
text-to-speech through DAC to audio amp/speaker
Embed:
(wiki syntax)
Show/hide line numbers
TTS.h
00001 /** 00002 * Text To Speech synthesis library 00003 * Copyright (c) 2008 Clive Webster. All rights reserved. 00004 * 00005 * Nov. 29th 2009 - Modified to work with Arduino by Gabriel Petrut: 00006 * The Text To Speech library uses Timer1 to generate the PWM 00007 * output on digital pin 10. The output signal needs to be fed 00008 * to an RC filter then through an amplifier to the speaker. 00009 * http://www.tehnorama.ro/minieric-modulul-de-control-si-sinteza-vocala/ 00010 * 00011 * Modified to allow use of different PWM pins by Stephen Crane. 00012 */ 00013 00014 #ifndef TTS_h 00015 #define TTS_h 00016 00017 #include "mbed.h" 00018 #include "english.h" 00019 00020 #define byte uint8_t 00021 00022 // DAC pins assume 8-bit DAC 00023 #ifdef TARGET_K64F 00024 #define DACpin DAC0_OUT 00025 #endif 00026 00027 #ifdef TARGET_NUCLEO_F446RE 00028 #define DACpin A2 00029 #endif 00030 00031 #ifdef TARGET_DISCO_F469NI 00032 #define DACpin A5 00033 00034 #endif 00035 00036 #ifdef TARGET_ARCH_MAX 00037 #define DACpin PA_4 00038 #define DACmax 4096 00039 #endif 00040 00041 #ifdef TARGET_LPC1768 00042 #define DACpin p18 00043 #endif 00044 00045 #ifdef TARGET_DISCO_L476VG 00046 #define DACpin PA_5 00047 #endif 00048 00049 00050 00051 class TTS { 00052 public: 00053 /** 00054 * constructs a new text-to-speech 00055 * pin is the PWM pin on which audio is output 00056 * (valid values: 9, 10, 3) 00057 */ 00058 TTS(void); 00059 00060 /** 00061 * speaks a string of (english) text 00062 */ 00063 void sayText(const char *text); 00064 00065 /** 00066 * speaks a string of phonemes 00067 */ 00068 void sayPhonemes(const char *phonemes); 00069 00070 /** 00071 * sets the pitch; higher values: lower pitch 00072 */ 00073 void setPitch(byte pitch); 00074 00075 /** 00076 * gets the pitch 00077 */ 00078 byte getPitch(void); 00079 }; 00080 00081 #endif
Generated on Wed Jul 13 2022 01:54:20 by
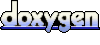