
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
PalGap.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_PAL_GAP_H_ 00018 #define BLE_PAL_GAP_H_ 00019 00020 #include "platform/Callback.h" 00021 #include "GapTypes.h" 00022 #include "GapEvents.h" 00023 00024 namespace ble { 00025 namespace pal { 00026 00027 /** 00028 * Adaptation interface for the GAP layer. 00029 * 00030 * Define the primitive necessary to realize GAP operations. the API and event 00031 * follow closely the definition of the HCI commands and events used 00032 * by that layer. 00033 */ 00034 struct Gap { 00035 /** 00036 * Initialisation of the instance. An implementation can use this function 00037 * to initialise the subsystems needed to realize the operations of this 00038 * interface. 00039 * 00040 * This function has to be called before any other operations. 00041 * 00042 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00043 * appropriate error otherwise. 00044 */ 00045 virtual ble_error_t initialize() = 0; 00046 00047 /** 00048 * Termination of the instance. An implementation can use this function 00049 * to release the subsystems initialised to realise the operations of 00050 * this interface. 00051 * 00052 * After a call to this function, initialise should be called again to 00053 * allow use of the interface. 00054 * 00055 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00056 * appropriate error otherwise. 00057 */ 00058 virtual ble_error_t terminate() = 0; 00059 00060 /** 00061 * Return the public device address. 00062 * 00063 * @note The public device address is usually acquired at initialization and 00064 * stored in the instance. 00065 * 00066 * @return the public device address. 00067 */ 00068 virtual address_t get_device_address() = 0; 00069 00070 /** 00071 * Return the current random address. 00072 * 00073 * @note The random address is usually acquired at initialization and stored 00074 * in the instance. 00075 * 00076 * @return the random device address. 00077 */ 00078 virtual address_t get_random_address() = 0; 00079 00080 /** 00081 * Set the random address which will used be during scan, connection or 00082 * advertising process if the own address type selected is random. 00083 * 00084 * Changing the address during scan, connection or advertising process is 00085 * forbiden. 00086 * 00087 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.4 LE set random address command. 00088 * 00089 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00090 * appropriate error otherwise. 00091 */ 00092 virtual ble_error_t set_random_address(const address_t& address) = 0; 00093 00094 /** 00095 * Set the advertising parameters which will be used during the advertising 00096 * process. 00097 * 00098 * @param advertising_interval_min: Minimum advertising interval which can 00099 * be used during undirected and low duty cycle directed advertising. This 00100 * parameter shall be less than or equal to advertising_interval_max. This 00101 * parameter shall be in the range [0x20 : 0x4000] where each unit is equal 00102 * to 0.625ms. 00103 * This parameter is not used by directed high duty cycle advertising. 00104 * 00105 * @param advertising_interval_max: Maximum advertising interval which can 00106 * be used during undirected and low duty cycle directed advertising. This 00107 * parameter shall be more than or equal to advertising_interval_min. This 00108 * parameter shall be in the range [0x20 : 0x4000] where each unit is equal 00109 * to 0.625ms. 00110 * This parameter is not used by directed high duty cycle advertising. 00111 * 00112 * @param advertising_type Packet type that is used during the 00113 * advertising process. Direct advertising require valid peer addresses 00114 * parameters and ignore the filter policy set. 00115 * If the High duty cycle advertising is used then the advertising parameter 00116 * intervals are ignored. 00117 * 00118 * @param own_address_type Own address type used during advertising. 00119 * If own address type is equal to RESOLVABLE_PRIVATE_ADDRESS_PUBLIC_FALLBACK 00120 * or RESOLVABLE_PRIVATE_ADDRESS_RANDOM_FALLBACK then the peer address 00121 * parameters (type and address) will be used to find the local IRK. 00122 * 00123 * @param peer_address_type Address type of the peer. 00124 * This parameter shall be valid if directed advertising is used ( 00125 * ADV_DIRECT_IND or ADV_DIRECT_IND_LOW_DUTY_CYCLE). This parameter should 00126 * be valid if the own address type is equal to 0x02 or 0x03. 00127 * In other cases, this parameter is ignored. 00128 * 00129 * @param peer_address Public device address, Random device addres, Public 00130 * identity address or Random static identity address of the device targeted 00131 * by the advertising. 00132 * This parameter shall be valid if directed advertising is used ( 00133 * ADV_DIRECT_IND or ADV_DIRECT_IND_LOW_DUTY_CYCLE). This parameter should 00134 * be valid if the own address type is equal to 0x02 or 0x03. 00135 * In other cases, this parameter is ignored. 00136 * 00137 * @param advertising_channel_map Map of the channel used to send 00138 * advertising data. 00139 * 00140 * @param advertising_filter_policy Filter policy applied during the 00141 * advertising process. The subsystem should use the whitelist to apply the 00142 * policy. This parameter is ignored if the advertising type is directed ( 00143 * ADV_DIRECT_IND or ADV_DIRECT_IND_LOW_DUTY_CYCLE). 00144 * 00145 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00146 * appropriate error otherwise. 00147 * 00148 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.5 LE set advertising parameters 00149 * command. 00150 */ 00151 virtual ble_error_t set_advertising_parameters( 00152 uint16_t advertising_interval_min, 00153 uint16_t advertising_interval_max, 00154 advertising_type_t advertising_type, 00155 own_address_type_t own_address_type, 00156 advertising_peer_address_type_t peer_address_type, 00157 const address_t& peer_address, 00158 advertising_channel_map_t advertising_channel_map, 00159 advertising_filter_policy_t advertising_filter_policy 00160 ) = 0; 00161 00162 /** 00163 * Set the data sends in advertising packet. If the advertising is 00164 * currently enabled, the data shall be used when a new advertising packet 00165 * is issued. 00166 * 00167 * @param advertising_data_length Number of significant bytes in the 00168 * advertising data. 00169 * 00170 * @param advertising_data The data sends in advertising packets. Non 00171 * significant bytes shall be equal to 0. 00172 * 00173 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00174 * appropriate error otherwise. 00175 * 00176 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.5 LE set advertising data 00177 * command. 00178 */ 00179 virtual ble_error_t set_advertising_data( 00180 uint8_t advertising_data_length, 00181 const advertising_data_t& advertising_data 00182 ) = 0; 00183 00184 /** 00185 * Set the data sends in scan response packets. If the advertising is 00186 * currently enabled, the data shall be used when a new scan response is 00187 * issued. 00188 * 00189 * @param scan_response_data_length Number of significant bytes in the 00190 * scan response data. 00191 * 00192 * @param scan_response_data The data sends in scan response packets. Non 00193 * significant bytes shall be equal to 0. 00194 * 00195 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00196 * appropriate error otherwise. 00197 * 00198 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.8 LE set scan response data 00199 * command. 00200 */ 00201 virtual ble_error_t set_scan_response_data( 00202 uint8_t scan_response_data_length, 00203 const advertising_data_t& scan_response_data 00204 ) = 0; 00205 00206 /** 00207 * Start or stop advertising. 00208 * 00209 * The process use advertising and scan response data set with 00210 * set_advertising_data and set_scan_response_data while the parameters used 00211 * are the one set by set_advertising_parameters. 00212 * 00213 * The advertising shall continue until: 00214 * - The advertising is manually disabled (advertising_enable(false)). 00215 * - A connection is created. 00216 * - Time out in case of high duty cycle directed advertising. 00217 * 00218 * If the random address has not been set and the advertising parameter 00219 * own_address_type is set to 0x01 then the procedure shall fail. 00220 * 00221 * If the random address has not been set and the advertising parameter 00222 * own_address_type is set to RESOLVABLE_PRIVATE_ADDRESS_RANDOM_FALLBACK and 00223 * the peer address is not in the resolving list then the procedure shall 00224 * fail. 00225 * 00226 * @param enable If true start the advertising process, if the process was 00227 * already runing and own_address_type is equal to 0x02 or 0x03, the 00228 * subsystem can change the random address. 00229 * If false and the advertising is running then the process should be 00230 * stoped. 00231 * 00232 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00233 * appropriate error otherwise. 00234 * 00235 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.9 LE set advertising enable 00236 * command. 00237 * 00238 * @note If the advertising type is ADV_DIRECT_IND and the connection is not 00239 * created during the time allowed to the procedure then a 00240 * ConnectionComplete event shall be emmited with its error code set to 00241 * ADVERTISING_TIMEOUT. 00242 * 00243 * @note Successfull connection shall emit a ConnectionComplete event. It 00244 * also means advertising is disabled. 00245 */ 00246 virtual ble_error_t advertising_enable(bool enable) = 0; 00247 00248 /** 00249 * Set the parameter of the scan process. 00250 * 00251 * This command shall not be effective when the scanning process is running. 00252 * 00253 * @param active_scanning If true the subsystem does active scanning and 00254 * the bluetooth subsystem shall send scanning PDUs. It shall also listen 00255 * to scan responses. If false no scanning PDUs are sent during the scan 00256 * process. 00257 * 00258 * @param scan_interval The time interval between two subsequent LE scans in 00259 * unit of 0.625ms. This parameter shall be in the range [0x0004 : 0x4000]. 00260 * 00261 * @param scan_window Duration of the LE scan. It shall be less than or 00262 * equal to scan_interval value. This parameter shall be in the range 00263 * [0x0004 : 0x4000] and is in unit of 0.625ms. 00264 * 00265 * @param own_address_type Own address type used in scan request packets. 00266 * 00267 * @param filter_policy Filter policy applied when scanning. 00268 * 00269 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00270 * appropriate error otherwise. 00271 * 00272 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.10 LE set scan parameters 00273 * command. 00274 */ 00275 virtual ble_error_t set_scan_parameters( 00276 bool active_scanning, 00277 uint16_t scan_interval, 00278 uint16_t scan_window, 00279 own_address_type_t own_address_type, 00280 scanning_filter_policy_t filter_policy 00281 ) = 0; 00282 00283 /** 00284 * Start/stop scanning process. 00285 * 00286 * Parameters of the scanning process shall be set before the scan launch 00287 * by using the function set_scan_parameters. 00288 * 00289 * @parameter enable Start the scanning process if true and stop it if 00290 * false. If the scan process is already started, enabling it again will 00291 * only update the duplicate filtering; based on the new parameter. 00292 * 00293 * @parameter filter_duplicates Enable duplicate filtering if true, 00294 * otherwise disable it. 00295 * 00296 * @important advertising data or scan response data is not considered 00297 * significant when determining duplicate advertising reports. 00298 * 00299 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00300 * appropriate error otherwise. 00301 * 00302 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.11 LE set scan enable command. 00303 */ 00304 virtual ble_error_t scan_enable( 00305 bool enable, 00306 bool filter_duplicates 00307 ) = 0; 00308 00309 /** 00310 * Create a new le connection to a connectable advertiser. 00311 * 00312 * @param scan_interval Hint to the le subsystem indicating how 00313 * frequently it should scan for the peer address. It represent the time 00314 * interval between two subsequent scan for the peer. It shall be in the 00315 * range [0x0004 : 0x4000] and the time is in unit of 0.625ms. 00316 * 00317 * @param scan_window Hint to the le subsystem indicating for how long it 00318 * should scan during a scan interval. The value shall be smaller or equal 00319 * to scan_interval. If it is equal to scan_interval then scanning should 00320 * run continuously. It shall be in the range [0x0004 : 0x4000] and the time 00321 * is in unit of 0.625ms. 00322 * 00323 * @param initiator_policy Used to determine if the whitelist is used to 00324 * determine the address to connect to. If the whitelist is not used, the 00325 * connection shall be made against an advertiser matching the peer_address 00326 * and the peer_address_type parameters. Otherwise those parameters are 00327 * ignored. 00328 * 00329 * @param peer_address_type Type of address used by the advertiser. Not used 00330 * if initiator_policy use the whitelist. 00331 * 00332 * @param Address used by the advertiser in its advertising packets. Not 00333 * used if initiator_policy use the whitelist. 00334 * 00335 * @param own_address_type Type of address used in the connection request 00336 * packet. 00337 * 00338 * @param connection_interval_min Minimum interval between two connection 00339 * events allowed for the connection. It shall be less than or equal to 00340 * connection_interval_max. This value shall be in range [0x0006 : 0x0C80] 00341 * and is in unit of 1.25ms. 00342 * 00343 * @param connection_interval_max Maximum interval between two connection 00344 * events allowed for the connection. It shall be greater than or equal to 00345 * connection_interval_min. This value shall be in range [0x0006 : 0x0C80] 00346 * and is in unit of 1.25ms. 00347 * 00348 * @param connection_latency Number of connection events the slave can drop 00349 * if it has nothing to communicate to the master. This value shall be in 00350 * the range [0x0000 : 0x01F3]. 00351 * 00352 * @param supervision_timeout Link supervision timeout for the connection. 00353 * It shall be larger than: 00354 * (1 + connection_latency) * connection_interval_max * 2 00355 * Note: connection_interval_max is in ms in this formulae. 00356 * Everytime the master or the slave receive a valid packet from the peer, 00357 * the supervision timer is reset. If the supervision timer reaches 00358 * supervision_timeout then the connection is considered lost and a 00359 * disconnect event shall be emmited. 00360 * This value shall be in the range [0x000A : 0x0C80] and is in unit of 10 00361 * ms. 00362 * 00363 * @param minimum_connection_event_length Informative parameter of the 00364 * minimum length a connection event. It shall be less than or equal to 00365 * maximum_connection_event_length. It shall be in the range 00366 * [0x0000 : 0xFFFF]. It should also be less than the expected connection 00367 * interval. The unit is 0.625ms. 00368 * 00369 * @param maximum_connection_event_length Informative parameter of the 00370 * maximum length a connection event. It shall be more than or equal to 00371 * minimum_connection_event_length. It shall be in the range 00372 * [0x0000 : 0xFFFF]. It should also be less than the expected connection 00373 * interval. The unit is 0.625ms. 00374 * 00375 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00376 * appropriate error otherwise. 00377 * 00378 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.12 LE create connection command. 00379 */ 00380 virtual ble_error_t create_connection( 00381 uint16_t scan_interval, 00382 uint16_t scan_window, 00383 initiator_policy_t initiator_policy, 00384 connection_peer_address_type_t peer_address_type, 00385 const address_t& peer_address, 00386 own_address_type_t own_address_type, 00387 uint16_t connection_interval_min, 00388 uint16_t connection_interval_max, 00389 uint16_t connection_latency, 00390 uint16_t supervision_timeout, 00391 uint16_t minimum_connection_event_length, 00392 uint16_t maximum_connection_event_length 00393 ) = 0; 00394 00395 /** 00396 * Cancel the ongoing connection creation process. 00397 * 00398 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00399 * appropriate error otherwise. 00400 * 00401 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.13 LE create connection cancel 00402 * command. 00403 */ 00404 virtual ble_error_t cancel_connection_creation() = 0; 00405 00406 /** 00407 * Return the number of total whitelist entries that can be stored in the 00408 * le subsystem. 00409 * 00410 * @note The value returned can change over time. 00411 * 00412 * @return The number of entries that can be stored in the LE subsystem. It 00413 * range from 0x01 to 0xFF. 00414 * 00415 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.14 LE read white list size 00416 * command. 00417 */ 00418 virtual uint8_t read_white_list_capacity() = 0; 00419 00420 /** 00421 * Clear the whitelist stored in the LE subsystem. 00422 * 00423 * @important This command shall not be issued if the whitelist is being 00424 * used by the advertising, scanning or connection creation procedure. 00425 * 00426 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00427 * appropriate error otherwise. 00428 * 00429 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.15 LE clear white list command. 00430 */ 00431 virtual ble_error_t clear_whitelist() = 0; 00432 00433 /** 00434 * Add a device to the LE subsystem Whitelist. 00435 * 00436 * @param address_type address_type Type of the address to add in the 00437 * whitelist. 00438 * 00439 * @param address Address of the device. 00440 * 00441 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00442 * appropriate error otherwise. 00443 * 00444 * @important This command shall not be issued if the whitelist is being 00445 * used by the advertising, scanning or connection creation procedure. 00446 * 00447 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.16 LE add device to white list 00448 * command. 00449 */ 00450 virtual ble_error_t add_device_to_whitelist( 00451 whitelist_address_type_t address_type, 00452 address_t address 00453 ) = 0; 00454 00455 /** 00456 * Remove a device from the LE subsystem Whitelist. 00457 * 00458 * @param address_type address_type Type of the address of the device to 00459 * remove from the whitelist. 00460 * 00461 * @param address Address of the device to remove from the whitelist 00462 * 00463 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00464 * appropriate error otherwise. 00465 * 00466 * @important This command shall not be issued if the whitelist is being 00467 * used by the advertising, scanning or connection creation procedure. 00468 * 00469 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.17 LE remove device from white 00470 * list command. 00471 */ 00472 virtual ble_error_t remove_device_from_whitelist( 00473 whitelist_address_type_t address_type, 00474 address_t address 00475 ) = 0; 00476 00477 /** 00478 * Start a connection update procedure. 00479 * 00480 * This procedure change the parameter used for a connection it can be 00481 * master or slave initiated. 00482 * 00483 * The peer will received a connection parameters request and will either 00484 * accept or reject the new parameters for the connection. 00485 * 00486 * Once the peer response has been received, the procedure ends and a 00487 * Connection update complete event is emmited. 00488 * 00489 * @param connection Handle of the connection. 00490 * 00491 * @param connection_interval_min Minimum interval between two connection 00492 * events allowed for the connection. It shall be less than or equal to 00493 * connection_interval_max. This value shall be in range [0x0006 : 0x0C80] 00494 * and is in unit of 1.25ms. 00495 * 00496 * @param connection_interval_max Maximum interval between two connection 00497 * events allowed for the connection. It shall be greater than or equal to 00498 * connection_interval_min. This value shall be in range [0x0006 : 0x0C80] 00499 * and is in unit of 1.25ms. 00500 * 00501 * @param connection_latency Number of connection events the slave can drop 00502 * if it has nothing to communicate to the master. This value shall be in 00503 * the range [0x0000 : 0x01F3]. 00504 * 00505 * @param supervision_timeout Link supervision timeout for the connection. 00506 * It shall be larger than: 00507 * (1 + connection_latency) * connection_interval_max * 2 00508 * Note: connection_interval_max is in ms in this formulae. 00509 * Everytime the master or the slave receive a valid packet from the peer, 00510 * the supervision timer is reset. If the supervision timer reaches 00511 * supervision_timeout then the connection is considered lost and a 00512 * disconnect event shall be emmited. 00513 * This value shall be in the range [0x000A : 0x0C80] and is in unit of 10 00514 * ms. 00515 * 00516 * @param minimum_connection_event_length Informative parameter of the 00517 * minimum length a connection event. It shall be less than or equal to 00518 * maximum_connection_event_length. It shall be in the range 00519 * [0x0000 : 0xFFFF]. It should also be less than the expected connection 00520 * interval. The unit is 0.625ms. 00521 * 00522 * @param maximum_connection_event_length Informative parameter of the 00523 * maximum length a connection event. It shall be more than or equal to 00524 * minimum_connection_event_length. It shall be in the range 00525 * [0x0000 : 0xFFFF]. It should also be less than the expected connection 00526 * interval. The unit is 0.625ms. 00527 * 00528 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00529 * appropriate error otherwise. 00530 * 00531 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.18 LE Connection update command. 00532 * 00533 */ 00534 virtual ble_error_t connection_parameters_update( 00535 connection_handle_t connection, 00536 uint16_t connection_interval_min, 00537 uint16_t connection_interval_max, 00538 uint16_t connection_latency, 00539 uint16_t supervision_timeout, 00540 uint16_t minimum_connection_event_length, 00541 uint16_t maximum_connection_event_length 00542 ) = 0; 00543 00544 /** 00545 * Accept connection parameter request. 00546 * 00547 * This command sends a positive response to a connection parameter request 00548 * from a peer. 00549 * 00550 * @param connection Handle of the connection. 00551 * 00552 * @param connection_interval_min Minimum interval between two connection 00553 * events allowed for the connection. It shall be less than or equal to 00554 * connection_interval_max. This value shall be in range [0x0006 : 0x0C80] 00555 * and is in unit of 1.25ms. 00556 * 00557 * @param connection_interval_max Maximum interval between two connection 00558 * events allowed for the connection. It shall be greater than or equal to 00559 * connection_interval_min. This value shall be in range [0x0006 : 0x0C80] 00560 * and is in unit of 1.25ms. 00561 * 00562 * @param connection_latency Number of connection events the slave can drop 00563 * if it has nothing to communicate to the master. This value shall be in 00564 * the range [0x0000 : 0x01F3]. 00565 * 00566 * @param supervision_timeout Link supervision timeout for the connection. 00567 * It shall be larger than: 00568 * (1 + connection_latency) * connection_interval_max * 2 00569 * Note: connection_interval_max is in ms in this formulae. 00570 * Everytime the master or the slave receive a valid packet from the peer, 00571 * the supervision timer is reset. If the supervision timer reaches 00572 * supervision_timeout then the connection is considered lost and a 00573 * disconnect event shall be emmited. 00574 * This value shall be in the range [0x000A : 0x0C80] and is in unit of 10 00575 * ms. 00576 * 00577 * @param minimum_connection_event_length Informative parameter of the 00578 * minimum length a connection event. It shall be less than or equal to 00579 * maximum_connection_event_length. It shall be in the range 00580 * [0x0000 : 0xFFFF]. It should also be less than the expected connection 00581 * interval. The unit is 0.625ms. 00582 * 00583 * @param maximum_connection_event_length Informative parameter of the 00584 * maximum length a connection event. It shall be more than or equal to 00585 * minimum_connection_event_length. It shall be in the range 00586 * [0x0000 : 0xFFFF]. It should also be less than the expected connection 00587 * interval. The unit is 0.625ms. 00588 * 00589 * @note Usually parameters of this function match the connection parameters 00590 * received in the connection parameter request event. 00591 * 00592 * @important: Once the new connection parameters are in used a Connection 00593 * Update Complete event shall be emmited. 00594 * 00595 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00596 * appropriate error otherwise. 00597 * 00598 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.31 LE remote connection parameter 00599 * request reply command. 00600 */ 00601 virtual ble_error_t accept_connection_parameter_request( 00602 connection_handle_t connection_handle, 00603 uint16_t interval_min, 00604 uint16_t interval_max, 00605 uint16_t latency, 00606 uint16_t supervision_timeout, 00607 uint16_t minimum_connection_event_length, 00608 uint16_t maximum_connection_event_length 00609 ) = 0; 00610 00611 /** 00612 * Reject a connection parameter update request. 00613 * 00614 * @param connection_handle handle to the peer which has issued the 00615 * connection parameter request. 00616 * 00617 * @param rejection_reason Indicate to the peer why the proposed connection 00618 * parameters were rejected. 00619 * 00620 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00621 * appropriate error otherwise. 00622 * 00623 * @note: See Bluetooth 5 Vol 2 PartE: 7.8.32 LE Remote Connection Parameter 00624 * Request Negative Reply Command. 00625 */ 00626 virtual ble_error_t reject_connection_parameter_request( 00627 connection_handle_t connection_handle, 00628 hci_error_code_t rejection_reason 00629 ) = 0; 00630 00631 /** 00632 * Start a disconnection procedure. 00633 * 00634 * Once the procedure is complete it should emit a disconnection complete 00635 * event. 00636 * 00637 * @param connection Handle of the connection to terminate. 00638 * 00639 * @param disconnection_reason Indicates the reason for ending the 00640 * connection. 00641 * 00642 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00643 * appropriate error otherwise. 00644 * 00645 * @note: See Bluetooth 5 Vol 2 PartE: 7.1.6 disconenct command. 00646 */ 00647 virtual ble_error_t disconnect( 00648 connection_handle_t connection, 00649 disconnection_reason_t disconnection_reason 00650 ) = 0; 00651 00652 /** 00653 * Register a callback which will handle Gap events. 00654 * 00655 * @param cb The callback object which will handle Gap events from the 00656 * LE subsystem. 00657 * It accept a single parameter in input: The event received. 00658 */ 00659 void when_gap_event_received(mbed::Callback<void(const GapEvent&)> cb) 00660 { 00661 _gap_event_cb = cb; 00662 } 00663 00664 protected: 00665 Gap() { } 00666 00667 virtual ~Gap() { } 00668 00669 /** 00670 * Implementation shall call this function whenever the LE subsystem 00671 * generate a Gap event. 00672 * 00673 * @param gap_event The event to emit to higher layer. 00674 */ 00675 void emit_gap_event(const GapEvent& gap_event) 00676 { 00677 if (_gap_event_cb) { 00678 _gap_event_cb(gap_event); 00679 } 00680 } 00681 00682 private: 00683 /** 00684 * Callback called when an event is emitted by the LE subsystem. 00685 */ 00686 mbed::Callback<void(const GapEvent&)> _gap_event_cb; 00687 00688 // Disallow copy construction and copy assignment. 00689 Gap(const Gap&); 00690 Gap& operator=(const Gap&); 00691 }; 00692 00693 } // namespace pal 00694 } // namespace ble 00695 00696 #endif /* BLE_PAL_GAP_H_ */
Generated on Sun Jul 17 2022 08:25:29 by
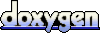