Kalman filter for Eurobot
Matrix Class Reference
This class provide basic manipulation for 2D matrices see Log.c for more info version 1.6.4. More...
#include <Matrix.h>
Public Member Functions | |
Matrix (int Rows, int Cols) | |
Creates a nex Matrix of Size [ Row x Cols ]. | |
Matrix (const Matrix &base) | |
Creates a new Matrix identical to an input Matrix. | |
Matrix () | |
Default Constructor. | |
float & | operator() (int row, int col) |
Subindex for Matrix elements assignation. | |
float | operator() (int row, int col) const |
Subindex for Matrix element. | |
Matrix & | operator= (const Matrix &rightM) |
Overwrites all data. | |
const Matrix | operator- () |
All elements in matrix are multiplied by (-1). | |
bool | isZero () const |
Returns TRUE if the matrix is zero, FALSE otherwhise. | |
bool | isVector () const |
Determines weather a Matrix is a Single Column or Row. | |
void | Resize (int Rows, int Cols) |
This function resizes the Matrix to fit new data or cropped it, operator << can overwrite entire Matrix. | |
virtual void | FillMatrix () |
Asks user for numbers to fill the Matrix elements, one by one. | |
virtual void | print () const |
Prints the entire Matrix using standard PRINTF. | |
void | Clear () |
Makes all values on Matrix object zero. | |
void | add (int Row, int Col, float number) |
Assigns a float number to the matrix in a specified position Index starts at [1][1]. | |
float | sum () const |
Returns the sum of every cell in the Matrix. | |
float | getNumber (int Row, int Col) const |
Return the number in position [Row],[Col]. | |
int | getCols () const |
Retuns the number of Columns in Matrix, index starts at 1. | |
int | getRows () const |
Retruns the number of Rows in Matrix, index starts at 1. | |
Static Public Member Functions | |
static const Matrix | ToPackedVector (const Matrix &Mat) |
Shatters the matrix into a single Row Vector. | |
static void | AddRow (Matrix &Mat, int index) |
Invoking this static method will increase a Row in Mat in the desired position. | |
static void | AddRow (Matrix &Receip, const Matrix &Row, int index) |
Adds to Receip a new Row from another Matrix in desired index. | |
static void | AddCol (Matrix &Mat, int index) |
Invoking this static method will increase a Column in Matrix in the desired Position. | |
static void | AddCol (Matrix &Receip, const Matrix &Col, int index) |
This will copy a Column Matrix into Receip in desired Position, Must be same size. | |
static void | DeleteRow (Matrix &Mat, int Row) |
Static Function Deletes Row from Matrix, Static to prevent missuse. | |
static void | DeleteCol (Matrix &Mat, int Col) |
Static Function Deletes Column from Matrix, it's Static to prevent missuse. | |
static const Matrix | ExportRow (const Matrix &Mat, int row) |
This method extracts a Row from a Matrix and Saves it in Mat. | |
static const Matrix | ExportCol (const Matrix &Mat, int col) |
This method extracts a Column from a Matrix and returns the Column as a new Matrix. | |
Friends | |
class | MatrixMath |
This includes the Class to handle Matrix Operations. | |
bool | operator== (const Matrix &leftM, const Matrix &rightM) |
Overload opeartor for the compare Matrices. | |
bool | operator!= (const Matrix &leftM, const Matrix &rightM) |
Overload opeartor for the compare Matrices. | |
Matrix & | operator+= (Matrix &leftM, const Matrix &rightM) |
Overload Copmpound assignment. | |
Matrix & | operator-= (Matrix &leftM, const Matrix &rightM) |
Overload Compund decrease. | |
Matrix & | operator*= (Matrix &leftM, const Matrix &rightM) |
Overload Compound CrossProduct Matrix operation. | |
Matrix & | operator*= (Matrix &leftM, float number) |
Overload Compund Element-by-elemnt scalar multiplication. | |
const Matrix | operator+= (Matrix &leftM, float number) |
Overload Compound add with scalar. | |
const Matrix | operator-= (Matrix &leftM, float number) |
Compound substract with scalar. | |
const Matrix | operator+ (const Matrix &leftM, const Matrix &rightM) |
Adds two matrices of the same dimensions, element-by-element. | |
const Matrix | operator+ (const Matrix &leftM, float number) |
Adds the given nomber to each element of matrix. | |
const Matrix | operator+ (float number, const Matrix &leftM) |
Adds the given number to each element in Matrix. | |
const Matrix | operator- (const Matrix &leftM, const Matrix &rightM) |
Substracts two matrices of the same size, element-by-element. | |
const Matrix | operator- (const Matrix &leftM, float number) |
Substracts each element in Matrix by number. | |
const Matrix | operator- (float number, const Matrix &leftM) |
Substracts each element in Matrix by number. | |
const Matrix | operator* (const Matrix &leftM, const Matrix &rightM) |
Preforms Crossproduct between two matrices. | |
const Matrix | operator* (const Matrix &leftM, float number) |
Multiplies a scalar number with each element on Matrix. | |
const Matrix | operator* (float number, const Matrix &leftM) |
Multiplies a scalar number with each element on Matrix. | |
Matrix & | operator<< (Matrix &leftM, float number) |
Inputs numbres into a Matrix, the matrix needs to be costructed as Matrix( _nRows, _nCols ). |
Detailed Description
This class provide basic manipulation for 2D matrices see Log.c for more info version 1.6.4.
Definition at line 26 of file Matrix.h.
Constructor & Destructor Documentation
Matrix | ( | int | Rows, |
int | Cols | ||
) |
Creates a nex Matrix of Size [ Row x Cols ].
Rows by Cols Matrix Constructor.
Definition at line 17 of file Matrix.cpp.
Creates a new Matrix identical to an input Matrix.
Copies one matrix into a new one.
Definition at line 31 of file Matrix.cpp.
Matrix | ( | ) |
Default Constructor.
Definition at line 50 of file Matrix.cpp.
Member Function Documentation
void add | ( | int | Row, |
int | Col, | ||
float | number | ||
) |
Assigns a float number to the matrix in a specified position Index starts at [1][1].
Inserts a Single element in a desired Position( Index starts at [1][1] );.
Definition at line 352 of file Matrix.cpp.
void AddCol | ( | Matrix & | Mat, |
int | index | ||
) | [static] |
Invoking this static method will increase a Column in Matrix in the desired Position.
To add (Insert) a single Column to a Matrix.
- Parameters:
-
Mat,: Matrix in wich to insert a Column Col,: Number of column, strats with one, not zero.
Definition at line 146 of file Matrix.cpp.
void AddRow | ( | Matrix & | Mat, |
int | index | ||
) | [static] |
Invoking this static method will increase a Row in Mat in the desired position.
To add (Insert) a Single Row to a Matrix.
The current Row will be moved down to allocate space, and all elements will be initialized to zero in the new row.
- Parameters:
-
Mat,: Matrix in wich to insert a Row Row,: Number of row to insert, starts with one, not zero.
Definition at line 109 of file Matrix.cpp.
Adds to Receip a new Row from another Matrix in desired index.
Must be same size. The Row matrix must be SingleRow Matrix, you can use ExportRow to extract a Row from another Matrix.
- Parameters:
-
Receip Matrix to be Modified. Row Row to be added. index position in wich to be added, _nRow + 1 last position.
Definition at line 134 of file Matrix.cpp.
void Clear | ( | ) |
Makes all values on Matrix object zero.
Fills matrix with zeros.
Also make posible use the '<<' operator to add elements and keep track of last element added.
Definition at line 338 of file Matrix.cpp.
void DeleteCol | ( | Matrix & | Mat, |
int | Col | ||
) | [static] |
Static Function Deletes Column from Matrix, it's Static to prevent missuse.
Delete a Single Column From Matrix.
Print error and does nothing if out of limits.
- Parameters:
-
Col,: Number of Col to delete (first Col = 1) Mat,: Matrix to delete from.
Definition at line 183 of file Matrix.cpp.
void DeleteRow | ( | Matrix & | Mat, |
int | Row | ||
) | [static] |
Static Function Deletes Row from Matrix, Static to prevent missuse.
Delete a Single Row form Matrix.
- Parameters:
-
Mat,: Matrix to delete Row from Row,: Number of Row (first Row = 1)
Definition at line 214 of file Matrix.cpp.
This method extracts a Column from a Matrix and returns the Column as a new Matrix.
Extracts a single column form calling matrix and saves it to another matrix.
If Row is out of the parameters, it does nothing and prints a warning.
- Parameters:
-
Col,: number of Column to extract elements. this->_nCols. Mat,: Matrix to extract from.
- Returns:
- New Row Matrix.
Definition at line 262 of file Matrix.cpp.
This method extracts a Row from a Matrix and Saves it in Mat.
Extracts a single row form calling matrix and saves it to another matrix.
If Row is out of the parameters it does nothing, but prints a warning.
- Parameters:
-
Row,: number of row to extract elements. this->_nRows. Mat,: Matrix to extract from.
- Returns:
- New Row Matrix.
Definition at line 236 of file Matrix.cpp.
void FillMatrix | ( | ) | [virtual] |
Asks user for numbers to fill the Matrix elements, one by one.
Ask user for elemnts in Matrix.
It uses printf(); by default the USBTX, USBRX, 9600, 1N8.
Definition at line 301 of file Matrix.cpp.
int getCols | ( | ) | const |
Retuns the number of Columns in Matrix, index starts at 1.
Returns the number of Columns in Matrix.
Definition at line 387 of file Matrix.cpp.
float getNumber | ( | int | Row, |
int | Col | ||
) | const |
Return the number in position [Row],[Col].
Returns the specified element. Index Starts at [1][1].
- Parameters:
-
Row = number of row in matrix Col = number of Col in matrix
- Returns:
- Num = float number in matrix
Definition at line 379 of file Matrix.cpp.
int getRows | ( | ) | const |
Retruns the number of Rows in Matrix, index starts at 1.
Returns the number of Rows in Matrix.
Definition at line 383 of file Matrix.cpp.
bool isVector | ( | ) | const |
Determines weather a Matrix is a Single Column or Row.
Returns true if Matrix is Single Row ot Single Column.
Definition at line 75 of file Matrix.cpp.
bool isZero | ( | ) | const |
Returns TRUE if the matrix is zero, FALSE otherwhise.
Returns true if matrix is full of zeros.
- Parameters:
-
mat,: Matrix to be tested
Definition at line 63 of file Matrix.cpp.
float & operator() | ( | int | row, |
int | col | ||
) |
Subindex for Matrix elements assignation.
Subindex in Matrix left side.
- Parameters:
-
row col
- Returns:
- pointer to the element.
Definition at line 16 of file Operators.cpp.
float operator() | ( | int | row, |
int | col | ||
) | const |
Subindex for Matrix element.
Subindex in Matrix right side.
- Parameters:
-
row col
- Returns:
- the element.
Definition at line 29 of file Operators.cpp.
const Matrix operator- | ( | ) |
All elements in matrix are multiplied by (-1).
- Returns:
- A new Matrix object with inverted values.
Definition at line 64 of file Operators.cpp.
Overwrites all data.
Overloaded Asign Operator. Resizes Matrix.
To be used Carefully!
Definition at line 43 of file Operators.cpp.
void print | ( | ) | const [virtual] |
Prints the entire Matrix using standard PRINTF.
Prints out Matrix.
Definition at line 323 of file Matrix.cpp.
void Resize | ( | int | Rows, |
int | Cols | ||
) |
This function resizes the Matrix to fit new data or cropped it, operator << can overwrite entire Matrix.
Makes matrix Bigger!
- Parameters:
-
Rows,: New Number of Rows Cols,: New numbler of columns
Definition at line 285 of file Matrix.cpp.
float sum | ( | ) | const |
Returns the sum of every cell in the Matrix.
Adds all elements in matrix and returns the answer.
Definition at line 367 of file Matrix.cpp.
Shatters the matrix into a single Row Vector.
Returns all elements in Matrix as a single Row vector.
Important: Returns NEW matrix, does no modify existing one.
Definition at line 86 of file Matrix.cpp.
Friends And Related Function Documentation
friend class MatrixMath [friend] |
Overload opeartor for the compare Matrices.
- Parameters:
-
rightM
- Returns:
- Boolean 'true' if different
Definition at line 95 of file Operators.cpp.
Multiplies a scalar number with each element on Matrix.
- Returns:
- A new object with the result.
Definition at line 284 of file Operators.cpp.
Multiplies a scalar number with each element on Matrix.
- Returns:
Definition at line 295 of file Operators.cpp.
Overload Compound CrossProduct Matrix operation.
- Parameters:
-
rightM
- Returns:
Definition at line 135 of file Operators.cpp.
Overload Compund Element-by-elemnt scalar multiplication.
- Parameters:
-
number
- Returns:
Definition at line 153 of file Operators.cpp.
Adds two matrices of the same dimensions, element-by-element.
If diferent dimensions -> ERROR.
- Returns:
- A new object Matrix with the result.
Definition at line 186 of file Operators.cpp.
Adds the given nomber to each element of matrix.
Mimic MATLAB operation.
- Returns:
- A new matrix object with the result.
Definition at line 207 of file Operators.cpp.
Adds the given number to each element in Matrix.
- Returns:
- A new Matrix object with the result.
Definition at line 219 of file Operators.cpp.
Overload Compound add with scalar.
Because the '=' operator checks for self Assign, no extra operations are needed.
- Returns:
- Same Matrix to self Assign.
Definition at line 168 of file Operators.cpp.
Overload Copmpound assignment.
- Parameters:
-
rightM
- Returns:
- A new Matrix to be assigned to itself.
Element by element adition.
Definition at line 103 of file Operators.cpp.
Substracts two matrices of the same size, element-by-element.
If different dimensions -> ERROR.
- Returns:
- A new object Matrix with the result.
Definition at line 225 of file Operators.cpp.
Substracts each element in Matrix by number.
- Returns:
- A new matrix object with the result.
Definition at line 256 of file Operators.cpp.
Substracts each element in Matrix by number.
- Returns:
- A new matrix object with the result.
Definition at line 244 of file Operators.cpp.
Overload Compund decrease.
- Parameters:
-
rightM Right hand matrix
- Returns:
- A new Matrix to be assigned to itself
Element by element Substraction
Definition at line 119 of file Operators.cpp.
Compound substract with scalar.
- Returns:
- Same matrix to self Assign.
Definition at line 177 of file Operators.cpp.
Inputs numbres into a Matrix, the matrix needs to be costructed as Matrix( _nRows, _nCols ).
This does NOT work on an only declared Matrix such as: Matrix obj; obj << 5; //Error
- Returns:
Definition at line 301 of file Operators.cpp.
Overload opeartor for the compare Matrices.
- Parameters:
-
rightM
- Returns:
- Boolean 'false' if different.
Definition at line 77 of file Operators.cpp.
Generated on Wed Jul 13 2022 22:04:07 by
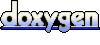