Kalman filter for Eurobot
Embed:
(wiki syntax)
Show/hide line numbers
Matrix.h
Go to the documentation of this file.
00001 /** 00002 * @brief API for Matrix Library 00003 * @file Matrix.h 00004 * @author Ernesto Palacios 00005 * 00006 * Created on 13 de septiembre de 2011, 03:49 PM 00007 * 00008 * Develop Under GPL v3.0 License 00009 * http://www.gnu.org/licenses/gpl-3.0.html 00010 * 00011 */ 00012 00013 #ifndef MATRIX_H 00014 #define MATRIX_H 00015 00016 00017 #include <vector> 00018 00019 class MatrixMath; 00020 00021 /** 00022 * @brief This class provide basic manipulation for 2D matrices see Log.c for more info 00023 * version 1.6.4. 00024 * 00025 */ 00026 class Matrix{ 00027 public: 00028 00029 /// Creates a nex Matrix of Size [ Row x Cols ] 00030 Matrix( int Rows, int Cols ); 00031 00032 00033 /// Creates a new Matrix identical to an input Matrix 00034 Matrix( const Matrix& base ); 00035 00036 00037 /// Default Constructor 00038 Matrix(); 00039 00040 00041 /******************************************************************************/ 00042 00043 00044 /**@brief This includes the Class to handle Matrix Operations. 00045 */ 00046 friend class MatrixMath; 00047 00048 00049 /**@brief 00050 * Subindex for Matrix elements assignation. 00051 * @param row 00052 * @param col 00053 * @return pointer to the element. 00054 */ 00055 float& operator() ( int row, int col ); 00056 00057 00058 /**@brief 00059 *Subindex for Matrix element. 00060 * @param row 00061 * @param col 00062 * @return the element. 00063 */ 00064 float operator() ( int row, int col ) const; 00065 00066 00067 00068 /** @brief 00069 * Overwrites all data. To be used Carefully! 00070 */ 00071 Matrix& operator = ( const Matrix& rightM ); 00072 00073 00074 /** @brief 00075 * Overload opeartor for the compare Matrices 00076 * 00077 * @param rightM 00078 * @return Boolean 'false' if different. 00079 */ 00080 friend bool operator == ( const Matrix& leftM, const Matrix& rightM ); 00081 00082 00083 /** @brief 00084 * Overload opeartor for the compare Matrices 00085 * 00086 * @param rightM 00087 * @return Boolean 'true' if different 00088 */ 00089 friend bool operator != ( const Matrix& leftM, const Matrix& rightM ); 00090 00091 00092 /** @brief 00093 * Overload Copmpound assignment. 00094 * @param rightM 00095 * @return A new Matrix to be assigned to itself. 00096 */ 00097 friend Matrix& operator += ( Matrix& leftM, const Matrix& rightM ); 00098 00099 00100 /** @brief 00101 * Overload Compund decrease. 00102 * @param rightM Right hand matrix 00103 * @return A new Matrix to be assigned to itself 00104 */ 00105 friend Matrix& operator -= ( Matrix& leftM, const Matrix& rightM ); 00106 00107 00108 /** @brief 00109 * Overload Compound CrossProduct Matrix operation. 00110 * @param rightM 00111 * @return 00112 */ 00113 friend Matrix& operator *=( Matrix& leftM, const Matrix& rightM ); 00114 00115 00116 /** @brief 00117 * Overload Compund Element-by-elemnt scalar multiplication. 00118 * @param number 00119 * @return 00120 */ 00121 friend Matrix& operator *=( Matrix& leftM, float number ); 00122 00123 00124 00125 /**@brief 00126 * All elements in matrix are multiplied by (-1). 00127 * @return A new Matrix object with inverted values. 00128 */ 00129 const Matrix operator -(); 00130 00131 00132 /**@brief 00133 * Overload Compound add with scalar. 00134 * Because the '=' operator checks for self Assign, no extra operations 00135 * are needed. 00136 * @return Same Matrix to self Assign. 00137 */ 00138 friend const Matrix operator +=( Matrix& leftM, float number ); 00139 00140 00141 /**@brief 00142 * Compound substract with scalar. 00143 * @return Same matrix to self Assign. 00144 */ 00145 friend const Matrix operator -=( Matrix& leftM, float number ); 00146 00147 00148 /** @brief 00149 * Adds two matrices of the same dimensions, element-by-element. 00150 * If diferent dimensions -> ERROR. 00151 * @return A new object Matrix with the result. 00152 */ 00153 friend const Matrix operator +( const Matrix& leftM, const Matrix& rightM); 00154 00155 00156 /** @brief 00157 * Adds the given nomber to each element of matrix. 00158 * Mimic MATLAB operation. 00159 * @return A new matrix object with the result. 00160 */ 00161 friend const Matrix operator +( const Matrix& leftM, float number ); 00162 00163 00164 00165 /**@brief 00166 * Adds the given number to each element in Matrix. 00167 * @return A new Matrix object with the result. 00168 */ 00169 friend const Matrix operator +( float number, const Matrix& leftM ); 00170 00171 00172 /**@brief 00173 * Substracts two matrices of the same size, element-by-element. 00174 * If different dimensions -> ERROR. 00175 * @return A new object Matrix with the result. 00176 */ 00177 friend const Matrix operator -( const Matrix& leftM, const Matrix& rightM ); 00178 00179 00180 /**@brief 00181 * Substracts each element in Matrix by number. 00182 * @return A new matrix object with the result. 00183 */ 00184 friend const Matrix operator -( const Matrix& leftM, float number ); 00185 00186 00187 /**@brief 00188 * Substracts each element in Matrix by number 00189 * @return A new matrix object with the result. 00190 */ 00191 friend const Matrix operator -( float number, const Matrix& leftM ); 00192 00193 00194 /** 00195 * Preforms Crossproduct between two matrices. 00196 * @return 00197 */ 00198 friend const Matrix operator *( const Matrix& leftM, const Matrix& rightM ); 00199 00200 00201 /**@brief 00202 * Multiplies a scalar number with each element on Matrix. 00203 * @return A new object with the result. 00204 */ 00205 friend const Matrix operator *( const Matrix& leftM, float number ); 00206 00207 00208 /**@brief 00209 * Multiplies a scalar number with each element on Matrix. 00210 * @return 00211 */ 00212 friend const Matrix operator *( float number, const Matrix& leftM ); 00213 00214 00215 /**@brief 00216 * Inputs numbres into a Matrix, the matrix needs to be costructed as 00217 * Matrix( _nRows, _nCols ). 00218 * This does NOT work on an only declared Matrix such as: 00219 * Matrix obj; 00220 * obj << 5; //Error 00221 * @return 00222 */ 00223 friend Matrix& operator <<( Matrix& leftM, float number ); 00224 00225 /***********************************************************************/ 00226 00227 /** @brief 00228 * Returns TRUE if the matrix is zero, FALSE otherwhise 00229 * @param mat: Matrix to be tested 00230 */ 00231 bool isZero() const; 00232 00233 00234 /** @brief 00235 * Determines weather a Matrix is a Single Column or Row. 00236 */ 00237 bool isVector() const; 00238 00239 00240 /** @brief 00241 * Shatters the matrix into a single Row Vector. 00242 * Important: Returns NEW matrix, does no modify existing one. 00243 */ 00244 static const Matrix ToPackedVector( const Matrix& Mat ); 00245 00246 00247 /** @brief 00248 * Invoking this static method will increase a Row in Mat in the desired 00249 * position. 00250 * The current Row will be moved down to allocate space, and all elements will 00251 * be initialized to zero in the new row. 00252 * @param Mat: Matrix in wich to insert a Row 00253 * @param Row: Number of row to insert, starts with one, not zero. 00254 */ 00255 static void AddRow( Matrix& Mat, int index ); 00256 00257 00258 /**@brief 00259 * Adds to Receip a new Row from another Matrix in desired index. 00260 * Must be same size. 00261 * The Row matrix must be SingleRow Matrix, you can use ExportRow 00262 * to extract a Row from another Matrix. 00263 * @param Receip Matrix to be Modified. 00264 * @param Row Row to be added. 00265 * @param index position in wich to be added, _nRow + 1 last position. 00266 */ 00267 static void AddRow( Matrix& Receip, const Matrix& Row, int index ); 00268 00269 00270 /** @brief 00271 * Invoking this static method will increase a Column in Matrix in the 00272 * desired Position. 00273 * @param Mat: Matrix in wich to insert a Column 00274 * @param Col: Number of column, strats with one, not zero. 00275 */ 00276 static void AddCol( Matrix& Mat, int index ); 00277 00278 00279 /**@brief 00280 * This will copy a Column Matrix into Receip in desired Position, 00281 * Must be same size. 00282 * The Col Matrix must be a SingleCol Matrix, you can use ExportCol 00283 * to extract a Column from another Matrix. 00284 * @param Receip Matrix to be modified. 00285 * @param Column Data to be copied. 00286 * @param index Postion in Receip Matrix . 00287 */ 00288 static void AddCol( Matrix& Receip, const Matrix& Col, int index ); 00289 00290 00291 /** @brief 00292 * Static Function Deletes Row from Matrix, Static to prevent missuse 00293 * @param Mat: Matrix to delete Row from 00294 * @param Row: Number of Row (first Row = 1) 00295 */ 00296 static void DeleteRow( Matrix& Mat, int Row ); 00297 00298 00299 /** @brief 00300 * Static Function Deletes Column from Matrix, it's Static to prevent 00301 * missuse. 00302 * Print error and does nothing if out of limits. 00303 * @param Col: Number of Col to delete (first Col = 1) 00304 * @param Mat: Matrix to delete from. 00305 */ 00306 static void DeleteCol( Matrix& Mat, int Col ); 00307 00308 00309 /** @brief 00310 * This method extracts a Row from a Matrix and Saves it in Mat. 00311 * If Row is out of the parameters it does nothing, but prints a warning. 00312 * @param Row: number of row to extract elements. this->_nRows. 00313 * @param Mat: Matrix to extract from. 00314 * @return New Row Matrix. 00315 */ 00316 static const Matrix ExportRow( const Matrix& Mat, int row ); 00317 00318 00319 /** @brief 00320 * This method extracts a Column from a Matrix and returns the Column 00321 * as a new Matrix. 00322 * If Row is out of the parameters, it does nothing and prints a warning. 00323 * @param Col: number of Column to extract elements. this->_nCols. 00324 * @param Mat: Matrix to extract from. 00325 * @return New Row Matrix. 00326 */ 00327 static const Matrix ExportCol( const Matrix& Mat, int col ); 00328 00329 00330 /** @brief 00331 * This function resizes the Matrix to fit new data or cropped it, 00332 * operator << can overwrite entire Matrix. 00333 * 00334 * @param Rows: New Number of Rows 00335 * @param Cols: New numbler of columns 00336 */ 00337 void Resize( int Rows, int Cols ); 00338 00339 00340 /** @brief 00341 * Asks user for numbers to fill the Matrix elements, one by one. 00342 * It uses printf(); by default the USBTX, USBRX, 9600, 1N8. 00343 */ 00344 virtual void FillMatrix(); 00345 00346 00347 /** @brief 00348 * Prints the entire Matrix using standard PRINTF 00349 */ 00350 virtual void print() const; 00351 00352 00353 /** @brief 00354 * Makes all values on Matrix object zero. 00355 * Also make posible use the '<<' operator to add elements and keep 00356 * track of last element added. 00357 */ 00358 void Clear(); 00359 00360 00361 /** @brief 00362 * Assigns a float number to the matrix in a specified position 00363 * Index starts at [1][1]. 00364 * 00365 * @param number: Number to be set 00366 * @param Row: Row of Matrix 00367 * @param Col: Column of Matrix 00368 */ 00369 void add( int Row, int Col, float number ); 00370 00371 00372 /** @brief 00373 * Returns the sum of every cell in the Matrix. 00374 */ 00375 float sum() const; 00376 00377 00378 /** @brief 00379 * Return the number in position [Row],[Col] 00380 * @param Row = number of row in matrix 00381 * @param Col = number of Col in matrix 00382 * @return Num = float number in matrix 00383 */ 00384 float getNumber( int Row, int Col ) const; 00385 00386 00387 /**@brief 00388 * Retuns the number of Columns in Matrix, index starts at 1. 00389 */ 00390 int getCols() const; 00391 00392 00393 /**@brief 00394 *Retruns the number of Rows in Matrix, index starts at 1. 00395 */ 00396 int getRows() const; 00397 00398 00399 private: 00400 00401 /** 2-D Vector Array*/ 00402 std::vector < std::vector<float> > _matrix; 00403 00404 00405 00406 /** Number of Rows in Matrix*/ 00407 int _nRows; 00408 00409 /**Number of Columns in Matrix*/ 00410 int _nCols; 00411 00412 00413 00414 /**Last Element Row position in Matrix*/ 00415 int _pRow; 00416 00417 /**Last Element Col position in Matrix*/ 00418 int _pCol; 00419 00420 }; 00421 00422 #endif /* MATRIX_H */ 00423
Generated on Wed Jul 13 2022 22:04:07 by
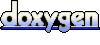