Texas Instruments INA230 hi/lo side, bi-directional current and voltage monitor with I2C. Code hacked and enhanced from the INA219 driver from TI
Fork of INA219 by
INA230.h
00001 /* 00002 * mbed library program 00003 * INA230 High/Low-Side Measurement,Bi-Directional CURRENT/POWER MONITOR with I2C Interface 00004 * by Texas Instruments 00005 * 00006 * Kevin Braun hack of INA219 code by TI 00007 * 23-MAR-2017 00008 */ 00009 00010 #ifndef MBED_INA230 00011 #define MBED_INA230 00012 00013 // Set data into "addr" 00014 #define INA230_ADDR_GG 0x80 00015 #define INA230_ADDR_GV 0x82 00016 #define INA230_ADDR_GA 0x84 00017 #define INA230_ADDR_GL 0x86 00018 #define INA230_ADDR_VG 0x88 00019 #define INA230_ADDR_VV 0x8a 00020 #define INA230_ADDR_VA 0x8c 00021 #define INA230_ADDR_VL 0x8e 00022 #define INA230_ADDR_AG 0x90 00023 #define INA230_ADDR_AV 0x92 00024 #define INA230_ADDR_AA 0x94 00025 #define INA230_ADDR_AL 0x96 00026 #define INA230_ADDR_LG 0x98 00027 #define INA230_ADDR_LV 0x9a 00028 #define INA230_ADDR_LA 0x9c 00029 #define INA230_ADDR_LL 0x9e 00030 00031 // INA230 ID 00032 #define INA_219_DIE 0x4000 00033 #define INA_230_DIE 0x2260 00034 00035 // INA230 register set 00036 #define INA230_CONFIG 0x00 00037 #define INA230_SHUNT_V 0x01 00038 #define INA230_BUS_VOLT 0x02 00039 #define INA230_POWER 0x03 00040 #define INA230_CURRENT 0x04 00041 #define INA230_CALIB 0x05 00042 #define INA230_MASK_ENABLE 0x06 00043 #define INA230_ALERT_LIMIT 0x07 00044 #define INA230_DIE_ID 0xff 00045 00046 // CONFIG regisrer bits 00047 00048 //Number of Averages in 230 CONFIG register 00049 #define INA230_AVG_1 0x0000 00050 #define INA230_AVG_4 0x0200 00051 #define INA230_AVG_16 0x0400 00052 #define INA230_AVG_64 0x0600 00053 #define INA230_AVG_128 0x0800 00054 #define INA230_AVG_256 0x0a00 00055 #define INA230_AVG_512 0x0c00 00056 #define INA230_AVG_1024 0x0e00 00057 00058 //Bus Voltage Conv Time in 230 CONFIG REG 00059 #define INA230_BUS_CT_140u 0x0000 00060 #define INA230_BUS_CT_204u 0x0040 00061 #define INA230_BUS_CT_332u 0x0080 00062 #define INA230_BUS_CT_588u 0x00c0 00063 #define INA230_BUS_CT_1m100 0x0100 00064 #define INA230_BUS_CT_2m116 0x0140 00065 #define INA230_BUS_CT_4m156 0x0180 00066 #define INA230_BUS_CT_8m244 0x01c0 00067 00068 //Shunt Voltage Conv Time in 230 CONFIG REG 00069 #define INA230_SHUNT_CT_140u 0x0000 00070 #define INA230_SHUNT_CT_204u 0x0008 00071 #define INA230_SHUNT_CT_332u 0x0010 00072 #define INA230_SHUNT_CT_588u 0x0018 00073 #define INA230_SHUNT_CT_1m100 0x0020 00074 #define INA230_SHUNT_CT_2m116 0x0028 00075 #define INA230_SHUNT_CT_4m156 0x0030 00076 #define INA230_SHUNT_CT_8m244 0x0038 00077 00078 // Set data into "mode" 00079 #define INA230_PAR_M_PDWN 0 00080 #define INA230_PAR_M_SHNT_TRG 1 00081 #define INA230_PAR_M_BUS_TRG 2 00082 #define INA230_PAR_M_SHNTBUS_TRG 3 00083 #define INA230_PAR_M_ADC_OFF 4 00084 #define INA230_PAR_M_SHNT_CONT 5 00085 #define INA230_PAR_M_BUS_CONT 6 00086 #define INA230_PAR_M_SHNTBUS_CONT 7 // Default 00087 00088 // Mask/Enable and Alert/Limit regisrer bits 00089 #define INA230_MEAL_SOL 0x8000 00090 #define INA230_MEAL_SUL 0x4000 00091 #define INA230_MEAL_BOL 0x2000 00092 #define INA230_MEAL_BUL 0x1000 00093 #define INA230_MEAL_POL 0x0800 00094 #define INA230_MEAL_CNVR 0x0400 00095 00096 #define INA230_MEAL_AFF 0x0010 00097 #define INA230_MEAL_CVRF 0x0008 00098 #define INA230_MEAL_OVF 0x0004 00099 #define INA230_MEAL_APOL 0x0002 00100 #define INA230_MEAL_LEN 0x0001 00101 00102 // Set data into "shunt_register" 00103 #define INA230_PAR_R_005MOHM 5 00104 #define INA230_PAR_R_010MOHM 10 00105 #define INA230_PAR_R_020MOHM 20 00106 #define INA230_PAR_R_025MOHM 25 00107 #define INA230_PAR_R_033MOHM 33 00108 #define INA230_PAR_R_050MOHM 50 00109 #define INA230_PAR_R_068MOHM 68 00110 #define INA230_PAR_R_075MOHM 75 00111 #define INA230_PAR_R_100MOHM 100 00112 00113 00114 /** 00115 * Private data structure for INA230 data values. 00116 * 00117 **/ 00118 typedef struct { 00119 // I2C Address 00120 uint8_t addr ; /*!< I2C address*/ 00121 //Alternate CONFIG 00122 uint16_t average ; /*!< CONFIG Reg - Averaging bits 11-9*/ 00123 uint16_t bus_ct ; /*!< CONFIG Reg - Bus CT bits 8-6 */ 00124 uint16_t shunt_ct ; /*!< CONFIG Reg - Shunt CT bits 5-3*/ 00125 uint16_t mode ; /*!< CONFIG Reg - Mode bits 2-0*/ 00126 // CALBLATION REG 00127 uint16_t cal_data ; /*!< CALIB Reg value*/ 00128 //DIE ID REG 00129 uint16_t die_id_data ; /*!< Device ID - s/b 0x2260*/ 00130 int16_t shunt_res ; /*!< Shunt Resistor value * 100, 100 = 0.100 ohm*/ 00131 } INA230_TypeDef; 00132 00133 /** 00134 * Default values for data structure above 00135 * 00136 **/ 00137 const INA230_TypeDef ina230_std_paramtr = { 00138 // I2C Address 00139 INA230_ADDR_GG, 00140 // CONFIG Register 00141 INA230_AVG_16, // averages 00142 INA230_BUS_CT_588u, // bus voltage conv time 00143 INA230_SHUNT_CT_588u, // bus voltage conv time 00144 INA230_PAR_M_SHNTBUS_CONT, // Measure continuously both Shunt voltage and Bus voltage 00145 // Calibration Register 00146 16384, // Calibration data 00147 //Die ID Register 00148 0, // should be non-zero if read correctly 00149 // Shuny Resistor 00150 INA230_PAR_R_075MOHM // shunt resistor value 00151 }; 00152 00153 /** INA230 High/Low-Side Measurement,Bi-Directional CURRENT/POWER MONITOR with I2C Interface 00154 * 00155 * @code 00156 * // 00157 * // to date, only tested with... 00158 * // * 0.050 shunt resistor 00159 * // * I2C address 0x80 (1000000xb) 00160 * // * +-0-1.5A range 00161 * // * 0-2.8V range 00162 * // 00163 * @endcode 00164 */ 00165 00166 class INA230 00167 { 00168 public: 00169 /** Configure data pin 00170 * @param data SDA and SCL pins 00171 * @param parameter address chip (INA230_TypeDef) 00172 * @param or just set address or just port 00173 */ 00174 INA230(PinName p_sda, PinName p_scl, const INA230_TypeDef *ina230_parameter); 00175 INA230(PinName p_sda, PinName p_scl, uint8_t addr); 00176 INA230(PinName p_sda, PinName p_scl); 00177 00178 /** Configure data pin (with other devices on I2C line) 00179 * @param I2C previous definition 00180 * @param parameter address chip (INA230_TypeDef) 00181 * @param or just set address or just port 00182 */ 00183 INA230(I2C& p_i2c, const INA230_TypeDef *ina230_parameter); 00184 INA230(I2C& p_i2c, uint8_t addr); 00185 INA230(I2C& p_i2c); 00186 00187 /** Read Current data 00188 * @param none 00189 * @return current [mA] 00190 */ 00191 float read_current(void); 00192 int16_t read_current_reg(void); 00193 float read_current_by_shuntvolt(void); 00194 00195 /** Read Power data 00196 * @param none 00197 * @return power [w] 00198 */ 00199 float read_power(void); 00200 00201 /** Read Bus voltage 00202 * @param none 00203 * @return voltage [v] 00204 */ 00205 float read_bus_voltage(void); 00206 00207 /** Read Shunt voltage data 00208 * @param none 00209 * @return voltage [v] 00210 */ 00211 float read_shunt_voltage(void); 00212 00213 /** Read configration reg. 00214 * @param none 00215 * @return configrartion register value 00216 */ 00217 uint16_t read_config(void); 00218 00219 /** Set configration reg. 00220 * @param 00221 * @return configrartion register value 00222 */ 00223 uint16_t set_config(uint16_t cfg); 00224 00225 /** Read calibration reg. 00226 * @param none 00227 * @return calibration register value 00228 */ 00229 uint16_t read_calb(void); 00230 00231 /** Set calibration reg. 00232 * @param 00233 * @return calibration register value 00234 */ 00235 uint16_t set_calb(uint16_t clb); 00236 00237 /** Set I2C clock frequency 00238 * @param freq. 00239 * @return none 00240 */ 00241 void frequency(int hz); 00242 00243 /** Read register (general purpose) 00244 * @param register's address 00245 * @return register data 00246 */ 00247 uint8_t read_reg(uint8_t addr); 00248 00249 /** Write register (general purpose) 00250 * @param register's address 00251 * @param data 00252 * @return register data 00253 */ 00254 uint8_t write_reg(uint8_t addr, uint8_t data); 00255 /* 00256 / ** Temporary display of data structure for debug 00257 * - Needs "Rawserial pc;" statement 00258 * @param none 00259 * @return none 00260 * / 00261 void dumpStructure(); 00262 */ 00263 /** Get the Die ID value 00264 * @param none 00265 * @return register data 00266 */ 00267 uint16_t read_die_id(); 00268 00269 /** Get the Mask/Enable value 00270 * @param none 00271 * @return register data 00272 */ 00273 uint16_t read_mask_enable(); 00274 00275 /** Get the Alert/Limit value 00276 * @param none 00277 * @return register data 00278 */ 00279 uint16_t read_alert_limit(); 00280 00281 /** Set the Mask/Enable value 00282 * @param data for register 00283 * @return data sent 00284 */ 00285 uint16_t set_mask_enable(uint16_t cfg); 00286 00287 /** Set the Alert/Limit value 00288 * @param data for register 00289 * @return data sent 00290 */ 00291 uint16_t set_alert_limit(uint16_t cfg); 00292 00293 /** Get the Shunt Resistor value 00294 * @param none 00295 * @return resistor value * 100 00296 */ 00297 int16_t get_shunt_res(); 00298 00299 protected: 00300 I2C _i2c; 00301 00302 void initialize(void); 00303 00304 private: 00305 INA230_TypeDef ina230_set_data; 00306 int32_t scale_factor; 00307 uint8_t dt[4]; 00308 00309 }; 00310 00311 #endif // MBED_INA230
Generated on Thu Jul 14 2022 06:57:18 by
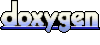