
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
FileSystem.h
00001 00002 /* mbed Microcontroller Library 00003 * Copyright (c) 2006-2013 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef MBED_FILESYSTEM_H 00018 #define MBED_FILESYSTEM_H 00019 00020 #include "platform/platform.h" 00021 00022 #include "platform/FileBase.h" 00023 #include "platform/FileHandle.h" 00024 #include "platform/DirHandle.h" 00025 #include "platform/FileSystemLike.h" 00026 #include "BlockDevice.h" 00027 00028 namespace mbed { 00029 /** \addtogroup filesystem */ 00030 /** @{*/ 00031 00032 00033 // Opaque pointer representing files and directories 00034 typedef void *fs_file_t; 00035 typedef void *fs_dir_t; 00036 00037 // Predeclared classes 00038 class Dir; 00039 class File; 00040 00041 /** A filesystem object provides filesystem operations and file operations 00042 * for the File and Dir classes on a block device. 00043 * 00044 * Implementations must provide at minimum file operations and mount 00045 * operations for block devices. 00046 * 00047 * @note Synchronization level: Set by subclass 00048 */ 00049 class FileSystem : public FileSystemLike { 00050 public: 00051 /** FileSystem lifetime 00052 * 00053 * @param name Name to add filesystem to tree as 00054 */ 00055 FileSystem(const char *name = NULL); 00056 virtual ~FileSystem() {} 00057 00058 /** Mounts a filesystem to a block device 00059 * 00060 * @param bd BlockDevice to mount to 00061 * @return 0 on success, negative error code on failure 00062 */ 00063 virtual int mount(BlockDevice *bd) = 0; 00064 00065 /** Unmounts a filesystem from the underlying block device 00066 * 00067 * @return 0 on success, negative error code on failure 00068 */ 00069 virtual int unmount() = 0; 00070 00071 /** Reformats a filesystem, results in an empty and mounted filesystem 00072 * 00073 * @param bd BlockDevice to reformat and mount. If NULL, the mounted 00074 * block device will be used. 00075 * Note: if mount fails, bd must be provided. 00076 * Default: NULL 00077 * @return 0 on success, negative error code on failure 00078 */ 00079 virtual int reformat(BlockDevice *bd = NULL); 00080 00081 /** Remove a file from the filesystem. 00082 * 00083 * @param path The name of the file to remove. 00084 * @return 0 on success, negative error code on failure 00085 */ 00086 virtual int remove(const char *path); 00087 00088 /** Rename a file in the filesystem. 00089 * 00090 * @param path The name of the file to rename. 00091 * @param newpath The name to rename it to 00092 * @return 0 on success, negative error code on failure 00093 */ 00094 virtual int rename(const char *path, const char *newpath); 00095 00096 /** Store information about the file in a stat structure 00097 * 00098 * @param path The name of the file to find information about 00099 * @param st The stat buffer to write to 00100 * @return 0 on success, negative error code on failure 00101 */ 00102 virtual int stat(const char *path, struct stat *st); 00103 00104 /** Create a directory in the filesystem. 00105 * 00106 * @param path The name of the directory to create. 00107 * @param mode The permissions with which to create the directory 00108 * @return 0 on success, negative error code on failure 00109 */ 00110 virtual int mkdir(const char *path, mode_t mode); 00111 00112 protected: 00113 friend class File; 00114 friend class Dir; 00115 00116 /** Open a file on the filesystem 00117 * 00118 * @param file Destination for the handle to a newly created file 00119 * @param path The name of the file to open 00120 * @param flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, 00121 * bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND 00122 * @return 0 on success, negative error code on failure 00123 */ 00124 virtual int file_open(fs_file_t *file, const char *path, int flags) = 0; 00125 00126 /** Close a file 00127 * 00128 * @param file File handle 00129 * @return 0 on success, negative error code on failure 00130 */ 00131 virtual int file_close(fs_file_t file) = 0; 00132 00133 /** Read the contents of a file into a buffer 00134 * 00135 * @param file File handle 00136 * @param buffer The buffer to read in to 00137 * @param size The number of bytes to read 00138 * @return The number of bytes read, 0 at end of file, negative error on failure 00139 */ 00140 virtual ssize_t file_read(fs_file_t file, void *buffer, size_t size) = 0; 00141 00142 /** Write the contents of a buffer to a file 00143 * 00144 * @param file File handle 00145 * @param buffer The buffer to write from 00146 * @param size The number of bytes to write 00147 * @return The number of bytes written, negative error on failure 00148 */ 00149 virtual ssize_t file_write(fs_file_t file, const void *buffer, size_t size) = 0; 00150 00151 /** Flush any buffers associated with the file 00152 * 00153 * @param file File handle 00154 * @return 0 on success, negative error code on failure 00155 */ 00156 virtual int file_sync(fs_file_t file); 00157 00158 /** Check if the file in an interactive terminal device 00159 * If so, line buffered behaviour is used by default 00160 * 00161 * @param file File handle 00162 * @return True if the file is a terminal 00163 */ 00164 virtual int file_isatty(fs_file_t file); 00165 00166 /** Move the file position to a given offset from from a given location 00167 * 00168 * @param file File handle 00169 * @param offset The offset from whence to move to 00170 * @param whence The start of where to seek 00171 * SEEK_SET to start from beginning of file, 00172 * SEEK_CUR to start from current position in file, 00173 * SEEK_END to start from end of file 00174 * @return The new offset of the file 00175 */ 00176 virtual off_t file_seek(fs_file_t file, off_t offset, int whence) = 0; 00177 00178 /** Get the file position of the file 00179 * 00180 * @param file File handle 00181 * @return The current offset in the file 00182 */ 00183 virtual off_t file_tell(fs_file_t file); 00184 00185 /** Rewind the file position to the beginning of the file 00186 * 00187 * @param file File handle 00188 * @note This is equivalent to file_seek(file, 0, FS_SEEK_SET) 00189 */ 00190 virtual void file_rewind(fs_file_t file); 00191 00192 /** Get the size of the file 00193 * 00194 * @param file File handle 00195 * @return Size of the file in bytes 00196 */ 00197 virtual off_t file_size(fs_file_t file); 00198 00199 /** Open a directory on the filesystem 00200 * 00201 * @param dir Destination for the handle to the directory 00202 * @param path Name of the directory to open 00203 * @return 0 on success, negative error code on failure 00204 */ 00205 virtual int dir_open(fs_dir_t *dir, const char *path); 00206 00207 /** Close a directory 00208 * 00209 * @param dir Dir handle 00210 * @return 0 on success, negative error code on failure 00211 */ 00212 virtual int dir_close(fs_dir_t dir); 00213 00214 /** Read the next directory entry 00215 * 00216 * @param dir Dir handle 00217 * @param ent The directory entry to fill out 00218 * @return 1 on reading a filename, 0 at end of directory, negative error on failure 00219 */ 00220 virtual ssize_t dir_read(fs_dir_t dir, struct dirent *ent); 00221 00222 /** Set the current position of the directory 00223 * 00224 * @param dir Dir handle 00225 * @param offset Offset of the location to seek to, 00226 * must be a value returned from dir_tell 00227 */ 00228 virtual void dir_seek(fs_dir_t dir, off_t offset); 00229 00230 /** Get the current position of the directory 00231 * 00232 * @param dir Dir handle 00233 * @return Position of the directory that can be passed to dir_rewind 00234 */ 00235 virtual off_t dir_tell(fs_dir_t dir); 00236 00237 /** Rewind the current position to the beginning of the directory 00238 * 00239 * @param dir Dir handle 00240 */ 00241 virtual void dir_rewind(fs_dir_t dir); 00242 00243 /** Get the sizeof the directory 00244 * 00245 * @param dir Dir handle 00246 * @return Number of files in the directory 00247 */ 00248 virtual size_t dir_size(fs_dir_t dir); 00249 00250 protected: 00251 // Hooks for FileSystemHandle 00252 virtual int open(FileHandle **file, const char *path, int flags); 00253 virtual int open(DirHandle **dir, const char *path); 00254 }; 00255 00256 00257 /** @}*/ 00258 } // namespace mbed 00259 00260 #endif
Generated on Sun Jul 17 2022 08:25:23 by
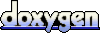