
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
BLE.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_BLE_H__ 00018 #define MBED_BLE_H__ 00019 00020 #include "blecommon.h" 00021 #include "Gap.h" 00022 #include "GattServer.h" 00023 #include "GattClient.h" 00024 #include "SecurityManager.h" 00025 00026 #include "ble/FunctionPointerWithContext.h " 00027 00028 #ifdef YOTTA_CFG_MBED_OS 00029 #include "mbed-drivers/mbed_error.h" 00030 #else 00031 #include "mbed_error.h" 00032 #endif 00033 00034 #include "platform/mbed_toolchain.h" 00035 00036 /* Forward declaration for the implementation class */ 00037 class BLEInstanceBase; 00038 00039 /** 00040 * @addtogroup ble 00041 * @{ 00042 */ 00043 00044 /** 00045 * Abstract away BLE-capable radio transceivers or SOCs. 00046 * 00047 * Instances of this class have three responsibilities: 00048 * - Initialize the inner BLE subsystem. 00049 * - Signal user code that BLE events are available and an API to process them. 00050 * - Manage access to the instances abstracting each BLE layer: 00051 * + GAP: Handle advertising and scan, as well as connection and 00052 * disconnection. 00053 * + GATTServer: API to construct and manage a GATT server, which connected peers can 00054 * access. 00055 * + GATTClient: API to interact with a peer GATT server. 00056 * + SecurityManager: API to manage security. 00057 * 00058 * The user should not create BLE instances directly but rather access to the 00059 * singleton(s) holding the BLE interfaces present in the system by using the 00060 * static function Instance(). 00061 * 00062 * @code 00063 * #include "ble/BLE.h" 00064 * 00065 * BLE& ble_interface = BLE::Instance(); 00066 * @endcode 00067 * 00068 * Next, the signal handling/process mechanism should be set up. By design, 00069 * Mbed BLE does not impose to the user an event handling/processing mechanism; 00070 * however, it exposes APIs, which allows an application to compose its own: 00071 * - onEventsToProcess(), whichs register a callback that 00072 * the BLE subsystem will call when there is an event ready to be processed. 00073 * - processEvents(), which processes all the events present in the BLE subsystem. 00074 * 00075 * It is common to bind BLE event mechanism with Mbed EventQueue: 00076 * 00077 * @code 00078 * #include <events/mbed_events.h> 00079 * #include "ble/BLE.h" 00080 * 00081 * // declare the event queue, which the whole application will share. 00082 * static EventQueue event_queue( 4 * EVENTS_EVENT_SIZE); 00083 * 00084 * // Function invoked when there is a BLE event available. 00085 * // It put into the event queue the processing of the event(s) 00086 * void schedule_ble_processing(BLE::OnEventsToProcessCallbackContext* context) { 00087 * event_queue.call(callback(&(context->ble), &BLE::processEvents)); 00088 * } 00089 * 00090 * int main() 00091 * { 00092 * BLE &ble_interface = BLE::Instance(); 00093 * 00094 * // Bind event signaling to schedule_ble_processing 00095 * ble_interface.onEventsToProcess(schedule_ble_processing); 00096 * 00097 * // Launch BLE initialisation 00098 * 00099 * // Dispatch events in the event queue 00100 * event_queue.dispatch_forever(); 00101 * return 0; 00102 * } 00103 * @endcode 00104 * 00105 * Once the event processing mechanism is in place, the Bluetooth subsystem can 00106 * be initialized with the init() function. That function accepts in input a 00107 * callback, which will be invoked once the initialization process has finished. 00108 * 00109 * @code 00110 * void on_ble_init_complete(BLE::InitializationCompleteCallbackContext *context) 00111 * { 00112 * BLE& ble_interface = context->ble; 00113 * ble_error_t initialization_error = context->error; 00114 * 00115 * if (initialization_error) { 00116 * // handle error 00117 * return; 00118 * } 00119 * 00120 * // The BLE interface can be accessed now. 00121 * } 00122 * 00123 * int main() { 00124 * BLE &ble_interface = BLE::Instance(); 00125 * ble_interface.onEventsToProcess(schedule_ble_processing); 00126 * 00127 * // Initialize the BLE interface 00128 * ble_interface.init(on_ble_init_complete); 00129 * 00130 * event_queue.dispatch_forever(); 00131 * return 0; 00132 * } 00133 * @endcode 00134 */ 00135 class BLE 00136 { 00137 public: 00138 /** 00139 * Opaque type used to store the ID of a BLE instance. 00140 */ 00141 typedef unsigned InstanceID_t; 00142 00143 /** 00144 * The value of the BLE::InstanceID_t for the default BLE instance. 00145 */ 00146 static const InstanceID_t DEFAULT_INSTANCE = 0; 00147 00148 #ifndef YOTTA_CFG_BLE_INSTANCES_COUNT 00149 /** 00150 * The number of permitted BLE instances for the application. 00151 */ 00152 static const InstanceID_t NUM_INSTANCES = 1; 00153 #else 00154 /** 00155 * The number of permitted BLE instances for the application. 00156 */ 00157 static const InstanceID_t NUM_INSTANCES = YOTTA_CFG_BLE_INSTANCES_COUNT; 00158 #endif 00159 00160 /** 00161 * Get a reference to the BLE singleton corresponding to a given interface. 00162 * 00163 * There is a static array of BLE singletons. 00164 * 00165 * @note Calling Instance() is preferred over constructing a BLE object 00166 * directly because it returns references to singletons. 00167 * 00168 * @param[in] id BLE Instance ID to get. 00169 * 00170 * @return A reference to a single object. 00171 * 00172 * @pre id shall be less than NUM_INSTANCES. 00173 */ 00174 static BLE &Instance(InstanceID_t id = DEFAULT_INSTANCE); 00175 00176 /** 00177 * Fetch the ID of a BLE instance. 00178 * 00179 * @return Instance id of this BLE instance. 00180 */ 00181 InstanceID_t getInstanceID(void) const { 00182 return instanceID; 00183 } 00184 00185 /** 00186 * Events to process event. 00187 * 00188 * Instances of OnEventsToProcessCallbackContext are passed to the event 00189 * handler registered with onEventsToProcess(). 00190 */ 00191 struct OnEventsToProcessCallbackContext { 00192 /** 00193 * The ble instance which have events to process. 00194 */ 00195 BLE& ble; 00196 }; 00197 00198 /** 00199 * Events to process event handler 00200 */ 00201 typedef FunctionPointerWithContext<OnEventsToProcessCallbackContext*> 00202 OnEventsToProcessCallback_t; 00203 00204 /** 00205 * Register a callback called when the BLE stack has pending work. 00206 * 00207 * By registering a callback, application code can know when event processing 00208 * has to be scheduled. 00209 * 00210 * @param on_event_cb Callback invoked when there are new events to process. 00211 */ 00212 void onEventsToProcess(const OnEventsToProcessCallback_t & on_event_cb); 00213 00214 /** 00215 * Process ALL pending events living in the BLE stack and return once all 00216 * events have been consumed. 00217 * 00218 * @see onEventsToProcess() 00219 */ 00220 void processEvents(); 00221 00222 /** 00223 * Initialization complete event. 00224 * 00225 * This event is generated at the end of the init() procedure and is passed 00226 * to the completion callback passed to init(). 00227 */ 00228 struct InitializationCompleteCallbackContext { 00229 /** 00230 * Reference to the BLE object that has been initialized 00231 */ 00232 BLE& ble; 00233 00234 /** 00235 * Error status of the initialization. 00236 * 00237 * That value is set to BLE_ERROR_NONE if initialization completed 00238 * successfully or the appropriate error code otherwise. 00239 * */ 00240 ble_error_t error; 00241 }; 00242 00243 /** 00244 * Initialization complete event handler. 00245 * 00246 * @note There are two versions of init(). In addition to the 00247 * function-pointer, init() can also take an <Object, member> tuple as its 00248 * callback target. In case of the latter, the following declaration doesn't 00249 * apply. 00250 */ 00251 typedef void (*InitializationCompleteCallback_t)( 00252 InitializationCompleteCallbackContext *context 00253 ); 00254 00255 /** 00256 * Initialize the BLE controller/stack. 00257 * 00258 * init() hands control to the underlying BLE module to accomplish 00259 * initialization. This initialization may tacitly depend on other hardware 00260 * setup (such as clocks or power-modes) that happens early on during system 00261 * startup. It may not be safe to call init() from a global static context 00262 * where ordering is compiler-specific and can't be guaranteed - it is safe 00263 * to call BLE::init() from within main(). 00264 * 00265 * @param[in] completion_cb A callback for when initialization completes for 00266 * a BLE instance. This is an optional parameter; if no callback is set up, 00267 * the application can still determine the status of initialization using 00268 * BLE::hasInitialized() (see below). 00269 * 00270 * @return BLE_ERROR_NONE if the initialization procedure started 00271 * successfully. 00272 * 00273 * @note If init() returns BLE_ERROR_NONE, the underlying stack must invoke 00274 * the initialization completion callback at some point. 00275 * 00276 * @note Nearly all BLE APIs would return BLE_ERROR_INITIALIZATION_INCOMPLETE 00277 * if used on an instance before the corresponding transport is initialized. 00278 * 00279 * @note There are two versions of init(). In addition to the 00280 * function-pointer, init() can also take an <Object, member> pair as its 00281 * callback target. 00282 * 00283 * @important This should be called before using anything else in the BLE 00284 * API. 00285 */ 00286 ble_error_t init(InitializationCompleteCallback_t completion_cb = NULL) { 00287 FunctionPointerWithContext<InitializationCompleteCallbackContext *> callback(completion_cb); 00288 return initImplementation(callback); 00289 } 00290 00291 /** 00292 * Initialize the BLE controller/stack. 00293 * 00294 * This is an alternate declaration for init(). This one takes an 00295 * <Object, member> pair as its callback target. 00296 * 00297 * @param[in] object Object, which will be used to invoke the completion callback. 00298 * @param[in] completion_cb Member function pointer, which will be invoked when 00299 * initialization is complete. 00300 */ 00301 template<typename T> 00302 ble_error_t init(T *object, void (T::*completion_cb)(InitializationCompleteCallbackContext *context)) { 00303 FunctionPointerWithContext<InitializationCompleteCallbackContext *> callback(object, completion_cb); 00304 return initImplementation(callback); 00305 } 00306 00307 /** 00308 * Indicate if the BLE instance has been initialized. 00309 * 00310 * @return true if initialization has completed for the underlying BLE 00311 * transport. 00312 * 00313 * @note The application should set up a callback to signal completion of 00314 * initialization when using init(). 00315 */ 00316 bool hasInitialized(void) const; 00317 00318 /** 00319 * Shut down the underlying stack, and reset state of this BLE instance. 00320 * 00321 * @return BLE_ERROR_NONE if the instance was shut down without error or the 00322 * appropriate error code. 00323 * 00324 * @important init() must be called afterward to reinstate services and 00325 * GAP state. This API offers a way to repopulate the GATT database with new 00326 * services and characteristics. 00327 */ 00328 ble_error_t shutdown(void); 00329 00330 /** 00331 * This call allows the application to get the BLE stack version information. 00332 * 00333 * @return A pointer to a const string representing the version. 00334 * 00335 * @note The BLE API owns the string returned. 00336 */ 00337 const char *getVersion(void); 00338 00339 /** 00340 * Accessor to Gap. All Gap-related functionality requires going through 00341 * this accessor. 00342 * 00343 * @return A reference to a Gap object associated to this BLE instance. 00344 */ 00345 Gap &gap(); 00346 00347 /** 00348 * A const alternative to gap(). 00349 * 00350 * @return A const reference to a Gap object associated to this BLE instance. 00351 */ 00352 const Gap &gap() const; 00353 00354 /** 00355 * Accessor to GattServer. All GattServer related functionality requires 00356 * going through this accessor. 00357 * 00358 * @return A reference to a GattServer object associated to this BLE instance. 00359 */ 00360 GattServer& gattServer(); 00361 00362 /** 00363 * A const alternative to gattServer(). 00364 * 00365 * @return A const reference to a GattServer object associated to this BLE 00366 * instance. 00367 */ 00368 const GattServer& gattServer() const; 00369 00370 /** 00371 * Accessors to GattClient. All GattClient related functionality requires 00372 * going through this accessor. 00373 * 00374 * @return A reference to a GattClient object associated to this BLE instance. 00375 */ 00376 GattClient& gattClient(); 00377 00378 /** 00379 * A const alternative to gattClient(). 00380 * 00381 * @return A const reference to a GattClient object associated to this BLE 00382 * instance. 00383 */ 00384 const GattClient& gattClient() const; 00385 00386 /** 00387 * Accessors to SecurityManager. All SecurityManager-related functionality 00388 * requires going through this accessor. 00389 * 00390 * @return A reference to a SecurityManager object associated to this BLE 00391 * instance. 00392 */ 00393 SecurityManager& securityManager(); 00394 00395 /** 00396 * A const alternative to securityManager(). 00397 * 00398 * @return A const reference to a SecurityManager object associated to this 00399 * BLE instance. 00400 */ 00401 const SecurityManager& securityManager() const; 00402 00403 /* 00404 * Deprecation alert! 00405 * All of the following are deprecated and may be dropped in a future 00406 * release. Documentation should refer to alternative APIs. 00407 */ 00408 public: 00409 /** 00410 * Constructor for a handle to a BLE instance (the BLE stack). BLE handles 00411 * are thin wrappers around a transport object (that is, ptr. to 00412 * BLEInstanceBase). 00413 * 00414 * It is better to create BLE objects as singletons accessed through the 00415 * Instance() method. If multiple BLE handles are constructed for the same 00416 * interface (using this constructor), they share the same underlying 00417 * transport object. 00418 * 00419 * @deprecated Use the Instance() function instead of the constructor. 00420 */ 00421 MBED_DEPRECATED("Use BLE::Instance() instead of BLE constructor.") 00422 BLE(InstanceID_t instanceID = DEFAULT_INSTANCE); 00423 00424 /** 00425 * Yield control to the BLE stack or to other tasks waiting for events. 00426 * 00427 * This is a sleep function that returns when there is an application-specific 00428 * interrupt. This is not interchangeable with WFE() considering that the 00429 * MCU might wake up several times to service the stack before returning 00430 * control to the caller. 00431 * 00432 * @deprecated This function block the CPU prefer to use the pair 00433 * onEventsToProcess() and processEvents(). 00434 */ 00435 MBED_DEPRECATED("Use BLE::processEvents() and BLE::onEventsToProcess().") 00436 void waitForEvent(void); 00437 00438 /** 00439 * Set the BTLE MAC address and type. 00440 * 00441 * @return BLE_ERROR_NONE on success. 00442 * 00443 * @deprecated You should use the parallel API from Gap directly, refer to 00444 * Gap::setAddress(). A former call to ble.setAddress(...) should be 00445 * replaced with ble.gap().setAddress(...). 00446 */ 00447 MBED_DEPRECATED("Use ble.gap().setAddress(...)") 00448 ble_error_t setAddress( 00449 BLEProtocol::AddressType_t type, 00450 const BLEProtocol::AddressBytes_t address 00451 ) { 00452 return gap().setAddress(type, address); 00453 } 00454 00455 /** 00456 * Fetch the Bluetooth Low Energy MAC address and type. 00457 * 00458 * @return BLE_ERROR_NONE on success. 00459 * 00460 * @deprecated You should use the parallel API from Gap directly and refer to 00461 * Gap::getAddress(). A former call to ble.getAddress(...) should be 00462 * replaced with ble.gap().getAddress(...). 00463 */ 00464 MBED_DEPRECATED("Use ble.gap().getAddress(...)") 00465 ble_error_t getAddress( 00466 BLEProtocol::AddressType_t *typeP, BLEProtocol::AddressBytes_t address 00467 ) { 00468 return gap().getAddress(typeP, address); 00469 } 00470 00471 /** 00472 * Set the GAP advertising mode to use for this device. 00473 * 00474 * @deprecated You should use the parallel API from Gap directly and refer to 00475 * Gap::setAdvertisingType(). A former call to 00476 * ble.setAdvertisingType(...) should be replaced with 00477 * ble.gap().setAdvertisingType(...). 00478 */ 00479 MBED_DEPRECATED("Use ble.gap().setAdvertisingType(...)") 00480 void setAdvertisingType(GapAdvertisingParams::AdvertisingType advType) { 00481 gap().setAdvertisingType(advType); 00482 } 00483 00484 /** 00485 * @param[in] interval 00486 * Advertising interval in units of milliseconds. Advertising 00487 * is disabled if interval is 0. If interval is smaller than 00488 * the minimum supported value, then the minimum supported 00489 * value is used instead. This minimum value can be discovered 00490 * using getMinAdvertisingInterval(). 00491 * 00492 * This field must be set to 0 if connectionMode is equal 00493 * to ADV_CONNECTABLE_DIRECTED. 00494 * 00495 * @note Decreasing this value allows central devices to detect a 00496 * peripheral faster at the expense of more power being used by the radio 00497 * due to the higher data transmit rate. 00498 * 00499 * @deprecated You should use the parallel API from Gap directly and refer to 00500 * Gap::setAdvertisingInterval(). A former call to 00501 * ble.setAdvertisingInterval(...) should be replaced with 00502 * ble.gap().setAdvertisingInterval(...). 00503 * 00504 * @note WARNING: This API previously used 0.625ms as the unit for its 00505 * 'interval' argument. That required an explicit conversion from 00506 * milliseconds using Gap::MSEC_TO_GAP_DURATION_UNITS(). This conversion is 00507 * no longer required as the new units are milliseconds. Any application 00508 * code depending on the old semantics needs to be updated accordingly. 00509 */ 00510 MBED_DEPRECATED("Use ble.gap().setAdvertisingInterval(...)") 00511 void setAdvertisingInterval(uint16_t interval) { 00512 gap().setAdvertisingInterval(interval); 00513 } 00514 00515 /** 00516 * @return Minimum Advertising interval in milliseconds. 00517 * 00518 * @deprecated You should use the parallel API from Gap directly, refer to 00519 * Gap::getMinAdvertisingInterval(). A former call to 00520 * ble.getMinAdvertisingInterval(...) should be replaced with 00521 * ble.gap().getMinAdvertisingInterval(...). 00522 */ 00523 MBED_DEPRECATED("Use ble.gap().getMinAdvertisingInterval(...)") 00524 uint16_t getMinAdvertisingInterval(void) const { 00525 return gap().getMinAdvertisingInterval(); 00526 } 00527 00528 /** 00529 * @return Minimum Advertising interval in milliseconds for nonconnectible mode. 00530 * 00531 * @deprecated You should use the parallel API from Gap directly, refer to 00532 * Gap::MinNonConnectableAdvertisingInterval(). A former call to 00533 * ble.getMinNonConnectableAdvertisingInterval(...) should be replaced with 00534 * ble.gap().getMinNonConnectableAdvertisingInterval(...). 00535 */ 00536 MBED_DEPRECATED("Use ble.gap().getMinNonConnectableAdvertisingInterval(...)") 00537 uint16_t getMinNonConnectableAdvertisingInterval(void) const { 00538 return gap().getMinNonConnectableAdvertisingInterval(); 00539 } 00540 00541 /** 00542 * @return Maximum Advertising interval in milliseconds. 00543 * 00544 * @deprecated You should use the parallel API from Gap directly, refer to 00545 * Gap::getMaxAdvertisingInterval(). A former call to 00546 * ble.getMaxAdvertisingInterval(...) should be replaced with 00547 * ble.gap().getMaxAdvertisingInterval(...). 00548 */ 00549 MBED_DEPRECATED("Use ble.gap().getMaxAdvertisingInterval(...)") 00550 uint16_t getMaxAdvertisingInterval(void) const { 00551 return gap().getMaxAdvertisingInterval(); 00552 } 00553 00554 /** 00555 * @param[in] timeout 00556 * Advertising timeout (in seconds) between 0x1 and 0x3FFF (1 00557 * and 16383). Use 0 to disable the advertising timeout. 00558 * 00559 * @deprecated You should use the parallel API from Gap directly and refer to 00560 * Gap::setAdvertisingTimeout(). A former call to 00561 * ble.setAdvertisingTimeout(...) should be replaced with 00562 * ble.gap().setAdvertisingTimeout(...). 00563 */ 00564 MBED_DEPRECATED("Use ble.gap().setAdvertisingTimeout(...)") 00565 void setAdvertisingTimeout(uint16_t timeout) { 00566 gap().setAdvertisingTimeout(timeout); 00567 } 00568 00569 /** 00570 * Set up a particular, user-constructed set of advertisement parameters for 00571 * the underlying stack. It would be uncommon for this API to be used 00572 * directly; there are other APIs to tweak advertisement parameters 00573 * individually (see above). 00574 * 00575 * @deprecated You should use the parallel API from Gap directly and refer to 00576 * Gap::setAdvertisingParams(). A former call to 00577 * ble.setAdvertisingParams(...) should be replaced with 00578 * ble.gap().setAdvertisingParams(...). 00579 */ 00580 MBED_DEPRECATED("Use ble.gap().setAdvertisingParams(...)") 00581 void setAdvertisingParams(const GapAdvertisingParams &advParams) { 00582 gap().setAdvertisingParams(advParams); 00583 } 00584 00585 /** 00586 * @return Read back advertising parameters. Useful for storing and 00587 * restoring parameters rapidly. 00588 * 00589 * @deprecated You should use the parallel API from Gap directly and refer to 00590 * Gap::getAdvertisingParams(). A former call to 00591 * ble.getAdvertisingParams(...) should be replaced with 00592 * ble.gap().getAdvertisingParams(...). 00593 */ 00594 MBED_DEPRECATED("Use ble.gap().getAdvertisingParams(...)") 00595 const GapAdvertisingParams &getAdvertisingParams(void) const { 00596 return gap().getAdvertisingParams(); 00597 } 00598 00599 /** 00600 * Accumulate an AD structure in the advertising payload. Please note that 00601 * the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used 00602 * as an additional 31 bytes if the advertising payload is too 00603 * small. 00604 * 00605 * @param[in] flags 00606 * The flags to add. Please refer to 00607 * GapAdvertisingData::Flags for valid flags. Multiple 00608 * flags may be specified in combination. 00609 * 00610 * @deprecated You should use the parallel API from Gap directly, refer to 00611 * Gap::accumulateAdvertisingPayload(uint8_t). A former call to 00612 * ble.accumulateAdvertisingPayload(flags) should be replaced with 00613 * ble.gap().accumulateAdvertisingPayload(flags). 00614 */ 00615 MBED_DEPRECATED("Use ble.gap().accumulateAdvertisingPayload(flags)") 00616 ble_error_t accumulateAdvertisingPayload(uint8_t flags) { 00617 return gap().accumulateAdvertisingPayload(flags); 00618 } 00619 00620 /** 00621 * Accumulate an AD structure in the advertising payload. Please note that 00622 * the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used 00623 * as an additional 31 bytes if the advertising payload is too 00624 * small. 00625 * 00626 * @param[in] app 00627 * The appearance of the peripheral. 00628 * 00629 * @deprecated You should use the parallel API from Gap directly and refer to 00630 * Gap::accumulateAdvertisingPayload(GapAdvertisingData::Appearance). 00631 * A former call to ble.accumulateAdvertisingPayload(appearance) 00632 * should be replaced with 00633 * ble.gap().accumulateAdvertisingPayload(appearance). 00634 */ 00635 MBED_DEPRECATED("Use ble.gap().accumulateAdvertisingPayload(appearance)") 00636 ble_error_t accumulateAdvertisingPayload(GapAdvertisingData::Appearance app) { 00637 return gap().accumulateAdvertisingPayload(app); 00638 } 00639 00640 /** 00641 * Accumulate an AD structure in the advertising payload. Please note that 00642 * the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used 00643 * as an additional 31 bytes if the advertising payload is too 00644 * small. 00645 * 00646 * @param[in] power 00647 * The max transmit power to be used by the controller. This 00648 * is only a hint. 00649 * 00650 * @deprecated You should use the parallel API from Gap directly and refer to 00651 * Gap::accumulateAdvertisingPayloadTxPower(). A former call to 00652 * ble.accumulateAdvertisingPayloadTxPower(txPower) should be replaced with 00653 * ble.gap().accumulateAdvertisingPayloadTxPower(txPower). 00654 */ 00655 MBED_DEPRECATED("Use ble.gap().accumulateAdvertisingPayloadTxPower(...)") 00656 ble_error_t accumulateAdvertisingPayloadTxPower(int8_t power) { 00657 return gap().accumulateAdvertisingPayloadTxPower(power); 00658 } 00659 00660 /** 00661 * Accumulate a variable length byte-stream as an AD structure in the 00662 * advertising payload. Please note that the payload is limited to 31 bytes. 00663 * The SCAN_RESPONSE message may be used as an additional 31 bytes if the 00664 * advertising payload is too small. 00665 * 00666 * @param type The type that describes the variable length data. 00667 * @param data Data bytes. 00668 * @param len Data length. 00669 * 00670 * @deprecated You should use the parallel API from Gap directly, refer to 00671 * Gap::accumulateAdvertisingPayload(GapAdvertisingData::DataType, const uint8_t, uint8_t). 00672 * A former call to ble.accumulateAdvertisingPayload(...) should 00673 * be replaced with ble.gap().accumulateAdvertisingPayload(...). 00674 */ 00675 MBED_DEPRECATED("Use ble.gap().accumulateAdvertisingPayload(...)") 00676 ble_error_t accumulateAdvertisingPayload(GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) { 00677 return gap().accumulateAdvertisingPayload(type, data, len); 00678 } 00679 00680 /** 00681 * Set up a particular, user-constructed advertisement payload for the 00682 * underlying stack. It would be uncommon for this API to be used directly; 00683 * there are other APIs to build an advertisement payload (see above). 00684 * 00685 * @deprecated You should use the parallel API from Gap directly, refer to 00686 * Gap::setAdvertisingData(). A former call to 00687 * ble.setAdvertisingData(...) should be replaced with 00688 * ble.gap().setAdvertisingPayload(...). 00689 */ 00690 MBED_DEPRECATED("Use ble.gap().setAdvertisingData(...)") 00691 ble_error_t setAdvertisingData(const GapAdvertisingData &advData) { 00692 return gap().setAdvertisingPayload(advData); 00693 } 00694 00695 /** 00696 * @return Read back advertising data. Useful for storing and 00697 * restoring payload. 00698 * 00699 * @deprecated You should use the parallel API from Gap directly, refer to 00700 * Gap::getAdvertisingData(). A former call to 00701 * ble.getAdvertisingData(...) should be replaced with 00702 * ble.gap().getAdvertisingPayload()(...). 00703 */ 00704 MBED_DEPRECATED("Use ble.gap().getAdvertisingData(...)") 00705 const GapAdvertisingData &getAdvertisingData(void) const { 00706 return gap().getAdvertisingPayload(); 00707 } 00708 00709 /** 00710 * Reset any advertising payload prepared from prior calls to 00711 * accumulateAdvertisingPayload(). This automatically propagates the re- 00712 * initialized advertising payload to the underlying stack. 00713 * 00714 * @deprecated You should use the parallel API from Gap directly and refer to 00715 * Gap::clearAdvertisingPayload(). A former call to 00716 * ble.clearAdvertisingPayload(...) should be replaced with 00717 * ble.gap().clearAdvertisingPayload(...). 00718 */ 00719 MBED_DEPRECATED("Use ble.gap().clearAdvertisingPayload(...)") 00720 void clearAdvertisingPayload(void) { 00721 gap().clearAdvertisingPayload(); 00722 } 00723 00724 /** 00725 * Dynamically reset the accumulated advertising 00726 * payload and scanResponse. The application must clear and re- 00727 * accumulate a new advertising payload (and scanResponse) before using this 00728 * API. 00729 * 00730 * @return BLE_ERROR_NONE when the advertising payload is set successfully. 00731 * 00732 * @deprecated You should use the parallel API from Gap directly, refer to 00733 * Gap::setAdvertisingPayload(). 00734 * 00735 * @note The new APIs in Gap update the underlying advertisement payload 00736 * implicitly. 00737 */ 00738 MBED_DEPRECATED("Use ble.gap().setAdvertisingPayload(...)") 00739 ble_error_t setAdvertisingPayload(void) { 00740 return BLE_ERROR_NONE; 00741 } 00742 00743 /** 00744 * Accumulate a variable length byte-stream as an AD structure in the 00745 * scanResponse payload. 00746 * 00747 * @param[in] type The type that describes the variable length data. 00748 * @param[in] data Data bytes. 00749 * @param[in] len Data length. 00750 * 00751 * @deprecated You should use the parallel API from Gap directly and refer to 00752 * Gap::accumulateScanResponse(). A former call to 00753 * ble.accumulateScanResponse(...) should be replaced with 00754 * ble.gap().accumulateScanResponse(...). 00755 */ 00756 MBED_DEPRECATED("Use ble.gap().accumulateScanResponse(...)") 00757 ble_error_t accumulateScanResponse(GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) { 00758 return gap().accumulateScanResponse(type, data, len); 00759 } 00760 00761 /** 00762 * Reset any scan response prepared from prior calls to 00763 * accumulateScanResponse(). 00764 * 00765 * @deprecated You should use the parallel API from Gap directly and refer to 00766 * Gap::clearScanResponse(). A former call to 00767 * ble.clearScanResponse(...) should be replaced with 00768 * ble.gap().clearScanResponse(...). 00769 */ 00770 MBED_DEPRECATED("Use ble.gap().clearScanResponse(...)") 00771 void clearScanResponse(void) { 00772 gap().clearScanResponse(); 00773 } 00774 00775 /** 00776 * Start advertising. 00777 * 00778 * @deprecated You should use the parallel API from Gap directly and refer to 00779 * Gap::startAdvertising(). A former call to 00780 * ble.startAdvertising(...) should be replaced with 00781 * ble.gap().startAdvertising(...). 00782 */ 00783 MBED_DEPRECATED("Use ble.gap().startAdvertising(...)") 00784 ble_error_t startAdvertising(void) { 00785 return gap().startAdvertising(); 00786 } 00787 00788 /** 00789 * Stop advertising. 00790 * 00791 * @deprecated You should use the parallel API from Gap directly and refer to 00792 * Gap::stopAdvertising(). A former call to 00793 * ble.stopAdvertising(...) should be replaced with 00794 * ble.gap().stopAdvertising(...). 00795 */ 00796 MBED_DEPRECATED("Use ble.gap().stopAdvertising(...)") 00797 ble_error_t stopAdvertising(void) { 00798 return gap().stopAdvertising(); 00799 } 00800 00801 /** 00802 * Set up parameters for GAP scanning (observer mode). 00803 * @param[in] interval 00804 * Scan interval (in milliseconds) [valid values lie between 2.5ms and 10.24s]. 00805 * @param[in] window 00806 * Scan Window (in milliseconds) [valid values lie between 2.5ms and 10.24s]. 00807 * @param[in] timeout 00808 * Scan timeout (in seconds) between 0x0001 and 0xFFFF; 0x0000 disables timeout. 00809 * @param[in] activeScanning 00810 * Set to True if active-scanning is required. This is used to fetch the 00811 * scan response from a peer if possible. 00812 * 00813 * The scanning window divided by the interval determines the duty cycle for 00814 * scanning. For example, if the interval is 100ms and the window is 10ms, 00815 * then the controller will scan for 10 percent of the time. It is possible 00816 * to have the interval and window set to the same value. In this case, 00817 * scanning is continuous, with a change of scanning frequency once every 00818 * interval. 00819 * 00820 * Once the scanning parameters have been configured, scanning can be 00821 * enabled by using startScan(). 00822 * 00823 * @note The scan interval and window are recommendations to the BLE stack. 00824 * 00825 * @deprecated You should use the parallel API from Gap directly and refer to 00826 * Gap::setScanParams(). A former call to 00827 * ble.setScanParams(...) should be replaced with 00828 * ble.gap().setScanParams(...). 00829 */ 00830 MBED_DEPRECATED("Use ble.gap().setScanParams(...)") 00831 ble_error_t setScanParams(uint16_t interval = GapScanningParams::SCAN_INTERVAL_MAX, 00832 uint16_t window = GapScanningParams::SCAN_WINDOW_MAX, 00833 uint16_t timeout = 0, 00834 bool activeScanning = false) { 00835 return gap().setScanParams(interval, window, timeout, activeScanning); 00836 } 00837 00838 /** 00839 * Set up the scanInterval parameter for GAP scanning (observer mode). 00840 * @param[in] interval 00841 * Scan interval (in milliseconds) [valid values lie between 2.5ms and 10.24s]. 00842 * 00843 * The scanning window divided by the interval determines the duty cycle for 00844 * scanning. For example, if the interval is 100ms and the window is 10ms, 00845 * then the controller will scan for 10 percent of the time. It is possible 00846 * to have the interval and window set to the same value. In this case, 00847 * scanning is continuous, with a change of scanning frequency once every 00848 * interval. 00849 * 00850 * Once the scanning parameters have been configured, scanning can be 00851 * enabled by using startScan(). 00852 * 00853 * @deprecated You should use the parallel API from Gap directly and refer to 00854 * Gap::setScanInterval(). A former call to 00855 * ble.setScanInterval(interval) should be replaced with 00856 * ble.gap().setScanInterval(interval). 00857 */ 00858 MBED_DEPRECATED("Use ble.gap().setScanInterval(...)") 00859 ble_error_t setScanInterval(uint16_t interval) { 00860 return gap().setScanInterval(interval); 00861 } 00862 00863 /** 00864 * Set up the scanWindow parameter for GAP scanning (observer mode). 00865 * @param[in] window 00866 * Scan Window (in milliseconds) [valid values lie between 2.5ms and 10.24s]. 00867 * 00868 * The scanning window divided by the interval determines the duty cycle for 00869 * scanning. For example, if the interval is 100ms and the window is 10ms, 00870 * then the controller will scan for 10 percent of the time. It is possible 00871 * to have the interval and window set to the same value. In this case, 00872 * scanning is continuous, with a change of scanning frequency once every 00873 * interval. 00874 * 00875 * Once the scanning parameters have been configured, scanning can be 00876 * enabled by using startScan(). 00877 * 00878 * @deprecated You should use the parallel API from Gap directly and refer to 00879 * Gap::setScanWindow(). A former call to 00880 * ble.setScanWindow(window) should be replaced with 00881 * ble.gap().setScanWindow(window). 00882 */ 00883 MBED_DEPRECATED("Use ble.gap().setScanWindow(...)") 00884 ble_error_t setScanWindow(uint16_t window) { 00885 return gap().setScanWindow(window); 00886 } 00887 00888 /** 00889 * Set up parameters for GAP scanning (observer mode). 00890 * @param[in] timeout 00891 * Scan timeout (in seconds) between 0x0001 and 0xFFFF; 0x0000 disables timeout. 00892 * 00893 * The scanning window divided by the interval determines the duty cycle for 00894 * scanning. For example, if the interval is 100ms and the window is 10ms, 00895 * then the controller will scan for 10 percent of the time. It is possible 00896 * to have the interval and window set to the same value. In this case, 00897 * scanning is continuous, with a change of scanning frequency once every 00898 * interval. 00899 * 00900 * Once the scanning parameters have been configured, scanning can be 00901 * enabled by using startScan(). 00902 * 00903 * @note The scan interval and window are recommendations to the BLE stack. 00904 * 00905 * @deprecated You should use the parallel API from Gap directly and refer to 00906 * Gap::setScanTimeout(). A former call to 00907 * ble.setScanTimeout(...) should be replaced with 00908 * ble.gap().setScanTimeout(...). 00909 */ 00910 MBED_DEPRECATED("Use ble.gap().setScanTimeout(...)") 00911 ble_error_t setScanTimeout(uint16_t timeout) { 00912 return gap().setScanTimeout(timeout); 00913 } 00914 00915 /** 00916 * Set up parameters for GAP scanning (observer mode). 00917 * @param[in] activeScanning 00918 * Set to True if active-scanning is required. This is used to fetch the 00919 * scan response from a peer if possible. 00920 * 00921 * Once the scanning parameters have been configured, scanning can be 00922 * enabled by using startScan(). 00923 * 00924 * @deprecated You should use the parallel API from Gap directly, refer to 00925 * Gap::setActiveScan(). A former call to 00926 * ble.setActiveScan(...) should be replaced with 00927 * ble.gap().setActiveScanning(...). 00928 */ 00929 MBED_DEPRECATED("Use ble.gap().setActiveScan(...)") 00930 void setActiveScan(bool activeScanning) { 00931 gap().setActiveScanning(activeScanning); 00932 } 00933 00934 /** 00935 * Start scanning (Observer Procedure) based on the parameters currently in 00936 * effect. 00937 * 00938 * @param[in] callback 00939 * The application-specific callback to be invoked upon 00940 * receiving every advertisement report. This can be passed in 00941 * as NULL, in which case scanning may not be enabled at all. 00942 * 00943 * @deprecated You should use the parallel API from Gap directly, refer to 00944 * Gap::startScan(). A former call to 00945 * ble.startScan(callback) should be replaced with 00946 * ble.gap().startScan(callback). 00947 */ 00948 MBED_DEPRECATED("Use ble.gap().startScan(callback)") 00949 ble_error_t startScan(void (*callback)(const Gap::AdvertisementCallbackParams_t *params)) { 00950 return gap().startScan(callback); 00951 } 00952 00953 /** 00954 * Same as above, but this takes an (object, method) pair for a callback. 00955 * 00956 * @deprecated You should use the parallel API from Gap directly, refer to 00957 * Gap::startScan(). A former call to 00958 * ble.startScan(callback) should be replaced with 00959 * ble.gap().startScan(object, callback). 00960 */ 00961 template<typename T> 00962 MBED_DEPRECATED("Use ble.gap().startScan(object, callback)") 00963 ble_error_t startScan(T *object, void (T::*memberCallback)(const Gap::AdvertisementCallbackParams_t *params)); 00964 00965 /** 00966 * Stop scanning. The current scanning parameters remain in effect. 00967 * 00968 * @retval BLE_ERROR_NONE if successfully stopped scanning procedure. 00969 * 00970 * @deprecated You should use the parallel API from Gap directly and refer to 00971 * Gap::stopScan(). A former call to 00972 * ble.stopScan() should be replaced with 00973 * ble.gap().stopScan(). 00974 */ 00975 MBED_DEPRECATED("Use ble.gap().stopScan()") 00976 ble_error_t stopScan(void) { 00977 return gap().stopScan(); 00978 } 00979 00980 /** 00981 * Create a connection (GAP Link Establishment). 00982 * @param peerAddr 00983 * 48-bit address, LSB format. 00984 * @param peerAddrType 00985 * Address type of the peer. 00986 * @param connectionParams 00987 * Connection parameters. 00988 * @param scanParams 00989 * Parameters to use while scanning for the peer. 00990 * @return BLE_ERROR_NONE if connection establishment procedure is started 00991 * successfully. The onConnection callback (if set) is invoked upon 00992 * a connection event. 00993 * 00994 * @deprecated You should use the parallel API from Gap directly and refer to 00995 * Gap::connect(). A former call to 00996 * ble.connect(...) should be replaced with 00997 * ble.gap().connect(...). 00998 */ 00999 MBED_DEPRECATED("Use ble.gap().connect(...)") 01000 ble_error_t connect(const BLEProtocol::AddressBytes_t peerAddr, 01001 BLEProtocol::AddressType_t peerAddrType = BLEProtocol::AddressType::RANDOM_STATIC, 01002 const Gap::ConnectionParams_t *connectionParams = NULL, 01003 const GapScanningParams *scanParams = NULL) { 01004 return gap().connect(peerAddr, peerAddrType, connectionParams, scanParams); 01005 } 01006 01007 /** 01008 * This call initiates the disconnection procedure, and its completion is 01009 * communicated to the application with an invocation of the 01010 * onDisconnection callback. 01011 * 01012 * @param[in] connectionHandle 01013 * @param[in] reason 01014 * The reason for disconnection; sent back to the peer. 01015 */ 01016 MBED_DEPRECATED("Use ble.gap().disconnect(...)") 01017 ble_error_t disconnect(Gap::Handle_t connectionHandle, Gap::DisconnectionReason_t reason) { 01018 return gap().disconnect(connectionHandle, reason); 01019 } 01020 01021 /** 01022 * This call initiates the disconnection procedure, and its completion 01023 * is communicated to the application with an invocation of the 01024 * onDisconnection callback. 01025 * 01026 * @param reason 01027 * The reason for disconnection; sent back to the peer. 01028 * 01029 * @deprecated You should use the parallel API from Gap directly and refer to 01030 * Gap::disconnect(). A former call to 01031 * ble.disconnect(reason) should be replaced with 01032 * ble.gap().disconnect(reason). 01033 * 01034 * @note This version of disconnect() doesn't take a connection handle. It 01035 * works reliably only for stacks that are limited to a single 01036 * connection. 01037 */ 01038 MBED_DEPRECATED("Use ble.gap().disconnect(...)") 01039 ble_error_t disconnect(Gap::DisconnectionReason_t reason) { 01040 return gap().disconnect(reason); 01041 } 01042 01043 /** 01044 * Returns the current Gap state of the device using a bitmask that 01045 * describes whether the device is advertising or connected. 01046 * 01047 * @deprecated You should use the parallel API from Gap directly and refer to 01048 * Gap::getState(). A former call to 01049 * ble.getGapState() should be replaced with 01050 * ble.gap().getState(). 01051 */ 01052 MBED_DEPRECATED("Use ble.gap().getGapState(...)") 01053 Gap::GapState_t getGapState(void) const { 01054 return gap().getState(); 01055 } 01056 01057 /** 01058 * Get the GAP peripheral's preferred connection parameters. These are the 01059 * defaults that the peripheral would like to have in a connection. The 01060 * choice of the connection parameters is eventually up to the central. 01061 * 01062 * @param[out] params 01063 * The structure where the parameters will be stored. The caller owns memory 01064 * for this. 01065 * 01066 * @return BLE_ERROR_NONE if the parameters were successfully filled into 01067 * the given structure pointed to by params. 01068 * 01069 * @deprecated You should use the parallel API from Gap directly and refer to 01070 * Gap::getPreferredConnectionParams(). A former call to 01071 * ble.getPreferredConnectionParams() should be replaced with 01072 * ble.gap().getPreferredConnectionParams(). 01073 */ 01074 MBED_DEPRECATED("Use ble.gap().getPreferredConnectionParams(...)") 01075 ble_error_t getPreferredConnectionParams(Gap::ConnectionParams_t *params) { 01076 return gap().getPreferredConnectionParams(params); 01077 } 01078 01079 /** 01080 * Set the GAP peripheral's preferred connection parameters. These are the 01081 * defaults that the peripheral would like to have in a connection. The 01082 * choice of the connection parameters is eventually up to the central. 01083 * 01084 * @param[in] params 01085 * The structure containing the desired parameters. 01086 * 01087 * @deprecated You should use the parallel API from Gap directly and refer to 01088 * Gap::setPreferredConnectionParams(). A former call to 01089 * ble.setPreferredConnectionParams() should be replaced with 01090 * ble.gap().setPreferredConnectionParams(). 01091 */ 01092 MBED_DEPRECATED("Use ble.gap().setPreferredConnectionParams(...)") 01093 ble_error_t setPreferredConnectionParams(const Gap::ConnectionParams_t *params) { 01094 return gap().setPreferredConnectionParams(params); 01095 } 01096 01097 /** 01098 * Update connection parameters while in the peripheral role. 01099 * @details In the peripheral role, this will send the corresponding L2CAP request to the connected peer and wait for 01100 * the central to perform the procedure. 01101 * @param[in] handle 01102 * Connection Handle 01103 * @param[in] params 01104 * Pointer to desired connection parameters. If NULL is provided on a peripheral role, 01105 * the parameters in the PPCP characteristic of the GAP service will be used instead. 01106 * 01107 * @deprecated You should use the parallel API from Gap directly and refer to 01108 * Gap::updateConnectionParams(). A former call to 01109 * ble.updateConnectionParams() should be replaced with 01110 * ble.gap().updateConnectionParams(). 01111 */ 01112 MBED_DEPRECATED("Use ble.gap().updateConnectionParams(...)") 01113 ble_error_t updateConnectionParams(Gap::Handle_t handle, const Gap::ConnectionParams_t *params) { 01114 return gap().updateConnectionParams(handle, params); 01115 } 01116 01117 /** 01118 * Set the device name characteristic in the Gap service. 01119 * @param[in] deviceName 01120 * The new value for the device-name. This is a UTF-8 encoded, <b>NULL-terminated</b> string. 01121 * 01122 * @deprecated You should use the parallel API from Gap directly and refer to 01123 * Gap::setDeviceName(). A former call to 01124 * ble.setDeviceName() should be replaced with 01125 * ble.gap().setDeviceName(). 01126 */ 01127 MBED_DEPRECATED("Use ble.gap().setDeviceName(...)") 01128 ble_error_t setDeviceName(const uint8_t *deviceName) { 01129 return gap().setDeviceName(deviceName); 01130 } 01131 01132 /** 01133 * Get the value of the device name characteristic in the Gap service. 01134 * @param[out] deviceName 01135 * Pointer to an empty buffer where the UTF-8 *non NULL- 01136 * terminated* string will be placed. Set this 01137 * value to NULL to obtain the deviceName-length 01138 * from the 'length' parameter. 01139 * 01140 * @param[in,out] lengthP 01141 * (on input) Length of the buffer pointed to by deviceName; 01142 * (on output) the complete device name length (without the 01143 * null terminator). 01144 * 01145 * @note If the device name is longer than the size of the supplied buffer, 01146 * the length will return the complete device name length and not the 01147 * number of bytes actually returned in deviceName. The application may 01148 * use this information to retry with a suitable buffer size. 01149 * 01150 * @deprecated You should use the parallel API from Gap directly and refer to 01151 * Gap::getDeviceName(). A former call to 01152 * ble.getDeviceName() should be replaced with 01153 * ble.gap().getDeviceName(). 01154 */ 01155 MBED_DEPRECATED("Use ble.gap().getDeviceName(...)") 01156 ble_error_t getDeviceName(uint8_t *deviceName, unsigned *lengthP) { 01157 return gap().getDeviceName(deviceName, lengthP); 01158 } 01159 01160 /** 01161 * Set the appearance characteristic in the Gap service. 01162 * @param[in] appearance 01163 * The new value for the device-appearance. 01164 * 01165 * @deprecated You should use the parallel API from Gap directly and refer to 01166 * Gap::setAppearance(). A former call to 01167 * ble.setAppearance() should be replaced with 01168 * ble.gap().setAppearance(). 01169 */ 01170 MBED_DEPRECATED("Use ble.gap().setAppearance(...)") 01171 ble_error_t setAppearance(GapAdvertisingData::Appearance appearance) { 01172 return gap().setAppearance(appearance); 01173 } 01174 01175 /** 01176 * Get the appearance characteristic in the Gap service. 01177 * @param[out] appearanceP 01178 * The new value for the device-appearance. 01179 * 01180 * @deprecated You should use the parallel API from Gap directly, refer to 01181 * Gap::getAppearance(). A former call to 01182 * ble.getAppearance() should be replaced with 01183 * ble.gap().getAppearance(). 01184 */ 01185 MBED_DEPRECATED("Use ble.gap().getAppearance(...)") 01186 ble_error_t getAppearance(GapAdvertisingData::Appearance *appearanceP) { 01187 return gap().getAppearance(appearanceP); 01188 } 01189 01190 /** 01191 * Set the radio's transmit power. 01192 * @param[in] txPower Radio transmit power in dBm. 01193 * 01194 * @deprecated You should use the parallel API from Gap directly and refer to 01195 * Gap::setTxPower(). A former call to 01196 * ble.setTxPower() should be replaced with 01197 * ble.gap().setTxPower(). 01198 */ 01199 MBED_DEPRECATED("Use ble.gap().setTxPower(...)") 01200 ble_error_t setTxPower(int8_t txPower) { 01201 return gap().setTxPower(txPower); 01202 } 01203 01204 /** 01205 * Query the underlying stack for permitted arguments for setTxPower(). 01206 * 01207 * @param[out] valueArrayPP 01208 * Out parameter to receive the immutable array of Tx values. 01209 * @param[out] countP 01210 * Out parameter to receive the array's size. 01211 * 01212 * @deprecated You should use the parallel API from Gap directly, refer to 01213 * Gap::getPermittedTxPowerValues(). A former call to 01214 * ble.getPermittedTxPowerValues() should be replaced with 01215 * ble.gap().getPermittedTxPowerValues(). 01216 */ 01217 MBED_DEPRECATED("Use ble.gap().getPermittedTxPowerValues(...)") 01218 void getPermittedTxPowerValues(const int8_t **valueArrayPP, size_t *countP) { 01219 gap().getPermittedTxPowerValues(valueArrayPP, countP); 01220 } 01221 01222 /** 01223 * Add a service declaration to the local server ATT table. Also add the 01224 * characteristics contained within. 01225 * 01226 * @deprecated You should use the parallel API from GattServer directly, refer to 01227 * GattServer::addService(). A former call 01228 * to ble.addService() should be replaced with 01229 * ble.gattServer().addService(). 01230 */ 01231 MBED_DEPRECATED("Use ble.gattServer().addService(...)") 01232 ble_error_t addService(GattService &service) { 01233 return gattServer().addService(service); 01234 } 01235 01236 /** 01237 * Read the value of a characteristic from the local GattServer. 01238 * @param[in] attributeHandle 01239 * Attribute handle for the value attribute of the characteristic. 01240 * @param[out] buffer 01241 * A buffer to hold the value being read. 01242 * @param[in,out] lengthP 01243 * Length of the buffer being supplied. If the attribute 01244 * value is longer than the size of the supplied buffer, 01245 * this variable will return the total attribute value length 01246 * (excluding offset). The application may use this 01247 * information to allocate a suitable buffer size. 01248 * 01249 * @return BLE_ERROR_NONE if a value was read successfully into the buffer. 01250 * 01251 * @deprecated You should use the parallel API from GattServer directly, 01252 * GattServer::read(GattAttribute::Handle_t,uint8_t,uint16_t). A former call 01253 * to ble.readCharacteristicValue() should be replaced with 01254 * ble.gattServer().read(). 01255 */ 01256 MBED_DEPRECATED("Use ble.gattServer().read(...)") 01257 ble_error_t readCharacteristicValue(GattAttribute::Handle_t attributeHandle, uint8_t *buffer, uint16_t *lengthP) { 01258 return gattServer().read(attributeHandle, buffer, lengthP); 01259 } 01260 01261 /** 01262 * Read the value of a characteristic from the local GattServer. 01263 * @param[in] connectionHandle 01264 * Connection Handle. 01265 * @param[in] attributeHandle 01266 * Attribute handle for the value attribute of the characteristic. 01267 * @param[out] buffer 01268 * A buffer to hold the value being read. 01269 * @param[in,out] lengthP 01270 * Length of the buffer being supplied. If the attribute 01271 * value is longer than the size of the supplied buffer, 01272 * this variable will return the total attribute value length 01273 * (excluding offset). The application may use this 01274 * information to allocate a suitable buffer size. 01275 * 01276 * @return BLE_ERROR_NONE if a value was read successfully into the buffer. 01277 * 01278 * @note This API is a version of the above, with an additional connection handle 01279 * parameter to allow fetches for connection-specific multivalued 01280 * attributes (such as the CCCDs). 01281 * 01282 * @deprecated You should use the parallel API from GattServer directly, refer to 01283 * GattServer::read(Gap::Handle_t,GattAttribute::Handle_t,uint8_t,uint16_t). 01284 * A former call to ble.readCharacteristicValue() should be replaced with 01285 * ble.gattServer().read(). 01286 */ 01287 MBED_DEPRECATED("Use ble.gattServer().read(...)") 01288 ble_error_t readCharacteristicValue(Gap::Handle_t connectionHandle, GattAttribute::Handle_t attributeHandle, uint8_t *buffer, uint16_t *lengthP) { 01289 return gattServer().read(connectionHandle, attributeHandle, buffer, lengthP); 01290 } 01291 01292 /** 01293 * Update the value of a characteristic on the local GattServer. 01294 * 01295 * @param[in] attributeHandle 01296 * Handle for the value attribute of the characteristic. 01297 * @param[in] value 01298 * A pointer to a buffer holding the new value. 01299 * @param[in] size 01300 * Size of the new value (in bytes). 01301 * @param[in] localOnly 01302 * Should this update be kept on the local 01303 * GattServer regardless of the state of the 01304 * notify/indicate flag in the CCCD for this 01305 * characteristic? If set to true, no notification 01306 * or indication is generated. 01307 * 01308 * @return BLE_ERROR_NONE if we have successfully set the value of the attribute. 01309 * 01310 * @deprecated You should use the parallel API from GattServer directly and refer to 01311 * GattServer::write(GattAttribute::Handle_t,const uint8_t,uint16_t,bool). 01312 * A former call to ble.updateCharacteristicValue() should be replaced with 01313 * ble.gattServer().write(). 01314 */ 01315 MBED_DEPRECATED("Use ble.gattServer().write(...)") 01316 ble_error_t updateCharacteristicValue(GattAttribute::Handle_t attributeHandle, 01317 const uint8_t *value, 01318 uint16_t size, 01319 bool localOnly = false) { 01320 return gattServer().write(attributeHandle, value, size, localOnly); 01321 } 01322 01323 /** 01324 * Update the value of a characteristic on the local GattServer. A version 01325 * of the above, with a connection handle parameter to allow updates 01326 * for connection-specific multivalued attributes (such as the CCCDs). 01327 * 01328 * @param[in] connectionHandle 01329 * Connection Handle. 01330 * @param[in] attributeHandle 01331 * Handle for the value attribute of the Characteristic. 01332 * @param[in] value 01333 * A pointer to a buffer holding the new value. 01334 * @param[in] size 01335 * Size of the new value (in bytes). 01336 * @param[in] localOnly 01337 * Should this update be kept on the local 01338 * GattServer regardless of the state of the 01339 * notify/indicate flag in the CCCD for this 01340 * Characteristic? If set to true, no notification 01341 * or indication is generated. 01342 * 01343 * @return BLE_ERROR_NONE if we have successfully set the value of the attribute. 01344 * 01345 * @deprecated You should use the parallel API from GattServer directly and refer to 01346 * GattServer::write(Gap::Handle_t,GattAttribute::Handle_t,const uint8_t,uint16_t,bool). 01347 * A former call to ble.updateCharacteristicValue() should be replaced with 01348 * ble.gattServer().write(). 01349 */ 01350 MBED_DEPRECATED("Use ble.gattServer().write(...)") 01351 ble_error_t updateCharacteristicValue(Gap::Handle_t connectionHandle, 01352 GattAttribute::Handle_t attributeHandle, 01353 const uint8_t *value, 01354 uint16_t size, 01355 bool localOnly = false) { 01356 return gattServer().write(connectionHandle, attributeHandle, value, size, localOnly); 01357 } 01358 01359 /** 01360 * Enable the BLE stack's Security Manager. The Security Manager implements 01361 * the cryptographic algorithms and protocol exchanges that allow two 01362 * devices to securely exchange data and privately detect each other. 01363 * Calling this API is a prerequisite for encryption and pairing (bonding). 01364 * 01365 * @param[in] enableBonding Allow for bonding. 01366 * @param[in] requireMITM Require protection against man-in-the-middle attacks. 01367 * @param[in] iocaps To specify the I/O capabilities of this peripheral, 01368 * such as availability of a display or keyboard, to 01369 * support out-of-band exchanges of security data. 01370 * @param[in] passkey To specify a static passkey. 01371 * 01372 * @return BLE_ERROR_NONE on success. 01373 * 01374 * @deprecated You should use the parallel API from SecurityManager directly, refer to 01375 * SecurityManager.init(). A former 01376 * call to ble.initializeSecurity(...) should be replaced with 01377 * ble.securityManager().init(...). 01378 */ 01379 MBED_DEPRECATED("Use ble.gattServer().write(...)") 01380 ble_error_t initializeSecurity(bool enableBonding = true, 01381 bool requireMITM = true, 01382 SecurityManager::SecurityIOCapabilities_t iocaps = SecurityManager::IO_CAPS_NONE, 01383 const SecurityManager::Passkey_t passkey = NULL) { 01384 return securityManager().init(enableBonding, requireMITM, iocaps, passkey); 01385 } 01386 01387 /** 01388 * Get the security status of a connection. 01389 * 01390 * @param[in] connectionHandle Handle to identify the connection. 01391 * @param[out] securityStatusP Security status. 01392 * 01393 * @return BLE_SUCCESS or appropriate error code indicating the reason of failure. 01394 * 01395 * @deprecated You should use the parallel API from SecurityManager directly, refer to 01396 * SecurityManager::getLinkSecurity(). A former 01397 * call to ble.getLinkSecurity(...) should be replaced with 01398 * ble.securityManager().getLinkSecurity(...). 01399 */ 01400 MBED_DEPRECATED("ble.securityManager().getLinkSecurity(...)") 01401 ble_error_t getLinkSecurity(Gap::Handle_t connectionHandle, SecurityManager::LinkSecurityStatus_t *securityStatusP) { 01402 return securityManager().getLinkSecurity(connectionHandle, securityStatusP); 01403 } 01404 01405 /** 01406 * Delete all peer device context and all related bonding information from 01407 * the database within the security manager. 01408 * 01409 * @retval BLE_ERROR_NONE On success; else returns an error code indicating the reason for the failure. 01410 * @retval BLE_ERROR_INVALID_STATE If the API is called without module initialization or 01411 * application registration. 01412 * 01413 * @deprecated You should use the parallel API from SecurityManager directly and refer to 01414 * SecurityManager::purgeAllBondingState(). A former 01415 * call to ble.purgeAllBondingState() should be replaced with 01416 * ble.securityManager().purgeAllBondingState(). 01417 */ 01418 MBED_DEPRECATED("ble.securityManager().purgeAllBondingState(...)") 01419 ble_error_t purgeAllBondingState(void) { 01420 return securityManager().purgeAllBondingState(); 01421 } 01422 01423 /** 01424 * Set up a callback for timeout events. Refer to Gap::TimeoutSource_t for 01425 * possible event types. 01426 * 01427 * @deprecated You should use the parallel API from Gap directly and refer to 01428 * Gap::onTimeout(). A former call 01429 * to ble.onTimeout(callback) should be replaced with 01430 * ble.gap().onTimeout(callback). 01431 */ 01432 MBED_DEPRECATED("ble.gap().onTimeout(callback)") 01433 void onTimeout(Gap::TimeoutEventCallback_t timeoutCallback) { 01434 gap().onTimeout(timeoutCallback); 01435 } 01436 01437 /** 01438 * Set up a callback for connection events. Refer to Gap::ConnectionEventCallback_t. 01439 * 01440 * @deprecated You should use the parallel API from Gap directly, refer to 01441 * Gap::onConnection(). A former call 01442 * to ble.onConnection(callback) should be replaced with 01443 * ble.gap().onConnection(callback). 01444 */ 01445 MBED_DEPRECATED("ble.gap().onConnection(callback)") 01446 void onConnection(Gap::ConnectionEventCallback_t connectionCallback) { 01447 gap().onConnection(connectionCallback); 01448 } 01449 01450 /** 01451 * Append to a chain of callbacks to be invoked upon GAP disconnection. 01452 * 01453 * @deprecated You should use the parallel API from Gap directly and refer to 01454 * Gap::onDisconnection(). A former call 01455 * to ble.onDisconnection(callback) should be replaced with 01456 * ble.gap().onDisconnection(callback). 01457 */ 01458 MBED_DEPRECATED("ble.gap().onDisconnection(callback)") 01459 void onDisconnection(Gap::DisconnectionEventCallback_t disconnectionCallback) { 01460 gap().onDisconnection(disconnectionCallback); 01461 } 01462 01463 /** 01464 * The same as onDisconnection() but allows an object reference and member function 01465 * to be added to the chain of callbacks. 01466 * 01467 * @deprecated You should use the parallel API from Gap directly and refer to 01468 * Gap::onDisconnection(). A former call 01469 * to ble.onDisconnection(callback) should be replaced with 01470 * ble.gap().onDisconnection(callback). 01471 */ 01472 template<typename T> 01473 MBED_DEPRECATED("ble.gap().onDisconnection(callback)") 01474 void onDisconnection(T *tptr, void (T::*mptr)(const Gap::DisconnectionCallbackParams_t*)) { 01475 gap().onDisconnection(tptr, mptr); 01476 } 01477 01478 /** 01479 * Radio Notification is a feature that enables ACTIVE and INACTIVE 01480 * (nACTIVE) signals from the stack. These notify the application when the 01481 * radio is in use. The signal is sent using software interrupt. 01482 * 01483 * The ACTIVE signal is sent before the radio event starts. The nACTIVE 01484 * signal is sent at the end of the radio event. These signals can be used 01485 * by the application programmer to synchronize application logic with radio 01486 * activity. For example, the ACTIVE signal can be used to shut off external 01487 * devices to manage peak current drawn during periods when the radio is on, 01488 * or to trigger sensor data collection for transmission in the radio event. 01489 * 01490 * @param callback 01491 * The application handler to be invoked in response to a radio 01492 * ACTIVE/INACTIVE event. 01493 * 01494 * @deprecated You should use the parallel API from Gap directly, refer to 01495 * Gap::onRadioNotification(). A former call 01496 * to ble.onRadioNotification(...) should be replaced with 01497 * ble.gap().onRadioNotification(...). 01498 */ 01499 MBED_DEPRECATED("ble.gap().onRadioNotification(...)") 01500 void onRadioNotification(void (*callback)(bool)) { 01501 gap().onRadioNotification(callback); 01502 } 01503 01504 /** 01505 * Add a callback for the GATT event DATA_SENT (which is triggered when 01506 * updates are sent out by GATT in the form of notifications). 01507 * 01508 * @note It is possible to chain together multiple onDataSent callbacks 01509 * (potentially from different modules of an application) to receive updates 01510 * to characteristics. 01511 * 01512 * @note It is also possible to set up a callback into a member function of 01513 * some object. 01514 * 01515 * @deprecated You should use the parallel API from GattServer directly and refer to 01516 * GattServer::onDataSent(). A former call 01517 * to ble.onDataSent(...) should be replaced with 01518 * ble.gattServer().onDataSent(...). 01519 */ 01520 MBED_DEPRECATED("ble.gattServer().onDataSent(...)") 01521 void onDataSent(void (*callback)(unsigned count)) { 01522 gattServer().onDataSent(callback); 01523 } 01524 01525 /** 01526 * The same as onDataSent() but allows an object reference and member function 01527 * to be added to the chain of callbacks. 01528 * 01529 * @deprecated You should use the parallel API from GattServer directly and refer to 01530 * GattServer::onDataSent(). A former call 01531 * to ble.onDataSent(...) should be replaced with 01532 * ble.gattServer().onDataSent(...). 01533 */ 01534 template <typename T> 01535 MBED_DEPRECATED("ble.gattServer().onDataSent(...)") 01536 void onDataSent(T * objPtr, void (T::*memberPtr)(unsigned count)) { 01537 gattServer().onDataSent(objPtr, memberPtr); 01538 } 01539 01540 /** 01541 * Set up a callback for when an attribute has its value updated by or at the 01542 * connected peer. For a peripheral, this callback is triggered when the local 01543 * GATT server has an attribute updated by a write command from the peer. 01544 * For a Central, this callback is triggered when a response is received for 01545 * a write request. 01546 * 01547 * @note It is possible to chain together multiple onDataWritten callbacks 01548 * (potentially from different modules of an application) to receive updates 01549 * to characteristics. Many services, such as DFU and UART, add their own 01550 * onDataWritten callbacks behind the scenes to trap interesting events. 01551 * 01552 * @note It is also possible to set up a callback into a member function of 01553 * some object. 01554 * 01555 * @deprecated You should use the parallel API from GattServer directly and refer to 01556 * GattServer::onDataWritten(). A former call 01557 * to ble.onDataWritten(...) should be replaced with 01558 * ble.gattServer().onDataWritten(...). 01559 */ 01560 MBED_DEPRECATED("ble.gattServer().onDataWritten(...)") 01561 void onDataWritten(void (*callback)(const GattWriteCallbackParams *eventDataP)) { 01562 gattServer().onDataWritten(callback); 01563 } 01564 01565 /** 01566 * The same as onDataWritten() but allows an object reference and member function 01567 * to be added to the chain of callbacks. 01568 * 01569 * @deprecated You should use the parallel API from GattServer directly, refer to 01570 * GattServer::onDataWritten(). A former call 01571 * to ble.onDataWritten(...) should be replaced with 01572 * ble.gattServer().onDataWritten(...). 01573 */ 01574 template <typename T> 01575 MBED_DEPRECATED("ble.gattServer().onDataWritten(...)") 01576 void onDataWritten(T * objPtr, void (T::*memberPtr)(const GattWriteCallbackParams *context)) { 01577 gattServer().onDataWritten(objPtr, memberPtr); 01578 } 01579 01580 /** 01581 * Set up a callback to be invoked on the peripheral when an attribute is 01582 * being read by a remote client. 01583 * 01584 * @note This functionality may not be available on all underlying stacks. 01585 * You could use GattCharacteristic::setReadAuthorizationCallback() as an 01586 * alternative. 01587 * 01588 * @note It is possible to chain together multiple onDataRead callbacks 01589 * (potentially from different modules of an application) to receive updates 01590 * to characteristics. Services may add their own onDataRead callbacks 01591 * behind the scenes to trap interesting events. 01592 * 01593 * @note It is also possible to set up a callback into a member function of 01594 * some object. 01595 * 01596 * @return BLE_ERROR_NOT_IMPLEMENTED if this functionality isn't available; 01597 * else BLE_ERROR_NONE. 01598 * 01599 * @deprecated You should use the parallel API from GattServer directly and refer to 01600 * GattServer::onDataRead(). A former call 01601 * to ble.onDataRead(...) should be replaced with 01602 * ble.gattServer().onDataRead(...). 01603 */ 01604 MBED_DEPRECATED("ble.gattServer().onDataRead(...)") 01605 ble_error_t onDataRead(void (*callback)(const GattReadCallbackParams *eventDataP)) { 01606 return gattServer().onDataRead(callback); 01607 } 01608 01609 /** 01610 * The same as onDataRead() but allows an object reference and member function 01611 * to be added to the chain of callbacks. 01612 * 01613 * @deprecated You should use the parallel API from GattServer directly and refer to 01614 * GattServer::onDataRead(). A former call 01615 * to ble.onDataRead(...) should be replaced with 01616 * ble.gattServer().onDataRead(...). 01617 */ 01618 template <typename T> 01619 MBED_DEPRECATED("ble.gattServer().onDataRead(...)") 01620 ble_error_t onDataRead(T * objPtr, void (T::*memberPtr)(const GattReadCallbackParams *context)) { 01621 return gattServer().onDataRead(objPtr, memberPtr); 01622 } 01623 01624 /** 01625 * Set up a callback for when notifications or indications are enabled for a 01626 * characteristic on the local GattServer. 01627 * 01628 * @deprecated You should use the parallel API from GattServer directly and refer to 01629 * GattServer::onUpdatesEnabled(). A former call 01630 * to ble.onUpdatesEnabled(callback) should be replaced with 01631 * ble.gattServer().onUpdatesEnabled(callback). 01632 */ 01633 MBED_DEPRECATED("ble.gattServer().onUpdatesEnabled(...)") 01634 void onUpdatesEnabled(GattServer::EventCallback_t callback) { 01635 gattServer().onUpdatesEnabled(callback); 01636 } 01637 01638 /** 01639 * Set up a callback for when notifications or indications are disabled for a 01640 * characteristic on the local GattServer. 01641 * 01642 * @deprecated You should use the parallel API from GattServer directly and refer to 01643 * GattServer::onUpdatesDisabled(). A former call 01644 * to ble.onUpdatesDisabled(callback) should be replaced with 01645 * ble.gattServer().onUpdatesDisabled(callback). 01646 */ 01647 MBED_DEPRECATED("ble.gattServer().onUpdatesDisabled(...)") 01648 void onUpdatesDisabled(GattServer::EventCallback_t callback) { 01649 gattServer().onUpdatesDisabled(callback); 01650 } 01651 01652 /** 01653 * Set up a callback for when the GATT server receives a response for an 01654 * indication event sent previously. 01655 * 01656 * @deprecated You should use the parallel API from GattServer directly and refer to 01657 * GattServer::onConfirmationReceived(). A former call 01658 * to ble.onConfirmationReceived(callback) should be replaced with 01659 * ble.gattServer().onConfirmationReceived(callback). 01660 */ 01661 MBED_DEPRECATED("ble.gattServer().onConfirmationReceived(...)") 01662 void onConfirmationReceived(GattServer::EventCallback_t callback) { 01663 gattServer().onConfirmationReceived(callback); 01664 } 01665 01666 /** 01667 * Set up a callback for when the security setup procedure (key generation 01668 * and exchange) for a link has started. This will be skipped for bonded 01669 * devices. The callback is passed in parameters received from the peer's 01670 * security request: bool allowBonding, bool requireMITM and 01671 * SecurityIOCapabilities_t. 01672 * 01673 * @deprecated You should use the parallel API from SecurityManager directly and refer to 01674 * SecurityManager::onSecuritySetupInitiated(). A former 01675 * call to ble.onSecuritySetupInitiated(callback) should be replaced with 01676 * ble.securityManager().onSecuritySetupInitiated(callback). 01677 */ 01678 MBED_DEPRECATED("ble.securityManager().onSecuritySetupInitiated(callback)") 01679 void onSecuritySetupInitiated(SecurityManager::SecuritySetupInitiatedCallback_t callback) { 01680 securityManager().onSecuritySetupInitiated(callback); 01681 } 01682 01683 /** 01684 * Set up a callback for when the security setup procedure (key generation 01685 * and exchange) for a link has completed. This will be skipped for bonded 01686 * devices. The callback is passed in the success/failure status of the 01687 * security setup procedure. 01688 * 01689 * @deprecated You should use the parallel API from SecurityManager directly and refer to 01690 * SecurityManager::onSecuritySetupCompleted(). A former 01691 * call to ble.onSecuritySetupCompleted(callback) should be replaced with 01692 * ble.securityManager().onSecuritySetupCompleted(callback). 01693 */ 01694 MBED_DEPRECATED("ble.securityManager().onSecuritySetupCompleted(callback)") 01695 void onSecuritySetupCompleted(SecurityManager::SecuritySetupCompletedCallback_t callback) { 01696 securityManager().onSecuritySetupCompleted(callback); 01697 } 01698 01699 /** 01700 * Set up a callback for when a link with the peer is secured. For bonded 01701 * devices, subsequent reconnections with a bonded peer will result only in 01702 * this callback when the link is secured, and setup procedures will not 01703 * occur unless the bonding information is either lost or deleted on either 01704 * or both sides. The callback is passed in a SecurityManager::SecurityMode_t according 01705 * to the level of security in effect for the secured link. 01706 * 01707 * @deprecated You should use the parallel API from SecurityManager directly and refer to 01708 * SecurityManager::onLinkSecured(). A former 01709 * call to ble.onLinkSecured(callback) should be replaced with 01710 * ble.securityManager().onLinkSecured(callback). 01711 */ 01712 MBED_DEPRECATED("ble.securityManager().onLinkSecured(callback)") 01713 void onLinkSecured(SecurityManager::LinkSecuredCallback_t callback) { 01714 securityManager().onLinkSecured(callback); 01715 } 01716 01717 /** 01718 * Set up a callback for successful bonding, meaning that link-specific security 01719 * context is stored persistently for a peer device. 01720 * 01721 * @deprecated You should use the parallel API from SecurityManager directly and refer to 01722 * SecurityManager::onSecurityContextStored(). A former 01723 * call to ble.onSecurityContextStored(callback) should be replaced with 01724 * ble.securityManager().onSecurityContextStored(callback). 01725 */ 01726 MBED_DEPRECATED("ble.securityManager().onSecurityContextStored(callback)") 01727 void onSecurityContextStored(SecurityManager::HandleSpecificEvent_t callback) { 01728 securityManager().onSecurityContextStored(callback); 01729 } 01730 01731 /** 01732 * Set up a callback for when the passkey needs to be displayed on a 01733 * peripheral with DISPLAY capability. This happens when security is 01734 * configured to prevent man-in-the-middle attacks, and the peers need to exchange 01735 * a passkey (or PIN) to authenticate the connection 01736 * attempt. 01737 * 01738 * @deprecated You should use the parallel API from SecurityManager directly and refer to 01739 * SecurityManager::onPasskeyDisplay(). A former 01740 * call to ble.onPasskeyDisplay(callback) should be replaced with 01741 * ble.securityManager().onPasskeyDisplay(callback). 01742 */ 01743 MBED_DEPRECATED("ble.securityManager().onPasskeyDisplay(callback)") 01744 void onPasskeyDisplay(SecurityManager::PasskeyDisplayCallback_t callback) { 01745 return securityManager().onPasskeyDisplay(callback); 01746 } 01747 01748 private: 01749 friend class BLEInstanceBase; 01750 01751 /** 01752 * This function allows the BLE stack to signal that there is work to do and 01753 * event processing should be done (BLE::processEvent()). 01754 * 01755 * @note This function should be called by the port of BLE_API. It is not 01756 * meant to be used by end users. 01757 */ 01758 void signalEventsToProcess(); 01759 01760 /** 01761 * Implementation of init() [internal to BLE_API]. 01762 * 01763 * The implementation is separated into a private method because it isn't 01764 * suitable to be included in the header. 01765 */ 01766 ble_error_t initImplementation( 01767 FunctionPointerWithContext<InitializationCompleteCallbackContext *> callback 01768 ); 01769 01770 private: 01771 // Prevent copy construction and copy assignment of BLE. 01772 BLE(const BLE&); 01773 BLE &operator=(const BLE &); 01774 01775 private: 01776 InstanceID_t instanceID; 01777 BLEInstanceBase *transport; /* The device-specific backend */ 01778 OnEventsToProcessCallback_t whenEventsToProcess; 01779 bool event_signaled; 01780 }; 01781 01782 /** 01783 * @deprecated This type alias is retained for the sake of compatibility with 01784 * older code. This will be dropped at some point. 01785 */ 01786 typedef BLE BLEDevice ; 01787 01788 /** 01789 * @namespace ble Entry namespace for all %BLE API definitions. 01790 */ 01791 01792 /** 01793 * @} 01794 */ 01795 01796 #endif /* ifndef MBED_BLE_H__ */
Generated on Sun Jul 17 2022 08:25:20 by
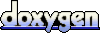