
An fully working IMU-Filter and Sensor drivers for the 10DOF-Board over I2C. All in one simple class. Include, calibrate sensors, call read, get angles. (3D Visualisation code for Python also included) Sensors: L3G4200D, ADXL345, HMC5883, BMP085
ADXL345.cpp
00001 #include "ADXL345.h" 00002 00003 ADXL345::ADXL345(PinName sda, PinName scl) : I2C_Sensor(sda, scl, ADXL345_I2C_ADDRESS) 00004 { 00005 #warning these three offsets are calibration values to make shure the measurement is 0 when no acceleration is present 00006 offset[0] = -9.1; // offset calculated by hand... (min + ((max - min) / 2) 00007 offset[1] = -7.1; // TODO: make this automatic with saving to filesystem 00008 offset[2] = 6; 00009 00010 // Set Offset - programmed into the OFSX, OFSY, and OFXZ registers, respectively, as 0xFD, 0x03 and 0xFE. 00011 writeRegister(ADXL345_OFSX_REG, 0xFA); // to get these offsets just lie your sensor down on the table always the axis pointing down to earth has 200+ and the others should have more or less 0 00012 writeRegister(ADXL345_OFSY_REG, 0xFE); 00013 writeRegister(ADXL345_OFSZ_REG, 0x0A); 00014 00015 writeRegister(ADXL345_BW_RATE_REG, 0x0F); // 3200Hz BW-Rate 00016 writeRegister(ADXL345_DATA_FORMAT_REG, 0x0B); // set data format to full resolution and +-16g 00017 writeRegister(ADXL345_POWER_CTL_REG, 0x08); // set mode 00018 } 00019 00020 void ADXL345::read(){ 00021 readraw(); 00022 for (int i = 0; i < 3; i++) 00023 data[i] = raw[i] - offset[i]; // TODO: didnt care about units 00024 } 00025 00026 void ADXL345::readraw(){ 00027 char buffer[6]; 00028 readMultiRegister(ADXL345_DATAX0_REG, buffer, 6); 00029 00030 raw[0] = (short) ((int)buffer[1] << 8 | (int)buffer[0]); 00031 raw[1] = (short) ((int)buffer[3] << 8 | (int)buffer[2]); 00032 raw[2] = (short) ((int)buffer[5] << 8 | (int)buffer[4]); 00033 } 00034 00035 void ADXL345::calibrate(int times, float separation_time) 00036 { 00037 // calibrate sensor with an average of count samples (result of calibration stored in offset[]) 00038 float calib[3] = {0,0,0}; // temporary array for the sum of calibration measurement 00039 00040 for (int j = 0; j < times; j++) { // read 'times' times the data in a very short time 00041 readraw(); 00042 for (int i = 0; i < 3; i++) 00043 calib[i] += raw[i]; 00044 wait(separation_time); 00045 } 00046 00047 for (int i = 0; i < 3; i++) 00048 offset[i] = calib[i]/times; // take the average of the calibration measurements 00049 }
Generated on Wed Jul 13 2022 22:03:48 by
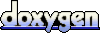