
Firmware for head gesture sensor
Dependencies: BLE_API MPU6050 mbed nRF51822
main.cpp
00001 #include "mbed.h" 00002 #include "MPU6050.h" 00003 #include "ble/BLE.h" 00004 00005 00006 DigitalOut myled(LED1); 00007 00008 MPU6050 ark(I2C_SDA0, I2C_SCL0); 00009 00010 BLE ble; 00011 00012 uint8_t AdvData[] = {0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff}; 00013 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00014 { 00015 BLE::Instance().gap().startAdvertising(); 00016 } 00017 00018 void periodicCallback(void) 00019 { 00020 } 00021 00022 void disconnectionCallback(Gap::Handle_t handle, Gap::DisconnectionReason_t reason) // Mod 00023 { 00024 ble.startAdvertising(); 00025 } 00026 00027 void timeoutCallback(const Gap::TimeoutSource_t source) 00028 { 00029 ble.startAdvertising(); 00030 } 00031 00032 int main() 00033 { 00034 ble.init(); 00035 00036 while(1) { 00037 //reading Temprature 00038 float temp = ark.getTemp(); 00039 // int tempData = floor(temp); 00040 // AdvData[1] = tempData; 00041 00042 //reading Gyrometer readings 00043 float gyro[3]; 00044 ark.getGyro(gyro); 00045 00046 00047 float* inputAddress = &gyro[0]; 00048 float* inputAddress2 = &gyro[1]; 00049 float* inputAddress3 = &gyro[2]; 00050 // float* inputAddress = &input; 00051 long int* ptr = (long int*)inputAddress; 00052 long int* ptr2 = (long int*)inputAddress2; 00053 long int* ptr3 = (long int*)inputAddress3; 00054 00055 // uint32_t typeCastedx = (uint32_t)x; 00056 //int gyroData1 = floor(gyro[0]*1000); 00057 AdvData[0] = *ptr >> 24; 00058 AdvData[1] = *ptr >> 16; 00059 AdvData[2] = *ptr >> 8; 00060 AdvData[3] = *ptr; 00061 00062 AdvData[4] = *ptr2 >> 24; 00063 AdvData[5] = *ptr2 >> 16; 00064 AdvData[6] = *ptr2 >> 8; 00065 AdvData[7] = *ptr2; 00066 00067 AdvData[8] = *ptr3 >> 24; 00068 AdvData[9] = *ptr3 >> 16; 00069 AdvData[10] = *ptr3 >> 8; 00070 AdvData[11] = *ptr3; 00071 // int gyroData2 = floor(gyro[1]*1000); 00072 // uint8_t gyroData2 = gyro[1]; 00073 // AdvData[2] = gyroData2; 00074 // int gyroData3 = floor(gyro[2]*1000); 00075 // uint8_t gyroData3 = gyro[2]; 00076 // AdvData[3] = gyroData3; 00077 00078 //reading Acclerometer readings 00079 float acce[3]; 00080 ark.getAccelero(acce); 00081 int acceData1 = floor(acce[0]*1000); 00082 // AdvData[5] = acceData1; 00083 int acceData2 = floor(acce[1]*1000); 00084 // AdvData[6] = acceData2; 00085 int acceData3 = floor(acce[2]*1000); 00086 // AdvData[7] = acceData3; 00087 00088 wait(0.1); 00089 00090 ble.setAdvertisingType(GapAdvertisingParams::ADV_NON_CONNECTABLE_UNDIRECTED); 00091 ble.setAdvertisingInterval(160); /* 100ms; in multiples of 0.625ms. */ 00092 ble.accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, AdvData, sizeof(AdvData)); 00093 ble.startAdvertising(); 00094 00095 00096 /* SpinWait for initialization to complete. This is necessary because the 00097 * BLE object is used in the main loop below. */ 00098 while (ble.hasInitialized() == false) { 00099 /* spin loop */ 00100 } 00101 00102 00103 } 00104 00105 }
Generated on Tue Jul 12 2022 21:35:37 by
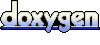