
Rtos API example
Rtos
Data Structures | |
class | ConditionVariable |
This class provides a safe way to wait for or send notifications of condition changes. More... | |
Modules | |
EventFlags class | |
Mail class | |
MemoryPool class | |
Mutex class | |
Idle hook function | |
RtosTimer class | |
Semaphore class | |
Thread class | |
Functions | |
void | wait () |
Wait for a notification. | |
bool | wait_for (uint32_t millisec) |
Wait for a notification or timeout. | |
void | notify_one () |
Notify one waiter on this condition variable that a condition changed. | |
void | notify_all () |
Notify all waiters on this condition variable that a condition changed. |
Function Documentation
void notify_all | ( | ) | [inherited] |
Notify all waiters on this condition variable that a condition changed.
- Note:
- - The thread calling this function must be the owner of the ConditionVariable's mutex
Definition at line 76 of file ConditionVariable.cpp.
void notify_one | ( | ) | [inherited] |
Notify one waiter on this condition variable that a condition changed.
- Note:
- - The thread calling this function must be the owner of the ConditionVariable's mutex
Definition at line 67 of file ConditionVariable.cpp.
void wait | ( | ) | [inherited] |
Wait for a notification.
Wait until a notification occurs.
- Note:
- - The thread calling this function must be the owner of the ConditionVariable's mutex and it must be locked exactly once
- - Spurious notifications can occur so the caller of this API should check to make sure the condition they are waiting on has been met
Example:
mutex.lock();
while (!condition_met) {
cond.wait();
}
function_to_handle_condition();
mutex.unlock();
Definition at line 41 of file ConditionVariable.cpp.
bool wait_for | ( | uint32_t | millisec ) | [inherited] |
Wait for a notification or timeout.
- Parameters:
-
millisec timeout value or osWaitForever in case of no time-out.
- Returns:
- true if a timeout occurred, false otherwise.
- Note:
- - The thread calling this function must be the owner of the ConditionVariable's mutex and it must be locked exactly once
- - Spurious notifications can occur so the caller of this API should check to make sure the condition they are waiting on has been met
Example:
mutex.lock(); Timer timer; timer.start(); bool timed_out = false; uint32_t time_left = TIMEOUT; while (!condition_met && !timed_out) { timed_out = cond.wait_for(time_left); uint32_t elapsed = timer.read_ms(); time_left = elapsed > TIMEOUT ? 0 : TIMEOUT - elapsed; } if (condition_met) { function_to_handle_condition(); } mutex.unlock();
Definition at line 46 of file ConditionVariable.cpp.
Generated on Sun Jul 17 2022 08:25:38 by
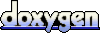