USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
USBHostHub.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBHOSTHUB_H 00018 #define USBHOSTHUB_H 00019 00020 #include "USBHostConf.h" 00021 00022 #if MAX_HUB_NB 00023 00024 #include "USBHostTypes.h" 00025 #include "IUSBEnumerator.h" 00026 00027 class USBHost; 00028 class USBDeviceConnected; 00029 class USBEndpoint; 00030 00031 /** 00032 * A class to use a USB Hub 00033 */ 00034 class USBHostHub : public IUSBEnumerator 00035 { 00036 public: 00037 /** 00038 * Constructor 00039 */ 00040 USBHostHub(); 00041 00042 /** 00043 * Check if a USB Hub is connected 00044 * 00045 * @return true if a serial device is connected 00046 */ 00047 bool connected(); 00048 00049 /** 00050 * Try to connect device 00051 * 00052 * @param dev device to connect 00053 * @return true if connection was successful 00054 */ 00055 bool connect(USBDeviceConnected * dev); 00056 00057 /** 00058 * Automatically called by USBHost when a device 00059 * has been enumerated by usb_thread 00060 * 00061 * @param dev device connected 00062 */ 00063 void deviceConnected(USBDeviceConnected * dev); 00064 00065 /** 00066 * Automatically called by USBHost when a device 00067 * has been disconnected from this hub 00068 * 00069 * @param dev device disconnected 00070 */ 00071 void deviceDisconnected(USBDeviceConnected * dev); 00072 00073 /** 00074 * Rest a specific port 00075 * 00076 * @param port port number 00077 */ 00078 void portReset(uint8_t port); 00079 00080 /* 00081 * Called by USBHost to set the instance of USBHost 00082 * 00083 * @param host host instance 00084 */ 00085 void setHost(USBHost * host); 00086 00087 /** 00088 * Called by USBhost when a hub has been disconnected 00089 */ 00090 void hubDisconnected(); 00091 00092 protected: 00093 //From IUSBEnumerator 00094 virtual void setVidPid(uint16_t vid, uint16_t pid); 00095 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00096 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00097 00098 private: 00099 USBHost * host; 00100 USBDeviceConnected * dev; 00101 bool dev_connected; 00102 USBEndpoint * int_in; 00103 uint8_t nb_port; 00104 uint8_t hub_characteristics; 00105 00106 void rxHandler(); 00107 00108 uint8_t buf[sizeof(HubDescriptor)]; 00109 00110 int hub_intf; 00111 bool hub_device_found; 00112 00113 void setPortFeature(uint32_t feature, uint8_t port); 00114 void clearPortFeature(uint32_t feature, uint8_t port); 00115 uint32_t getPortStatus(uint8_t port); 00116 00117 USBDeviceConnected * device_children[MAX_HUB_PORT]; 00118 00119 void init(); 00120 void disconnect(); 00121 00122 }; 00123 00124 #endif 00125 00126 #endif
Generated on Tue Jul 12 2022 13:32:26 by
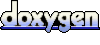