
Georgia Tech ECE 4180 Final Project
Dependencies: Camera_LS_Y201 FatFileSystem MODSERIAL SDFileSystem SHTx Servo TextLCD mbed
project_defines.hpp
00001 #include "mbed.h" 00002 #include "MODSERIAL.h" 00003 #include "Camera_LS_Y201.h" 00004 #include "SDFileSystem.h" 00005 #include "SHTx/sht15.hpp" 00006 #include "Servo.h" 00007 #include "TextLCD.h" 00008 00009 #define IR_THRESHOLD 0.009f 00010 00011 #define DEBMSG printf 00012 #define NEWLINE() printf("\r\n") 00013 00014 00015 MODSERIAL sms(p13, p14); 00016 Serial pc(USBTX, USBRX); 00017 Servo myservo(p22); 00018 TextLCD lcd(p15, p16, p17, p18, p19, p21); 00019 AnalogIn ir(p20); 00020 SHTx::SHT15 temp_sensor(p9, p10); 00021 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00022 Camera_LS_Y201 cam1(p28, p27); 00023 DigitalOut light1(p29); 00024 DigitalOut light2(p30); 00025 PwmOut speaker(p23); 00026 00027 typedef struct work { 00028 FILE *fp; 00029 } work_t; 00030 00031 work_t work; 00032 00033 00034 bool movement = false; 00035 float current_avg; 00036 00037 bool activate = true; 00038 00039 float current_temperature; 00040 float target_temperature; 00041 bool heat = true; 00042 00043 int index = 1; 00044 00045 //char* mobile_number = "6784628602"; 00046 //char* mobile_number = "4043887322"; 00047 char* mobile_number = "4045789581"; 00048 00049 00050 DigitalOut led1(LED1); 00051 DigitalOut led2(LED2); 00052 DigitalOut led3(LED3); 00053 DigitalOut led4(LED4); 00054 00055 bool message_received = false; 00056 char message[256]; 00057 char sms_buffer[256]; 00058 int message_count = 0; 00059 00060 void delete_messages() 00061 { 00062 wait(0.25); 00063 sms.printf("AT+CMGF=1%c", 13); 00064 wait(0.25); 00065 sms.printf("AT+CMGD=1,4%c", 13); 00066 while(!sms.txBufferEmpty()) { 00067 } 00068 wait(2); 00069 sms.rxBufferFlush(); 00070 } 00071 00072 void send_sms(char * message) 00073 { 00074 led3 = 1; 00075 pc.printf("Sending SMS\n"); 00076 wait(0.25); 00077 sms.printf("AT+CMGF=1%c", 13); 00078 wait(.25); 00079 sms.printf("AT+CMGS=\"%s\"%c", mobile_number, 13); 00080 wait(0.25); 00081 sms.printf("%s%c", message ,26); 00082 while(!sms.txBufferEmpty()) { 00083 } 00084 wait(0.5); 00085 led3 = 0; 00086 } 00087 00088 void receive_sms() 00089 { 00090 wait(0.25); 00091 sms.printf("AT+CMGF=1%c", 13); 00092 wait(0.25); 00093 //sms.printf("AT+CMGL=\"ALL\"%c", 13); 00094 sms.printf("AT+CMGL=\"REC UNREAD\"%c", 13); 00095 while(!sms.txBufferEmpty()) { 00096 } 00097 } 00098 00099 void get_data(char *buffer) 00100 { 00101 int index = 0; 00102 char c = sms.getc(); 00103 while(c != 10) { 00104 buffer[index++] = c; 00105 c = sms.getc(); 00106 } 00107 buffer[index] = 0; 00108 } 00109 00110 void adjust_temperature(){ 00111 temp_sensor.update(); 00112 current_temperature = temp_sensor.getTemperature(); 00113 if(current_temperature > 85.0f){ 00114 send_sms("High Temperature Alert!!!"); 00115 } 00116 if(!heat){ 00117 temp_sensor.setHeater(false); 00118 return; 00119 } 00120 if(current_temperature < target_temperature){ 00121 temp_sensor.setHeater(true); 00122 } else { 00123 temp_sensor.setHeater(false); 00124 } 00125 } 00126 00127 void read_message() 00128 { 00129 while(sms.readable()) { 00130 get_data(sms_buffer); 00131 if(strstr(sms_buffer, "CMGL") != NULL) { 00132 for(int i = 0; i < 256; i++) message[i] = 0; 00133 get_data(message); 00134 message_received = true; 00135 delete_messages(); 00136 return; 00137 } 00138 sms_buffer[0] = 0; 00139 } 00140 receive_sms(); 00141 } 00142 00143 00144 00145 void setup_devices() 00146 { 00147 sms.baud(115200); 00148 pc.baud(115200); 00149 //pc.printf("\x1B[2J"); 00150 //pc.printf("\x1B[H"); 00151 message_received = false; 00152 led4 = 1; 00153 delete_messages(); 00154 mkdir("/sd/mydir", 0777); 00155 temp_sensor.setScale(true); 00156 current_temperature = temp_sensor.getTemperature(); 00157 target_temperature = current_temperature; 00158 } 00159 00160 bool check_movement() 00161 { 00162 float value = ir; 00163 if (value > (current_avg + IR_THRESHOLD) || value < (current_avg - IR_THRESHOLD)){ 00164 led1 = 1; 00165 printf("MOVEMENT_DETECTED: IR value is %f, Current_avg: %f\r\n",value, current_avg); 00166 return true; 00167 } else { 00168 led1 = 0; 00169 //printf("IR value is %f, Current_avg: %f\r\n", value, current_avg); 00170 return false; 00171 } 00172 } 00173 00174 void calibrate_ir() 00175 { 00176 float current_sum = 0.0f; 00177 for(int i = 0; i < 10000; i++) { 00178 current_sum = current_sum + ir; 00179 } 00180 current_avg = current_sum / 10000.0f; 00181 } 00182 00183 /** 00184 * Callback function for readJpegFileContent. 00185 * 00186 * @param buf A pointer to a buffer. 00187 * @param siz A size of the buffer. 00188 */ 00189 void callback_func(int done, int total, uint8_t *buf, size_t siz) 00190 { 00191 fwrite(buf, siz, 1, work.fp); 00192 00193 static int n = 0; 00194 int tmp = done * 100 / total; 00195 if (n != tmp) { 00196 n = tmp; 00197 DEBMSG("Writing...: %3d%%", n); 00198 NEWLINE(); 00199 } 00200 } 00201 00202 00203 /** 00204 * Capture. 00205 * 00206 * @param cam A pointer to a camera object. 00207 * @param filename The file name. 00208 * 00209 * @return Return 0 if it succeed. 00210 */ 00211 int capture(Camera_LS_Y201 *cam, char *filename) 00212 { 00213 /* 00214 * Take a picture. 00215 */ 00216 if (cam->takePicture() != 0) { 00217 return -1; 00218 } 00219 DEBMSG("Captured."); 00220 NEWLINE(); 00221 00222 /* 00223 * Open file. 00224 */ 00225 work.fp = fopen(filename, "wb"); 00226 if (work.fp == NULL) { 00227 return -2; 00228 } 00229 00230 /* 00231 * Read the content. 00232 */ 00233 DEBMSG("%s", filename); 00234 NEWLINE(); 00235 if (cam->readJpegFileContent(callback_func) != 0) { 00236 fclose(work.fp); 00237 return -3; 00238 } 00239 fclose(work.fp); 00240 00241 /* 00242 * Stop taking pictures. 00243 */ 00244 cam->stopTakingPictures(); 00245 00246 return 0; 00247 } 00248 00249 void take_picture() 00250 { 00251 00252 myservo.write(0); 00253 int a; 00254 wait(1); 00255 char buffer[25]; 00256 sprintf(buffer, "/sd/mydir/%d_1.jpg", index); 00257 a = capture(&cam1,buffer); 00258 switch (a) { 00259 case -1: 00260 led1=1; 00261 break; 00262 case -2: 00263 //led2=1; 00264 break; 00265 case -3: 00266 led3=1; 00267 break; 00268 case 0: 00269 led4=1; 00270 lcd.printf("Took picture 1!\n"); 00271 break; 00272 } 00273 00274 wait(1); 00275 led1=0; 00276 //led2=0; 00277 led3=0; 00278 led4=0; 00279 00280 myservo.write(0.5); 00281 wait(1); 00282 sprintf(buffer, "/sd/mydir/%d_2.jpg", index); 00283 a= capture(&cam1,buffer); 00284 00285 switch (a) { 00286 case -1: 00287 led1=1; 00288 break; 00289 case -2: 00290 //led2=1; 00291 break; 00292 case -3: 00293 led3=1; 00294 break; 00295 case 0: 00296 led4=1; 00297 lcd.printf("Took picture 2!\n"); 00298 break; 00299 } 00300 00301 wait(1); 00302 led1=0; 00303 //led2=0; 00304 led3=0; 00305 led4=0; 00306 myservo.write(1); 00307 wait(1); 00308 sprintf(buffer, "/sd/mydir/%d_3.jpg", index++); 00309 a= capture(&cam1,buffer); 00310 switch (a) { 00311 case -1: 00312 led1=1; 00313 break; 00314 case -2: 00315 //led2=1; 00316 break; 00317 case -3: 00318 led3=1; 00319 break; 00320 case 0: 00321 led4=1; 00322 lcd.printf("Took picture 3!\n"); 00323 break; 00324 } 00325 00326 wait(1); 00327 led1=0; 00328 //led2=0; 00329 led3=0; 00330 led4=0; 00331 00332 } 00333 00334 00335 void handle_message() 00336 { 00337 int temp; 00338 pc.printf("MESSAGE: %s\n", message); 00339 if(strstr(message, "take picture") != NULL){ 00340 take_picture(); 00341 } else if(strstr(message, "turn off alarm") != NULL){ 00342 speaker = 0; 00343 } else if(strstr(message, "get status") != NULL){ 00344 char msg[100]; 00345 int l1 = light1; 00346 int l2 = light2; 00347 int spk = speaker > 0.0f; 00348 sprintf(msg, "Status: Light1 = %d, Light2 = %d, Temperature = %f, Alarm = %d", l1, l2, temp_sensor.getTemperature(), spk); 00349 send_sms(msg); 00350 pc.printf(msg); 00351 } else if (strstr(message, "deactivate") != NULL){ 00352 speaker = 0; 00353 activate = false; 00354 led2 = 0; 00355 pc.printf("\nIntrusion alert not active\n"); 00356 } else if (strstr(message, "activate") != NULL){ 00357 activate = true; 00358 calibrate_ir(); 00359 light1 = 0; 00360 light2 = 0; 00361 heat = false; 00362 temp_sensor.setHeater(false); 00363 led2 = 1; 00364 pc.printf("\nIntrusion alert active\n"); 00365 } else if (strstr(message, "turn off light 1") != NULL){ 00366 pc.printf("\nTurning off light 1\n"); 00367 light1 = 0; 00368 } else if (strstr(message, "turn off light 2") != NULL){ 00369 pc.printf("\nTurning off light 2\n"); 00370 light2 = 0; 00371 } else if (strstr(message, "turn on light 1") != NULL){ 00372 pc.printf("\nTurning on light 1\n"); 00373 light1 = 1; 00374 } else if (strstr(message, "turn on light 2") != NULL){ 00375 pc.printf("\nTurning on light 2\n"); 00376 light2 = 1; 00377 } else if (strstr(message, "turn off heat") != NULL){ 00378 heat = false; 00379 lcd.printf("Heat off!\n"); 00380 pc.printf("\nTurning off heat\n"); 00381 } else if (strstr(message, "get temperature") != NULL){ 00382 char new_message[30]; 00383 sprintf(new_message, "Current Temperature is %f", temp_sensor.getTemperature()); 00384 send_sms(new_message); 00385 pc.printf("\nSending current temperature %f\n", temp_sensor.getTemperature()); 00386 } else if (sscanf(message, "set temperature %d", &temp) >=1){ 00387 target_temperature = (float)temp; 00388 lcd.cls(); 00389 lcd.printf("Target temp: %d\n", temp); 00390 heat = true; 00391 if(current_temperature < target_temperature){ 00392 lcd.printf("Heater ON\n"); 00393 } else { 00394 lcd.printf("Heater OFF\n"); 00395 } 00396 pc.printf("Setting target temperature to %d\n", temp); 00397 } 00398 message[0] = 0; 00399 message_received = false; 00400 00401 } 00402 00403 void play_sound(){ 00404 for(int i = 0; i < 5000; i++){ 00405 for(float j = 0.0; j <= 1.0; j+=0.1){ 00406 speaker = j; 00407 wait(0.0001); 00408 00409 } 00410 } 00411 //speaker = 0.0; 00412 }
Generated on Sun Jul 17 2022 04:01:01 by
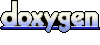