
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
NetworkInterface.h
00001 /* NetworkStack 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef NETWORK_INTERFACE_H 00018 #define NETWORK_INTERFACE_H 00019 00020 #include "netsocket/nsapi_types.h" 00021 #include "netsocket/SocketAddress.h" 00022 00023 // Predeclared class 00024 class NetworkStack; 00025 00026 00027 /** NetworkInterface class 00028 * 00029 * Common interface that is shared between network devices 00030 * @addtogroup netsocket 00031 */ 00032 class NetworkInterface { 00033 public: 00034 virtual ~NetworkInterface() {}; 00035 00036 /** Get the local MAC address 00037 * 00038 * Provided MAC address is intended for info or debug purposes and 00039 * may not be provided if the underlying network interface does not 00040 * provide a MAC address 00041 * 00042 * @return Null-terminated representation of the local MAC address 00043 * or null if no MAC address is available 00044 */ 00045 virtual const char *get_mac_address(); 00046 00047 /** Get the local IP address 00048 * 00049 * @return Null-terminated representation of the local IP address 00050 * or null if no IP address has been recieved 00051 */ 00052 virtual const char *get_ip_address(); 00053 00054 /** Get the local network mask 00055 * 00056 * @return Null-terminated representation of the local network mask 00057 * or null if no network mask has been recieved 00058 */ 00059 virtual const char *get_netmask(); 00060 00061 /** Get the local gateway 00062 * 00063 * @return Null-terminated representation of the local gateway 00064 * or null if no network mask has been recieved 00065 */ 00066 virtual const char *get_gateway(); 00067 00068 /** Set a static IP address 00069 * 00070 * Configures this network interface to use a static IP address. 00071 * Implicitly disables DHCP, which can be enabled in set_dhcp. 00072 * Requires that the network is disconnected. 00073 * 00074 * @param ip_address Null-terminated representation of the local IP address 00075 * @param netmask Null-terminated representation of the local network mask 00076 * @param gateway Null-terminated representation of the local gateway 00077 * @return 0 on success, negative error code on failure 00078 */ 00079 virtual nsapi_error_t set_network( 00080 const char *ip_address, const char *netmask, const char *gateway); 00081 00082 /** Enable or disable DHCP on the network 00083 * 00084 * Enables DHCP on connecting the network. Defaults to enabled unless 00085 * a static IP address has been assigned. Requires that the network is 00086 * disconnected. 00087 * 00088 * @param dhcp True to enable DHCP 00089 * @return 0 on success, negative error code on failure 00090 */ 00091 virtual nsapi_error_t set_dhcp(bool dhcp); 00092 00093 /** Start the interface 00094 * 00095 * @return 0 on success, negative error code on failure 00096 */ 00097 virtual nsapi_error_t connect() = 0; 00098 00099 /** Stop the interface 00100 * 00101 * @return 0 on success, negative error code on failure 00102 */ 00103 virtual nsapi_error_t disconnect() = 0; 00104 00105 /** Translates a hostname to an IP address with specific version 00106 * 00107 * The hostname may be either a domain name or an IP address. If the 00108 * hostname is an IP address, no network transactions will be performed. 00109 * 00110 * If no stack-specific DNS resolution is provided, the hostname 00111 * will be resolve using a UDP socket on the stack. 00112 * 00113 * @param address Destination for the host SocketAddress 00114 * @param host Hostname to resolve 00115 * @param version IP version of address to resolve, NSAPI_UNSPEC indicates 00116 * version is chosen by the stack (defaults to NSAPI_UNSPEC) 00117 * @return 0 on success, negative error code on failure 00118 */ 00119 virtual nsapi_error_t gethostbyname(const char *host, 00120 SocketAddress *address, nsapi_version_t version = NSAPI_UNSPEC ); 00121 00122 /** Add a domain name server to list of servers to query 00123 * 00124 * @param address Destination for the host address 00125 * @return 0 on success, negative error code on failure 00126 */ 00127 virtual nsapi_error_t add_dns_server(const SocketAddress &address); 00128 00129 protected: 00130 friend class Socket; 00131 friend class UDPSocket; 00132 friend class TCPSocket; 00133 friend class TCPServer; 00134 friend class SocketAddress; 00135 template <typename IF> 00136 friend NetworkStack *nsapi_create_stack(IF *iface); 00137 00138 /** Provide access to the NetworkStack object 00139 * 00140 * @return The underlying NetworkStack object 00141 */ 00142 virtual NetworkStack *get_stack() = 0; 00143 }; 00144 00145 00146 #endif
Generated on Sun Jul 17 2022 08:25:28 by
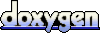