
Rtos API example
EthernetInterface Class Reference
EthernetInterface class Implementation of the NetworkStack for LWIP. More...
#include <EthernetInterface.h>
Inherits EthInterface.
Public Member Functions | |
EthernetInterface () | |
EthernetInterface lifetime. | |
virtual nsapi_error_t | set_network (const char *ip_address, const char *netmask, const char *gateway) |
Set a static IP address. | |
virtual nsapi_error_t | set_dhcp (bool dhcp) |
Enable or disable DHCP on the network. | |
virtual nsapi_error_t | connect () |
Start the interface. | |
virtual nsapi_error_t | disconnect () |
Stop the interface. | |
virtual const char * | get_mac_address () |
Get the local MAC address. | |
virtual const char * | get_ip_address () |
Get the local IP address. | |
virtual const char * | get_netmask () |
Get the local network mask. | |
virtual const char * | get_gateway () |
Get the local gateways. | |
virtual nsapi_error_t | gethostbyname (const char *host, SocketAddress *address, nsapi_version_t version=NSAPI_UNSPEC) |
Translates a hostname to an IP address with specific version. | |
virtual nsapi_error_t | add_dns_server (const SocketAddress &address) |
Add a domain name server to list of servers to query. | |
Protected Member Functions | |
virtual NetworkStack * | get_stack () |
Provide access to the underlying stack. | |
Friends | |
class | Socket |
class | UDPSocket |
class | TCPSocket |
Detailed Description
EthernetInterface class Implementation of the NetworkStack for LWIP.
Definition at line 31 of file EthernetInterface.h.
Constructor & Destructor Documentation
EthernetInterface lifetime.
Definition at line 22 of file EthernetInterface.cpp.
Member Function Documentation
nsapi_error_t add_dns_server | ( | const SocketAddress & | address ) | [virtual, inherited] |
Add a domain name server to list of servers to query.
- Parameters:
-
address Destination for the host address
- Returns:
- 0 on success, negative error code on failure
Definition at line 63 of file NetworkInterface.cpp.
nsapi_error_t connect | ( | ) | [virtual] |
Start the interface.
- Returns:
- 0 on success, negative on failure
Definition at line 47 of file EthernetInterface.cpp.
nsapi_error_t disconnect | ( | ) | [virtual] |
Stop the interface.
- Returns:
- 0 on success, negative on failure
Definition at line 56 of file EthernetInterface.cpp.
const char * get_gateway | ( | ) | [virtual] |
Get the local gateways.
- Returns:
- Null-terminated representation of the local gateway or null if no network mask has been recieved
Definition at line 84 of file EthernetInterface.cpp.
const char * get_ip_address | ( | ) | [virtual] |
Get the local IP address.
- Returns:
- Null-terminated representation of the local IP address or null if no IP address has been recieved
Definition at line 66 of file EthernetInterface.cpp.
const char * get_mac_address | ( | ) | [virtual] |
Get the local MAC address.
Provided MAC address is intended for info or debug purposes and may not be provided if the underlying network interface does not provide a MAC address
- Returns:
- Null-terminated representation of the local MAC address or null if no MAC address is available
Definition at line 61 of file EthernetInterface.cpp.
const char * get_netmask | ( | ) | [virtual] |
Get the local network mask.
- Returns:
- Null-terminated representation of the local network mask or null if no network mask has been recieved
Definition at line 75 of file EthernetInterface.cpp.
NetworkStack * get_stack | ( | void | ) | [protected, virtual] |
Provide access to the underlying stack.
- Returns:
- The underlying network stack
Definition at line 93 of file EthernetInterface.cpp.
nsapi_error_t gethostbyname | ( | const char * | host, |
SocketAddress * | address, | ||
nsapi_version_t | version = NSAPI_UNSPEC |
||
) | [virtual, inherited] |
Translates a hostname to an IP address with specific version.
The hostname may be either a domain name or an IP address. If the hostname is an IP address, no network transactions will be performed.
If no stack-specific DNS resolution is provided, the hostname will be resolve using a UDP socket on the stack.
- Parameters:
-
address Destination for the host SocketAddress host Hostname to resolve version IP version of address to resolve, NSAPI_UNSPEC indicates version is chosen by the stack (defaults to NSAPI_UNSPEC)
- Returns:
- 0 on success, negative error code on failure
Definition at line 58 of file NetworkInterface.cpp.
nsapi_error_t set_dhcp | ( | bool | dhcp ) | [virtual] |
Enable or disable DHCP on the network.
Requires that the network is disconnected
- Parameters:
-
dhcp False to disable dhcp (defaults to enabled)
- Returns:
- 0 on success, negative error code on failure
Definition at line 41 of file EthernetInterface.cpp.
nsapi_error_t set_network | ( | const char * | ip_address, |
const char * | netmask, | ||
const char * | gateway | ||
) | [virtual] |
Set a static IP address.
Configures this network interface to use a static IP address. Implicitly disables DHCP, which can be enabled in set_dhcp. Requires that the network is disconnected.
- Parameters:
-
address Null-terminated representation of the local IP address netmask Null-terminated representation of the local network mask gateway Null-terminated representation of the local gateway
- Returns:
- 0 on success, negative error code on failure
Definition at line 27 of file EthernetInterface.cpp.
Generated on Sun Jul 17 2022 08:25:40 by
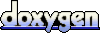