
Rtos API example
ConditionVariable Class Reference
[Rtos]
This class provides a safe way to wait for or send notifications of condition changes. More...
#include <ConditionVariable.h>
Inherits NonCopyable< ConditionVariable >.
Public Member Functions | |
ConditionVariable (Mutex &mutex) | |
Create and Initialize a ConditionVariable object. | |
void | wait () |
Wait for a notification. | |
bool | wait_for (uint32_t millisec) |
Wait for a notification or timeout. | |
void | notify_one () |
Notify one waiter on this condition variable that a condition changed. | |
void | notify_all () |
Notify all waiters on this condition variable that a condition changed. | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
This class provides a safe way to wait for or send notifications of condition changes.
This class is used in conjunction with a mutex to safely wait for or notify waiters of condition changes to a resource accessible by multiple threads.
# Defined behavior
- All threads waiting on the condition variable wake when ConditionVariable::notify_all is called.
- If one or more threads are waiting on the condition variable at least one of them wakes when ConditionVariable::notify is called.
# Undefined behavior
- The thread which is unblocked on ConditionVariable::notify_one is undefined if there are multiple waiters.
- The order which in which waiting threads acquire the condition variable's mutex after ConditionVariable::notify_all is called is undefined.
- When ConditionVariable::notify_one or ConditionVariable::notify_all is called and there are one or more waiters and one or more threads attempting to acquire the condition variable's mutex the order in which the mutex is acquired is undefined.
- The behavior of ConditionVariable::wait and ConditionVariable::wait_for is undefined if the condition variable's mutex is locked more than once by the calling thread.
- Spurious notifications (not triggered by the application) can occur and it is not defined when these occur.
- Note:
- Synchronization level: Thread safe
Example:
#include "mbed.h" Mutex mutex; ConditionVariable cond(mutex); // These variables are protected by locking mutex uint32_t count = 0; bool done = false; void worker_thread() { mutex.lock(); do { printf("Worker: Count %lu\r\n", count); // Wait for a condition to change cond.wait(); } while (!done); printf("Worker: Exiting\r\n"); mutex.unlock(); } int main() { Thread thread; thread.start(worker_thread); for (int i = 0; i < 5; i++) { mutex.lock(); // Change count and notify waiters of this count++; printf("Main: Set count to %lu\r\n", count); cond.notify_all(); mutex.unlock(); wait(1.0); } mutex.lock(); // Change done and notify waiters of this done = true; printf("Main: Set done\r\n"); cond.notify_all(); mutex.unlock(); thread.join(); }
Definition at line 119 of file ConditionVariable.h.
Constructor & Destructor Documentation
ConditionVariable | ( | Mutex & | mutex ) |
Create and Initialize a ConditionVariable object.
Definition at line 36 of file ConditionVariable.cpp.
Generated on Sun Jul 17 2022 08:25:44 by
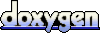