
Rtos API example
Mutex Class Reference
[Mutex class]
The Mutex class is used to synchronize the execution of threads. More...
#include <Mutex.h>
Inherits NonCopyable< Mutex >.
Public Member Functions | |
Mutex () | |
Create and Initialize a Mutex object. | |
Mutex (const char *name) | |
Create and Initialize a Mutex object. | |
osStatus | lock (uint32_t millisec=osWaitForever) |
Wait until a Mutex becomes available. | |
bool | trylock () |
Try to lock the mutex, and return immediately. | |
osStatus | unlock () |
Unlock the mutex that has previously been locked by the same thread. | |
osThreadId | get_owner () |
Get the owner the this mutex. | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
The Mutex class is used to synchronize the execution of threads.
This is for example used to protect access to a shared resource.
- Note:
- Memory considerations: The mutex control structures will be created on current thread's stack, both for the mbed OS and underlying RTOS objects (static or dynamic RTOS memory pools are not being used).
Definition at line 47 of file Mutex.h.
Constructor & Destructor Documentation
Mutex | ( | const char * | name ) |
Member Function Documentation
osThreadId get_owner | ( | ) |
osStatus lock | ( | uint32_t | millisec = osWaitForever ) |
Wait until a Mutex becomes available.
- Parameters:
-
millisec timeout value or 0 in case of no time-out. (default: osWaitForever)
- Returns:
- status code that indicates the execution status of the function: osOK the mutex has been obtained. osErrorTimeout the mutex could not be obtained in the given time. osErrorParameter internal error. osErrorResource the mutex could not be obtained when no timeout was specified. osErrorISR this function cannot be called from the interrupt service routine.
bool trylock | ( | ) |
osStatus unlock | ( | void | ) |
Unlock the mutex that has previously been locked by the same thread.
- Returns:
- status code that indicates the execution status of the function: osOK the mutex has been released. osErrorParameter internal error. osErrorResource the mutex was not locked or the current thread wasn't the owner. osErrorISR this function cannot be called from the interrupt service routine.
Generated on Sun Jul 17 2022 08:25:44 by
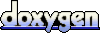