
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
RtosTimer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2012 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef RTOS_TIMER_H 00023 #define RTOS_TIMER_H 00024 00025 #include <stdint.h> 00026 #include "cmsis_os2.h" 00027 #include "mbed_rtos_storage.h" 00028 #include "platform/Callback.h" 00029 #include "platform/NonCopyable.h" 00030 #include "platform/mbed_toolchain.h" 00031 #include "mbed_rtos1_types.h" 00032 00033 namespace rtos { 00034 /** \addtogroup rtos */ 00035 /** @{*/ 00036 /** 00037 * \defgroup rtos_RtosTimer RtosTimer class 00038 * @{ 00039 */ 00040 00041 /** The RtosTimer class allow creating and and controlling of timer functions in the system. 00042 A timer function is called when a time period expires whereby both on-shot and 00043 periodic timers are possible. A timer can be started, restarted, or stopped. 00044 00045 Timers are handled in the thread osTimerThread. 00046 Callback functions run under control of this thread and may use CMSIS-RTOS API calls. 00047 00048 @deprecated 00049 The RtosTimer has been superseded by the EventQueue. The RtosTimer and EventQueue duplicate 00050 the functionality of timing events outside of interrupt context, however the EventQueue 00051 has additional features to handle deferring other events to multiple contexts. 00052 00053 For an example, the following code shows a simple use of the RtosTimer: 00054 @code 00055 DigitalOut led(LED1); 00056 void blink() { 00057 led = !led; 00058 } 00059 00060 RtosTimer timer(&blink); 00061 int main() { 00062 timer.start(1000); // call blink every 1s 00063 wait_ms(5000); 00064 timer.stop(); // stop after 5s 00065 } 00066 @endcode 00067 00068 This is the above example rewritten to use the EventQueue: 00069 @code 00070 DigitalOut led(LED1); 00071 void blink() { 00072 led = !led; 00073 } 00074 00075 EventQueue queue(4*EVENTS_EVENT_SIZE); 00076 int main() { 00077 int blink_id = queue.call_every(1000, &blink); // call blink every 1s 00078 queue.dispatch(5000); 00079 queue.cancel(blink_id); // stop after 5s 00080 } 00081 @endcode 00082 00083 @note 00084 Memory considerations: The timer control structures will be created on current thread's stack, both for the mbed OS 00085 and underlying RTOS objects (static or dynamic RTOS memory pools are not being used). 00086 */ 00087 class RtosTimer : private mbed::NonCopyable<RtosTimer> { 00088 public: 00089 /** Create timer. 00090 @param func function to be executed by this timer. 00091 @param type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic) 00092 @param argument argument to the timer call back function. (default: NULL) 00093 @deprecated Replaced with RtosTimer(Callback<void()>, os_timer_type) 00094 @deprecated 00095 The RtosTimer has been superseded by the EventQueue. See RtosTimer.h for more details 00096 */ 00097 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00098 "Replaced with RtosTimer(Callback<void()>, os_timer_type)") 00099 MBED_DEPRECATED_SINCE("mbed-os-5.2", 00100 "The RtosTimer has been superseded by the EventQueue. See RtosTimer.h for more details") 00101 RtosTimer(void (*func)(void const *argument), os_timer_type type=osTimerPeriodic, void *argument=NULL) { 00102 constructor(mbed::callback((void (*)(void *))func, argument), type); 00103 } 00104 00105 /** Create timer. 00106 @param func function to be executed by this timer. 00107 @param type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic) 00108 @deprecated 00109 The RtosTimer has been superseded by the EventQueue. See RtosTimer.h for more details 00110 */ 00111 MBED_DEPRECATED_SINCE("mbed-os-5.2", 00112 "The RtosTimer has been superseded by the EventQueue. See RtosTimer.h for more details") 00113 RtosTimer(mbed::Callback<void()> func, os_timer_type type=osTimerPeriodic) { 00114 constructor(func, type); 00115 } 00116 00117 /** Create timer. 00118 @param obj pointer to the object to call the member function on. 00119 @param method member function to be executed by this timer. 00120 @param type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic) 00121 @deprecated 00122 The RtosTimer constructor does not support cv-qualifiers. Replaced by 00123 RtosTimer(callback(obj, method), os_timer_type). 00124 @deprecated 00125 The RtosTimer has been superseded by the EventQueue. See RtosTimer.h for more details 00126 */ 00127 template <typename T, typename M> 00128 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00129 "The RtosTimer constructor does not support cv-qualifiers. Replaced by " 00130 "RtosTimer(callback(obj, method), os_timer_type).") 00131 MBED_DEPRECATED_SINCE("mbed-os-5.2", 00132 "The RtosTimer has been superseded by the EventQueue. See RtosTimer.h for more details") 00133 RtosTimer(T *obj, M method, os_timer_type type=osTimerPeriodic) { 00134 constructor(mbed::callback(obj, method), type); 00135 } 00136 00137 /** Stop the timer. 00138 @return status code that indicates the execution status of the function: 00139 @a osOK the timer has been stopped. 00140 @a osErrorISR @a stop cannot be called from interrupt service routines. 00141 @a osErrorParameter internal error. 00142 @a osErrorResource the timer is not running. 00143 */ 00144 osStatus stop(void); 00145 00146 /** Start or restart the timer. 00147 @param millisec non-zero value of the timer. 00148 @return status code that indicates the execution status of the function: 00149 @a osOK the timer has been started or restarted. 00150 @a osErrorISR @a start cannot be called from interrupt service routines. 00151 @a osErrorParameter internal error or incorrect parameter value. 00152 @a osErrorResource internal error (the timer is in an invalid timer state). 00153 */ 00154 osStatus start(uint32_t millisec); 00155 00156 ~RtosTimer(); 00157 00158 private: 00159 // Required to share definitions without 00160 // delegated constructors 00161 void constructor(mbed::Callback<void()> func, os_timer_type type); 00162 00163 osTimerId_t _id; 00164 mbed_rtos_storage_timer_t _obj_mem; 00165 mbed::Callback<void()> _function; 00166 }; 00167 /** @}*/ 00168 /** @}*/ 00169 00170 } 00171 00172 #endif 00173 00174
Generated on Sun Jul 17 2022 08:25:29 by
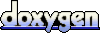