitializers(0) 00031 { 00032 host = USBHost::getHostInst(); 00033 init(); 00034 } 00035 00036 00037 bool WANDongle::connected() { 00038 return dev_connected; 00039 } 00040 00041 bool WANDongle::tryConnect() 00042 { 00043 //FIXME should run on USB thread 00044 00045 USB_DBG("Trying to connect device"); 00046 00047 if (dev_connected) { 00048 USB_DBG("Device is already connected!"); 00049 return true; 00050 } 00051 00052 m_pInitializer = NULL; 00053 00054 //Protect from concurrent access from USB thread 00055 USBHost::Lock lock(host); 00056 00057 for (int i = 0; i < MAX_DEVICE_CONNECTED; i++) 00058 { 00059 if ((dev = host->getDevice(i)) != NULL) 00060 { 00061 m_pInitializer = NULL; //Will be set in setVidPid callback 00062 00063 USB_DBG("Enumerate"); 00064 int ret = host->enumerate(dev, this); 00065 if(ret) 00066 { 00067 return false; 00068 } 00069 00070 USB_DBG("Device has VID:%04x PID:%04x", dev->getVid(), dev->getPid()); 00071 00072 if(m_pInitializer) //If an initializer has been found 00073 { 00074 USB_DBG("m_pInitializer=%p", m_pInitializer); 00075 USB_DBG("m_pInitializer->getSerialVid()=%04x", m_pInitializer->getSerialVid()); 00076 USB_DBG("m_pInitializer->getSerialPid()=%04x", m_pInitializer->getSerialPid()); 00077 if ((dev->getVid() == m_pInitializer->getSerialVid()) && (dev->getPid() == m_pInitializer->getSerialPid())) 00078 { 00079 USB_DBG("The dongle is in virtual serial mode"); 00080 host->registerDriver(dev, 0, this, &WANDongle::init); 00081 m_serialCount = m_pInitializer->getSerialPortCount(); 00082 if( m_serialCount > WANDONGLE_MAX_SERIAL_PORTS ) 00083 { 00084 m_serialCount = WANDONGLE_MAX_SERIAL_PORTS; 00085 } 00086 for(int j = 0; j < m_serialCount; j++) 00087 { 00088 USB_DBG("Connecting serial port #%d", j+1); 00089 USB_DBG("Ep %p", m_pInitializer->getEp(dev, j, false)); 00090 USB_DBG("Ep %p", m_pInitializer->getEp(dev, j, true)); 00091 m_serial[j].connect( dev, m_pInitializer->getEp(dev, j, false), m_pInitializer->getEp(dev, j, true) ); 00092 } 00093 00094 USB_DBG("Device connected"); 00095 00096 dev_connected = true; 00097 00098 00099 return true; 00100 } 00101 else if ((dev->getVid() == m_pInitializer->getMSDVid()) && (dev->getPid() == m_pInitializer->getMSDPid())) 00102 { 00103 USB_DBG("Vodafone K3370 dongle detected in MSD mode"); 00104 //Try to switch 00105 if( m_pInitializer->switchMode(dev) ) 00106 { 00107 USB_DBG("Switched OK"); 00108 return false; //Will be connected on a next iteration 00109 } 00110 else 00111 { 00112 USB_ERR("Could not switch mode"); 00113 return false; 00114 } 00115 } 00116 } //if() 00117 } //if() 00118 } //for() 00119 return false; 00120 } 00121 00122 bool WANDongle::disconnect() 00123 { 00124 dev_connected = false; 00125 for(int i = 0; i < WANDONGLE_MAX_SERIAL_PORTS; i++) 00126 { 00127 m_serial[i].disconnect(); 00128 } 00129 return true; 00130 } 00131 00132 int WANDongle::getDongleType() 00133 { 00134 if( m_pInitializer != NULL ) 00135 { 00136 return m_pInitializer->getType(); 00137 } 00138 else 00139 { 00140 return WAN_DONGLE_TYPE_UNKNOWN; 00141 } 00142 } 00143 00144 IUSBHostSerial& WANDongle::getSerial(int index) 00145 { 00146 return m_serial[index]; 00147 } 00148 00149 int WANDongle::getSerialCount() 00150 { 00151 return m_serialCount; 00152 } 00153 00154 //Private methods 00155 void WANDongle::init() 00156 { 00157 m_pInitializer = NULL; 00158 dev_connected = false; 00159 for(int i = 0; i < WANDONGLE_MAX_SERIAL_PORTS; i++) 00160 { 00161 m_serial[i].init(host); 00162 } 00163 } 00164 00165 00166 /*virtual*/ void WANDongle::setVidPid(uint16_t vid, uint16_t pid) 00167 { 00168 WANDongleInitializer* initializer; 00169 00170 for(int i = 0; i < m_totalInitializers; i++) 00171 { 00172 initializer = m_Initializers[i]; 00173 USB_DBG("initializer=%p", initializer); 00174 USB_DBG("initializer->getSerialVid()=%04x", initializer->getSerialVid()); 00175 USB_DBG("initializer->getSerialPid()=%04x", initializer->getSerialPid()); 00176 if ((dev->getVid() == initializer->getSerialVid()) && (dev->getPid() == initializer->getSerialPid())) 00177 { 00178 USB_DBG("The dongle is in virtual serial mode"); 00179 m_pInitializer = initializer; 00180 break; 00181 } 00182 else if ((dev->getVid() == initializer->getMSDVid()) && (dev->getPid() == initializer->getMSDPid())) 00183 { 00184 USB_DBG("Dongle detected in MSD mode"); 00185 m_pInitializer = initializer; 00186 break; 00187 } 00188 initializer++; 00189 } //for 00190 if(m_pInitializer) 00191 { 00192 m_pInitializer->setVidPid(vid, pid); 00193 } 00194 } 00195 00196 /*virtual*/ bool WANDongle::parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol) //Must return true if the interface should be parsed 00197 { 00198 if(m_pInitializer) 00199 { 00200 return m_pInitializer->parseInterface(intf_nb, intf_class, intf_subclass, intf_protocol); 00201 } 00202 else 00203 { 00204 return false; 00205 } 00206 } 00207 00208 /*virtual*/ bool WANDongle::useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir) //Must return true if the endpoint will be used 00209 { 00210 if(m_pInitializer) 00211 { 00212 return m_pInitializer->useEndpoint(intf_nb, type, dir); 00213 } 00214 else 00215 { 00216 return false; 00217 } 00218 } 00219 00220 00221 bool WANDongle::addInitializer(WANDongleInitializer* pInitializer) 00222 { 00223 if (m_totalInitializers >= WANDONGLE_MAX_INITIALIZERS) 00224 return false; 00225 m_Initializers[m_totalInitializers++] = pInitializer; 00226 return true; 00227 } 00228 00229 WANDongle::~WANDongle() 00230 { 00231 for(int i = 0; i < m_totalInitializers; i++) 00232 delete m_Initializers[i]; 00233 } 00234 00235 #endif /* USBHOST_3GMODULE */
Generated on Tue Jul 12 2022 13:32:26 by
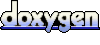