USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
USBHostKeyboard.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBHOSTKEYBOARD_H 00018 #define USBHOSTKEYBOARD_H 00019 00020 #include "USBHostConf.h" 00021 00022 #if USBHOST_KEYBOARD 00023 00024 #include "USBHost.h" 00025 00026 /** 00027 * A class to communicate a USB keyboard 00028 */ 00029 class USBHostKeyboard : public IUSBEnumerator 00030 { 00031 public: 00032 00033 /** 00034 * Constructor 00035 */ 00036 USBHostKeyboard(); 00037 00038 /** 00039 * Try to connect a keyboard device 00040 * 00041 * @return true if connection was successful 00042 */ 00043 bool connect(); 00044 00045 /** 00046 * Check if a keyboard is connected 00047 * 00048 * @returns true if a keyboard is connected 00049 */ 00050 bool connected(); 00051 00052 /** 00053 * Attach a callback called when a keyboard event is received 00054 * 00055 * @param ptr function pointer 00056 */ 00057 inline void attach(void (*ptr)(uint8_t key)) 00058 { 00059 if (ptr != NULL) { 00060 onKey = ptr; 00061 } 00062 } 00063 00064 /** 00065 * Attach a callback called when a keyboard event is received 00066 * 00067 * @param ptr function pointer 00068 */ 00069 inline void attach(void (*ptr)(uint8_t keyCode, uint8_t modifier)) 00070 { 00071 if (ptr != NULL) { 00072 onKeyCode = ptr; 00073 } 00074 } 00075 00076 protected: 00077 //From IUSBEnumerator 00078 virtual void setVidPid(uint16_t vid, uint16_t pid); 00079 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00080 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00081 00082 private: 00083 USBHost * host; 00084 USBDeviceConnected * dev; 00085 USBEndpoint * int_in; 00086 uint8_t report[9]; 00087 int keyboard_intf; 00088 bool keyboard_device_found; 00089 00090 bool dev_connected; 00091 00092 void rxHandler(); 00093 00094 void (*onKey)(uint8_t key); 00095 void (*onKeyCode)(uint8_t key, uint8_t modifier); 00096 00097 int report_id; 00098 00099 void init(); 00100 00101 }; 00102 00103 #endif 00104 00105 #endif
Generated on Tue Jul 12 2022 13:32:26 by
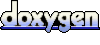