
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2013-2017, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef MBED_CONF_APP_CONNECT_STATEMENT 00019 #error [NOT_SUPPORTED] No network configuration found for this target. 00020 #endif 00021 00022 #include "mbed.h" 00023 #include "greentea-client/test_env.h" 00024 #include "unity.h" 00025 #include "utest.h" 00026 #include MBED_CONF_APP_HEADER_FILE 00027 00028 using namespace utest::v1; 00029 00030 // Bringing the network up and down 00031 template <int COUNT> 00032 void test_bring_up_down() { 00033 NetworkInterface* net = MBED_CONF_APP_OBJECT_CONSTRUCTION; 00034 00035 for (int i = 0; i < COUNT; i++) { 00036 int err = MBED_CONF_APP_CONNECT_STATEMENT; 00037 TEST_ASSERT_EQUAL(0, err); 00038 00039 printf("MBED: IP Address %s\r\n", net->get_ip_address()); 00040 TEST_ASSERT(net->get_ip_address()); 00041 00042 UDPSocket udp; 00043 err = udp.open(net); 00044 TEST_ASSERT_EQUAL(0, err); 00045 err = udp.close(); 00046 TEST_ASSERT_EQUAL(0, err); 00047 00048 TCPSocket tcp; 00049 err = tcp.open(net); 00050 TEST_ASSERT_EQUAL(0, err); 00051 err = tcp.close(); 00052 TEST_ASSERT_EQUAL(0, err); 00053 00054 err = net->disconnect(); 00055 TEST_ASSERT_EQUAL(0, err); 00056 } 00057 } 00058 00059 00060 // Test setup 00061 utest::v1::status_t test_setup(const size_t number_of_cases) { 00062 GREENTEA_SETUP(120, "default_auto"); 00063 return verbose_test_setup_handler(number_of_cases); 00064 } 00065 00066 Case cases[] = { 00067 Case("Bringing the network up and down", test_bring_up_down<1>), 00068 Case("Bringing the network up and down twice", test_bring_up_down<2>), 00069 }; 00070 00071 Specification specification(test_setup, cases); 00072 00073 int main() { 00074 return !Harness::run(specification); 00075 }
Generated on Sun Jul 17 2022 08:25:27 by
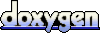