
The program sends the current location over the cellular network.
Dependencies: aconno_I2C ublox-at-cellular-interface gnss ublox-cellular-base Lis2dh12 ublox-cellular-base-n2xx ublox-at-cellular-interface-n2xx low-power-sleep
Fork of example-gnss by
uBloxSara.cpp
00001 /** 00002 * U-Blox SARA nb-iot module class 00003 * Made by Jurica Resetar @ aconno 00004 * More info @ aconno.de 00005 */ 00006 00007 #include "mbed.h" 00008 #include "uBloxSara.h" 00009 #include "udp.h" 00010 #include "onboard_modem_api.h" 00011 00012 UBloxSara::UBloxSara(ATCmdParser *at, Udp udp): 00013 _at(at), _udp(udp) 00014 { 00015 } 00016 00017 bool UBloxSara::setup() 00018 { 00019 bool success = false; 00020 00021 /* Initialize GPIO lines */ 00022 onboard_modem_init(); 00023 00024 /* Give modem a little time to settle down */ 00025 wait_ms(250); 00026 00027 if(!CUSTOM_BOARD) 00028 { 00029 // DO NOT CALL THIS IF YOU WORK ON ACONNO CUSTOM MADE BOARD 00030 // POWER UP PIN (PE_14) IS CONNECTED ON OC DIGITAL OUTPUT 00031 // AND POWER UP PIN IS NOT CONNECTED ON SARA MODULE 00032 onboard_modem_power_up(); 00033 } 00034 00035 wait_ms(5000); 00036 00037 sendCommand("AT+CFUN=1"); 00038 00039 // Set AT parser timeout to 1sec for AT OK check 00040 _at->set_timeout(1000); 00041 00042 return true; 00043 00044 printf("Checking for AT response from the modem\r\n"); 00045 for (int retry_count = 0; !success && (retry_count < 20); retry_count++) 00046 { 00047 printf("...wait\r\n"); 00048 // The modem tends to sends out some garbage during power up. 00049 _at->flush(); 00050 00051 // AT OK talk to the modem 00052 if (_at->send("AT")) { 00053 wait_ms(100); 00054 if (_at->recv("OK")) { 00055 success = true; 00056 } 00057 } 00058 } 00059 // Increase the parser time to 8 sec 00060 _at->set_timeout(8000); 00061 00062 if (success) 00063 { 00064 printf("Configuring the modem...\r\n"); 00065 // Turn off modem echoing and turn on verbose responses 00066 //success = _at->send("AT+CMEE=1"); 00067 } 00068 return success; 00069 } 00070 00071 void UBloxSara::sendCommand(char *command) 00072 { 00073 char buffer[505]; 00074 for (int i=0; i<505; i++)buffer[i]=0; 00075 printf("Sending the following command:"); 00076 printf(command); 00077 printf("\r\n"); 00078 00079 if (!_at->send(command)) { 00080 printf("Failed!\r\n"); 00081 return; 00082 } 00083 printf("Response:\r\n"); 00084 _at->read(buffer,500); 00085 printf(buffer); 00086 printf ("\r\n"); 00087 } 00088 00089 void UBloxSara::checkNetworkStatus(char *response) 00090 { 00091 char command[] = "AT+NUESTATS"; 00092 printf("Checking network status..."); 00093 00094 if(!_at->send(command)) 00095 { 00096 printf("Failed!\r\n"); 00097 return; 00098 } 00099 printf("Response:\r\n"); 00100 _at->read(response,500); 00101 printf(response); 00102 printf ("\r\n"); 00103 } 00104 00105 void UBloxSara::sendUdpMsg(char *msg, char *flags) 00106 { 00107 char myCommandbuffer[250]; 00108 int msgSize = strlen(msg); 00109 char data[msgSize*2]; 00110 int flagsSize = strlen(flags); 00111 char flagsHex[flagsSize*2]; 00112 00113 // Create UDP socket on port 'port' 00114 sprintf(myCommandbuffer,"AT+NSOCR=\"DGRAM\",17,%d",_udp._port); 00115 sendCommand(myCommandbuffer); 00116 // Prepaire data for transmition 00117 for (int i=0, j=0; i<msgSize; i++, j+=2) 00118 { 00119 // Take i-th byte and make hex string out of it 00120 sprintf((data+j),"%x", *(msg+i)); 00121 } 00122 for(int i=0, j=0; i<flagsSize; i++, j+=2) 00123 { 00124 // Take i-th byte and make hex string out of it 00125 sprintf((flagsHex+j), "%x", *(flags+i)); 00126 } 00127 00128 sprintf(myCommandbuffer,"AT+NSOST=0,\"%s\",%d,%d,\"%s\"", 00129 _udp._ip, _udp._port, msgSize, data); 00130 00131 //msgSize += strlen(flags); 00132 //sprintf(myCommandbuffer,"AT+NSOST=0,\"%s\",%d,%d,\"%s%s\"", 00133 // _udp._ip, _udp._port, msgSize, flagsHex, data); 00134 sendCommand(myCommandbuffer); 00135 } 00136 00137 #define SHORT_DELAY_MS (1000) 00138 uint8_t UBloxSara::connectNB() 00139 { 00140 sendCommand("at+NCONFIG=\"AUTOCONNECT\",\"FALSE\""); 00141 wait_ms(SHORT_DELAY_MS); 00142 sendCommand("at+NCONFIG=\"CR_0354_0338_SCRAMBLING\",\"TRUE\""); 00143 wait_ms(SHORT_DELAY_MS); 00144 sendCommand("at+NCONFIG=\"CR_0859_SI_AVOID\",\"TRUE\""); 00145 wait_ms(SHORT_DELAY_MS); 00146 sendCommand("at+NCONFIG?"); 00147 wait_ms(SHORT_DELAY_MS); 00148 sendCommand("at+cfun=0"); 00149 wait_ms(SHORT_DELAY_MS); 00150 sendCommand("AT+CGDCONT=1, \"IP\",\"nb.inetd.gdsp\""); 00151 wait_ms(SHORT_DELAY_MS); 00152 sendCommand("at+cfun=1"); 00153 wait_ms(SHORT_DELAY_MS); 00154 00155 sendCommand("at+cimi"); 00156 wait_ms(SHORT_DELAY_MS); 00157 00158 sendCommand("at+cgatt=1"); 00159 wait_ms(SHORT_DELAY_MS); 00160 00161 sendCommand("at+cops=1,2,\"26202\""); 00162 wait_ms(5000); 00163 sendCommand("at+cereg?"); 00164 wait_ms(5000); 00165 sendCommand("AT+CSQ"); 00166 wait_ms(5000); 00167 sendCommand("AT+COPS?"); 00168 sendCommand("AT+NBAND?"); 00169 sendCommand("AT+NBAND=20"); 00170 wait_ms(SHORT_DELAY_MS); 00171 sendCommand("AT+NUESTATS"); 00172 wait_ms(SHORT_DELAY_MS); 00173 sendCommand("AT+CGATT?"); 00174 wait_ms(5000); 00175 sendCommand("AT+CGPADDR"); 00176 wait_ms(SHORT_DELAY_MS); 00177 }
Generated on Tue Jul 12 2022 15:46:17 by
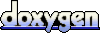