
Rtos API example
RtosTimer Class Reference
[RtosTimer class]
The RtosTimer class allow creating and and controlling of timer functions in the system. More...
#include <RtosTimer.h>
Inherits NonCopyable< RtosTimer >.
Public Member Functions | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Replaced with RtosTimer(Callback<void()>, os_timer_type)") MBED_DEPRECATED_SINCE("mbed-os-5.2" | |
Create timer. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.2","The RtosTimer has been superseded by the EventQueue. See RtosTimer.h for more details") RtosTimer(mbed | |
Create timer. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The RtosTimer constructor does not support cv-qualifiers. Replaced by ""RtosTimer(callback(obj, method), os_timer_type).") MBED_DEPRECATED_SINCE("mbed-os-5.2" | |
Create timer. | |
osStatus | stop (void) |
Stop the timer. | |
osStatus | start (uint32_t millisec) |
Start or restart the timer. | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
The RtosTimer class allow creating and and controlling of timer functions in the system.
A timer function is called when a time period expires whereby both on-shot and periodic timers are possible. A timer can be started, restarted, or stopped.
Timers are handled in the thread osTimerThread. Callback functions run under control of this thread and may use CMSIS-RTOS API calls.
For an example, the following code shows a simple use of the RtosTimer:
DigitalOut led(LED1); void blink() { led = !led; } RtosTimer timer(&blink); int main() { timer.start(1000); // call blink every 1s wait_ms(5000); timer.stop(); // stop after 5s }
This is the above example rewritten to use the EventQueue:
DigitalOut led(LED1); void blink() { led = !led; } EventQueue queue(4*EVENTS_EVENT_SIZE); int main() { int blink_id = queue.call_every(1000, &blink); // call blink every 1s queue.dispatch(5000); queue.cancel(blink_id); // stop after 5s }
- Note:
- Memory considerations: The timer control structures will be created on current thread's stack, both for the mbed OS and underlying RTOS objects (static or dynamic RTOS memory pools are not being used).
Definition at line 87 of file RtosTimer.h.
Member Function Documentation
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Replaced with RtosTimer(Callback<void()>, os_timer_type)" | |||
) |
Create timer.
- Parameters:
-
func function to be executed by this timer. type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic) argument argument to the timer call back function. (default: NULL)
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.2" | , |
"The RtosTimer has been superseded by the EventQueue. See RtosTimer.h for more details" | |||
) |
Create timer.
- Parameters:
-
func function to be executed by this timer. type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic)
Definition at line 111 of file RtosTimer.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The RtosTimer constructor does not support cv-qualifiers. Replaced by ""RtosTimer(callback(obj, method), os_timer_type)." | |||
) |
Create timer.
- Parameters:
-
obj pointer to the object to call the member function on. method member function to be executed by this timer. type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic)
osStatus start | ( | uint32_t | millisec ) |
Start or restart the timer.
- Parameters:
-
millisec non-zero value of the timer.
- Returns:
- status code that indicates the execution status of the function: osOK the timer has been started or restarted. osErrorISR start cannot be called from interrupt service routines. osErrorParameter internal error or incorrect parameter value. osErrorResource internal error (the timer is in an invalid timer state).
Definition at line 41 of file RtosTimer.cpp.
osStatus stop | ( | void | ) |
Stop the timer.
- Returns:
- status code that indicates the execution status of the function: osOK the timer has been stopped. osErrorISR stop cannot be called from interrupt service routines. osErrorParameter internal error. osErrorResource the timer is not running.
Definition at line 45 of file RtosTimer.cpp.
Generated on Sun Jul 17 2022 08:25:44 by
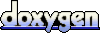