ms2s,STM32 Private
Fork of WakeUp by
Embed:
(wiki syntax)
Show/hide line numbers
WakeUp.h
00001 #include "mbed.h" 00002 00003 /** 00004 * Class to make wake up a microcontroller from deepsleep using a low-power timer. 00005 * 00006 * @code 00007 * // Depending on the LED connections either the LED is off the 2 seconds 00008 * // the target spends in deepsleep(), and on for the other second. Or it is inverted 00009 * 00010 * #include "mbed.h" 00011 * #include "WakeUp.h" 00012 * 00013 * DigitalOut myled(LED1); 00014 * 00015 * int main() { 00016 * wait(5); 00017 * 00018 * //The low-power oscillator can be quite inaccurate on some targets 00019 * //this function calibrates it against the main clock 00020 * WakeUp::calibrate(); 00021 * 00022 * while(1) { 00023 * //Set LED to zero 00024 * myled = 0; 00025 * 00026 * //Set wakeup time for 2 seconds 00027 * WakeUp::set_ms(2000); 00028 * 00029 * //Enter deepsleep, the program won't go beyond this point until it is woken up 00030 * deepsleep(); 00031 * 00032 * //Set LED for 1 second to one 00033 * myled = 1; 00034 * wait(1); 00035 * } 00036 * } 00037 * @endcode 00038 */ 00039 class WakeUp 00040 { 00041 public: 00042 /** 00043 * Set the timeout 00044 * 00045 * @param s required time in seconds 00046 */ 00047 static void set(uint32_t s) { 00048 set_ms(1000 * s); 00049 } 00050 00051 /** 00052 * Set the timeout 00053 * 00054 * @param ms required time in milliseconds 00055 */ 00056 static void set_ms(uint32_t ms); 00057 00058 /** 00059 * Attach a function to be called after timeout 00060 * 00061 * This is optional, if you just want to wake up you 00062 * do not need to attach a function. 00063 * 00064 * Important: Many targets will run the wake-up routine 00065 * at reduced clock speed, afterwards clock speed is restored. 00066 * This means that clock speed dependent functions, such as printf 00067 * might end up distorted. 00068 * 00069 * Also supports normal way to attach member functions 00070 * (not documented for simplicity) 00071 * 00072 * @param *function function to call 00073 */ 00074 static void attach(void (*function)(void)) { 00075 callback.attach(function); 00076 } 00077 00078 template<typename T> 00079 static void attach(T *object, void (T::*member)(void)) { 00080 callback.attach(object, member); 00081 } 00082 00083 /** 00084 * Calibrate the timer 00085 * 00086 * Some of the low-power timers have very bad accuracy. 00087 * This function calibrates it against the main timer. 00088 * 00089 * Warning: Blocks for 100ms! 00090 */ 00091 static void calibrate(void); 00092 00093 00094 private: 00095 static FunctionPointer callback; 00096 static void irq_handler(void); 00097 static float cycles_per_ms; 00098 };
Generated on Sun Jul 17 2022 01:34:26 by
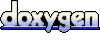