
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
AttClient.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_PAL_ATTCLIENT_H_ 00018 #define BLE_PAL_ATTCLIENT_H_ 00019 00020 #include "ble/UUID.h " 00021 #include "ble/BLETypes.h" 00022 #include "ble/ArrayView.h " 00023 #include "ble/blecommon.h" 00024 #include "platform/Callback.h" 00025 #include "AttServerMessage.h" 00026 00027 namespace ble { 00028 namespace pal { 00029 00030 /** 00031 * Send attribute protocol requests to an ATT server. It also handle reception 00032 * of ATT response and server indication/notification. 00033 * 00034 * Every request send and response or response event received is for a specified 00035 * connection. 00036 * 00037 * @warning This class should not be used outside mbed BLE, availability is not 00038 * guaranteed for all ports. 00039 * 00040 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F 00041 */ 00042 struct AttClient { 00043 /** 00044 * Initialization of the instance. An implementation can use this function 00045 * to initialise the subsystems needed to realize the ATT operations of this 00046 * interface. 00047 * 00048 * This function has to be called before any other operations. 00049 * 00050 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00051 * appropriate error otherwise. 00052 */ 00053 virtual ble_error_t initialize() = 0; 00054 00055 /** 00056 * Termination of the instance. An implementation can use this function 00057 * to release the subsystems initialised to realise the ATT operations of 00058 * this interface. 00059 * 00060 * After a call to this function, initialise should be called again to 00061 * allow usage of the interface. 00062 * 00063 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00064 * appropriate error otherwise. 00065 */ 00066 virtual ble_error_t terminate() = 0; 00067 00068 /** 00069 * Send an exchange MTU request which negotiate the size of the MTU used by 00070 * the connection. 00071 * 00072 * First the client send to the server the maximum rx mtu that it can receive 00073 * then the client reply with the maximum rx mtu it can receive. 00074 * 00075 * The mtu choosen for the connection is the minimum of the client Rx mtu 00076 * and server Rx mtu values. 00077 * 00078 * If an error occured then the mtu used remains the default value. 00079 * 00080 * @param connection The handle of the connection to send this request to. 00081 * 00082 * @return BLE_ERROR_NONE if the request has been succesfully sent or the 00083 * appropriate error otherwise. 00084 * 00085 * @see ble::pal::AttExchangeMTUResponse The type of response received from 00086 * the server 00087 * @see ble::pal::AttErrorResponse::REQUEST_NOT_SUPPORTED The error code 00088 * returned by the server in case of error. 00089 * 00090 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.2.1 00091 */ 00092 virtual ble_error_t exchange_mtu_request(connection_handle_t connection) = 0; 00093 00094 /** 00095 * Acquire the size of the mtu for a given connection. 00096 * 00097 * @param connection The handle of the connection for which the the MTU size 00098 * should be acquired. 00099 * 00100 * @param mtu_size Output parameter which will contain the MTU size. 00101 * 00102 * @return BLE_ERROR_NONE if the MTU size has been acquired or the 00103 * appropriate error otherwise. 00104 */ 00105 virtual ble_error_t get_mtu_size( 00106 connection_handle_t connection_handle, 00107 uint16_t& mtu_size 00108 ) = 0; 00109 00110 /** 00111 * Send a find information request to a server in order to obtain the 00112 * mapping of attribute handles with their associated types. 00113 * 00114 * The server will reply with a ble::pal::AttFindInformationResponse 00115 * containing at least one [attribute handle, attribute type] pair. If the 00116 * last handle in the response is not equal to the end handle of the finding 00117 * range then this request can be issued again with an updated range (begin 00118 * equal to last handle received + 1) to discover the remaining attributes. 00119 * 00120 * To discover the whole ATT server, the first find information request 00121 * should have a discovery range of [0x0001 - 0xFFFF]. 00122 * 00123 * The server can send a ble::pal::AttErrorResponse with the code 00124 * ble::pal::AttErrorResponse::ATTRIBUTE_NOT_FOUND if no attributes have 00125 * been found in the range specified. The attribute handle in the response 00126 * is then equal to the first handle of the discovery range. 00127 * 00128 * If the range is malformed the server will reply a 00129 * ble::pal::AttErrorResponse with the error code ble::pal::INVALID_HANDLE. 00130 * 00131 * @param connection_handle The handle of the connection to send this 00132 * request to. 00133 * @param discovery_range The attribute range where handle-type informations 00134 * should be discovered. 00135 * 00136 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00137 * appropriate error otherwise. 00138 * 00139 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.3.1 00140 */ 00141 virtual ble_error_t find_information_request( 00142 connection_handle_t connection_handle, 00143 attribute_handle_range_t discovery_range 00144 ) = 0; 00145 00146 /** 00147 * Send a Find By Type Value Request which retrieve the handles of attributes 00148 * that have known 16-bit UUID attribute type and known attribute value. 00149 * 00150 * The server should reply with a ble::pal::AttFindByTypeValueResponse 00151 * containing the handle (or handle range in case of grouping attributes) of 00152 * the attribute found. 00153 * 00154 * If not all attributes can be contained in the response it is necessary to 00155 * send again this request with an updated range to continue the discovery. 00156 * 00157 * The server can send a ble::pal::AttErrorResponse with the code 00158 * ble::pal::AttErrorResponse::ATTRIBUTE_NOT_FOUND if no attributes have 00159 * been found in the range specified. The attribute handle in the response 00160 * is then equal to the first handle of the discovery range. 00161 * 00162 * If the range is malformed the server will reply a 00163 * ble::pal::AttErrorResponse with the error code ble::pal::INVALID_HANDLE. 00164 * 00165 * @param connection_handle The handle of the connection to send this 00166 * request to. 00167 * @param discovery_range The handle range where attributes with type and 00168 * value are searched. 00169 * @param type The type of attribute to find (it is a 16 bit UUID). 00170 * @param value The value of the attributes to found. 00171 * 00172 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00173 * appropriate error otherwise. 00174 * 00175 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.3.3 00176 */ 00177 virtual ble_error_t find_by_type_value_request( 00178 connection_handle_t connection_handle, 00179 attribute_handle_range_t discovery_range, 00180 uint16_t type, 00181 const ArrayView<const uint8_t> & value 00182 ) = 0; 00183 00184 /** 00185 * Send a Read By Type Request used to obtain the values of attributes where 00186 * the attribute type is known but the handle is not known. 00187 * 00188 * If attributes with the type requested are present in the range, the server 00189 * should reply with a ble::pal::AttReadByTypeResponse. If the response does 00190 * not cover the full range, the request should be sent again with an updated 00191 * range. 00192 * 00193 * In case of error, the server will send a ble::pal::AttErrorResponse. The 00194 * error code depends on the situation: 00195 * - ble::pal::AttErrorResponse::ATTRIBUTE_NOT_FOUND: If there is no 00196 * attributes matching type in the range. 00197 * - ble::pal::AttErrorResponse::INVALID_HANDLE: If the range is 00198 * invalid. 00199 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHENTICATION: If the client 00200 * security is not sufficient. 00201 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHORIZATION: If the client 00202 * authorization is not sufficient. 00203 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION_KEY_SIZE: If the 00204 * client has an insufficient encryption key size. 00205 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION: If the client 00206 * has not enabled encryption. 00207 * - ble::pal::AttErrorResponse::READ_NOT_PERMITTED: If the attribute 00208 * value cannot be read. 00209 * 00210 * @param connection_handle The handle of the connection to send this 00211 * request to. 00212 * @param read_range The handle range where attributes with the given type 00213 * should be read. 00214 * @param type The type of attributes to read. 00215 * 00216 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00217 * appropriate error otherwise. 00218 * 00219 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.1 00220 */ 00221 virtual ble_error_t read_by_type_request( 00222 connection_handle_t connection_handle, 00223 attribute_handle_range_t read_range, 00224 const UUID& type 00225 ) = 0; 00226 00227 /** 00228 * Send a Read Request to read the value of an attribute in the server. 00229 * 00230 * In case of success, the server will reply with a ble::pal::AttReadResponse. 00231 * containing the value of the attribute. If the length of the value in the 00232 * response is equal to (mtu - 1) then the remaining part of the value can 00233 * be obtained by a read_blob_request. 00234 * 00235 * In case of error, the server will send a ble::pal::AttErrorResponse. The 00236 * error code depends on the situation: 00237 * - ble::pal::AttErrorResponse::INVALID_HANDLE: If the attribute handle 00238 * is invalid. 00239 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHENTICATION: If the client 00240 * security is not sufficient. 00241 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHORIZATION: If the client 00242 * authorization is not sufficient. 00243 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION_KEY_SIZE: If the 00244 * client has an insufficient encryption key size. 00245 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION: If the client 00246 * has not enabled encryption. 00247 * - ble::pal::AttErrorResponse::READ_NOT_PERMITTED: If the attribute 00248 * value cannot be read. 00249 * Higher layer can also set an application error code (0x80 - 0x9F). 00250 * 00251 * @param connection_handle The handle of the connection to send this 00252 * request to. 00253 * @param attribute_handle The handle of the attribute to read. 00254 * 00255 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00256 * appropriate error otherwise. 00257 * 00258 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.3 00259 */ 00260 virtual ble_error_t read_request( 00261 connection_handle_t connection_handle, 00262 attribute_handle_t attribute_handle 00263 ) = 0; 00264 00265 /** 00266 * Send a read blob request to a server to read a part of the value of an 00267 * attribute at a given offset. 00268 * 00269 * In case of success, the server will reply with a ble::pal::AttReadBlobResponse 00270 * containing the value read. If the value of the attribute starting at the 00271 * offset requested is longer than (mtu - 1) octets then only the first 00272 * (mtu - 1) octets will be present in the response. 00273 * The remaining octets can be acquired by another Read Blob Request with an 00274 * updated index. 00275 * 00276 * In case of error, the server will send a ble::pal::AttErrorResponse. The 00277 * error code depends on the situation: 00278 * - ble::pal::AttErrorResponse::INVALID_HANDLE: If the attribute handle 00279 * is invalid. 00280 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHENTICATION: If the client 00281 * security is not sufficient. 00282 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHORIZATION: If the client 00283 * authorization is not sufficient. 00284 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION_KEY_SIZE: If the 00285 * client has an insufficient encryption key size. 00286 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION: If the client 00287 * has not enabled encryption. 00288 * - ble::pal::AttErrorResponse::READ_NOT_PERMITTED: If the attribute 00289 * value cannot be read. 00290 * - ble::pal::AttErrorResponse::INVALID_OFFSET: If the offset is greater 00291 * than the attribute length. 00292 * - ble::pal::AttErrorResponse::ATTRIBUTE_NOT_LONG: If the attribute 00293 * value has a length that is less than or equal to (mtu - 1). 00294 * Higher layer can also set an application error code (0x80 - 0x9F). 00295 * 00296 * @param connection_handle The handle of the connection to send this 00297 * request to. 00298 * @param attribute_handle The handle of the attribute to read. 00299 * @param offset The offset of the first octet to read. 00300 * 00301 * @return BLE_ERROR_NONE if the request has been successfully sent or an 00302 * appropriate error otherwise. 00303 * 00304 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.5 00305 */ 00306 virtual ble_error_t read_blob_request( 00307 connection_handle_t connection_handle, 00308 attribute_handle_t attribute_handle, 00309 uint16_t offset 00310 ) = 0; 00311 00312 /** 00313 * Send a read multiple request to the server. It is used to read two or more 00314 * attributes values at once. 00315 * 00316 * In case of success, the server will reply with a 00317 * ble::pal::AttReadMultipleResponse containing the concatenation of the 00318 * values read. Given that values are concatained, all attributes values 00319 * should be of fixed size except for the last one. The concatained value 00320 * is also truncated to (mtu - 1) if it doesn't fit in the response. 00321 * 00322 * In case of error, the server will send a ble::pal::AttErrorResponse. The 00323 * error code depends on the situation: 00324 * - ble::pal::AttErrorResponse::INVALID_HANDLE: If any of the attribute 00325 * handle is invalid. 00326 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHENTICATION: If the client 00327 * security is not sufficient to read any of the attribute. 00328 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHORIZATION: If the client 00329 * authorization is not sufficient to read any of the attribute. 00330 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION_KEY_SIZE: If the 00331 * client has an insufficient encryption key size to read any of the 00332 * attributes. 00333 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION: If the client 00334 * has not enabled encryption required to read any of the attributes. 00335 * - ble::pal::AttErrorResponse::READ_NOT_PERMITTED: If any of the 00336 * attributes value cannot be read. 00337 * The first attribute causing the error is reporter in the handle_in_error 00338 * field in the error response. 00339 * Higher layer can also set an application error code (0x80 - 0x9F). 00340 * 00341 * @param connection_handle The handle of the connection to send this 00342 * request to. 00343 * @param attribute_handles Set of attribute handles to read. 00344 * 00345 * @return BLE_ERROR_NONE if the request has been successfully sent or an 00346 * appropriate error otherwise. 00347 * 00348 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.7 00349 */ 00350 virtual ble_error_t read_multiple_request( 00351 connection_handle_t connection_handle, 00352 const ArrayView<const attribute_handle_t>& attribute_handles 00353 ) = 0; 00354 00355 /** 00356 * Send a read by group type request to the server. It is used to get 00357 * informations about grouping attribute of a given type on a server. 00358 * 00359 * The server will reply with a ble::pal::ReadByGroupTypeResponse containing 00360 * informations about the grouping attribute found. Informations are: 00361 * - handle of the grouping attribute. 00362 * - last handle of the group . 00363 * - attribute value. 00364 * 00365 * If the last handle received is not the last handle of the discovery range 00366 * then it is necessary to send another request with a discovery range 00367 * updated to: [last handle + 1 : end]. 00368 * 00369 * In case of error, the server will send a ble::pal::AttErrorResponse. The 00370 * error code depends on the situation: 00371 * - ble::pal::AttErrorResponse::INVALID_HANDLE: If the range of handle 00372 * provided is invalid. 00373 * - ble::pal::AttErrorResponse::UNSUPPORTED_GROUP_TYPE: if the group type 00374 * is not a supported grouping attribute. 00375 * - ble::pal::AttErrorResponse::ATTRIBUTE_NOT_FOUND: If no attribute with 00376 * the given type exists within the range provided. 00377 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHENTICATION: If the client 00378 * security is not sufficient to read the requested attribute. 00379 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHORIZATION: If the client 00380 * authorization is not sufficient to read the requested attribute. 00381 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION_KEY_SIZE: If the 00382 * client has an insufficient encryption key size to read the requested 00383 * attributes. 00384 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION: If the client 00385 * has not enabled encryption required to read the requested attributes. 00386 * - ble::pal::AttErrorResponse::READ_NOT_PERMITTED: If any of the 00387 * attributes value cannot be read. 00388 * Higher layer can also set an application error code (0x80 - 0x9F). 00389 * 00390 * @param connection_handle The handle of the connection to send this 00391 * request to. 00392 * @param read_range Range where this request apply. 00393 * @param group_type Type of the grouping attribute to find and read. 00394 * 00395 * @return BLE_ERROR_NONE if the request has been successfully sent or an 00396 * appropriate error otherwise. 00397 * 00398 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.4.9 00399 */ 00400 virtual ble_error_t read_by_group_type_request( 00401 connection_handle_t connection_handle, 00402 attribute_handle_range_t read_range, 00403 const UUID& group_type 00404 ) = 0; 00405 00406 /** 00407 * Send a write request to the server to write the value of an attribute. 00408 * 00409 * In case of success, the server will reply with a 00410 * ble::pal::AttWriteResponse to acknowledge that the write operation went 00411 * well. 00412 * 00413 * If the attribute value has a variable length, then the attribute value 00414 * shall be truncated or lengthened to match the length of the value in the 00415 * request. 00416 * 00417 * If the attribute value has a fixed length and the Attribute Value parameter length 00418 * is less than or equal to the length of the attribute value, the octets of the 00419 * attribute value parameter length shall be written; all other octets in this attribute 00420 * value shall be unchanged. 00421 * 00422 * In case of error, the server will send a ble::pal::AttErrorResponse. The 00423 * error code depends on the situation: 00424 * - ble::pal::AttErrorResponse::INVALID_HANDLE: If the handle to write is 00425 * invalid. 00426 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHENTICATION: If the client 00427 * security is not sufficient to write the requested attribute. 00428 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHORIZATION: If the client 00429 * authorization is not sufficient to write the requested attribute. 00430 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION_KEY_SIZE: If the 00431 * client has an insufficient encryption key size to write the requested 00432 * attributes. 00433 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION: If the client 00434 * has not enabled encryption required to write the requested attributes. 00435 * - ble::pal::AttErrorResponse::WRITE_NOT_PERMITTED: If the attribute 00436 * value cannot be written due to permission. 00437 * - ble::pal::AttErrorResponse::INVALID_ATTRIBUTE_VALUE_LENGTH: If the 00438 * value to write exceeds the maximum valid length or of the attribute 00439 * value; whether the attribute has a variable length value or a fixed 00440 * length value. 00441 * Higher layer can also set an application error code (0x80 - 0x9F). 00442 * 00443 * @param connection_handle The handle of the connection to send this 00444 * request to. 00445 * @param attribute_handle Handle of the attribute to write. 00446 * @param value Value to write. It can't be longer than (mtu - 3). 00447 * 00448 * @return BLE_ERROR_NONE if the request has been successfully sent or an 00449 * appropriate error otherwise. 00450 * 00451 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.5.1 00452 */ 00453 virtual ble_error_t write_request( 00454 connection_handle_t connection_handle, 00455 attribute_handle_t attribute_handle, 00456 const ArrayView<const uint8_t> & value 00457 ) = 0; 00458 00459 /** 00460 * Send a write command to the server. A write command is similar to a write 00461 * request except that it won't receive any response from the server 00462 * 00463 * @param connection_handle The handle of the connection to send this 00464 * request to. 00465 * @param attribute_handle Handle of the attribute to write. 00466 * @param value Value to write. It can't be longer than (mtu - 3). 00467 * 00468 * @return BLE_ERROR_NONE if the request has been successfully sent or an 00469 * appropriate error otherwise. 00470 * 00471 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.5.3 00472 */ 00473 virtual ble_error_t write_command( 00474 connection_handle_t connection_handle, 00475 attribute_handle_t attribute_handle, 00476 const ArrayView<const uint8_t> & value 00477 ) = 0; 00478 00479 /** 00480 * Send a signed write command to the server. Behaviour is similar to a write 00481 * command except that 12 bytes of the mtu are reserved for the authentication 00482 * signature. 00483 * 00484 * @param connection_handle The handle of the connection to send this 00485 * request to. 00486 * @param attribute_handle Handle of the attribute to write. 00487 * @param value Value to write. It can't be longer than (mtu - 15). 00488 * 00489 * @note the authentication signature to send with this request is 00490 * computed by the implementation following the rules defined in BLUETOOTH 00491 * SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.3.1. 00492 * 00493 * @return BLE_ERROR_NONE if the request has been successfully sent or an 00494 * appropriate error otherwise. 00495 * 00496 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.5.4 00497 */ 00498 virtual ble_error_t signed_write_command( 00499 connection_handle_t connection_handle, 00500 attribute_handle_t attribute_handle, 00501 const ArrayView<const uint8_t> & value 00502 ) = 0; 00503 00504 /** 00505 * The Prepare Write Request is used to request the server to prepare to 00506 * write the value of an attribute. The client can send multiple prepare 00507 * write request which will be put in a queue until the client send an 00508 * Execute Write Request which will execute sequentially the write request 00509 * in the queue. 00510 * 00511 * In case of success the server will respond with a 00512 * ble::pal::AttPrepareWriteResponse containing the values (attribute handle, 00513 * offset and value) present in the write request. 00514 * 00515 * If a prepare write request is rejected by the server, the state queue of 00516 * the prepare write request queue remains unaltered. 00517 * 00518 * In case of error, the server will send a ble::pal::AttErrorResponse. The 00519 * error code depends on the situation: 00520 * - ble::pal::AttErrorResponse::INVALID_HANDLE: If the handle to write is 00521 * invalid. 00522 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHENTICATION: If the client 00523 * security is not sufficient to write the requested attribute. 00524 * - ble::pal::AttErrorResponse::INSUFFICIENT_AUTHORIZATION: If the client 00525 * authorization is not sufficient to write the requested attribute. 00526 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION_KEY_SIZE: If the 00527 * client has an insufficient encryption key size to write the requested 00528 * attributes. 00529 * - ble::pal::AttErrorResponse::INSUFFICIENT_ENCRYPTION: If the client 00530 * has not enabled encryption required to write the requested attributes. 00531 * - ble::pal::AttErrorResponse::WRITE_NOT_PERMITTED: If the attribute 00532 * value cannot be written due to permission. 00533 * - ble::pal::PREPARE_QUEUE_FULL: If the queue of prepare write request 00534 * is full. 00535 * Higher layer can also set an application error code (0x80 - 0x9F). 00536 * 00537 * @param connection_handle The handle of the connection to send this 00538 * request to. 00539 * @param attribute_handle The handle of the attribute to be written. 00540 * @param offset The offset of the first octet to be written. 00541 * @param value The value of the attribute to be written. It can't be longer 00542 * than (mtu - 5). 00543 * 00544 * @return BLE_ERROR_NONE if the request has been successfully sent or an 00545 * appropriate error otherwise. 00546 * 00547 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.6.1 00548 * 00549 */ 00550 virtual ble_error_t prepare_write_request( 00551 connection_handle_t connection_handle, 00552 attribute_handle_t attribute_handle, 00553 uint16_t offset, 00554 const ArrayView<const uint8_t> & value 00555 ) = 0; 00556 00557 /** 00558 * Send an Execute Write Request to the server. This request will instruct 00559 * the server to execute or cancel the prepare write requests currently held 00560 * in the prepare queue from this client. 00561 * 00562 * If the execute parameter is set to true, the server should execute the 00563 * request held in the queue. If the parameter is equal to false then the 00564 * server should cancel the requests in the queue. 00565 * 00566 * In case of success, the server will respond with a 00567 * ble::pal::AttExecuteWriteResponse indicating that the request was correctly 00568 * handled. 00569 * 00570 * In case of error, the server will send a ble::pal::AttErrorResponse. The 00571 * error code depends on the situation: 00572 * - ble::pal::AttErrorResponse::INVALID_OFFSET: If the value offset is 00573 * greater than the current length of the attribute to write. 00574 * - ble::pal::AttErrorResponse::INVALID_ATTRIBUTE_VALUE_LENGTH: If the 00575 * length of the value write exceeds the length of the attribute value 00576 * about to be written. 00577 * Higher layer can also set an application error code (0x80 - 0x9F). 00578 * 00579 * The error response will contains the attribute handle which as caused the 00580 * error and the remaining of the prepare queue is discarded. The state of 00581 * the attributes that were to be written from the prepare queue is not 00582 * defined in this case. 00583 * 00584 * @param connection_handle The handle of the connection to send this 00585 * request to. 00586 * @param execute Boolean indicating if the prepare queue should be executed 00587 * or cleared. 00588 * 00589 * @return BLE_ERROR_NONE if the request has been successfully sent or an 00590 * appropriate error otherwise. 00591 * 00592 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.4.6.3 00593 */ 00594 virtual ble_error_t execute_write_request( 00595 connection_handle_t connection_handle, 00596 bool execute 00597 ) = 0; 00598 00599 /** 00600 * Register a callback which will handle messages from the server. 00601 * 00602 * @param cb The callback object which will handle messages from the server. 00603 * It accept two parameters in input: The handle of the connection where the 00604 * message was received and the message received. Real type of the message 00605 * can be obtained from its opcode. 00606 */ 00607 void when_server_message_received( 00608 mbed::Callback<void(connection_handle_t, const AttServerMessage&)> cb 00609 ) { 00610 _server_message_cb = cb; 00611 } 00612 00613 /** 00614 * Register a callback handling transaction timeout. 00615 * 00616 * @param cb The callback handling timeout of a transaction. It accepts as 00617 * a parameter the connection handle involved in the timeout. 00618 * 00619 * @note No more attribute protocol requests, commands, indication or 00620 * notification shall be sent over a connection implied in a transaction 00621 * timeout. To send a new ATT message, the conenction should be 00622 * reestablished. 00623 */ 00624 void when_transaction_timeout( 00625 mbed::Callback<void(connection_handle_t)> cb 00626 ) { 00627 _transaction_timeout_cb = cb; 00628 } 00629 00630 protected: 00631 AttClient() { } 00632 00633 virtual ~AttClient() { } 00634 00635 /** 00636 * Upon server message reception an implementation shall call this function. 00637 * 00638 * @param connection_handle The handle of the connection which has received 00639 * the server message. 00640 * @param server_message The message received from the server. 00641 */ 00642 void on_server_event( 00643 connection_handle_t connection_handle, 00644 const AttServerMessage& server_message 00645 ) { 00646 if (_server_message_cb) { 00647 _server_message_cb(connection_handle, server_message); 00648 } 00649 } 00650 00651 /** 00652 * Upon transaction timeout an implementation shall call this function. 00653 * 00654 * @param connection_handle The handle of the connection of the transaction 00655 * which has times out. 00656 * 00657 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.3.3 00658 */ 00659 void on_transaction_timeout( 00660 connection_handle_t connection_handle 00661 ) { 00662 if (_transaction_timeout_cb) { 00663 _transaction_timeout_cb(connection_handle); 00664 } 00665 } 00666 00667 private: 00668 /** 00669 * Callback called when the client receive a message from the server. 00670 */ 00671 mbed::Callback<void(connection_handle_t, const AttServerMessage&)> _server_message_cb; 00672 00673 /** 00674 * Callback called when a transaction times out. 00675 */ 00676 mbed::Callback<void(connection_handle_t)> _transaction_timeout_cb; 00677 00678 // Disallow copy construction and copy assignment. 00679 AttClient(const AttClient&); 00680 AttClient& operator=(const AttClient&); 00681 }; 00682 00683 00684 } // namespace pal 00685 } // namespace ble 00686 00687 #endif /* BLE_PAL_ATTCLIENT_H_ */
Generated on Sun Jul 17 2022 08:25:20 by
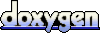