
opencv on mbed
KNNUniqueResultSet< DistanceType > Class Template Reference
Class that holds the k NN neighbors Faster than KNNResultSet as it uses a binary heap and does not maintain two arrays. More...
#include <result_set.h>
Inherits cvflann::UniqueResultSet< DistanceType >.
Inherited by KNNRadiusUniqueResultSet< DistanceType >.
Public Member Functions | |
KNNUniqueResultSet (unsigned int capacity) | |
Constructor. | |
void | addPoint (DistanceType dist, int index) |
Add a possible candidate to the best neighbors. | |
void | clear () |
Remove all elements in the set. | |
bool | full () const |
Check the status of the set. | |
virtual void | copy (int *indices, DistanceType *dist, int n_neighbors=-1) const |
Copy the set to two C arrays. | |
virtual void | sortAndCopy (int *indices, DistanceType *dist, int n_neighbors=-1) const |
Copy the set to two C arrays but sort it according to the distance first. | |
size_t | size () const |
The number of neighbors in the set. | |
DistanceType | worstDist () const |
The distance of the furthest neighbor If we don't have enough neighbors, it returns the max possible value. | |
Protected Attributes | |
unsigned int | capacity_ |
The number of neighbors to keep. | |
bool | is_full_ |
Flag to say if the set is full. | |
DistanceType | worst_distance_ |
The worst distance found so far. | |
std::set< DistIndex > | dist_indices_ |
The best candidates so far. |
Detailed Description
template<typename DistanceType>
class cvflann::KNNUniqueResultSet< DistanceType >
Class that holds the k NN neighbors Faster than KNNResultSet as it uses a binary heap and does not maintain two arrays.
Definition at line 389 of file result_set.h.
Constructor & Destructor Documentation
KNNUniqueResultSet | ( | unsigned int | capacity ) |
Constructor.
- Parameters:
-
capacity the number of neighbors to store at max
Definition at line 395 of file result_set.h.
Member Function Documentation
void addPoint | ( | DistanceType | dist, |
int | index | ||
) |
Add a possible candidate to the best neighbors.
- Parameters:
-
dist distance for that neighbor index index of that neighbor
Definition at line 405 of file result_set.h.
void clear | ( | ) | [virtual] |
Remove all elements in the set.
Implements UniqueResultSet< DistanceType >.
Reimplemented in KNNRadiusUniqueResultSet< DistanceType >.
Definition at line 425 of file result_set.h.
virtual void copy | ( | int * | indices, |
DistanceType * | dist, | ||
int | n_neighbors = -1 |
||
) | const [virtual, inherited] |
Copy the set to two C arrays.
- Parameters:
-
indices pointer to a C array of indices dist pointer to a C array of distances n_neighbors the number of neighbors to copy
Definition at line 327 of file result_set.h.
bool full | ( | ) | const [inherited] |
Check the status of the set.
- Returns:
- true if we have k NN
Reimplemented in RadiusUniqueResultSet< DistanceType >.
Definition at line 313 of file result_set.h.
size_t size | ( | ) | const [inherited] |
virtual void sortAndCopy | ( | int * | indices, |
DistanceType * | dist, | ||
int | n_neighbors = -1 |
||
) | const [virtual, inherited] |
Copy the set to two C arrays but sort it according to the distance first.
- Parameters:
-
indices pointer to a C array of indices dist pointer to a C array of distances n_neighbors the number of neighbors to copy
Definition at line 351 of file result_set.h.
DistanceType worstDist | ( | ) | const [inherited] |
The distance of the furthest neighbor If we don't have enough neighbors, it returns the max possible value.
- Returns:
Reimplemented in RadiusUniqueResultSet< DistanceType >.
Definition at line 368 of file result_set.h.
Field Documentation
unsigned int capacity_ [protected] |
The number of neighbors to keep.
Definition at line 439 of file result_set.h.
std::set<DistIndex> dist_indices_ [protected, inherited] |
The best candidates so far.
Definition at line 380 of file result_set.h.
bool is_full_ [protected, inherited] |
Flag to say if the set is full.
Definition at line 374 of file result_set.h.
DistanceType worst_distance_ [protected, inherited] |
The worst distance found so far.
Definition at line 377 of file result_set.h.
Generated on Tue Jul 12 2022 16:42:45 by
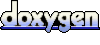