
LINKED LIST TEST on mbed
Embed:
(wiki syntax)
Show/hide line numbers
LPC17xx.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file LPC17xx.h 00003 * @brief CMSIS Cortex-M3 Core Peripheral Access Layer Header File for 00004 * NXP LPC17xx Device Series 00005 * @version: V1.08 00006 * @date: 21. December 2009 00007 * 00008 * @note 00009 * Copyright (C) 2009 ARM Limited. All rights reserved. 00010 * 00011 * @par 00012 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00013 * processor based microcontrollers. This file can be freely distributed 00014 * within development tools that are supporting such ARM based processors. 00015 * 00016 * @par 00017 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00018 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00019 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00020 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00021 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00022 * 00023 ******************************************************************************/ 00024 00025 00026 #ifndef __LPC17xx_H__ 00027 #define __LPC17xx_H__ 00028 00029 /* 00030 * ========================================================================== 00031 * ---------- Interrupt Number Definition ----------------------------------- 00032 * ========================================================================== 00033 */ 00034 00035 /** @addtogroup LPC17xx_System 00036 * @{ 00037 */ 00038 00039 /** @brief IRQ interrupt source definition */ 00040 typedef enum IRQn 00041 { 00042 /****** Cortex-M3 Processor Exceptions Numbers ***************************************************/ 00043 NonMaskableInt_IRQn = -14, /*!< 2 Non Maskable Interrupt */ 00044 MemoryManagement_IRQn = -12, /*!< 4 Cortex-M3 Memory Management Interrupt */ 00045 BusFault_IRQn = -11, /*!< 5 Cortex-M3 Bus Fault Interrupt */ 00046 UsageFault_IRQn = -10, /*!< 6 Cortex-M3 Usage Fault Interrupt */ 00047 SVCall_IRQn = -5, /*!< 11 Cortex-M3 SV Call Interrupt */ 00048 DebugMonitor_IRQn = -4, /*!< 12 Cortex-M3 Debug Monitor Interrupt */ 00049 PendSV_IRQn = -2, /*!< 14 Cortex-M3 Pend SV Interrupt */ 00050 SysTick_IRQn = -1, /*!< 15 Cortex-M3 System Tick Interrupt */ 00051 00052 /****** LPC17xx Specific Interrupt Numbers *******************************************************/ 00053 WDT_IRQn = 0, /*!< Watchdog Timer Interrupt */ 00054 TIMER0_IRQn = 1, /*!< Timer0 Interrupt */ 00055 TIMER1_IRQn = 2, /*!< Timer1 Interrupt */ 00056 TIMER2_IRQn = 3, /*!< Timer2 Interrupt */ 00057 TIMER3_IRQn = 4, /*!< Timer3 Interrupt */ 00058 UART0_IRQn = 5, /*!< UART0 Interrupt */ 00059 UART1_IRQn = 6, /*!< UART1 Interrupt */ 00060 UART2_IRQn = 7, /*!< UART2 Interrupt */ 00061 UART3_IRQn = 8, /*!< UART3 Interrupt */ 00062 PWM1_IRQn = 9, /*!< PWM1 Interrupt */ 00063 I2C0_IRQn = 10, /*!< I2C0 Interrupt */ 00064 I2C1_IRQn = 11, /*!< I2C1 Interrupt */ 00065 I2C2_IRQn = 12, /*!< I2C2 Interrupt */ 00066 SPI_IRQn = 13, /*!< SPI Interrupt */ 00067 SSP0_IRQn = 14, /*!< SSP0 Interrupt */ 00068 SSP1_IRQn = 15, /*!< SSP1 Interrupt */ 00069 PLL0_IRQn = 16, /*!< PLL0 Lock (Main PLL) Interrupt */ 00070 RTC_IRQn = 17, /*!< Real Time Clock Interrupt */ 00071 EINT0_IRQn = 18, /*!< External Interrupt 0 Interrupt */ 00072 EINT1_IRQn = 19, /*!< External Interrupt 1 Interrupt */ 00073 EINT2_IRQn = 20, /*!< External Interrupt 2 Interrupt */ 00074 EINT3_IRQn = 21, /*!< External Interrupt 3 Interrupt */ 00075 ADC_IRQn = 22, /*!< A/D Converter Interrupt */ 00076 BOD_IRQn = 23, /*!< Brown-Out Detect Interrupt */ 00077 USB_IRQn = 24, /*!< USB Interrupt */ 00078 CAN_IRQn = 25, /*!< CAN Interrupt */ 00079 DMA_IRQn = 26, /*!< General Purpose DMA Interrupt */ 00080 I2S_IRQn = 27, /*!< I2S Interrupt */ 00081 ENET_IRQn = 28, /*!< Ethernet Interrupt */ 00082 RIT_IRQn = 29, /*!< Repetitive Interrupt Timer Interrupt */ 00083 MCPWM_IRQn = 30, /*!< Motor Control PWM Interrupt */ 00084 QEI_IRQn = 31, /*!< Quadrature Encoder Interface Interrupt */ 00085 PLL1_IRQn = 32, /*!< PLL1 Lock (USB PLL) Interrupt */ 00086 USBActivity_IRQn = 33, /*!< USB Activity Interrupt */ 00087 CANActivity_IRQn = 34, /*!< CAN Activity Interrupt */ 00088 } IRQn_Type; 00089 00090 00091 /* 00092 * ========================================================================== 00093 * ----------- Processor and Core Peripheral Section ------------------------ 00094 * ========================================================================== 00095 */ 00096 00097 /* Configuration of the Cortex-M3 Processor and Core Peripherals */ 00098 #define __MPU_PRESENT 1 /*!< MPU present or not */ 00099 #define __NVIC_PRIO_BITS 5 /*!< Number of Bits used for Priority Levels */ 00100 #define __Vendor_SysTickConfig 0 /*!< Set to 1 if different SysTick Config is used */ 00101 00102 00103 #include "core_cm3.h" /* Cortex-M3 processor and core peripherals */ 00104 #include "system_LPC17xx.h" /* System Header */ 00105 00106 00107 /******************************************************************************/ 00108 /* Device Specific Peripheral registers structures */ 00109 /******************************************************************************/ 00110 00111 #if defined ( __CC_ARM ) 00112 #pragma anon_unions 00113 #endif 00114 00115 /*------------- System Control (SC) ------------------------------------------*/ 00116 /** @brief System Control (SC) register structure definition */ 00117 typedef struct 00118 { 00119 __IO uint32_t FLASHCFG; /* Flash Accelerator Module */ 00120 uint32_t RESERVED0[31]; 00121 __IO uint32_t PLL0CON; /* Clocking and Power Control */ 00122 __IO uint32_t PLL0CFG; 00123 __I uint32_t PLL0STAT; 00124 __O uint32_t PLL0FEED; 00125 uint32_t RESERVED1[4]; 00126 __IO uint32_t PLL1CON; 00127 __IO uint32_t PLL1CFG; 00128 __I uint32_t PLL1STAT; 00129 __O uint32_t PLL1FEED; 00130 uint32_t RESERVED2[4]; 00131 __IO uint32_t PCON; 00132 __IO uint32_t PCONP; 00133 uint32_t RESERVED3[15]; 00134 __IO uint32_t CCLKCFG; 00135 __IO uint32_t USBCLKCFG; 00136 __IO uint32_t CLKSRCSEL; 00137 __IO uint32_t CANSLEEPCLR; 00138 __IO uint32_t CANWAKEFLAGS; 00139 uint32_t RESERVED4[10]; 00140 __IO uint32_t EXTINT; /* External Interrupts */ 00141 uint32_t RESERVED5; 00142 __IO uint32_t EXTMODE; 00143 __IO uint32_t EXTPOLAR; 00144 uint32_t RESERVED6[12]; 00145 __IO uint32_t RSID; /* Reset */ 00146 uint32_t RESERVED7[7]; 00147 __IO uint32_t SCS; /* Syscon Miscellaneous Registers */ 00148 __IO uint32_t IRCTRIM; /* Clock Dividers */ 00149 __IO uint32_t PCLKSEL0; 00150 __IO uint32_t PCLKSEL1; 00151 uint32_t RESERVED8[4]; 00152 __IO uint32_t USBIntSt; /* USB Device/OTG Interrupt Register */ 00153 __IO uint32_t DMAREQSEL; 00154 __IO uint32_t CLKOUTCFG; /* Clock Output Configuration */ 00155 } LPC_SC_TypeDef; 00156 00157 /*------------- Pin Connect Block (PINCON) -----------------------------------*/ 00158 /** @brief Pin Connect Block (PINCON) register structure definition */ 00159 typedef struct 00160 { 00161 __IO uint32_t PINSEL0; 00162 __IO uint32_t PINSEL1; 00163 __IO uint32_t PINSEL2; 00164 __IO uint32_t PINSEL3; 00165 __IO uint32_t PINSEL4; 00166 __IO uint32_t PINSEL5; 00167 __IO uint32_t PINSEL6; 00168 __IO uint32_t PINSEL7; 00169 __IO uint32_t PINSEL8; 00170 __IO uint32_t PINSEL9; 00171 __IO uint32_t PINSEL10; 00172 uint32_t RESERVED0[5]; 00173 __IO uint32_t PINMODE0; 00174 __IO uint32_t PINMODE1; 00175 __IO uint32_t PINMODE2; 00176 __IO uint32_t PINMODE3; 00177 __IO uint32_t PINMODE4; 00178 __IO uint32_t PINMODE5; 00179 __IO uint32_t PINMODE6; 00180 __IO uint32_t PINMODE7; 00181 __IO uint32_t PINMODE8; 00182 __IO uint32_t PINMODE9; 00183 __IO uint32_t PINMODE_OD0; 00184 __IO uint32_t PINMODE_OD1; 00185 __IO uint32_t PINMODE_OD2; 00186 __IO uint32_t PINMODE_OD3; 00187 __IO uint32_t PINMODE_OD4; 00188 __IO uint32_t I2CPADCFG; 00189 } LPC_PINCON_TypeDef; 00190 00191 /*------------- General Purpose Input/Output (GPIO) --------------------------*/ 00192 /** @brief General Purpose Input/Output (GPIO) register structure definition */ 00193 typedef struct 00194 { 00195 union { 00196 __IO uint32_t FIODIR; 00197 struct { 00198 __IO uint16_t FIODIRL; 00199 __IO uint16_t FIODIRH; 00200 }; 00201 struct { 00202 __IO uint8_t FIODIR0; 00203 __IO uint8_t FIODIR1; 00204 __IO uint8_t FIODIR2; 00205 __IO uint8_t FIODIR3; 00206 }; 00207 }; 00208 uint32_t RESERVED0[3]; 00209 union { 00210 __IO uint32_t FIOMASK; 00211 struct { 00212 __IO uint16_t FIOMASKL; 00213 __IO uint16_t FIOMASKH; 00214 }; 00215 struct { 00216 __IO uint8_t FIOMASK0; 00217 __IO uint8_t FIOMASK1; 00218 __IO uint8_t FIOMASK2; 00219 __IO uint8_t FIOMASK3; 00220 }; 00221 }; 00222 union { 00223 __IO uint32_t FIOPIN; 00224 struct { 00225 __IO uint16_t FIOPINL; 00226 __IO uint16_t FIOPINH; 00227 }; 00228 struct { 00229 __IO uint8_t FIOPIN0; 00230 __IO uint8_t FIOPIN1; 00231 __IO uint8_t FIOPIN2; 00232 __IO uint8_t FIOPIN3; 00233 }; 00234 }; 00235 union { 00236 __IO uint32_t FIOSET; 00237 struct { 00238 __IO uint16_t FIOSETL; 00239 __IO uint16_t FIOSETH; 00240 }; 00241 struct { 00242 __IO uint8_t FIOSET0; 00243 __IO uint8_t FIOSET1; 00244 __IO uint8_t FIOSET2; 00245 __IO uint8_t FIOSET3; 00246 }; 00247 }; 00248 union { 00249 __O uint32_t FIOCLR; 00250 struct { 00251 __O uint16_t FIOCLRL; 00252 __O uint16_t FIOCLRH; 00253 }; 00254 struct { 00255 __O uint8_t FIOCLR0; 00256 __O uint8_t FIOCLR1; 00257 __O uint8_t FIOCLR2; 00258 __O uint8_t FIOCLR3; 00259 }; 00260 }; 00261 } LPC_GPIO_TypeDef; 00262 00263 /** @brief General Purpose Input/Output interrupt (GPIOINT) register structure definition */ 00264 typedef struct 00265 { 00266 __I uint32_t IntStatus; 00267 __I uint32_t IO0IntStatR; 00268 __I uint32_t IO0IntStatF; 00269 __O uint32_t IO0IntClr; 00270 __IO uint32_t IO0IntEnR; 00271 __IO uint32_t IO0IntEnF; 00272 uint32_t RESERVED0[3]; 00273 __I uint32_t IO2IntStatR; 00274 __I uint32_t IO2IntStatF; 00275 __O uint32_t IO2IntClr; 00276 __IO uint32_t IO2IntEnR; 00277 __IO uint32_t IO2IntEnF; 00278 } LPC_GPIOINT_TypeDef; 00279 00280 /*------------- Timer (TIM) --------------------------------------------------*/ 00281 /** @brief Timer (TIM) register structure definition */ 00282 typedef struct 00283 { 00284 __IO uint32_t IR; 00285 __IO uint32_t TCR; 00286 __IO uint32_t TC; 00287 __IO uint32_t PR; 00288 __IO uint32_t PC; 00289 __IO uint32_t MCR; 00290 __IO uint32_t MR0; 00291 __IO uint32_t MR1; 00292 __IO uint32_t MR2; 00293 __IO uint32_t MR3; 00294 __IO uint32_t CCR; 00295 __I uint32_t CR0; 00296 __I uint32_t CR1; 00297 uint32_t RESERVED0[2]; 00298 __IO uint32_t EMR; 00299 uint32_t RESERVED1[12]; 00300 __IO uint32_t CTCR; 00301 } LPC_TIM_TypeDef; 00302 00303 /*------------- Pulse-Width Modulation (PWM) ---------------------------------*/ 00304 /** @brief Pulse-Width Modulation (PWM) register structure definition */ 00305 typedef struct 00306 { 00307 __IO uint32_t IR; 00308 __IO uint32_t TCR; 00309 __IO uint32_t TC; 00310 __IO uint32_t PR; 00311 __IO uint32_t PC; 00312 __IO uint32_t MCR; 00313 __IO uint32_t MR0; 00314 __IO uint32_t MR1; 00315 __IO uint32_t MR2; 00316 __IO uint32_t MR3; 00317 __IO uint32_t CCR; 00318 __I uint32_t CR0; 00319 __I uint32_t CR1; 00320 __I uint32_t CR2; 00321 __I uint32_t CR3; 00322 uint32_t RESERVED0; 00323 __IO uint32_t MR4; 00324 __IO uint32_t MR5; 00325 __IO uint32_t MR6; 00326 __IO uint32_t PCR; 00327 __IO uint32_t LER; 00328 uint32_t RESERVED1[7]; 00329 __IO uint32_t CTCR; 00330 } LPC_PWM_TypeDef; 00331 00332 /*------------- Universal Asynchronous Receiver Transmitter (UART) -----------*/ 00333 /** @brief Universal Asynchronous Receiver Transmitter (UART) register structure definition */ 00334 typedef struct 00335 { 00336 union { 00337 __I uint8_t RBR; 00338 __O uint8_t THR; 00339 __IO uint8_t DLL; 00340 uint32_t RESERVED0; 00341 }; 00342 union { 00343 __IO uint8_t DLM; 00344 __IO uint32_t IER; 00345 }; 00346 union { 00347 __I uint32_t IIR; 00348 __O uint8_t FCR; 00349 }; 00350 __IO uint8_t LCR; 00351 uint8_t RESERVED1[7]; 00352 __I uint8_t LSR; 00353 uint8_t RESERVED2[7]; 00354 __IO uint8_t SCR; 00355 uint8_t RESERVED3[3]; 00356 __IO uint32_t ACR; 00357 __IO uint8_t ICR; 00358 uint8_t RESERVED4[3]; 00359 __IO uint8_t FDR; 00360 uint8_t RESERVED5[7]; 00361 __IO uint8_t TER; 00362 uint8_t RESERVED6[39]; 00363 __I uint8_t FIFOLVL; 00364 } LPC_UART_TypeDef; 00365 00366 /** @brief Universal Asynchronous Receiver Transmitter 0 (UART0) register structure definition */ 00367 typedef struct 00368 { 00369 union { 00370 __I uint8_t RBR; 00371 __O uint8_t THR; 00372 __IO uint8_t DLL; 00373 uint32_t RESERVED0; 00374 }; 00375 union { 00376 __IO uint8_t DLM; 00377 __IO uint32_t IER; 00378 }; 00379 union { 00380 __I uint32_t IIR; 00381 __O uint8_t FCR; 00382 }; 00383 __IO uint8_t LCR; 00384 uint8_t RESERVED1[7]; 00385 __I uint8_t LSR; 00386 uint8_t RESERVED2[7]; 00387 __IO uint8_t SCR; 00388 uint8_t RESERVED3[3]; 00389 __IO uint32_t ACR; 00390 __IO uint8_t ICR; 00391 uint8_t RESERVED4[3]; 00392 __IO uint8_t FDR; 00393 uint8_t RESERVED5[7]; 00394 __IO uint8_t TER; 00395 uint8_t RESERVED6[39]; 00396 __I uint8_t FIFOLVL; 00397 } LPC_UART0_TypeDef; 00398 00399 /** @brief Universal Asynchronous Receiver Transmitter 1 (UART1) register structure definition */ 00400 typedef struct 00401 { 00402 union { 00403 __I uint8_t RBR; 00404 __O uint8_t THR; 00405 __IO uint8_t DLL; 00406 uint32_t RESERVED0; 00407 }; 00408 union { 00409 __IO uint8_t DLM; 00410 __IO uint32_t IER; 00411 }; 00412 union { 00413 __I uint32_t IIR; 00414 __O uint8_t FCR; 00415 }; 00416 __IO uint8_t LCR; 00417 uint8_t RESERVED1[3]; 00418 __IO uint8_t MCR; 00419 uint8_t RESERVED2[3]; 00420 __I uint8_t LSR; 00421 uint8_t RESERVED3[3]; 00422 __I uint8_t MSR; 00423 uint8_t RESERVED4[3]; 00424 __IO uint8_t SCR; 00425 uint8_t RESERVED5[3]; 00426 __IO uint32_t ACR; 00427 uint32_t RESERVED6; 00428 __IO uint32_t FDR; 00429 uint32_t RESERVED7; 00430 __IO uint8_t TER; 00431 uint8_t RESERVED8[27]; 00432 __IO uint8_t RS485CTRL; 00433 uint8_t RESERVED9[3]; 00434 __IO uint8_t ADRMATCH; 00435 uint8_t RESERVED10[3]; 00436 __IO uint8_t RS485DLY; 00437 uint8_t RESERVED11[3]; 00438 __I uint8_t FIFOLVL; 00439 } LPC_UART1_TypeDef; 00440 00441 /*------------- Serial Peripheral Interface (SPI) ----------------------------*/ 00442 /** @brief Serial Peripheral Interface (SPI) register structure definition */ 00443 typedef struct 00444 { 00445 __IO uint32_t SPCR; 00446 __I uint32_t SPSR; 00447 __IO uint32_t SPDR; 00448 __IO uint32_t SPCCR; 00449 uint32_t RESERVED0[3]; 00450 __IO uint32_t SPINT; 00451 } LPC_SPI_TypeDef; 00452 00453 /*------------- Synchronous Serial Communication (SSP) -----------------------*/ 00454 /** @brief Synchronous Serial Communication (SSP) register structure definition */ 00455 typedef struct 00456 { 00457 __IO uint32_t CR0; 00458 __IO uint32_t CR1; 00459 __IO uint32_t DR; 00460 __I uint32_t SR; 00461 __IO uint32_t CPSR; 00462 __IO uint32_t IMSC; 00463 __IO uint32_t RIS; 00464 __IO uint32_t MIS; 00465 __IO uint32_t ICR; 00466 __IO uint32_t DMACR; 00467 } LPC_SSP_TypeDef; 00468 00469 /*------------- Inter-Integrated Circuit (I2C) -------------------------------*/ 00470 /** @brief Inter-Integrated Circuit (I2C) register structure definition */ 00471 typedef struct 00472 { 00473 __IO uint32_t I2CONSET; 00474 __I uint32_t I2STAT; 00475 __IO uint32_t I2DAT; 00476 __IO uint32_t I2ADR0; 00477 __IO uint32_t I2SCLH; 00478 __IO uint32_t I2SCLL; 00479 __O uint32_t I2CONCLR; 00480 __IO uint32_t MMCTRL; 00481 __IO uint32_t I2ADR1; 00482 __IO uint32_t I2ADR2; 00483 __IO uint32_t I2ADR3; 00484 __I uint32_t I2DATA_BUFFER; 00485 __IO uint32_t I2MASK0; 00486 __IO uint32_t I2MASK1; 00487 __IO uint32_t I2MASK2; 00488 __IO uint32_t I2MASK3; 00489 } LPC_I2C_TypeDef; 00490 00491 /*------------- Inter IC Sound (I2S) -----------------------------------------*/ 00492 /** @brief Inter IC Sound (I2S) register structure definition */ 00493 typedef struct 00494 { 00495 __IO uint32_t I2SDAO; 00496 __IO uint32_t I2SDAI; 00497 __O uint32_t I2STXFIFO; 00498 __I uint32_t I2SRXFIFO; 00499 __I uint32_t I2SSTATE; 00500 __IO uint32_t I2SDMA1; 00501 __IO uint32_t I2SDMA2; 00502 __IO uint32_t I2SIRQ; 00503 __IO uint32_t I2STXRATE; 00504 __IO uint32_t I2SRXRATE; 00505 __IO uint32_t I2STXBITRATE; 00506 __IO uint32_t I2SRXBITRATE; 00507 __IO uint32_t I2STXMODE; 00508 __IO uint32_t I2SRXMODE; 00509 } LPC_I2S_TypeDef; 00510 00511 /*------------- Repetitive Interrupt Timer (RIT) -----------------------------*/ 00512 /** @brief Repetitive Interrupt Timer (RIT) register structure definition */ 00513 typedef struct 00514 { 00515 __IO uint32_t RICOMPVAL; 00516 __IO uint32_t RIMASK; 00517 __IO uint8_t RICTRL; 00518 uint8_t RESERVED0[3]; 00519 __IO uint32_t RICOUNTER; 00520 } LPC_RIT_TypeDef; 00521 00522 /*------------- Real-Time Clock (RTC) ----------------------------------------*/ 00523 /** @brief Real-Time Clock (RTC) register structure definition */ 00524 typedef struct 00525 { 00526 __IO uint8_t ILR; 00527 uint8_t RESERVED0[7]; 00528 __IO uint8_t CCR; 00529 uint8_t RESERVED1[3]; 00530 __IO uint8_t CIIR; 00531 uint8_t RESERVED2[3]; 00532 __IO uint8_t AMR; 00533 uint8_t RESERVED3[3]; 00534 __I uint32_t CTIME0; 00535 __I uint32_t CTIME1; 00536 __I uint32_t CTIME2; 00537 __IO uint8_t SEC; 00538 uint8_t RESERVED4[3]; 00539 __IO uint8_t MIN; 00540 uint8_t RESERVED5[3]; 00541 __IO uint8_t HOUR; 00542 uint8_t RESERVED6[3]; 00543 __IO uint8_t DOM; 00544 uint8_t RESERVED7[3]; 00545 __IO uint8_t DOW; 00546 uint8_t RESERVED8[3]; 00547 __IO uint16_t DOY; 00548 uint16_t RESERVED9; 00549 __IO uint8_t MONTH; 00550 uint8_t RESERVED10[3]; 00551 __IO uint16_t YEAR; 00552 uint16_t RESERVED11; 00553 __IO uint32_t CALIBRATION; 00554 __IO uint32_t GPREG0; 00555 __IO uint32_t GPREG1; 00556 __IO uint32_t GPREG2; 00557 __IO uint32_t GPREG3; 00558 __IO uint32_t GPREG4; 00559 __IO uint8_t RTC_AUXEN; 00560 uint8_t RESERVED12[3]; 00561 __IO uint8_t RTC_AUX; 00562 uint8_t RESERVED13[3]; 00563 __IO uint8_t ALSEC; 00564 uint8_t RESERVED14[3]; 00565 __IO uint8_t ALMIN; 00566 uint8_t RESERVED15[3]; 00567 __IO uint8_t ALHOUR; 00568 uint8_t RESERVED16[3]; 00569 __IO uint8_t ALDOM; 00570 uint8_t RESERVED17[3]; 00571 __IO uint8_t ALDOW; 00572 uint8_t RESERVED18[3]; 00573 __IO uint16_t ALDOY; 00574 uint16_t RESERVED19; 00575 __IO uint8_t ALMON; 00576 uint8_t RESERVED20[3]; 00577 __IO uint16_t ALYEAR; 00578 uint16_t RESERVED21; 00579 } LPC_RTC_TypeDef; 00580 00581 /*------------- Watchdog Timer (WDT) -----------------------------------------*/ 00582 /** @brief Watchdog Timer (WDT) register structure definition */ 00583 typedef struct 00584 { 00585 __IO uint8_t WDMOD; 00586 uint8_t RESERVED0[3]; 00587 __IO uint32_t WDTC; 00588 __O uint8_t WDFEED; 00589 uint8_t RESERVED1[3]; 00590 __I uint32_t WDTV; 00591 __IO uint32_t WDCLKSEL; 00592 } LPC_WDT_TypeDef; 00593 00594 /*------------- Analog-to-Digital Converter (ADC) ----------------------------*/ 00595 /** @brief Analog-to-Digital Converter (ADC) register structure definition */ 00596 typedef struct 00597 { 00598 __IO uint32_t ADCR; 00599 __IO uint32_t ADGDR; 00600 uint32_t RESERVED0; 00601 __IO uint32_t ADINTEN; 00602 __I uint32_t ADDR0; 00603 __I uint32_t ADDR1; 00604 __I uint32_t ADDR2; 00605 __I uint32_t ADDR3; 00606 __I uint32_t ADDR4; 00607 __I uint32_t ADDR5; 00608 __I uint32_t ADDR6; 00609 __I uint32_t ADDR7; 00610 __I uint32_t ADSTAT; 00611 __IO uint32_t ADTRM; 00612 } LPC_ADC_TypeDef; 00613 00614 /*------------- Digital-to-Analog Converter (DAC) ----------------------------*/ 00615 /** @brief Digital-to-Analog Converter (DAC) register structure definition */ 00616 typedef struct 00617 { 00618 __IO uint32_t DACR; 00619 __IO uint32_t DACCTRL; 00620 __IO uint16_t DACCNTVAL; 00621 } LPC_DAC_TypeDef; 00622 00623 /*------------- Motor Control Pulse-Width Modulation (MCPWM) -----------------*/ 00624 /** @brief Motor Control Pulse-Width Modulation (MCPWM) register structure definition */ 00625 typedef struct 00626 { 00627 __I uint32_t MCCON; 00628 __O uint32_t MCCON_SET; 00629 __O uint32_t MCCON_CLR; 00630 __I uint32_t MCCAPCON; 00631 __O uint32_t MCCAPCON_SET; 00632 __O uint32_t MCCAPCON_CLR; 00633 __IO uint32_t MCTIM0; 00634 __IO uint32_t MCTIM1; 00635 __IO uint32_t MCTIM2; 00636 __IO uint32_t MCPER0; 00637 __IO uint32_t MCPER1; 00638 __IO uint32_t MCPER2; 00639 __IO uint32_t MCPW0; 00640 __IO uint32_t MCPW1; 00641 __IO uint32_t MCPW2; 00642 __IO uint32_t MCDEADTIME; 00643 __IO uint32_t MCCCP; 00644 __IO uint32_t MCCR0; 00645 __IO uint32_t MCCR1; 00646 __IO uint32_t MCCR2; 00647 __I uint32_t MCINTEN; 00648 __O uint32_t MCINTEN_SET; 00649 __O uint32_t MCINTEN_CLR; 00650 __I uint32_t MCCNTCON; 00651 __O uint32_t MCCNTCON_SET; 00652 __O uint32_t MCCNTCON_CLR; 00653 __I uint32_t MCINTFLAG; 00654 __O uint32_t MCINTFLAG_SET; 00655 __O uint32_t MCINTFLAG_CLR; 00656 __O uint32_t MCCAP_CLR; 00657 } LPC_MCPWM_TypeDef; 00658 00659 /*------------- Quadrature Encoder Interface (QEI) ---------------------------*/ 00660 /** @brief Quadrature Encoder Interface (QEI) register structure definition */ 00661 typedef struct 00662 { 00663 __O uint32_t QEICON; 00664 __I uint32_t QEISTAT; 00665 __IO uint32_t QEICONF; 00666 __I uint32_t QEIPOS; 00667 __IO uint32_t QEIMAXPOS; 00668 __IO uint32_t CMPOS0; 00669 __IO uint32_t CMPOS1; 00670 __IO uint32_t CMPOS2; 00671 __I uint32_t INXCNT; 00672 __IO uint32_t INXCMP; 00673 __IO uint32_t QEILOAD; 00674 __I uint32_t QEITIME; 00675 __I uint32_t QEIVEL; 00676 __I uint32_t QEICAP; 00677 __IO uint32_t VELCOMP; 00678 __IO uint32_t FILTER; 00679 uint32_t RESERVED0[998]; 00680 __O uint32_t QEIIEC; 00681 __O uint32_t QEIIES; 00682 __I uint32_t QEIINTSTAT; 00683 __I uint32_t QEIIE; 00684 __O uint32_t QEICLR; 00685 __O uint32_t QEISET; 00686 } LPC_QEI_TypeDef; 00687 00688 /*------------- Controller Area Network (CAN) --------------------------------*/ 00689 /** @brief Controller Area Network Acceptance Filter RAM (CANAF_RAM)structure definition */ 00690 typedef struct 00691 { 00692 __IO uint32_t mask[512]; /* ID Masks */ 00693 } LPC_CANAF_RAM_TypeDef; 00694 00695 /** @brief Controller Area Network Acceptance Filter(CANAF) register structure definition */ 00696 typedef struct /* Acceptance Filter Registers */ 00697 { 00698 __IO uint32_t AFMR; 00699 __IO uint32_t SFF_sa; 00700 __IO uint32_t SFF_GRP_sa; 00701 __IO uint32_t EFF_sa; 00702 __IO uint32_t EFF_GRP_sa; 00703 __IO uint32_t ENDofTable; 00704 __I uint32_t LUTerrAd; 00705 __I uint32_t LUTerr; 00706 __IO uint32_t FCANIE; 00707 __IO uint32_t FCANIC0; 00708 __IO uint32_t FCANIC1; 00709 } LPC_CANAF_TypeDef; 00710 00711 /** @brief Controller Area Network Central (CANCR) register structure definition */ 00712 typedef struct /* Central Registers */ 00713 { 00714 __I uint32_t CANTxSR; 00715 __I uint32_t CANRxSR; 00716 __I uint32_t CANMSR; 00717 } LPC_CANCR_TypeDef; 00718 00719 /** @brief Controller Area Network Controller (CAN) register structure definition */ 00720 typedef struct /* Controller Registers */ 00721 { 00722 __IO uint32_t MOD; 00723 __O uint32_t CMR; 00724 __IO uint32_t GSR; 00725 __I uint32_t ICR; 00726 __IO uint32_t IER; 00727 __IO uint32_t BTR; 00728 __IO uint32_t EWL; 00729 __I uint32_t SR; 00730 __IO uint32_t RFS; 00731 __IO uint32_t RID; 00732 __IO uint32_t RDA; 00733 __IO uint32_t RDB; 00734 __IO uint32_t TFI1; 00735 __IO uint32_t TID1; 00736 __IO uint32_t TDA1; 00737 __IO uint32_t TDB1; 00738 __IO uint32_t TFI2; 00739 __IO uint32_t TID2; 00740 __IO uint32_t TDA2; 00741 __IO uint32_t TDB2; 00742 __IO uint32_t TFI3; 00743 __IO uint32_t TID3; 00744 __IO uint32_t TDA3; 00745 __IO uint32_t TDB3; 00746 } LPC_CAN_TypeDef; 00747 00748 /*------------- General Purpose Direct Memory Access (GPDMA) -----------------*/ 00749 /** @brief General Purpose Direct Memory Access (GPDMA) register structure definition */ 00750 typedef struct /* Common Registers */ 00751 { 00752 __I uint32_t DMACIntStat; 00753 __I uint32_t DMACIntTCStat; 00754 __O uint32_t DMACIntTCClear; 00755 __I uint32_t DMACIntErrStat; 00756 __O uint32_t DMACIntErrClr; 00757 __I uint32_t DMACRawIntTCStat; 00758 __I uint32_t DMACRawIntErrStat; 00759 __I uint32_t DMACEnbldChns; 00760 __IO uint32_t DMACSoftBReq; 00761 __IO uint32_t DMACSoftSReq; 00762 __IO uint32_t DMACSoftLBReq; 00763 __IO uint32_t DMACSoftLSReq; 00764 __IO uint32_t DMACConfig; 00765 __IO uint32_t DMACSync; 00766 } LPC_GPDMA_TypeDef; 00767 00768 /** @brief General Purpose Direct Memory Access Channel (GPDMACH) register structure definition */ 00769 typedef struct /* Channel Registers */ 00770 { 00771 __IO uint32_t DMACCSrcAddr; 00772 __IO uint32_t DMACCDestAddr; 00773 __IO uint32_t DMACCLLI; 00774 __IO uint32_t DMACCControl; 00775 __IO uint32_t DMACCConfig; 00776 } LPC_GPDMACH_TypeDef; 00777 00778 /*------------- Universal Serial Bus (USB) -----------------------------------*/ 00779 /** @brief Universal Serial Bus (USB) register structure definition */ 00780 typedef struct 00781 { 00782 __I uint32_t HcRevision; /* USB Host Registers */ 00783 __IO uint32_t HcControl; 00784 __IO uint32_t HcCommandStatus; 00785 __IO uint32_t HcInterruptStatus; 00786 __IO uint32_t HcInterruptEnable; 00787 __IO uint32_t HcInterruptDisable; 00788 __IO uint32_t HcHCCA; 00789 __I uint32_t HcPeriodCurrentED; 00790 __IO uint32_t HcControlHeadED; 00791 __IO uint32_t HcControlCurrentED; 00792 __IO uint32_t HcBulkHeadED; 00793 __IO uint32_t HcBulkCurrentED; 00794 __I uint32_t HcDoneHead; 00795 __IO uint32_t HcFmInterval; 00796 __I uint32_t HcFmRemaining; 00797 __I uint32_t HcFmNumber; 00798 __IO uint32_t HcPeriodicStart; 00799 __IO uint32_t HcLSTreshold; 00800 __IO uint32_t HcRhDescriptorA; 00801 __IO uint32_t HcRhDescriptorB; 00802 __IO uint32_t HcRhStatus; 00803 __IO uint32_t HcRhPortStatus1; 00804 __IO uint32_t HcRhPortStatus2; 00805 uint32_t RESERVED0[40]; 00806 __I uint32_t Module_ID; 00807 00808 __I uint32_t OTGIntSt; /* USB On-The-Go Registers */ 00809 __IO uint32_t OTGIntEn; 00810 __O uint32_t OTGIntSet; 00811 __O uint32_t OTGIntClr; 00812 __IO uint32_t OTGStCtrl; 00813 __IO uint32_t OTGTmr; 00814 uint32_t RESERVED1[58]; 00815 00816 __I uint32_t USBDevIntSt; /* USB Device Interrupt Registers */ 00817 __IO uint32_t USBDevIntEn; 00818 __O uint32_t USBDevIntClr; 00819 __O uint32_t USBDevIntSet; 00820 00821 __O uint32_t USBCmdCode; /* USB Device SIE Command Registers */ 00822 __I uint32_t USBCmdData; 00823 00824 __I uint32_t USBRxData; /* USB Device Transfer Registers */ 00825 __O uint32_t USBTxData; 00826 __I uint32_t USBRxPLen; 00827 __O uint32_t USBTxPLen; 00828 __IO uint32_t USBCtrl; 00829 __O uint32_t USBDevIntPri; 00830 00831 __I uint32_t USBEpIntSt; /* USB Device Endpoint Interrupt Regs */ 00832 __IO uint32_t USBEpIntEn; 00833 __O uint32_t USBEpIntClr; 00834 __O uint32_t USBEpIntSet; 00835 __O uint32_t USBEpIntPri; 00836 00837 __IO uint32_t USBReEp; /* USB Device Endpoint Realization Reg*/ 00838 __O uint32_t USBEpInd; 00839 __IO uint32_t USBMaxPSize; 00840 00841 __I uint32_t USBDMARSt; /* USB Device DMA Registers */ 00842 __O uint32_t USBDMARClr; 00843 __O uint32_t USBDMARSet; 00844 uint32_t RESERVED2[9]; 00845 __IO uint32_t USBUDCAH; 00846 __I uint32_t USBEpDMASt; 00847 __O uint32_t USBEpDMAEn; 00848 __O uint32_t USBEpDMADis; 00849 __I uint32_t USBDMAIntSt; 00850 __IO uint32_t USBDMAIntEn; 00851 uint32_t RESERVED3[2]; 00852 __I uint32_t USBEoTIntSt; 00853 __O uint32_t USBEoTIntClr; 00854 __O uint32_t USBEoTIntSet; 00855 __I uint32_t USBNDDRIntSt; 00856 __O uint32_t USBNDDRIntClr; 00857 __O uint32_t USBNDDRIntSet; 00858 __I uint32_t USBSysErrIntSt; 00859 __O uint32_t USBSysErrIntClr; 00860 __O uint32_t USBSysErrIntSet; 00861 uint32_t RESERVED4[15]; 00862 00863 union { 00864 __I uint32_t I2C_RX; /* USB OTG I2C Registers */ 00865 __O uint32_t I2C_TX; 00866 }; 00867 __I uint32_t I2C_STS; 00868 __IO uint32_t I2C_CTL; 00869 __IO uint32_t I2C_CLKHI; 00870 __O uint32_t I2C_CLKLO; 00871 uint32_t RESERVED5[824]; 00872 00873 union { 00874 __IO uint32_t USBClkCtrl; /* USB Clock Control Registers */ 00875 __IO uint32_t OTGClkCtrl; 00876 }; 00877 union { 00878 __I uint32_t USBClkSt; 00879 __I uint32_t OTGClkSt; 00880 }; 00881 } LPC_USB_TypeDef; 00882 00883 /*------------- Ethernet Media Access Controller (EMAC) ----------------------*/ 00884 /** @brief Ethernet Media Access Controller (EMAC) register structure definition */ 00885 typedef struct 00886 { 00887 __IO uint32_t MAC1; /* MAC Registers */ 00888 __IO uint32_t MAC2; 00889 __IO uint32_t IPGT; 00890 __IO uint32_t IPGR; 00891 __IO uint32_t CLRT; 00892 __IO uint32_t MAXF; 00893 __IO uint32_t SUPP; 00894 __IO uint32_t TEST; 00895 __IO uint32_t MCFG; 00896 __IO uint32_t MCMD; 00897 __IO uint32_t MADR; 00898 __O uint32_t MWTD; 00899 __I uint32_t MRDD; 00900 __I uint32_t MIND; 00901 uint32_t RESERVED0[2]; 00902 __IO uint32_t SA0; 00903 __IO uint32_t SA1; 00904 __IO uint32_t SA2; 00905 uint32_t RESERVED1[45]; 00906 __IO uint32_t Command; /* Control Registers */ 00907 __I uint32_t Status; 00908 __IO uint32_t RxDescriptor; 00909 __IO uint32_t RxStatus; 00910 __IO uint32_t RxDescriptorNumber; 00911 __I uint32_t RxProduceIndex; 00912 __IO uint32_t RxConsumeIndex; 00913 __IO uint32_t TxDescriptor; 00914 __IO uint32_t TxStatus; 00915 __IO uint32_t TxDescriptorNumber; 00916 __IO uint32_t TxProduceIndex; 00917 __I uint32_t TxConsumeIndex; 00918 uint32_t RESERVED2[10]; 00919 __I uint32_t TSV0; 00920 __I uint32_t TSV1; 00921 __I uint32_t RSV; 00922 uint32_t RESERVED3[3]; 00923 __IO uint32_t FlowControlCounter; 00924 __I uint32_t FlowControlStatus; 00925 uint32_t RESERVED4[34]; 00926 __IO uint32_t RxFilterCtrl; /* Rx Filter Registers */ 00927 __IO uint32_t RxFilterWoLStatus; 00928 __IO uint32_t RxFilterWoLClear; 00929 uint32_t RESERVED5; 00930 __IO uint32_t HashFilterL; 00931 __IO uint32_t HashFilterH; 00932 uint32_t RESERVED6[882]; 00933 __I uint32_t IntStatus; /* Module Control Registers */ 00934 __IO uint32_t IntEnable; 00935 __O uint32_t IntClear; 00936 __O uint32_t IntSet; 00937 uint32_t RESERVED7; 00938 __IO uint32_t PowerDown; 00939 uint32_t RESERVED8; 00940 __IO uint32_t Module_ID; 00941 } LPC_EMAC_TypeDef; 00942 00943 00944 #if defined ( __CC_ARM ) 00945 #pragma no_anon_unions 00946 #endif 00947 00948 00949 /******************************************************************************/ 00950 /* Peripheral memory map */ 00951 /******************************************************************************/ 00952 /* Base addresses */ 00953 #define LPC_FLASH_BASE (0x00000000UL) 00954 #define LPC_RAM_BASE (0x10000000UL) 00955 #ifdef __LPC17XX_REV00 00956 #define LPC_AHBRAM0_BASE (0x20000000UL) 00957 #define LPC_AHBRAM1_BASE (0x20004000UL) 00958 #else 00959 #define LPC_AHBRAM0_BASE (0x2007C000UL) 00960 #define LPC_AHBRAM1_BASE (0x20080000UL) 00961 #endif 00962 #define LPC_GPIO_BASE (0x2009C000UL) 00963 #define LPC_APB0_BASE (0x40000000UL) 00964 #define LPC_APB1_BASE (0x40080000UL) 00965 #define LPC_AHB_BASE (0x50000000UL) 00966 #define LPC_CM3_BASE (0xE0000000UL) 00967 00968 /* APB0 peripherals */ 00969 #define LPC_WDT_BASE (LPC_APB0_BASE + 0x00000) 00970 #define LPC_TIM0_BASE (LPC_APB0_BASE + 0x04000) 00971 #define LPC_TIM1_BASE (LPC_APB0_BASE + 0x08000) 00972 #define LPC_UART0_BASE (LPC_APB0_BASE + 0x0C000) 00973 #define LPC_UART1_BASE (LPC_APB0_BASE + 0x10000) 00974 #define LPC_PWM1_BASE (LPC_APB0_BASE + 0x18000) 00975 #define LPC_I2C0_BASE (LPC_APB0_BASE + 0x1C000) 00976 #define LPC_SPI_BASE (LPC_APB0_BASE + 0x20000) 00977 #define LPC_RTC_BASE (LPC_APB0_BASE + 0x24000) 00978 #define LPC_GPIOINT_BASE (LPC_APB0_BASE + 0x28080) 00979 #define LPC_PINCON_BASE (LPC_APB0_BASE + 0x2C000) 00980 #define LPC_SSP1_BASE (LPC_APB0_BASE + 0x30000) 00981 #define LPC_ADC_BASE (LPC_APB0_BASE + 0x34000) 00982 #define LPC_CANAF_RAM_BASE (LPC_APB0_BASE + 0x38000) 00983 #define LPC_CANAF_BASE (LPC_APB0_BASE + 0x3C000) 00984 #define LPC_CANCR_BASE (LPC_APB0_BASE + 0x40000) 00985 #define LPC_CAN1_BASE (LPC_APB0_BASE + 0x44000) 00986 #define LPC_CAN2_BASE (LPC_APB0_BASE + 0x48000) 00987 #define LPC_I2C1_BASE (LPC_APB0_BASE + 0x5C000) 00988 00989 /* APB1 peripherals */ 00990 #define LPC_SSP0_BASE (LPC_APB1_BASE + 0x08000) 00991 #define LPC_DAC_BASE (LPC_APB1_BASE + 0x0C000) 00992 #define LPC_TIM2_BASE (LPC_APB1_BASE + 0x10000) 00993 #define LPC_TIM3_BASE (LPC_APB1_BASE + 0x14000) 00994 #define LPC_UART2_BASE (LPC_APB1_BASE + 0x18000) 00995 #define LPC_UART3_BASE (LPC_APB1_BASE + 0x1C000) 00996 #define LPC_I2C2_BASE (LPC_APB1_BASE + 0x20000) 00997 #define LPC_I2S_BASE (LPC_APB1_BASE + 0x28000) 00998 #define LPC_RIT_BASE (LPC_APB1_BASE + 0x30000) 00999 #define LPC_MCPWM_BASE (LPC_APB1_BASE + 0x38000) 01000 #define LPC_QEI_BASE (LPC_APB1_BASE + 0x3C000) 01001 #define LPC_SC_BASE (LPC_APB1_BASE + 0x7C000) 01002 01003 /* AHB peripherals */ 01004 #define LPC_EMAC_BASE (LPC_AHB_BASE + 0x00000) 01005 #define LPC_GPDMA_BASE (LPC_AHB_BASE + 0x04000) 01006 #define LPC_GPDMACH0_BASE (LPC_AHB_BASE + 0x04100) 01007 #define LPC_GPDMACH1_BASE (LPC_AHB_BASE + 0x04120) 01008 #define LPC_GPDMACH2_BASE (LPC_AHB_BASE + 0x04140) 01009 #define LPC_GPDMACH3_BASE (LPC_AHB_BASE + 0x04160) 01010 #define LPC_GPDMACH4_BASE (LPC_AHB_BASE + 0x04180) 01011 #define LPC_GPDMACH5_BASE (LPC_AHB_BASE + 0x041A0) 01012 #define LPC_GPDMACH6_BASE (LPC_AHB_BASE + 0x041C0) 01013 #define LPC_GPDMACH7_BASE (LPC_AHB_BASE + 0x041E0) 01014 #define LPC_USB_BASE (LPC_AHB_BASE + 0x0C000) 01015 01016 /* GPIOs */ 01017 #define LPC_GPIO0_BASE (LPC_GPIO_BASE + 0x00000) 01018 #define LPC_GPIO1_BASE (LPC_GPIO_BASE + 0x00020) 01019 #define LPC_GPIO2_BASE (LPC_GPIO_BASE + 0x00040) 01020 #define LPC_GPIO3_BASE (LPC_GPIO_BASE + 0x00060) 01021 #define LPC_GPIO4_BASE (LPC_GPIO_BASE + 0x00080) 01022 01023 /******************************************************************************/ 01024 /* Peripheral declaration */ 01025 /******************************************************************************/ 01026 #define LPC_SC ((LPC_SC_TypeDef *) LPC_SC_BASE ) 01027 #define LPC_GPIO0 ((LPC_GPIO_TypeDef *) LPC_GPIO0_BASE ) 01028 #define LPC_GPIO1 ((LPC_GPIO_TypeDef *) LPC_GPIO1_BASE ) 01029 #define LPC_GPIO2 ((LPC_GPIO_TypeDef *) LPC_GPIO2_BASE ) 01030 #define LPC_GPIO3 ((LPC_GPIO_TypeDef *) LPC_GPIO3_BASE ) 01031 #define LPC_GPIO4 ((LPC_GPIO_TypeDef *) LPC_GPIO4_BASE ) 01032 #define LPC_WDT ((LPC_WDT_TypeDef *) LPC_WDT_BASE ) 01033 #define LPC_TIM0 ((LPC_TIM_TypeDef *) LPC_TIM0_BASE ) 01034 #define LPC_TIM1 ((LPC_TIM_TypeDef *) LPC_TIM1_BASE ) 01035 #define LPC_TIM2 ((LPC_TIM_TypeDef *) LPC_TIM2_BASE ) 01036 #define LPC_TIM3 ((LPC_TIM_TypeDef *) LPC_TIM3_BASE ) 01037 #define LPC_RIT ((LPC_RIT_TypeDef *) LPC_RIT_BASE ) 01038 #define LPC_UART0 ((LPC_UART_TypeDef *) LPC_UART0_BASE ) 01039 #define LPC_UART1 ((LPC_UART1_TypeDef *) LPC_UART1_BASE ) 01040 #define LPC_UART2 ((LPC_UART_TypeDef *) LPC_UART2_BASE ) 01041 #define LPC_UART3 ((LPC_UART_TypeDef *) LPC_UART3_BASE ) 01042 #define LPC_PWM1 ((LPC_PWM_TypeDef *) LPC_PWM1_BASE ) 01043 #define LPC_I2C0 ((LPC_I2C_TypeDef *) LPC_I2C0_BASE ) 01044 #define LPC_I2C1 ((LPC_I2C_TypeDef *) LPC_I2C1_BASE ) 01045 #define LPC_I2C2 ((LPC_I2C_TypeDef *) LPC_I2C2_BASE ) 01046 #define LPC_I2S ((LPC_I2S_TypeDef *) LPC_I2S_BASE ) 01047 #define LPC_SPI ((LPC_SPI_TypeDef *) LPC_SPI_BASE ) 01048 #define LPC_RTC ((LPC_RTC_TypeDef *) LPC_RTC_BASE ) 01049 #define LPC_GPIOINT ((LPC_GPIOINT_TypeDef *) LPC_GPIOINT_BASE ) 01050 #define LPC_PINCON ((LPC_PINCON_TypeDef *) LPC_PINCON_BASE ) 01051 #define LPC_SSP0 ((LPC_SSP_TypeDef *) LPC_SSP0_BASE ) 01052 #define LPC_SSP1 ((LPC_SSP_TypeDef *) LPC_SSP1_BASE ) 01053 #define LPC_ADC ((LPC_ADC_TypeDef *) LPC_ADC_BASE ) 01054 #define LPC_DAC ((LPC_DAC_TypeDef *) LPC_DAC_BASE ) 01055 #define LPC_CANAF_RAM ((LPC_CANAF_RAM_TypeDef *) LPC_CANAF_RAM_BASE) 01056 #define LPC_CANAF ((LPC_CANAF_TypeDef *) LPC_CANAF_BASE ) 01057 #define LPC_CANCR ((LPC_CANCR_TypeDef *) LPC_CANCR_BASE ) 01058 #define LPC_CAN1 ((LPC_CAN_TypeDef *) LPC_CAN1_BASE ) 01059 #define LPC_CAN2 ((LPC_CAN_TypeDef *) LPC_CAN2_BASE ) 01060 #define LPC_MCPWM ((LPC_MCPWM_TypeDef *) LPC_MCPWM_BASE ) 01061 #define LPC_QEI ((LPC_QEI_TypeDef *) LPC_QEI_BASE ) 01062 #define LPC_EMAC ((LPC_EMAC_TypeDef *) LPC_EMAC_BASE ) 01063 #define LPC_GPDMA ((LPC_GPDMA_TypeDef *) LPC_GPDMA_BASE ) 01064 #define DMAREQSEL (*(__IO uint32_t *) ( 0x4000C1C4)) 01065 #define LPC_GPDMACH0 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH0_BASE ) 01066 #define LPC_GPDMACH1 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH1_BASE ) 01067 #define LPC_GPDMACH2 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH2_BASE ) 01068 #define LPC_GPDMACH3 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH3_BASE ) 01069 #define LPC_GPDMACH4 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH4_BASE ) 01070 #define LPC_GPDMACH5 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH5_BASE ) 01071 #define LPC_GPDMACH6 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH6_BASE ) 01072 #define LPC_GPDMACH7 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH7_BASE ) 01073 #define LPC_USB ((LPC_USB_TypeDef *) LPC_USB_BASE ) 01074 01075 /** 01076 * @} 01077 */ 01078 01079 #endif // __LPC17xx_H__
Generated on Wed Jul 13 2022 21:13:12 by
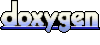