
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
OnboardCellularInterface.h
00001 /* Copyright (c) 2017 ARM Limited 00002 * 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 */ 00015 00016 #ifndef ONBOARD_CELLULAR_INTERFACE_ 00017 #define ONBOARD_CELLULAR_INTERFACE_ 00018 00019 #if MODEM_ON_BOARD && MODEM_ON_BOARD_UART && NSAPI_PPP_AVAILABLE 00020 00021 #include "UARTCellularInterface.h" 00022 00023 /** OnboardCellularInterface class 00024 * 00025 * This interface serves as the controller/driver for an 00026 * onboard modem implementing onboard_modem_api.h. 00027 * 00028 * Depending on the type of on-board modem, OnboardCellularInterface 00029 * could be derived from different implementation classes. 00030 * Portable applications should only rely on it being a CellularBase. 00031 */ 00032 class OnboardCellularInterface : public UARTCellularInterface { 00033 00034 public: 00035 00036 OnboardCellularInterface(bool debug = false); 00037 00038 virtual ~OnboardCellularInterface(); 00039 00040 protected: 00041 /** Sets the modem up for powering on 00042 * 00043 * modem_init() is equivalent to plugging in the device, e.g., attaching power and serial port. 00044 * Uses onboard_modem_api.h where the implementation of onboard_modem_api is provided by the target. 00045 */ 00046 virtual void modem_init(); 00047 00048 /** Sets the modem in unplugged state 00049 * 00050 * modem_deinit() will be equivalent to pulling the plug off of the device, i.e., detaching power 00051 * and serial port. This puts the modem in lowest power state. 00052 * Uses onboard_modem_api.h where the implementation of onboard_modem_api is provided by the target. 00053 */ 00054 virtual void modem_deinit(); 00055 00056 /** Powers up the modem 00057 * 00058 * modem_power_up() is equivalent to pressing the soft power button. 00059 * The driver may repeat this if the modem is not responsive to AT commands. 00060 * Uses onboard_modem_api.h where the implementation of onboard_modem_api is provided by the target. 00061 */ 00062 virtual void modem_power_up(); 00063 00064 /** Powers down the modem 00065 * 00066 * modem_power_down() is equivalent to turning off the modem by button press. 00067 * Uses onboard_modem_api.h where the implementation of onboard_modem_api is provided by the target. 00068 */ 00069 virtual void modem_power_down(); 00070 }; 00071 00072 #endif //MODEM_ON_BOARD && MODEM_ON_BOARD_UART && NSAPI_PPP_AVAILABLE 00073 #endif //ONBOARD_CELLULAR_INTERFACE_
Generated on Sun Jul 17 2022 08:25:29 by
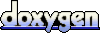