ms2s,STM32 Private
Fork of WakeUp by
WakeUp Class Reference
Class to make wake up a microcontroller from deepsleep using a low-power timer. More...
#include <WakeUp.h>
Static Public Member Functions | |
static void | set (uint32_t s) |
Set the timeout. | |
static void | set_ms (uint32_t ms) |
Set the timeout. | |
static void | attach (void(*function)(void)) |
Attach a function to be called after timeout. | |
static void | calibrate (void) |
Calibrate the timer. |
Detailed Description
Class to make wake up a microcontroller from deepsleep using a low-power timer.
// Depending on the LED connections either the LED is off the 2 seconds // the target spends in deepsleep(), and on for the other second. Or it is inverted #include "mbed.h" #include "WakeUp.h" DigitalOut myled(LED1); int main() { wait(5); //The low-power oscillator can be quite inaccurate on some targets //this function calibrates it against the main clock WakeUp::calibrate(); while(1) { //Set LED to zero myled = 0; //Set wakeup time for 2 seconds WakeUp::set_ms(2000); //Enter deepsleep, the program won't go beyond this point until it is woken up deepsleep(); //Set LED for 1 second to one myled = 1; wait(1); } }
Definition at line 39 of file WakeUp.h.
Member Function Documentation
static void attach | ( | void(*)(void) | function ) | [static] |
Attach a function to be called after timeout.
This is optional, if you just want to wake up you do not need to attach a function.
Important: Many targets will run the wake-up routine at reduced clock speed, afterwards clock speed is restored. This means that clock speed dependent functions, such as printf might end up distorted.
Also supports normal way to attach member functions (not documented for simplicity)
- Parameters:
-
*function function to call
void calibrate | ( | void | ) | [static] |
Calibrate the timer.
Some of the low-power timers have very bad accuracy. This function calibrates it against the main timer.
Warning: Blocks for 100ms!
Definition at line 120 of file WakeUp_STM_RTC.cpp.
static void set | ( | uint32_t | s ) | [static] |
void set_ms | ( | uint32_t | ms ) | [static] |
Set the timeout.
- Parameters:
-
ms required time in milliseconds
Definition at line 31 of file WakeUp_STM_RTC.cpp.
Generated on Sun Jul 17 2022 01:34:26 by
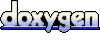