
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
EthernetInterface.cpp
00001 /* LWIP implementation of NetworkInterfaceAPI 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "EthernetInterface.h" 00018 #include "lwip_stack.h" 00019 00020 00021 /* Interface implementation */ 00022 EthernetInterface::EthernetInterface() 00023 : _dhcp(true), _ip_address(), _netmask(), _gateway() 00024 { 00025 } 00026 00027 nsapi_error_t EthernetInterface::set_network(const char *ip_address, const char *netmask, const char *gateway) 00028 { 00029 _dhcp = false; 00030 00031 strncpy(_ip_address, ip_address ? ip_address : "", sizeof(_ip_address)); 00032 _ip_address[sizeof(_ip_address) - 1] = '\0'; 00033 strncpy(_netmask, netmask ? netmask : "", sizeof(_netmask)); 00034 _netmask[sizeof(_netmask) - 1] = '\0'; 00035 strncpy(_gateway, gateway ? gateway : "", sizeof(_gateway)); 00036 _gateway[sizeof(_gateway) - 1] = '\0'; 00037 00038 return NSAPI_ERROR_OK ; 00039 } 00040 00041 nsapi_error_t EthernetInterface::set_dhcp(bool dhcp) 00042 { 00043 _dhcp = dhcp; 00044 return NSAPI_ERROR_OK ; 00045 } 00046 00047 nsapi_error_t EthernetInterface::connect() 00048 { 00049 return mbed_lwip_bringup_2(_dhcp, false, 00050 _ip_address[0] ? _ip_address : 0, 00051 _netmask[0] ? _netmask : 0, 00052 _gateway[0] ? _gateway : 0, 00053 DEFAULT_STACK); 00054 } 00055 00056 nsapi_error_t EthernetInterface::disconnect() 00057 { 00058 return mbed_lwip_bringdown_2(false); 00059 } 00060 00061 const char *EthernetInterface::get_mac_address() 00062 { 00063 return mbed_lwip_get_mac_address(); 00064 } 00065 00066 const char *EthernetInterface::get_ip_address() 00067 { 00068 if (mbed_lwip_get_ip_address(_ip_address, sizeof _ip_address)) { 00069 return _ip_address; 00070 } 00071 00072 return NULL; 00073 } 00074 00075 const char *EthernetInterface::get_netmask() 00076 { 00077 if (mbed_lwip_get_netmask(_netmask, sizeof _netmask)) { 00078 return _netmask; 00079 } 00080 00081 return 0; 00082 } 00083 00084 const char *EthernetInterface::get_gateway() 00085 { 00086 if (mbed_lwip_get_gateway(_gateway, sizeof _gateway)) { 00087 return _gateway; 00088 } 00089 00090 return 0; 00091 } 00092 00093 NetworkStack *EthernetInterface::get_stack() 00094 { 00095 return nsapi_create_stack(&lwip_stack); 00096 }
Generated on Sun Jul 17 2022 08:25:22 by
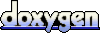