
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
dhcp_service_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2013-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 #ifndef DHCP_SERVICE_API_H_ 00016 #define DHCP_SERVICE_API_H_ 00017 00018 #include <ns_types.h> 00019 /** 00020 * \file dhcp_service_api.h 00021 * \brief DHCP server connection interfaces 00022 * 00023 * \section dhcp-service DHCP Service Instance 00024 * - dhcp_service_init(), Initializes a DHCP service. 00025 * - dhcp_service_delete(), Removes the DHCP service. 00026 * 00027 * \section dhcp-msg DHCP Service Messages 00028 * - dhcp_service_send_req(), Sends out DHCP request messages. 00029 * - dhcp_service_send_resp(), Sends out DHCP response messages. 00030 * 00031 * \section dhcp-tim DHCP Service Timers (retry timers) 00032 * - dhcp_service_send_req(), Sends out DHCP request messages. 00033 * - dhcp_service_set_retry_timers(), Sets the retransmission parameters. 00034 * - dhcp_service_req_remove(), Stops retrying and retransmissions. 00035 * - dhcp_service_timer_tick(), Indicates if a timeout occurred. 00036 * 00037 */ 00038 00039 /** Defines Debug Trace String for DHCP service */ 00040 #define DHCP_SERVICE_API_TRACE_STR "DHcS" 00041 00042 /* 00043 * Return values for callbacks 00044 */ 00045 00046 /** Message belongs to someone else. */ 00047 #define RET_MSG_NOT_MINE 0 00048 /** Message is handled. */ 00049 #define RET_MSG_ACCEPTED 1 00050 /** Message is not the final one and needs to hold on a bit. */ 00051 #define RET_MSG_WAIT_ANOTHER -1 00052 /** Message is unexpected or corrupted. */ 00053 #define RET_MSG_CORRUPTED -2 00054 00055 /** \name DHCP options */ 00056 ///@{ 00057 #define TX_OPT_NONE 0x00 /**< No options. */ 00058 #define TX_OPT_USE_SHORT_ADDR 0x01 /**< Use short addresses. */ 00059 #define TX_OPT_MULTICAST_HOP_LIMIT_64 0x02 /**< Use multicast hop limit of 64. */ 00060 ///@} 00061 00062 /** 00063 * /enum dhcp_instance_type 00064 * /brief DHCP instance types. 00065 */ 00066 typedef enum dhcp_instance_type 00067 { 00068 DHCP_INSTANCE_CLIENT, 00069 DHCP_INSTANCE_SERVER 00070 } dhcp_instance_type_e; 00071 00072 /** 00073 * \brief DHCP Service receive callback. 00074 * 00075 * When the DHCP service receives a DHCP message it will go through a list of registered DHCP services instances 00076 * until some instance acknowledges that the message belongs to it. 00077 * \param instance_id An instance of registered server. 00078 * \param msg_tr_id The message transaction ID. 00079 * \param msg_name Message type. 00080 * \param msg_ptr An allocated message pointer. Should not deallocate unless RET_MSG_ACCEPTED returned (then responsibility of client). 00081 * \param msg_len The length of the message. 00082 * 00083 * Return values 00084 * \return RET_MSG_ACCEPTED - Message is handled. 00085 * \return RET_MSG_CORRUPTED - Message is corrupted. 00086 * \return RET_MSG_NOT_MINE - Message belongs to someone else. 00087 */ 00088 00089 typedef int (dhcp_service_receive_req_cb)(uint16_t instance_id, uint32_t msg_tr_id, uint8_t msg_name, uint8_t *msg_ptr, uint16_t msg_len); 00090 00091 /** 00092 * \brief DHCP Service Message Response callback. 00093 * 00094 * When the DHCP service receives a response to a DHCP message, this callback receives it. 00095 * 00096 * \param instance_id An instance of a registered server. 00097 * \param ptr A pointer for the client object. 00098 * \param msg_name Message type. 00099 * \param msg_ptr An allocated message pointer. Should not deallocate unless RET_MSG_ACCEPTED returned (then responsibility of client). 00100 * \param msg_len The length of the message. 00101 * 00102 * Return values 00103 * \return RET_MSG_ACCEPTED - Message is handled 00104 * \return RET_MSG_WAIT_ANOTHER - This message was not the last one for this transaction and a new reply is expected. 00105 */ 00106 00107 typedef int (dhcp_service_receive_resp_cb)(uint16_t instance_id, void *ptr, uint8_t msg_name, uint8_t *msg_ptr, uint16_t msg_len); 00108 00109 00110 /** 00111 * \brief Initialize a new DHCP service instance. 00112 * 00113 * Creates and shares the socket for other DHCP services. 00114 * 00115 * \param interface_id Interface for the new DHCP instance. 00116 * \param instance_type The type of the new DHCP instance. 00117 * \param receive_req_cb A callback function to receive DHCP messages. 00118 * 00119 * \return Instance ID that is used to identify the service. 00120 */ 00121 00122 uint16_t dhcp_service_init(int8_t interface_id, dhcp_instance_type_e instance_type, dhcp_service_receive_req_cb *receive_req_cb); 00123 00124 /** 00125 * \brief Deletes a server instance. 00126 * 00127 * Removes all data related to this instance. 00128 * 00129 * \param instance The instance ID of the registered server. 00130 */ 00131 void dhcp_service_delete(uint16_t instance); 00132 00133 /** 00134 * \brief Sends a DHCP response message. 00135 * 00136 * \param msg_tr_id The message transaction ID. 00137 * \param options Options for this request. 00138 * \param msg_ptr An allocated message pointer. Should not deallocate unless RET_MSG_ACCEPTED returned (then responsibility of client). 00139 * \param msg_len The length of the message. 00140 * 00141 * \return 0, if everything went fine. 00142 * \return -1, if error occurred. 00143 */ 00144 int dhcp_service_send_resp(uint32_t msg_tr_id, uint8_t options, uint8_t *msg_ptr, uint16_t msg_len); 00145 00146 00147 /** 00148 * \brief Sends DHCP request message. 00149 * 00150 * Service takes care of retransmissions. 00151 * 00152 * \param instance_id The instance ID of the registered server. 00153 * \param options Options for this request. 00154 * \param ptr A void pointer to the client object. 00155 * \param addr The address of the server. 00156 * \param msg_ptr An allocated message pointer. This pointer is the responsibility of the service after this call. 00157 * \param msg_len The length of the message. 00158 * \param receive_resp_cb Callback pointer 00159 * 00160 * \return Transaction ID of the DHCP transaction 00161 * \return 0, if error occurred. 00162 */ 00163 uint32_t dhcp_service_send_req(uint16_t instance_id, uint8_t options, void *ptr, const uint8_t addr[static 16], uint8_t *msg_ptr, uint16_t msg_len, dhcp_service_receive_resp_cb *receive_resp_cb); 00164 00165 /** 00166 * \brief Setting retransmission parameters. 00167 * 00168 * Sets the retransmission parameters for this transaction. 00169 * 00170 * \param msg_tr_id The message transaction ID. 00171 * \param timeout_init An initial timeout value. 00172 * \param timeout_max The maximum timeout value when initial timeout is doubled with every retry. 00173 * \param retrans_max The maximum number of retries after which an error is received. 00174 * 00175 */ 00176 void dhcp_service_set_retry_timers(uint32_t msg_tr_id, uint16_t timeout_init, uint16_t timeout_max, uint8_t retrans_max); 00177 00178 /** 00179 * \brief Stops transactions for a message (retransmissions). 00180 * 00181 * Clears off sending retransmissions for a particular message transaction by finding it via its message transaction ID. 00182 * 00183 * \param msg_tr_id The message transaction ID. 00184 * 00185 */ 00186 void dhcp_service_req_remove(uint32_t msg_tr_id); 00187 00188 /** 00189 * \brief Timer tick function for retransmissions. 00190 * 00191 * Retransmission timer ticks should be increased with 100ms interval, if necessary. One tick is one millisecond. 00192 * 00193 */ 00194 bool dhcp_service_timer_tick(uint16_t ticks); 00195 00196 00197 #endif //DHCP_SERVICE_API_H_
Generated on Sun Jul 17 2022 08:25:21 by
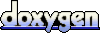