
Rtos API example
lwip_udp.c File Reference
User Datagram Protocol module
The code for the User Datagram Protocol UDP & UDPLite (RFC 3828).
More...
Go to the source code of this file.
Functions | |
void | udp_init (void) |
Initialize this module. | |
static u16_t | udp_new_port (void) |
Allocate a new local UDP port. | |
static u8_t | udp_input_local_match (struct udp_pcb *pcb, struct netif *inp, u8_t broadcast) |
Common code to see if the current input packet matches the pcb (current input packet is accessed via ip(4/6)_current_* macros) | |
void | udp_input (struct pbuf *p, struct netif *inp) |
Process an incoming UDP datagram. | |
err_t | udp_send (struct udp_pcb *pcb, struct pbuf *p) |
Send data using UDP. | |
err_t | udp_send_chksum (struct udp_pcb *pcb, struct pbuf *p, u8_t have_chksum, u16_t chksum) |
Same as udp_send() but with checksum. | |
err_t | udp_sendto (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port) |
Send data to a specified address using UDP. | |
err_t | udp_sendto_chksum (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, u8_t have_chksum, u16_t chksum) |
Same as udp_sendto(), but with checksum. | |
err_t | udp_sendto_if (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif) |
Send data to a specified address using UDP. | |
err_t | udp_sendto_if_chksum (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif, u8_t have_chksum, u16_t chksum) |
Same as udp_sendto_if(), but with checksum. | |
err_t | udp_sendto_if_src (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif, const ip_addr_t *src_ip) |
Same as udp_sendto_if, but with source address. | |
err_t | udp_sendto_if_src_chksum (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif, u8_t have_chksum, u16_t chksum, const ip_addr_t *src_ip) |
Same as udp_sendto_if_src(), but with checksum. | |
err_t | udp_bind (struct udp_pcb *pcb, const ip_addr_t *ipaddr, u16_t port) |
Bind an UDP PCB. | |
err_t | udp_connect (struct udp_pcb *pcb, const ip_addr_t *ipaddr, u16_t port) |
Connect an UDP PCB. | |
void | udp_disconnect (struct udp_pcb *pcb) |
Disconnect a UDP PCB. | |
void | udp_recv (struct udp_pcb *pcb, udp_recv_fn recv, void *recv_arg) |
Set a receive callback for a UDP PCB. | |
void | udp_remove (struct udp_pcb *pcb) |
Remove an UDP PCB. | |
struct udp_pcb * | udp_new (void) |
Create a UDP PCB. | |
struct udp_pcb * | udp_new_ip_type (u8_t type) |
Create a UDP PCB for specific IP type. | |
void | udp_netif_ip_addr_changed (const ip_addr_t *old_addr, const ip_addr_t *new_addr) |
This function is called from netif.c when address is changed. | |
void | udp_debug_print (struct udp_hdr *udphdr) |
Print UDP header information for debug purposes. |
Detailed Description
User Datagram Protocol module
The code for the User Datagram Protocol UDP & UDPLite (RFC 3828).
See also UDP
Definition in file lwip_udp.c.
Function Documentation
void udp_debug_print | ( | struct udp_hdr * | udphdr ) |
Print UDP header information for debug purposes.
- Parameters:
-
udphdr pointer to the udp header in memory.
Definition at line 1178 of file lwip_udp.c.
void udp_init | ( | void | ) |
Initialize this module.
Definition at line 87 of file lwip_udp.c.
Process an incoming UDP datagram.
Given an incoming UDP datagram (as a chain of pbufs) this function finds a corresponding UDP PCB and hands over the pbuf to the pcbs recv function. If no pcb is found or the datagram is incorrect, the pbuf is freed.
- Parameters:
-
p pbuf to be demultiplexed to a UDP PCB (p->payload pointing to the UDP header) inp network interface on which the datagram was received.
Definition at line 185 of file lwip_udp.c.
static u8_t udp_input_local_match | ( | struct udp_pcb * | pcb, |
struct netif * | inp, | ||
u8_t | broadcast | ||
) | [static] |
Common code to see if the current input packet matches the pcb (current input packet is accessed via ip(4/6)_current_* macros)
- Parameters:
-
pcb pcb to check inp network interface on which the datagram was received (only used for IPv4) broadcast 1 if his is an IPv4 broadcast (global or subnet-only), 0 otherwise (only used for IPv4)
- Returns:
- 1 on match, 0 otherwise
Definition at line 130 of file lwip_udp.c.
This function is called from netif.c when address is changed.
- Parameters:
-
old_addr IP address of the netif before change new_addr IP address of the netif after change
Definition at line 1155 of file lwip_udp.c.
static u16_t udp_new_port | ( | void | ) | [static] |
Allocate a new local UDP port.
- Returns:
- a new (free) local UDP port number
Definition at line 100 of file lwip_udp.c.
err_t udp_sendto_if_chksum | ( | struct udp_pcb * | pcb, |
struct pbuf * | p, | ||
const ip_addr_t * | dst_ip, | ||
u16_t | dst_port, | ||
struct netif * | netif, | ||
u8_t | have_chksum, | ||
u16_t | chksum | ||
) |
Same as udp_sendto_if(), but with checksum.
Definition at line 601 of file lwip_udp.c.
err_t udp_sendto_if_src_chksum | ( | struct udp_pcb * | pcb, |
struct pbuf * | p, | ||
const ip_addr_t * | dst_ip, | ||
u16_t | dst_port, | ||
struct netif * | netif, | ||
u8_t | have_chksum, | ||
u16_t | chksum, | ||
const ip_addr_t * | src_ip | ||
) |
Same as udp_sendto_if_src(), but with checksum.
Definition at line 670 of file lwip_udp.c.
Generated on Sun Jul 17 2022 08:25:35 by
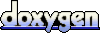