
Rtos API example
udp.h File Reference
UDP API (to be used from TCPIP thread)
See also UDP.
More...
Go to the source code of this file.
Data Structures | |
struct | udp_pcb |
the UDP protocol control block More... | |
Typedefs | |
typedef void(* | udp_recv_fn )(void *arg, struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *addr, u16_t port) |
Function prototype for udp pcb receive callback functions addr and port are in same byte order as in the pcb The callback is responsible for freeing the pbuf if it's not used any more. | |
Functions | |
struct udp_pcb * | udp_new (void) |
Create a UDP PCB. | |
struct udp_pcb * | udp_new_ip_type (u8_t type) |
Create a UDP PCB for specific IP type. | |
void | udp_remove (struct udp_pcb *pcb) |
Remove an UDP PCB. | |
err_t | udp_bind (struct udp_pcb *pcb, const ip_addr_t *ipaddr, u16_t port) |
Bind an UDP PCB. | |
err_t | udp_connect (struct udp_pcb *pcb, const ip_addr_t *ipaddr, u16_t port) |
Connect an UDP PCB. | |
void | udp_disconnect (struct udp_pcb *pcb) |
Disconnect a UDP PCB. | |
void | udp_recv (struct udp_pcb *pcb, udp_recv_fn recv, void *recv_arg) |
Set a receive callback for a UDP PCB. | |
err_t | udp_sendto_if (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif) |
Send data to a specified address using UDP. | |
err_t | udp_sendto_if_src (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif, const ip_addr_t *src_ip) |
Same as udp_sendto_if, but with source address. | |
err_t | udp_sendto (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port) |
Send data to a specified address using UDP. | |
err_t | udp_send (struct udp_pcb *pcb, struct pbuf *p) |
Send data using UDP. | |
err_t | udp_sendto_if_chksum (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif, u8_t have_chksum, u16_t chksum) |
Same as udp_sendto_if(), but with checksum. | |
err_t | udp_sendto_chksum (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, u8_t have_chksum, u16_t chksum) |
Same as udp_sendto(), but with checksum. | |
err_t | udp_send_chksum (struct udp_pcb *pcb, struct pbuf *p, u8_t have_chksum, u16_t chksum) |
Same as udp_send() but with checksum. | |
err_t | udp_sendto_if_src_chksum (struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *dst_ip, u16_t dst_port, struct netif *netif, u8_t have_chksum, u16_t chksum, const ip_addr_t *src_ip) |
Same as udp_sendto_if_src(), but with checksum. | |
void | udp_input (struct pbuf *p, struct netif *inp) |
Process an incoming UDP datagram. | |
void | udp_init (void) |
Initialize this module. | |
void | udp_debug_print (struct udp_hdr *udphdr) |
Print UDP header information for debug purposes. | |
void | udp_netif_ip_addr_changed (const ip_addr_t *old_addr, const ip_addr_t *new_addr) |
This function is called from netif.c when address is changed. |
Detailed Description
UDP API (to be used from TCPIP thread)
See also UDP.
Definition in file udp.h.
Typedef Documentation
typedef void(* udp_recv_fn)(void *arg, struct udp_pcb *pcb, struct pbuf *p, const ip_addr_t *addr, u16_t port) |
Function prototype for udp pcb receive callback functions addr and port are in same byte order as in the pcb The callback is responsible for freeing the pbuf if it's not used any more.
ATTENTION: Be aware that 'addr' might point into the pbuf 'p' so freeing this pbuf can make 'addr' invalid, too.
- Parameters:
-
arg user supplied argument (udp_pcb.recv_arg) pcb the udp_pcb which received data p the packet buffer that was received addr the remote IP address from which the packet was received port the remote port from which the packet was received
Function Documentation
void udp_debug_print | ( | struct udp_hdr * | udphdr ) |
Print UDP header information for debug purposes.
- Parameters:
-
udphdr pointer to the udp header in memory.
Definition at line 1178 of file lwip_udp.c.
void udp_init | ( | void | ) |
Initialize this module.
Definition at line 87 of file lwip_udp.c.
Process an incoming UDP datagram.
Given an incoming UDP datagram (as a chain of pbufs) this function finds a corresponding UDP PCB and hands over the pbuf to the pcbs recv function. If no pcb is found or the datagram is incorrect, the pbuf is freed.
- Parameters:
-
p pbuf to be demultiplexed to a UDP PCB (p->payload pointing to the UDP header) inp network interface on which the datagram was received.
Definition at line 185 of file lwip_udp.c.
This function is called from netif.c when address is changed.
- Parameters:
-
old_addr IP address of the netif before change new_addr IP address of the netif after change
Definition at line 1155 of file lwip_udp.c.
err_t udp_sendto_if_chksum | ( | struct udp_pcb * | pcb, |
struct pbuf * | p, | ||
const ip_addr_t * | dst_ip, | ||
u16_t | dst_port, | ||
struct netif * | netif, | ||
u8_t | have_chksum, | ||
u16_t | chksum | ||
) |
Same as udp_sendto_if(), but with checksum.
Definition at line 601 of file lwip_udp.c.
err_t udp_sendto_if_src_chksum | ( | struct udp_pcb * | pcb, |
struct pbuf * | p, | ||
const ip_addr_t * | dst_ip, | ||
u16_t | dst_port, | ||
struct netif * | netif, | ||
u8_t | have_chksum, | ||
u16_t | chksum, | ||
const ip_addr_t * | src_ip | ||
) |
Same as udp_sendto_if_src(), but with checksum.
Definition at line 670 of file lwip_udp.c.
Generated on Sun Jul 17 2022 08:25:36 by
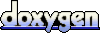