Implementation of the WifiPlusClick hardware module.
Dependents: WifiPlusKlickExample
DnsQuery Class Reference
Class DnsQuery implements DNS over UDP functionality. More...
#include <DnsQuery.h>
Public Member Functions | |
DnsQuery (Wifi *wifi, IPADDRESS_t *dnsip) | |
Constructor to instantiate a DnsQuery object. | |
bool | gethostbyname (const char *hostname, IPADDRESS_t &ipaddress) |
Function gethostbyname implements the functionality to query a domain name server for an IP-Address of a given hostname. |
Detailed Description
Class DnsQuery implements DNS over UDP functionality.
Example as a typical use case :
#include "mbed.h" #include "DnsQuery.h" void main(void) { IPADDRESS_t ipAddress; // will receive the ip address of the host IPADDRESS_t dnsIp = { 192, 168, 178, 1 }; // Ip Address of the DNS server DnsQuery dns(Wifi::getInstance(), &dnsIp); if (dns.gethostbyname("mbed.org", ipAddress)) { printf("Ip-Address of mbed.org is %d.%d.%d.%d\n", ipAddress.sin_addr.o1, ipAddress.sin_addr.o2, ipAddress.sin_addr.o3, ipAddress.sin_addr.o4); } else { printf("Unable to obtain IP-Address\n"); } }
Definition at line 44 of file DnsQuery.h.
Constructor & Destructor Documentation
Constructor to instantiate a DnsQuery object.
- Parameters:
-
wifi : A valid pointer to a Wifi Object, which can be used to obtain a UDP socket object. dnsip : A valid pointer which holds the IPAddress of the DNS server to query.
Definition at line 26 of file DnsQuery.cpp.
Member Function Documentation
bool gethostbyname | ( | const char * | hostname, |
IPADDRESS_t & | ipaddress | ||
) |
Function gethostbyname implements the functionality to query a domain name server for an IP-Address of a given hostname.
- Parameters:
-
hostname : the hostname of interest as a string. ipaddress : a reference to a IPADDRESS_t object which will receive the resolved IP Address of the host in question.
- Returns:
- true if successfull, or false otherwise.
Definition at line 37 of file DnsQuery.cpp.
Generated on Tue Jul 12 2022 23:18:35 by
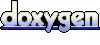