
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
Mutex.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2012 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef MUTEX_H 00023 #define MUTEX_H 00024 00025 #include <stdint.h> 00026 #include "cmsis_os2.h" 00027 #include "mbed_rtos1_types.h" 00028 #include "mbed_rtos_storage.h" 00029 00030 #include "platform/NonCopyable.h" 00031 00032 namespace rtos { 00033 /** \addtogroup rtos */ 00034 /** @{*/ 00035 /** 00036 * \defgroup rtos_Mutex Mutex class 00037 * @{ 00038 */ 00039 00040 /** The Mutex class is used to synchronize the execution of threads. 00041 This is for example used to protect access to a shared resource. 00042 00043 @note 00044 Memory considerations: The mutex control structures will be created on current thread's stack, both for the mbed OS 00045 and underlying RTOS objects (static or dynamic RTOS memory pools are not being used). 00046 */ 00047 class Mutex : private mbed::NonCopyable<Mutex> { 00048 public: 00049 /** Create and Initialize a Mutex object */ 00050 Mutex(); 00051 00052 /** Create and Initialize a Mutex object 00053 00054 @param name name to be used for this mutex. It has to stay allocated for the lifetime of the thread. 00055 */ 00056 Mutex(const char *name); 00057 00058 /** Wait until a Mutex becomes available. 00059 @param millisec timeout value or 0 in case of no time-out. (default: osWaitForever) 00060 @return status code that indicates the execution status of the function: 00061 @a osOK the mutex has been obtained. 00062 @a osErrorTimeout the mutex could not be obtained in the given time. 00063 @a osErrorParameter internal error. 00064 @a osErrorResource the mutex could not be obtained when no timeout was specified. 00065 @a osErrorISR this function cannot be called from the interrupt service routine. 00066 */ 00067 osStatus lock(uint32_t millisec=osWaitForever); 00068 00069 /** Try to lock the mutex, and return immediately 00070 @return true if the mutex was acquired, false otherwise. 00071 */ 00072 bool trylock(); 00073 00074 /** Unlock the mutex that has previously been locked by the same thread 00075 @return status code that indicates the execution status of the function: 00076 @a osOK the mutex has been released. 00077 @a osErrorParameter internal error. 00078 @a osErrorResource the mutex was not locked or the current thread wasn't the owner. 00079 @a osErrorISR this function cannot be called from the interrupt service routine. 00080 */ 00081 osStatus unlock(); 00082 00083 /** Get the owner the this mutex 00084 @return the current owner of this mutex. 00085 */ 00086 osThreadId get_owner(); 00087 00088 ~Mutex(); 00089 00090 private: 00091 void constructor(const char *name = NULL); 00092 friend class ConditionVariable; 00093 00094 osMutexId_t _id; 00095 mbed_rtos_storage_mutex_t _obj_mem; 00096 uint32_t _count; 00097 }; 00098 /** @}*/ 00099 /** @}*/ 00100 } 00101 #endif 00102 00103
Generated on Sun Jul 17 2022 08:25:28 by
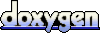