Implementation of the WifiPlusClick hardware module.
Dependents: WifiPlusKlickExample
Wifi Class Reference
Class Wifi encapsulates communication and access to Wifi Plus Click module. More...
#include <Wifi.h>
Inherited by WifiPlusClick.
Public Member Functions | |
bool | ResetMsg () |
Function to reset the Wifi Plus Click Device. | |
bool | GetVersionMsg (char &sw_ver_lo, char &sw_ver_hi, char &rad_ver_lo, char &rad_ver_hi) |
Function retrieves the software and radio version of the Wifi Plus Click Board. | |
bool | GpioMsg (GPIO_IDX_t pin, GPIO_OP_t operation, GPIO_RES_t &result) |
Function will set a GPIO pin to a specific output level or will set it to input. | |
bool | SetIpAddress (bool bUseDHCP, IPADDRESS_t *IPAddress) |
Function to set the IP address of the Wifi Plus Click device. | |
bool | SetSubnetMask (IPADDRESS_t *NetworkMask) |
Function to set the network mask of the Wifi Plus Click device. | |
bool | SetGatewayIpAddress (IPADDRESS_t *IPAddress) |
Function to set the gateway ip address to use. | |
bool | GetNetworkStatus (char *MacAddress, IPADDRESS_t *IPAddress, IPADDRESS_t *NetworkMask, IPADDRESS_t *GatewayAddress, NET_STAT_t &stat) |
Function to retrieve the current network status of the Wifi Plus Click device. | |
bool | SetMACAddress (char MacAddress[6]) |
Function to set the MAC Address of the device. | |
bool | SetARPTime (unsigned short ARPTime) |
Function to set the address resolution time from 1 to 65535 seconds. | |
bool | SetNetworkMode (char Profile, NETW_MODE_t NetMode) |
Function to set the network mode for one of two available profiles. | |
bool | SetSSID (char Profile, const char *ssid) |
Function to set the SSID for one of two profiles. | |
bool | SetPowerSaveMode (POWERSAVE_MODE_t pwrsave, short DTIM_Listen) |
Function switches device into one of 3 power saving modes, or sets it into awake mode. | |
bool | SetRegionalDomain (DOMAIN_COUNTRY_CODE_t dcode) |
Function to set the regional domain for all CPs. | |
bool | SetChannelList (char numListItems, char ListItems[]) |
Function to set the channels on which to scan. | |
bool | SetRetryCount (char infrastructureRetryCount=255, char adhocRetryCount=5) |
Function to specify the connection managaer retry count. | |
bool | SetSecurityOpen (char Profile) |
Function to set the Security mode to OPN. | |
bool | SetSecurityWEP40 (char Profile, bool bSharedKey, char DefaultWEPKeyIdx, char SecurityKeys[20]) |
Function to set the WEP40 security mode. | |
bool | SetSecurityWEP104 (char Profile, bool bSharedKey, char DefaultWEPKeyIdx, char SecurityKeys[52]) |
Function to set the WEP104 security mode. | |
bool | SetSecurityWPA (char Profile, WPA_SECURITY_t sec, int len, const char *secKeyOrPSK) |
Function to set the WPA security mode. | |
bool | GetWPAKey (char Profile, char Key[32]) |
Function to retrieve the WPA Key from the Wifi Plus Click device for a selected profile. | |
bool | ScanStartMsg (char Profile) |
Function to initiate the scan on one or both Profiles using the settings downloaded to the Wifi Plus Click. | |
bool | ScanGetResults (char index, char ssid[32], AP_CONFIG_t &apCfg, short &beaconInterval, short &ATIMWindow, char &RSSI, NETW_MODE_t &bssType, char &channelNo) |
Function to retrieve a specific scan result. | |
bool | Connect (char Profile) |
Function to connect using the specified profile and the settings previously downloaded to the device. | |
bool | Disconnect () |
Function to disconnect from any connected AP. | |
void | Poll () |
This is the main cyclic function, which needs to be called as often as possible. | |
Socket Functions | |
In the following section, all socket relevant functions are contained. | |
bool | SocketAllocate (char nTCPSvr, char nTCPClnt, unsigned short TCPSvrRxBuf, unsigned short TCPSvrTxBuf, unsigned short TCPClntRxBuf, unsigned short TCPClntTxBuf) |
Function to allocate the internal memory of the device and the number of sockets (which in total is limited to 8). | |
SOCKET_HANDLE_t | SocketCreate (SOCKET_TYPE_t sockType) |
Function to create a new socket object of the given type. | |
bool | SocketClose (SOCKET_HANDLE_t hSock) |
Function to close a previously created socket. | |
bool | SocketBind (SOCKET_HANDLE_t hSock, int Port) |
Function to bind to a specific port using the given socket. | |
bool | SocketListen (SOCKET_HANDLE_t hSock, int &Backlog) |
Function to prepare the a number of sockets for accepting remote connections. | |
bool | SocketAccept (SOCKET_HANDLE_t hSock, SOCKET_HANDLE_t &client, int &remotePort, IPADDRESS_t &remoteAddress) |
Function to accept a new remote connection. | |
SOCKET_HANDLE_t | SocketConnect (SOCKET_HANDLE_t hSock, IPADDRESS_t *IpAddress, int Port) |
Function to connect the socket to a remote socket specified by IpAddress and Port. | |
int | SocketSend (SOCKET_HANDLE_t hSock, char *data, int length) |
Function to send data on the connected socket. | |
int | SocketRecv (SOCKET_HANDLE_t hSock, char *data, int length) |
Function to receive data from the connected socket. | |
int | SocketSendTo (SOCKET_HANDLE_t hSock, IPADDRESS_t *remoteAddress, int remotePort, char *data, int length) |
Function to send data over a connectionless socket. | |
int | SocketRecvFrom (SOCKET_HANDLE_t hSock, IPADDRESS_t *remoteAddress, int *remotePort, char *data, int length) |
Function to receive data over a connectionless socket. |
Detailed Description
Class Wifi encapsulates communication and access to Wifi Plus Click module.
{ Henry Leinen }
Definition at line 245 of file Wifi.h.
Member Function Documentation
bool Connect | ( | char | Profile ) |
Function to connect using the specified profile and the settings previously downloaded to the device.
Please Note that the connection state will be transmitted asynchroneously be the device. You need to monitor the connected state.
- Parameters:
-
Profile : can be profile number one or two.
- Returns:
- : true if successfully submitted, or false otherwise.
bool Disconnect | ( | ) |
bool GetNetworkStatus | ( | char * | MacAddress, |
IPADDRESS_t * | IPAddress, | ||
IPADDRESS_t * | NetworkMask, | ||
IPADDRESS_t * | GatewayAddress, | ||
NET_STAT_t & | stat | ||
) |
Function to retrieve the current network status of the Wifi Plus Click device.
- Parameters:
-
MacAddress : pointer to an array of 6 Bytes to retrieve the MAC Address. Set to NULL if not required. IPAddress : pointer to an IPADDRESS_t structure which receives the IP address. Set to NULL if not required. NetworkMask : pointer to an IPADDRESS_t structure which receives the network mask. Set to NULL if not requried. GatewayAddress : pointer to an IPADDRESS_t structure which receives the gateway address. Set to NULL if no required. stat : will receive the actual status.
- Returns:
- : true if successfull, or false otherwise.
bool GetVersionMsg | ( | char & | sw_ver_lo, |
char & | sw_ver_hi, | ||
char & | rad_ver_lo, | ||
char & | rad_ver_hi | ||
) |
Function retrieves the software and radio version of the Wifi Plus Click Board.
- Parameters:
-
sw_ver_lo : receives the low WiComm Socket Version number sw_ver_hi : recevies the high WiComm Socket Version number rad_ver_lo,: receives the low Radio version number rad_ver_hi,: receives the high Radio version number
- Returns:
- true if successful or false otherwise
bool GetWPAKey | ( | char | Profile, |
char | Key[32] | ||
) |
bool GpioMsg | ( | GPIO_IDX_t | pin, |
GPIO_OP_t | operation, | ||
GPIO_RES_t & | result | ||
) |
Function will set a GPIO pin to a specific output level or will set it to input.
The function will return the result of the operation.
- Parameters:
-
pin : Reference to the GPIO pin of interest operation,: the operation to perform on the GPIO pin. Set to specific output level or read as input result : a reference to a variable which receives the actual status of the GPIO pin
void Poll | ( | ) |
This is the main cyclic function, which needs to be called as often as possible.
The function will check if a new message has been received and will decode and dispatch it.
bool ResetMsg | ( | ) |
bool ScanGetResults | ( | char | index, |
char | ssid[32], | ||
AP_CONFIG_t & | apCfg, | ||
short & | beaconInterval, | ||
short & | ATIMWindow, | ||
char & | RSSI, | ||
NETW_MODE_t & | bssType, | ||
char & | channelNo | ||
) |
Function to retrieve a specific scan result.
Will only work after scan has been started and finished.
- Parameters:
-
index : specify the result item to return. When this index is too high the function returns with a false return value. ssid : will receive the SSID of the item. apCfg : will receive the access points configuration. beaconInterval : will receive the beacon interval time. ATIMWindow : will receive the ATIM Window. RSSI : will receive the RSSI level. bssType : will receive information about the BSS type (INFRASTRUCTURE oR ADHOC). channelNo : will receive on which channel the AP transmits.
- Returns:
- : true if successfull, false otherwise.
bool ScanStartMsg | ( | char | Profile ) |
Function to initiate the scan on one or both Profiles using the settings downloaded to the Wifi Plus Click.
Please NOTE that the device will only accept the REBOOT message while a scan is active. A scan can only be started if the device is not connected.
- Parameters:
-
Profile : can be profile number one or two.
- Returns:
- : true if successfull, or false otherwise.
bool SetARPTime | ( | unsigned short | ARPTime ) |
bool SetChannelList | ( | char | numListItems, |
char | ListItems[] | ||
) |
Function to set the channels on which to scan.
- Parameters:
-
numListItems : the number of channels given in the list. ListItems : array consisting of numListItems channel numbers.
- Returns:
- : true if successfull, or false otherwise
bool SetGatewayIpAddress | ( | IPADDRESS_t * | IPAddress ) |
Function to set the gateway ip address to use.
If the gateway ip address is set to 0.0.0.0 the Wifi Plus Click will not use a gateway.
- Parameters:
-
IPAddress : Pointer to an IPADDRESS specifying the IP Address of the gateway to use.
- Returns:
- : true if successfull, or false otherwise.
bool SetIpAddress | ( | bool | bUseDHCP, |
IPADDRESS_t * | IPAddress | ||
) |
Function to set the IP address of the Wifi Plus Click device.
- Parameters:
-
bUseDHCP : set to true if DHCP shall be used, or set to false if you want to specify a static IP address IPAddress,: pointer to an IP address. Please note that the IP adress will only be used if bUseDHCP is set to false
- Returns:
- : true if successfull, or false otherwise. The function will not check for any asynchroneous result.
bool SetMACAddress | ( | char | MacAddress[6] ) |
bool SetNetworkMode | ( | char | Profile, |
NETW_MODE_t | NetMode | ||
) |
bool SetPowerSaveMode | ( | POWERSAVE_MODE_t | pwrsave, |
short | DTIM_Listen | ||
) |
Function switches device into one of 3 power saving modes, or sets it into awake mode.
- Parameters:
-
pwrsave : specifies which powersaving mode to set. DTIM_Listen : if pwrsave is in mode PSPOLL_SELF_DTIM uses this value as the DTIM listen interval. Value is given in units of 100ms.
- Returns:
- : true if successfull, or false otherwise
bool SetRegionalDomain | ( | DOMAIN_COUNTRY_CODE_t | dcode ) |
bool SetRetryCount | ( | char | infrastructureRetryCount = 255 , |
char | adhocRetryCount = 5 |
||
) |
Function to specify the connection managaer retry count.
There are separate values for infrastructure and ad hoc mode. Please note that a number of 0 means no retries and 255 means retry forever.
- Parameters:
-
infrastructureRetryCount : number of retries in infrastructure mode adhocRetryCount : number of retries in ad-hoc mode.
- Returns:
- : true if successfull, or false otherwise
bool SetSecurityOpen | ( | char | Profile ) |
bool SetSecurityWEP104 | ( | char | Profile, |
bool | bSharedKey, | ||
char | DefaultWEPKeyIdx, | ||
char | SecurityKeys[52] | ||
) |
Function to set the WEP104 security mode.
- Parameters:
-
Profile : can be profile number one or two. bShareKey : set to true for shared key, or false for open key DefaultWEPKeyIdx : the index (0-3) into the key array SecurityKeys : array or 4 security keys with 13 bytes each
- Returns:
- : true if successfull, or false otherwise
bool SetSecurityWEP40 | ( | char | Profile, |
bool | bSharedKey, | ||
char | DefaultWEPKeyIdx, | ||
char | SecurityKeys[20] | ||
) |
Function to set the WEP40 security mode.
- Parameters:
-
Profile : can be profile number one or two. bShareKey : set to true for shared key, or false for open key DefaultWEPKeyIdx : the index (0-3) into the key array SecurityKeys : array or 4 security keys with 5 bytes each
- Returns:
- : true if successfull, or false otherwise
bool SetSecurityWPA | ( | char | Profile, |
WPA_SECURITY_t | sec, | ||
int | len, | ||
const char * | secKeyOrPSK | ||
) |
Function to set the WPA security mode.
- Parameters:
-
Profile : can be profile number one or two. sec : can be one of the define security types. len : length of the security key or passphrase. secKeyOrPSK : security key or passphrase.
- Returns:
- : true if successfull, or false otherwise.
bool SetSSID | ( | char | Profile, |
const char * | ssid | ||
) |
bool SetSubnetMask | ( | IPADDRESS_t * | NetworkMask ) |
bool SocketAccept | ( | SOCKET_HANDLE_t | hSock, |
SOCKET_HANDLE_t & | client, | ||
int & | remotePort, | ||
IPADDRESS_t & | remoteAddress | ||
) |
Function to accept a new remote connection.
Will work asynchroneously, means if no new connection was accepted, will return immediately.
- Parameters:
-
hSock : Valid socket handle. client : reference to a variable which receives the new socket handle of the connection socket or InvalidSocketHandle if no connection could be made at this time. remotePort : receives the remote port of the remote connection. remoteAddress : receives the remote address of the remote connection.
- Returns:
- : true if successfull, or false otherwise. PLEASE NOTE that even if no connection was made, the return result may be true.
bool SocketAllocate | ( | char | nTCPSvr, |
char | nTCPClnt, | ||
unsigned short | TCPSvrRxBuf, | ||
unsigned short | TCPSvrTxBuf, | ||
unsigned short | TCPClntRxBuf, | ||
unsigned short | TCPClntTxBuf | ||
) |
Function to allocate the internal memory of the device and the number of sockets (which in total is limited to 8).
- Parameters:
-
nTCPSvr : Number of server sockets to allocate. nTCPClnt,: Number of client sockets to allocate. TCPSvrRxBuf : Size (in bytes) to allocate for the receive buffer for each server socket. TCPSvrTxBuf : Size (in bytes) to allocate for the transmit buffer for each server socket. TCPClntRxBuf,: Size (in bytes) to allocate for the receive buffer for each client socket. TCPClntTxBuf,: Size (in bytes) to allocate for the transmit buffer for each client socket.
- Returns:
- : true if successful, or false otherwise. Can return false if the requestet internal memory exceededs the total of 8192 internal byte orif the maximum number of 8 sockets is exceeded.
bool SocketBind | ( | SOCKET_HANDLE_t | hSock, |
int | Port | ||
) |
bool SocketClose | ( | SOCKET_HANDLE_t | hSock ) |
SOCKET_HANDLE_t SocketConnect | ( | SOCKET_HANDLE_t | hSock, |
IPADDRESS_t * | IpAddress, | ||
int | Port | ||
) |
Function to connect the socket to a remote socket specified by IpAddress and Port.
- Parameters:
-
hSock : Valid socket handle. IpAddress : valid IP Address of the remote host to connect with. Port : Port of the remote host to connect with.
- Returns:
- : true if a connection has been made successfully or false otherwise.
SOCKET_HANDLE_t SocketCreate | ( | SOCKET_TYPE_t | sockType ) |
bool SocketListen | ( | SOCKET_HANDLE_t | hSock, |
int & | Backlog | ||
) |
Function to prepare the a number of sockets for accepting remote connections.
- Parameters:
-
hSock : Valid socket handle. Backlog : Number of sockets to set in listening mode, will receive the number of new unassigned backlog count
- Returns:
- : true if successfull, or false otherwise.
int SocketRecv | ( | SOCKET_HANDLE_t | hSock, |
char * | data, | ||
int | length | ||
) |
Function to receive data from the connected socket.
- Parameters:
-
hSock : Valid socket handle of a socket which is already connected. data : Pointer to a valid buffer which shall receive the data. length : Maximum number of bytes which can be received and stored in the provided buffer.
- Returns:
- : Number of bytes actually received over the connection or -1 if a fault occured.
int SocketRecvFrom | ( | SOCKET_HANDLE_t | hSock, |
IPADDRESS_t * | remoteAddress, | ||
int * | remotePort, | ||
char * | data, | ||
int | length | ||
) |
Function to receive data over a connectionless socket.
- Parameters:
-
hSock : Valid socket handle. remoteAddress : The ip address of the remote origin from where to receive the data. remotePort : Point to receive the port of the remote origin from where to receive the data or NULL if not needed. data : Pointer to a buffer which will receive the data. length : Number of bytes to receive and which fit into the provided buffer.
- Returns:
- : Number of bytes actually received or -1 if a fault occured.
int SocketSend | ( | SOCKET_HANDLE_t | hSock, |
char * | data, | ||
int | length | ||
) |
int SocketSendTo | ( | SOCKET_HANDLE_t | hSock, |
IPADDRESS_t * | remoteAddress, | ||
int | remotePort, | ||
char * | data, | ||
int | length | ||
) |
Function to send data over a connectionless socket.
- Parameters:
-
hSock : Valid socket handle. remoteAddress : The ip address of the remote destination to send the data to. remotePort : The port of the remote destination to send the data to. data : Pointer to a buffer which contains the data to send. length : The number of bytes to sent to the specified address.
- Returns:
- : Number of bytes actually sent or -1 if a fault occured.
Generated on Tue Jul 12 2022 23:18:35 by
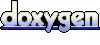