
The program sends the current location over the cellular network.
Dependencies: aconno_I2C ublox-at-cellular-interface gnss ublox-cellular-base Lis2dh12 ublox-cellular-base-n2xx ublox-at-cellular-interface-n2xx low-power-sleep
Fork of example-gnss by
main.cpp
00001 /** 00002 * SAP Project by aconno 00003 * Made by Jurica @ aconno 00004 * More info @ aconno.de 00005 * 00006 */ 00007 00008 #include "mbed.h" 00009 #include "ATCmdParser.h" 00010 #include "FileHandle.h" 00011 #include "gnss.h" 00012 #include "aconnoConfig.h" 00013 #include "uBloxSara.h" 00014 #include "aconnoHelpers.h" 00015 #include "Lis2dh12.h" 00016 #include "tasks/tasks.h" 00017 00018 #define RED_LED_PIN (PE_3) 00019 #define GREEN_LED_PIN (PE_4) 00020 #define BLUE_LED_PIN (PE_1) 00021 #define ALARM_OFF (0) 00022 #define ALARM_ON (1) 00023 00024 volatile MainStates state = STATE_IDLE; 00025 00026 /* File handler */ 00027 FileHandle *fh; 00028 00029 /* AT Command Parser handle */ 00030 ATCmdParser *at; 00031 GnssSerial gnss; // Serial interface (not I2C) 00032 char locationGlobal[UDP_MSG_SIZE_B]; 00033 bool gotGnssFlag = false; 00034 DigitalIn userButton(USER_BUTTON); 00035 DigitalOut gnssPower(PE_0, 0); 00036 00037 DigitalOut redLed(RED_LED_PIN); 00038 DigitalOut blueLed(BLUE_LED_PIN); 00039 DigitalOut greenLed(GREEN_LED_PIN); 00040 DigitalOut alarm(PE_14, 0); 00041 InterruptIn buttonInt(USER_BUTTON); 00042 00043 static bool wasAccInt = false; 00044 static bool wasButtPressed = false; 00045 static bool longPress = false; 00046 Timer buttonTimer; 00047 Thread timerThread; 00048 Thread idleState; 00049 Thread alarmState; 00050 Thread alarmOffState; 00051 Thread movementState; 00052 Thread gnssLocation; 00053 00054 void my_bsp_init() 00055 { 00056 redLed = 1; 00057 greenLed = 1; 00058 blueLed = 1; 00059 alarm = ALARM_OFF; 00060 } 00061 00062 void buttonRiseHandler(void) 00063 { 00064 buttonTimer.reset(); 00065 buttonTimer.start(); 00066 timerThread.signal_set(BUTTON_PRESSED_SIGNAL); 00067 } 00068 00069 void timerCallback(void) 00070 { 00071 float secondsPassed; 00072 while(true) 00073 { 00074 bool nextState = false; 00075 secondsPassed = 0; 00076 Thread::signal_wait(BUTTON_PRESSED_SIGNAL); 00077 Thread::signal_clr(BUTTON_PRESSED_SIGNAL); 00078 while(!nextState) 00079 { 00080 secondsPassed = buttonTimer.read(); 00081 if(state != STATE_ALARM && secondsPassed > START_ALARM_S) 00082 { 00083 wasButtPressed = true; 00084 buttonTimer.stop(); 00085 buttonTimer.reset(); 00086 nextState = true; 00087 } 00088 else if(state == STATE_ALARM && secondsPassed > STOP_ALARM_S) 00089 { 00090 longPress = true; 00091 buttonTimer.stop(); 00092 buttonTimer.reset(); 00093 nextState = true; 00094 } 00095 } 00096 } 00097 } 00098 00099 int main() 00100 { 00101 bool success = false; 00102 MainStates nextState = STATE_IDLE; 00103 00104 buttonInt.rise(buttonRiseHandler); 00105 my_bsp_init(); 00106 00107 printf("Initialising UART for modem communication: "); 00108 fh = new UARTSerial(MDMTXD, MDMRXD, 9600); 00109 printf("...done\r\n"); 00110 00111 printf("Initialising the modem AT command parser: "); 00112 at = new ATCmdParser(fh, OUTPUT_ENTER_KEY, AT_PARSER_BUFFER_SIZE, 00113 AT_PARSER_TIMEOUT, false); 00114 printf("...done\r\n"); 00115 00116 gnssPower = 1; 00117 wait_ms(500); 00118 00119 if(gnss.init()) 00120 { 00121 printf("Cold start... waiting to get first location data\r\n"); 00122 getGPSData(locationGlobal, &gnss); 00123 printf("I have the location.\r\n"); 00124 gotGnssFlag = true; 00125 // Turn green led only 00126 greenLed = 0; 00127 redLed = 1; 00128 } 00129 else 00130 { 00131 printf("Unable to initialise GNSS.\r\n"); 00132 } 00133 00134 Udp udp("52.215.10.12", 3334); 00135 UBloxSara sara(at, udp); 00136 printf("Initializing the modem: \r\n"); 00137 sara.setup(); 00138 sara.connectNB(); 00139 printf("done...\r\n"); 00140 00141 alarm = ALARM_OFF; 00142 greenLed = 1; 00143 blueLed = 0; 00144 myParams_t myParams; 00145 myParams.gnss = &gnss; 00146 myParams.sara = &sara; 00147 00148 timerThread.start(timerCallback); 00149 idleState.start(idleCallback); 00150 alarmState.start(callback(alarmCallback, &myParams)); 00151 alarmOffState.start(alarmOffCallback); 00152 movementState.start(movementCallback); 00153 gnssLocation.start(callback(gnssLocationCallback, &gnss)); 00154 00155 char commandbuffer[100]; 00156 00157 while(1) 00158 { 00159 switch(state) 00160 { 00161 case STATE_IDLE: 00162 if(wasAccInt) 00163 { 00164 wasAccInt = false; 00165 nextState = STATE_LIS_DETECTION; 00166 } 00167 if(wasButtPressed) 00168 { 00169 wasButtPressed = false; 00170 nextState = STATE_ALARM; 00171 alarmState.signal_set(ALARM_SIGNAL); 00172 greenLed = 1; 00173 redLed = 0; 00174 blueLed = 1; 00175 } 00176 break; 00177 case STATE_ALARM: 00178 if(longPress) 00179 { 00180 redLed = 1; 00181 longPress = false; 00182 nextState = STATE_ALARM_OFF; 00183 alarmOffState.signal_set(ALARM_OFF_SIGNAL); 00184 } 00185 else 00186 { 00187 alarm = ALARM_ON; 00188 } 00189 break; 00190 case STATE_ALARM_OFF: 00191 nextState = STATE_IDLE; 00192 idleState.signal_set(IDLE_SIGNAL); 00193 alarm = ALARM_OFF; 00194 blueLed = 0; 00195 break; 00196 case STATE_LIS_DETECTION: 00197 break; 00198 } 00199 state = nextState; 00200 wait_ms(125); 00201 } 00202 }
Generated on Tue Jul 12 2022 15:46:17 by
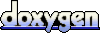