
Rtos API example
dhcp_service_api.h File Reference
DHCP server connection interfaces. More...
Go to the source code of this file.
Typedefs | |
typedef enum dhcp_instance_type | dhcp_instance_type_e |
/enum dhcp_instance_type /brief DHCP instance types. | |
typedef int( | dhcp_service_receive_req_cb )(uint16_t instance_id, uint32_t msg_tr_id, uint8_t msg_name, uint8_t *msg_ptr, uint16_t msg_len) |
DHCP Service receive callback. | |
typedef int( | dhcp_service_receive_resp_cb )(uint16_t instance_id, void *ptr, uint8_t msg_name, uint8_t *msg_ptr, uint16_t msg_len) |
DHCP Service Message Response callback. | |
Enumerations | |
enum | dhcp_instance_type |
/enum dhcp_instance_type /brief DHCP instance types. More... | |
Functions | |
uint16_t | dhcp_service_init (int8_t interface_id, dhcp_instance_type_e instance_type, dhcp_service_receive_req_cb *receive_req_cb) |
Initialize a new DHCP service instance. | |
void | dhcp_service_delete (uint16_t instance) |
Deletes a server instance. | |
int | dhcp_service_send_resp (uint32_t msg_tr_id, uint8_t options, uint8_t *msg_ptr, uint16_t msg_len) |
Sends a DHCP response message. | |
uint32_t | dhcp_service_send_req (uint16_t instance_id, uint8_t options, void *ptr, const uint8_t addr[static 16], uint8_t *msg_ptr, uint16_t msg_len, dhcp_service_receive_resp_cb *receive_resp_cb) |
Sends DHCP request message. | |
void | dhcp_service_set_retry_timers (uint32_t msg_tr_id, uint16_t timeout_init, uint16_t timeout_max, uint8_t retrans_max) |
Setting retransmission parameters. | |
void | dhcp_service_req_remove (uint32_t msg_tr_id) |
Stops transactions for a message (retransmissions). | |
bool | dhcp_service_timer_tick (uint16_t ticks) |
Timer tick function for retransmissions. |
Detailed Description
DHCP server connection interfaces.
DHCP Service Instance
- dhcp_service_init(), Initializes a DHCP service.
- dhcp_service_delete(), Removes the DHCP service.
DHCP Service Messages
- dhcp_service_send_req(), Sends out DHCP request messages.
- dhcp_service_send_resp(), Sends out DHCP response messages.
DHCP Service Timers (retry timers)
- dhcp_service_send_req(), Sends out DHCP request messages.
- dhcp_service_set_retry_timers(), Sets the retransmission parameters.
- dhcp_service_req_remove(), Stops retrying and retransmissions.
- dhcp_service_timer_tick(), Indicates if a timeout occurred.
Definition in file dhcp_service_api.h.
Typedef Documentation
typedef enum dhcp_instance_type dhcp_instance_type_e |
/enum dhcp_instance_type /brief DHCP instance types.
typedef int( dhcp_service_receive_req_cb)(uint16_t instance_id, uint32_t msg_tr_id, uint8_t msg_name, uint8_t *msg_ptr, uint16_t msg_len) |
DHCP Service receive callback.
When the DHCP service receives a DHCP message it will go through a list of registered DHCP services instances until some instance acknowledges that the message belongs to it.
- Parameters:
-
instance_id An instance of registered server. msg_tr_id The message transaction ID. msg_name Message type. msg_ptr An allocated message pointer. Should not deallocate unless RET_MSG_ACCEPTED returned (then responsibility of client). msg_len The length of the message.
Return values
- Returns:
- RET_MSG_ACCEPTED - Message is handled.
- RET_MSG_CORRUPTED - Message is corrupted.
- RET_MSG_NOT_MINE - Message belongs to someone else.
Definition at line 89 of file dhcp_service_api.h.
typedef int( dhcp_service_receive_resp_cb)(uint16_t instance_id, void *ptr, uint8_t msg_name, uint8_t *msg_ptr, uint16_t msg_len) |
DHCP Service Message Response callback.
When the DHCP service receives a response to a DHCP message, this callback receives it.
- Parameters:
-
instance_id An instance of a registered server. ptr A pointer for the client object. msg_name Message type. msg_ptr An allocated message pointer. Should not deallocate unless RET_MSG_ACCEPTED returned (then responsibility of client). msg_len The length of the message.
Return values
- Returns:
- RET_MSG_ACCEPTED - Message is handled
- RET_MSG_WAIT_ANOTHER - This message was not the last one for this transaction and a new reply is expected.
Definition at line 107 of file dhcp_service_api.h.
Enumeration Type Documentation
enum dhcp_instance_type |
/enum dhcp_instance_type /brief DHCP instance types.
Definition at line 66 of file dhcp_service_api.h.
Function Documentation
void dhcp_service_delete | ( | uint16_t | instance ) |
Deletes a server instance.
Removes all data related to this instance.
- Parameters:
-
instance The instance ID of the registered server.
uint16_t dhcp_service_init | ( | int8_t | interface_id, |
dhcp_instance_type_e | instance_type, | ||
dhcp_service_receive_req_cb * | receive_req_cb | ||
) |
Initialize a new DHCP service instance.
Creates and shares the socket for other DHCP services.
- Parameters:
-
interface_id Interface for the new DHCP instance. instance_type The type of the new DHCP instance. receive_req_cb A callback function to receive DHCP messages.
- Returns:
- Instance ID that is used to identify the service.
void dhcp_service_req_remove | ( | uint32_t | msg_tr_id ) |
Stops transactions for a message (retransmissions).
Clears off sending retransmissions for a particular message transaction by finding it via its message transaction ID.
- Parameters:
-
msg_tr_id The message transaction ID.
uint32_t dhcp_service_send_req | ( | uint16_t | instance_id, |
uint8_t | options, | ||
void * | ptr, | ||
const uint8_t | addr[static 16], | ||
uint8_t * | msg_ptr, | ||
uint16_t | msg_len, | ||
dhcp_service_receive_resp_cb * | receive_resp_cb | ||
) |
Sends DHCP request message.
Service takes care of retransmissions.
- Parameters:
-
instance_id The instance ID of the registered server. options Options for this request. ptr A void pointer to the client object. addr The address of the server. msg_ptr An allocated message pointer. This pointer is the responsibility of the service after this call. msg_len The length of the message. receive_resp_cb Callback pointer
- Returns:
- Transaction ID of the DHCP transaction
- 0, if error occurred.
int dhcp_service_send_resp | ( | uint32_t | msg_tr_id, |
uint8_t | options, | ||
uint8_t * | msg_ptr, | ||
uint16_t | msg_len | ||
) |
Sends a DHCP response message.
- Parameters:
-
msg_tr_id The message transaction ID. options Options for this request. msg_ptr An allocated message pointer. Should not deallocate unless RET_MSG_ACCEPTED returned (then responsibility of client). msg_len The length of the message.
- Returns:
- 0, if everything went fine.
- -1, if error occurred.
void dhcp_service_set_retry_timers | ( | uint32_t | msg_tr_id, |
uint16_t | timeout_init, | ||
uint16_t | timeout_max, | ||
uint8_t | retrans_max | ||
) |
Setting retransmission parameters.
Sets the retransmission parameters for this transaction.
- Parameters:
-
msg_tr_id The message transaction ID. timeout_init An initial timeout value. timeout_max The maximum timeout value when initial timeout is doubled with every retry. retrans_max The maximum number of retries after which an error is received.
bool dhcp_service_timer_tick | ( | uint16_t | ticks ) |
Timer tick function for retransmissions.
Retransmission timer ticks should be increased with 100ms interval, if necessary. One tick is one millisecond.
Generated on Sun Jul 17 2022 08:25:34 by
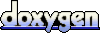