
An fully working IMU-Filter and Sensor drivers for the 10DOF-Board over I2C. All in one simple class. Include, calibrate sensors, call read, get angles. (3D Visualisation code for Python also included) Sensors: L3G4200D, ADXL345, HMC5883, BMP085
I2C_Sensor.h
00001 // by MaEtUgR 00002 00003 #ifndef I2C_Sensor_H 00004 #define I2C_Sensor_H 00005 00006 #include "mbed.h" 00007 00008 class I2C_Sensor 00009 { 00010 public: 00011 I2C_Sensor(PinName sda, PinName scl, char address); 00012 00013 float data[3]; // where the measured data is saved 00014 virtual void read() = 0; // read all axis from register to array data 00015 //TODO: virtual void calibrate() = 0; // calibrate the sensor and if desired write calibration values to a file 00016 00017 protected: 00018 // Calibration 00019 void saveCalibrationValues(float values[], int size, char * filename); 00020 void loadCalibrationValues(float values[], int size, char * filename); 00021 00022 // I2C functions 00023 char readRegister(char reg); 00024 void writeRegister(char reg, char data); 00025 void readMultiRegister(char reg, char* output, int size); 00026 00027 // raw data and function to measure it 00028 short raw[3]; 00029 virtual void readraw() = 0; 00030 00031 private: 00032 I2C i2c; // original mbed I2C-library just to initialise the control registers 00033 char i2c_address; // address 00034 00035 LocalFileSystem local; // file access to save calibration values 00036 }; 00037 00038 #endif
Generated on Wed Jul 13 2022 22:03:48 by
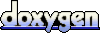