
opencv on mbed
Embed:
(wiki syntax)
Show/hide line numbers
types.hpp
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2000-2008, Intel Corporation, all rights reserved. 00014 // Copyright (C) 2009, Willow Garage Inc., all rights reserved. 00015 // Copyright (C) 2013, OpenCV Foundation, all rights reserved. 00016 // Third party copyrights are property of their respective owners. 00017 // 00018 // Redistribution and use in source and binary forms, with or without modification, 00019 // are permitted provided that the following conditions are met: 00020 // 00021 // * Redistribution's of source code must retain the above copyright notice, 00022 // this list of conditions and the following disclaimer. 00023 // 00024 // * Redistribution's in binary form must reproduce the above copyright notice, 00025 // this list of conditions and the following disclaimer in the documentation 00026 // and/or other materials provided with the distribution. 00027 // 00028 // * The name of the copyright holders may not be used to endorse or promote products 00029 // derived from this software without specific prior written permission. 00030 // 00031 // This software is provided by the copyright holders and contributors "as is" and 00032 // any express or implied warranties, including, but not limited to, the implied 00033 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00034 // In no event shall the Intel Corporation or contributors be liable for any direct, 00035 // indirect, incidental, special, exemplary, or consequential damages 00036 // (including, but not limited to, procurement of substitute goods or services; 00037 // loss of use, data, or profits; or business interruption) however caused 00038 // and on any theory of liability, whether in contract, strict liability, 00039 // or tort (including negligence or otherwise) arising in any way out of 00040 // the use of this software, even if advised of the possibility of such damage. 00041 // 00042 //M*/ 00043 00044 #ifndef __OPENCV_CORE_TYPES_HPP__ 00045 #define __OPENCV_CORE_TYPES_HPP__ 00046 00047 #ifndef __cplusplus 00048 # error types.hpp header must be compiled as C++ 00049 #endif 00050 00051 #include <climits> 00052 #include <cfloat> 00053 #include <vector> 00054 00055 #include "opencv2/core/cvdef.h" 00056 #include "opencv2/core/cvstd.hpp" 00057 #include "opencv2/core/matx.hpp" 00058 00059 namespace cv 00060 { 00061 00062 //! @addtogroup core_basic 00063 //! @{ 00064 00065 //////////////////////////////// Complex ////////////////////////////// 00066 00067 /** @brief A complex number class. 00068 00069 The template class is similar and compatible with std::complex, however it provides slightly 00070 more convenient access to the real and imaginary parts using through the simple field access, as opposite 00071 to std::complex::real() and std::complex::imag(). 00072 */ 00073 template<typename _Tp> class Complex 00074 { 00075 public: 00076 00077 //! constructors 00078 Complex(); 00079 Complex( _Tp _re, _Tp _im = 0 ); 00080 00081 //! conversion to another data type 00082 template<typename T2> operator Complex<T2>() const; 00083 //! conjugation 00084 Complex conj() const; 00085 00086 _Tp re, im; //< the real and the imaginary parts 00087 }; 00088 00089 typedef Complex<float> Complexf; 00090 typedef Complex<double> Complexd; 00091 00092 template<typename _Tp> class DataType< Complex<_Tp> > 00093 { 00094 public: 00095 typedef Complex<_Tp> value_type; 00096 typedef value_type work_type; 00097 typedef _Tp channel_type; 00098 00099 enum { generic_type = 0, 00100 depth = DataType<channel_type>::depth, 00101 channels = 2, 00102 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00103 type = CV_MAKETYPE(depth, channels) }; 00104 00105 typedef Vec<channel_type, channels> vec_type; 00106 }; 00107 00108 00109 00110 //////////////////////////////// Point_ //////////////////////////////// 00111 00112 /** @brief Template class for 2D points specified by its coordinates `x` and `y`. 00113 00114 An instance of the class is interchangeable with C structures, CvPoint and CvPoint2D32f . There is 00115 also a cast operator to convert point coordinates to the specified type. The conversion from 00116 floating-point coordinates to integer coordinates is done by rounding. Commonly, the conversion 00117 uses this operation for each of the coordinates. Besides the class members listed in the 00118 declaration above, the following operations on points are implemented: 00119 @code 00120 pt1 = pt2 + pt3; 00121 pt1 = pt2 - pt3; 00122 pt1 = pt2 * a; 00123 pt1 = a * pt2; 00124 pt1 = pt2 / a; 00125 pt1 += pt2; 00126 pt1 -= pt2; 00127 pt1 *= a; 00128 pt1 /= a; 00129 double value = norm(pt); // L2 norm 00130 pt1 == pt2; 00131 pt1 != pt2; 00132 @endcode 00133 For your convenience, the following type aliases are defined: 00134 @code 00135 typedef Point_<int> Point2i; 00136 typedef Point2i Point; 00137 typedef Point_<float> Point2f; 00138 typedef Point_<double> Point2d; 00139 @endcode 00140 Example: 00141 @code 00142 Point2f a(0.3f, 0.f), b(0.f, 0.4f); 00143 Point pt = (a + b)*10.f; 00144 cout << pt.x << ", " << pt.y << endl; 00145 @endcode 00146 */ 00147 template<typename _Tp> class Point_ 00148 { 00149 public: 00150 typedef _Tp value_type; 00151 00152 // various constructors 00153 Point_(); 00154 Point_(_Tp _x, _Tp _y); 00155 Point_(const Point_& pt); 00156 Point_(const Size_<_Tp>& sz); 00157 Point_(const Vec<_Tp, 2>& v); 00158 00159 Point_& operator = (const Point_& pt); 00160 //! conversion to another data type 00161 template<typename _Tp2> operator Point_<_Tp2>() const; 00162 00163 //! conversion to the old-style C structures 00164 operator Vec<_Tp, 2>() const; 00165 00166 //! dot product 00167 _Tp dot(const Point_& pt) const; 00168 //! dot product computed in double-precision arithmetics 00169 double ddot(const Point_& pt) const; 00170 //! cross-product 00171 double cross(const Point_& pt) const; 00172 //! checks whether the point is inside the specified rectangle 00173 bool inside(const Rect_<_Tp>& r) const; 00174 00175 _Tp x, y; //< the point coordinates 00176 }; 00177 00178 typedef Point_<int> Point2i; 00179 typedef Point_<float> Point2f ; 00180 typedef Point_<double> Point2d; 00181 typedef Point2i Point; 00182 00183 template<typename _Tp> class DataType< Point_<_Tp> > 00184 { 00185 public: 00186 typedef Point_<_Tp> value_type; 00187 typedef Point_<typename DataType<_Tp>::work_type> work_type; 00188 typedef _Tp channel_type; 00189 00190 enum { generic_type = 0, 00191 depth = DataType<channel_type>::depth, 00192 channels = 2, 00193 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00194 type = CV_MAKETYPE(depth, channels) 00195 }; 00196 00197 typedef Vec<channel_type, channels> vec_type; 00198 }; 00199 00200 00201 00202 //////////////////////////////// Point3_ //////////////////////////////// 00203 00204 /** @brief Template class for 3D points specified by its coordinates `x`, `y` and `z`. 00205 00206 An instance of the class is interchangeable with the C structure CvPoint2D32f . Similarly to 00207 Point_ , the coordinates of 3D points can be converted to another type. The vector arithmetic and 00208 comparison operations are also supported. 00209 00210 The following Point3_<> aliases are available: 00211 @code 00212 typedef Point3_<int> Point3i; 00213 typedef Point3_<float> Point3f; 00214 typedef Point3_<double> Point3d; 00215 @endcode 00216 @see cv::Point3i, cv::Point3f and cv::Point3d 00217 */ 00218 template<typename _Tp> class Point3_ 00219 { 00220 public: 00221 typedef _Tp value_type; 00222 00223 // various constructors 00224 Point3_(); 00225 Point3_(_Tp _x, _Tp _y, _Tp _z); 00226 Point3_(const Point3_& pt); 00227 explicit Point3_(const Point_<_Tp>& pt); 00228 Point3_(const Vec<_Tp, 3>& v); 00229 00230 Point3_& operator = (const Point3_& pt); 00231 //! conversion to another data type 00232 template<typename _Tp2> operator Point3_<_Tp2>() const; 00233 //! conversion to cv::Vec<> 00234 operator Vec<_Tp, 3>() const; 00235 00236 //! dot product 00237 _Tp dot(const Point3_& pt) const; 00238 //! dot product computed in double-precision arithmetics 00239 double ddot(const Point3_& pt) const; 00240 //! cross product of the 2 3D points 00241 Point3_ cross(const Point3_& pt) const; 00242 00243 _Tp x, y, z; //< the point coordinates 00244 }; 00245 00246 typedef Point3_<int> Point3i; 00247 typedef Point3_<float> Point3f; 00248 typedef Point3_<double> Point3d; 00249 00250 template<typename _Tp> class DataType< Point3_<_Tp> > 00251 { 00252 public: 00253 typedef Point3_<_Tp> value_type; 00254 typedef Point3_<typename DataType<_Tp>::work_type> work_type; 00255 typedef _Tp channel_type; 00256 00257 enum { generic_type = 0, 00258 depth = DataType<channel_type>::depth, 00259 channels = 3, 00260 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00261 type = CV_MAKETYPE(depth, channels) 00262 }; 00263 00264 typedef Vec<channel_type, channels> vec_type; 00265 }; 00266 00267 00268 00269 //////////////////////////////// Size_ //////////////////////////////// 00270 00271 /** @brief Template class for specifying the size of an image or rectangle. 00272 00273 The class includes two members called width and height. The structure can be converted to and from 00274 the old OpenCV structures CvSize and CvSize2D32f . The same set of arithmetic and comparison 00275 operations as for Point_ is available. 00276 00277 OpenCV defines the following Size_<> aliases: 00278 @code 00279 typedef Size_<int> Size2i; 00280 typedef Size2i Size; 00281 typedef Size_<float> Size2f; 00282 @endcode 00283 */ 00284 template<typename _Tp> class Size_ 00285 { 00286 public: 00287 typedef _Tp value_type; 00288 00289 //! various constructors 00290 Size_(); 00291 Size_(_Tp _width, _Tp _height); 00292 Size_(const Size_& sz); 00293 Size_(const Point_<_Tp>& pt); 00294 00295 Size_& operator = (const Size_& sz); 00296 //! the area (width*height) 00297 _Tp area() const; 00298 00299 //! conversion of another data type. 00300 template<typename _Tp2> operator Size_<_Tp2>() const; 00301 00302 _Tp width, height; // the width and the height 00303 }; 00304 00305 typedef Size_<int> Size2i; 00306 typedef Size_<float> Size2f ; 00307 typedef Size_<double> Size2d; 00308 typedef Size2i Size; 00309 00310 template<typename _Tp> class DataType< Size_<_Tp> > 00311 { 00312 public: 00313 typedef Size_<_Tp> value_type; 00314 typedef Size_<typename DataType<_Tp>::work_type> work_type; 00315 typedef _Tp channel_type; 00316 00317 enum { generic_type = 0, 00318 depth = DataType<channel_type>::depth, 00319 channels = 2, 00320 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00321 type = CV_MAKETYPE(depth, channels) 00322 }; 00323 00324 typedef Vec<channel_type, channels> vec_type; 00325 }; 00326 00327 00328 00329 //////////////////////////////// Rect_ //////////////////////////////// 00330 00331 /** @brief Template class for 2D rectangles 00332 00333 described by the following parameters: 00334 - Coordinates of the top-left corner. This is a default interpretation of Rect_::x and Rect_::y 00335 in OpenCV. Though, in your algorithms you may count x and y from the bottom-left corner. 00336 - Rectangle width and height. 00337 00338 OpenCV typically assumes that the top and left boundary of the rectangle are inclusive, while the 00339 right and bottom boundaries are not. For example, the method Rect_::contains returns true if 00340 00341 \f[x \leq pt.x < x+width, 00342 y \leq pt.y < y+height\f] 00343 00344 Virtually every loop over an image ROI in OpenCV (where ROI is specified by Rect_<int> ) is 00345 implemented as: 00346 @code 00347 for(int y = roi.y; y < roi.y + roi.height; y++) 00348 for(int x = roi.x; x < roi.x + roi.width; x++) 00349 { 00350 // ... 00351 } 00352 @endcode 00353 In addition to the class members, the following operations on rectangles are implemented: 00354 - \f$\texttt{rect} = \texttt{rect} \pm \texttt{point}\f$ (shifting a rectangle by a certain offset) 00355 - \f$\texttt{rect} = \texttt{rect} \pm \texttt{size}\f$ (expanding or shrinking a rectangle by a 00356 certain amount) 00357 - rect += point, rect -= point, rect += size, rect -= size (augmenting operations) 00358 - rect = rect1 & rect2 (rectangle intersection) 00359 - rect = rect1 | rect2 (minimum area rectangle containing rect1 and rect2 ) 00360 - rect &= rect1, rect |= rect1 (and the corresponding augmenting operations) 00361 - rect == rect1, rect != rect1 (rectangle comparison) 00362 00363 This is an example how the partial ordering on rectangles can be established (rect1 \f$\subseteq\f$ 00364 rect2): 00365 @code 00366 template<typename _Tp> inline bool 00367 operator <= (const Rect_<_Tp>& r1, const Rect_<_Tp>& r2) 00368 { 00369 return (r1 & r2) == r1; 00370 } 00371 @endcode 00372 For your convenience, the Rect_<> alias is available: cv::Rect 00373 */ 00374 template<typename _Tp> class Rect_ 00375 { 00376 public: 00377 typedef _Tp value_type; 00378 00379 //! various constructors 00380 Rect_(); 00381 Rect_(_Tp _x, _Tp _y, _Tp _width, _Tp _height); 00382 Rect_(const Rect_& r); 00383 Rect_(const Point_<_Tp>& org, const Size_<_Tp>& sz); 00384 Rect_(const Point_<_Tp>& pt1, const Point_<_Tp>& pt2); 00385 00386 Rect_& operator = ( const Rect_& r ); 00387 //! the top-left corner 00388 Point_<_Tp> tl() const; 00389 //! the bottom-right corner 00390 Point_<_Tp> br() const; 00391 00392 //! size (width, height) of the rectangle 00393 Size_<_Tp> size() const; 00394 //! area (width*height) of the rectangle 00395 _Tp area() const; 00396 00397 //! conversion to another data type 00398 template<typename _Tp2> operator Rect_<_Tp2>() const; 00399 00400 //! checks whether the rectangle contains the point 00401 bool contains(const Point_<_Tp>& pt) const; 00402 00403 _Tp x, y, width, height; //< the top-left corner, as well as width and height of the rectangle 00404 }; 00405 00406 typedef Rect_<int> Rect2i; 00407 typedef Rect_<float> Rect2f; 00408 typedef Rect_<double> Rect2d; 00409 typedef Rect2i Rect; 00410 00411 template<typename _Tp> class DataType< Rect_<_Tp> > 00412 { 00413 public: 00414 typedef Rect_<_Tp> value_type; 00415 typedef Rect_<typename DataType<_Tp>::work_type> work_type; 00416 typedef _Tp channel_type; 00417 00418 enum { generic_type = 0, 00419 depth = DataType<channel_type>::depth, 00420 channels = 4, 00421 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00422 type = CV_MAKETYPE(depth, channels) 00423 }; 00424 00425 typedef Vec<channel_type, channels> vec_type; 00426 }; 00427 00428 00429 00430 ///////////////////////////// RotatedRect ///////////////////////////// 00431 00432 /** @brief The class represents rotated (i.e. not up-right) rectangles on a plane. 00433 00434 Each rectangle is specified by the center point (mass center), length of each side (represented by 00435 cv::Size2f structure) and the rotation angle in degrees. 00436 00437 The sample below demonstrates how to use RotatedRect: 00438 @code 00439 Mat image(200, 200, CV_8UC3, Scalar(0)); 00440 RotatedRect rRect = RotatedRect(Point2f(100,100), Size2f(100,50), 30); 00441 00442 Point2f vertices[4]; 00443 rRect.points(vertices); 00444 for (int i = 0; i < 4; i++) 00445 line(image, vertices[i], vertices[(i+1)%4], Scalar(0,255,0)); 00446 00447 Rect brect = rRect.boundingRect(); 00448 rectangle(image, brect, Scalar(255,0,0)); 00449 00450 imshow("rectangles", image); 00451 waitKey(0); 00452 @endcode 00453  00454 00455 @sa CamShift, fitEllipse, minAreaRect, CvBox2D 00456 */ 00457 class CV_EXPORTS RotatedRect 00458 { 00459 public: 00460 //! various constructors 00461 RotatedRect(); 00462 /** 00463 @param center The rectangle mass center. 00464 @param size Width and height of the rectangle. 00465 @param angle The rotation angle in a clockwise direction. When the angle is 0, 90, 180, 270 etc., 00466 the rectangle becomes an up-right rectangle. 00467 */ 00468 RotatedRect(const Point2f & center, const Size2f & size, float angle); 00469 /** 00470 Any 3 end points of the RotatedRect. They must be given in order (either clockwise or 00471 anticlockwise). 00472 */ 00473 RotatedRect(const Point2f & point1, const Point2f & point2, const Point2f & point3); 00474 00475 /** returns 4 vertices of the rectangle 00476 @param pts The points array for storing rectangle vertices. 00477 */ 00478 void points(Point2f pts[]) const; 00479 //! returns the minimal up-right rectangle containing the rotated rectangle 00480 Rect boundingRect() const; 00481 00482 Point2f center; //< the rectangle mass center 00483 Size2f size; //< width and height of the rectangle 00484 float angle; //< the rotation angle. When the angle is 0, 90, 180, 270 etc., the rectangle becomes an up-right rectangle. 00485 }; 00486 00487 template<> class DataType< RotatedRect > 00488 { 00489 public: 00490 typedef RotatedRect value_type; 00491 typedef value_type work_type; 00492 typedef float channel_type; 00493 00494 enum { generic_type = 0, 00495 depth = DataType<channel_type>::depth, 00496 channels = (int)sizeof(value_type)/sizeof(channel_type), // 5 00497 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00498 type = CV_MAKETYPE(depth, channels) 00499 }; 00500 00501 typedef Vec<channel_type, channels> vec_type; 00502 }; 00503 00504 00505 00506 //////////////////////////////// Range ///////////////////////////////// 00507 00508 /** @brief Template class specifying a continuous subsequence (slice) of a sequence. 00509 00510 The class is used to specify a row or a column span in a matrix ( Mat ) and for many other purposes. 00511 Range(a,b) is basically the same as a:b in Matlab or a..b in Python. As in Python, start is an 00512 inclusive left boundary of the range and end is an exclusive right boundary of the range. Such a 00513 half-opened interval is usually denoted as \f$[start,end)\f$ . 00514 00515 The static method Range::all() returns a special variable that means "the whole sequence" or "the 00516 whole range", just like " : " in Matlab or " ... " in Python. All the methods and functions in 00517 OpenCV that take Range support this special Range::all() value. But, of course, in case of your own 00518 custom processing, you will probably have to check and handle it explicitly: 00519 @code 00520 void my_function(..., const Range& r, ....) 00521 { 00522 if(r == Range::all()) { 00523 // process all the data 00524 } 00525 else { 00526 // process [r.start, r.end) 00527 } 00528 } 00529 @endcode 00530 */ 00531 class CV_EXPORTS Range 00532 { 00533 public: 00534 Range(); 00535 Range(int _start, int _end); 00536 int size() const; 00537 bool empty() const; 00538 static Range all(); 00539 00540 int start, end; 00541 }; 00542 00543 template<> class DataType<Range> 00544 { 00545 public: 00546 typedef Range value_type; 00547 typedef value_type work_type; 00548 typedef int channel_type; 00549 00550 enum { generic_type = 0, 00551 depth = DataType<channel_type>::depth, 00552 channels = 2, 00553 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00554 type = CV_MAKETYPE(depth, channels) 00555 }; 00556 00557 typedef Vec<channel_type, channels> vec_type; 00558 }; 00559 00560 00561 00562 //////////////////////////////// Scalar_ /////////////////////////////// 00563 00564 /** @brief Template class for a 4-element vector derived from Vec. 00565 00566 Being derived from Vec<_Tp, 4> , Scalar_ and Scalar can be used just as typical 4-element 00567 vectors. In addition, they can be converted to/from CvScalar . The type Scalar is widely used in 00568 OpenCV to pass pixel values. 00569 */ 00570 template<typename _Tp> class Scalar_ : public Vec<_Tp, 4> 00571 { 00572 public: 00573 //! various constructors 00574 Scalar_(); 00575 Scalar_(_Tp v0, _Tp v1, _Tp v2=0, _Tp v3=0); 00576 Scalar_(_Tp v0); 00577 00578 template<typename _Tp2, int cn> 00579 Scalar_(const Vec<_Tp2, cn>& v); 00580 00581 //! returns a scalar with all elements set to v0 00582 static Scalar_<_Tp> all(_Tp v0); 00583 00584 //! conversion to another data type 00585 template<typename T2> operator Scalar_<T2>() const; 00586 00587 //! per-element product 00588 Scalar_<_Tp> mul(const Scalar_<_Tp>& a, double scale=1 ) const; 00589 00590 // returns (v0, -v1, -v2, -v3) 00591 Scalar_<_Tp> conj() const; 00592 00593 // returns true iff v1 == v2 == v3 == 0 00594 bool isReal() const; 00595 }; 00596 00597 typedef Scalar_<double> Scalar ; 00598 00599 template<typename _Tp> class DataType< Scalar_<_Tp> > 00600 { 00601 public: 00602 typedef Scalar_<_Tp> value_type; 00603 typedef Scalar_<typename DataType<_Tp>::work_type> work_type; 00604 typedef _Tp channel_type; 00605 00606 enum { generic_type = 0, 00607 depth = DataType<channel_type>::depth, 00608 channels = 4, 00609 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00610 type = CV_MAKETYPE(depth, channels) 00611 }; 00612 00613 typedef Vec<channel_type, channels> vec_type; 00614 }; 00615 00616 00617 00618 /////////////////////////////// KeyPoint //////////////////////////////// 00619 00620 /** @brief Data structure for salient point detectors. 00621 00622 The class instance stores a keypoint, i.e. a point feature found by one of many available keypoint 00623 detectors, such as Harris corner detector, cv::FAST, cv::StarDetector, cv::SURF, cv::SIFT, 00624 cv::LDetector etc. 00625 00626 The keypoint is characterized by the 2D position, scale (proportional to the diameter of the 00627 neighborhood that needs to be taken into account), orientation and some other parameters. The 00628 keypoint neighborhood is then analyzed by another algorithm that builds a descriptor (usually 00629 represented as a feature vector). The keypoints representing the same object in different images 00630 can then be matched using cv::KDTree or another method. 00631 */ 00632 class CV_EXPORTS_W_SIMPLE KeyPoint 00633 { 00634 public: 00635 //! the default constructor 00636 CV_WRAP KeyPoint(); 00637 /** 00638 @param _pt x & y coordinates of the keypoint 00639 @param _size keypoint diameter 00640 @param _angle keypoint orientation 00641 @param _response keypoint detector response on the keypoint (that is, strength of the keypoint) 00642 @param _octave pyramid octave in which the keypoint has been detected 00643 @param _class_id object id 00644 */ 00645 KeyPoint(Point2f _pt, float _size, float _angle=-1, float _response=0, int _octave=0, int _class_id=-1); 00646 /** 00647 @param x x-coordinate of the keypoint 00648 @param y y-coordinate of the keypoint 00649 @param _size keypoint diameter 00650 @param _angle keypoint orientation 00651 @param _response keypoint detector response on the keypoint (that is, strength of the keypoint) 00652 @param _octave pyramid octave in which the keypoint has been detected 00653 @param _class_id object id 00654 */ 00655 CV_WRAP KeyPoint(float x, float y, float _size, float _angle=-1, float _response=0, int _octave=0, int _class_id=-1); 00656 00657 size_t hash() const; 00658 00659 /** 00660 This method converts vector of keypoints to vector of points or the reverse, where each keypoint is 00661 assigned the same size and the same orientation. 00662 00663 @param keypoints Keypoints obtained from any feature detection algorithm like SIFT/SURF/ORB 00664 @param points2f Array of (x,y) coordinates of each keypoint 00665 @param keypointIndexes Array of indexes of keypoints to be converted to points. (Acts like a mask to 00666 convert only specified keypoints) 00667 */ 00668 CV_WRAP static void convert(const std::vector<KeyPoint>& keypoints, 00669 CV_OUT std::vector<Point2f>& points2f, 00670 const std::vector<int>& keypointIndexes=std::vector<int>()); 00671 /** @overload 00672 @param points2f Array of (x,y) coordinates of each keypoint 00673 @param keypoints Keypoints obtained from any feature detection algorithm like SIFT/SURF/ORB 00674 @param size keypoint diameter 00675 @param response keypoint detector response on the keypoint (that is, strength of the keypoint) 00676 @param octave pyramid octave in which the keypoint has been detected 00677 @param class_id object id 00678 */ 00679 CV_WRAP static void convert(const std::vector<Point2f>& points2f, 00680 CV_OUT std::vector<KeyPoint>& keypoints, 00681 float size=1, float response=1, int octave=0, int class_id=-1); 00682 00683 /** 00684 This method computes overlap for pair of keypoints. Overlap is the ratio between area of keypoint 00685 regions' intersection and area of keypoint regions' union (considering keypoint region as circle). 00686 If they don't overlap, we get zero. If they coincide at same location with same size, we get 1. 00687 @param kp1 First keypoint 00688 @param kp2 Second keypoint 00689 */ 00690 CV_WRAP static float overlap(const KeyPoint& kp1, const KeyPoint& kp2); 00691 00692 CV_PROP_RW Point2f pt; //!< coordinates of the keypoints 00693 CV_PROP_RW float size; //!< diameter of the meaningful keypoint neighborhood 00694 CV_PROP_RW float angle; //!< computed orientation of the keypoint (-1 if not applicable); 00695 //!< it's in [0,360) degrees and measured relative to 00696 //!< image coordinate system, ie in clockwise. 00697 CV_PROP_RW float response; //!< the response by which the most strong keypoints have been selected. Can be used for the further sorting or subsampling 00698 CV_PROP_RW int octave; //!< octave (pyramid layer) from which the keypoint has been extracted 00699 CV_PROP_RW int class_id; //!< object class (if the keypoints need to be clustered by an object they belong to) 00700 }; 00701 00702 template<> class DataType<KeyPoint> 00703 { 00704 public: 00705 typedef KeyPoint value_type; 00706 typedef float work_type; 00707 typedef float channel_type; 00708 00709 enum { generic_type = 0, 00710 depth = DataType<channel_type>::depth, 00711 channels = (int)(sizeof(value_type)/sizeof(channel_type)), // 7 00712 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00713 type = CV_MAKETYPE(depth, channels) 00714 }; 00715 00716 typedef Vec<channel_type, channels> vec_type; 00717 }; 00718 00719 00720 00721 //////////////////////////////// DMatch ///////////////////////////////// 00722 00723 /** @brief Class for matching keypoint descriptors 00724 00725 query descriptor index, train descriptor index, train image index, and distance between 00726 descriptors. 00727 */ 00728 class CV_EXPORTS_W_SIMPLE DMatch 00729 { 00730 public: 00731 CV_WRAP DMatch(); 00732 CV_WRAP DMatch(int _queryIdx, int _trainIdx, float _distance); 00733 CV_WRAP DMatch(int _queryIdx, int _trainIdx, int _imgIdx, float _distance); 00734 00735 CV_PROP_RW int queryIdx; // query descriptor index 00736 CV_PROP_RW int trainIdx; // train descriptor index 00737 CV_PROP_RW int imgIdx; // train image index 00738 00739 CV_PROP_RW float distance; 00740 00741 // less is better 00742 bool operator<(const DMatch &m) const; 00743 }; 00744 00745 template<> class DataType<DMatch> 00746 { 00747 public: 00748 typedef DMatch value_type; 00749 typedef int work_type; 00750 typedef int channel_type; 00751 00752 enum { generic_type = 0, 00753 depth = DataType<channel_type>::depth, 00754 channels = (int)(sizeof(value_type)/sizeof(channel_type)), // 4 00755 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00756 type = CV_MAKETYPE(depth, channels) 00757 }; 00758 00759 typedef Vec<channel_type, channels> vec_type; 00760 }; 00761 00762 00763 00764 ///////////////////////////// TermCriteria ////////////////////////////// 00765 00766 /** @brief The class defining termination criteria for iterative algorithms. 00767 00768 You can initialize it by default constructor and then override any parameters, or the structure may 00769 be fully initialized using the advanced variant of the constructor. 00770 */ 00771 class CV_EXPORTS TermCriteria 00772 { 00773 public: 00774 /** 00775 Criteria type, can be one of: COUNT, EPS or COUNT + EPS 00776 */ 00777 enum Type 00778 { 00779 COUNT=1, //!< the maximum number of iterations or elements to compute 00780 MAX_ITER=COUNT, //!< ditto 00781 EPS=2 //!< the desired accuracy or change in parameters at which the iterative algorithm stops 00782 }; 00783 00784 //! default constructor 00785 TermCriteria(); 00786 /** 00787 @param type The type of termination criteria, one of TermCriteria::Type 00788 @param maxCount The maximum number of iterations or elements to compute. 00789 @param epsilon The desired accuracy or change in parameters at which the iterative algorithm stops. 00790 */ 00791 TermCriteria(int type, int maxCount, double epsilon); 00792 00793 int type; //!< the type of termination criteria: COUNT, EPS or COUNT + EPS 00794 int maxCount; // the maximum number of iterations/elements 00795 double epsilon; // the desired accuracy 00796 }; 00797 00798 00799 //! @} core_basic 00800 00801 ///////////////////////// raster image moments ////////////////////////// 00802 00803 //! @addtogroup imgproc_shape 00804 //! @{ 00805 00806 /** @brief struct returned by cv::moments 00807 00808 The spatial moments \f$\texttt{Moments::m}_{ji}\f$ are computed as: 00809 00810 \f[\texttt{m} _{ji}= \sum _{x,y} \left ( \texttt{array} (x,y) \cdot x^j \cdot y^i \right )\f] 00811 00812 The central moments \f$\texttt{Moments::mu}_{ji}\f$ are computed as: 00813 00814 \f[\texttt{mu} _{ji}= \sum _{x,y} \left ( \texttt{array} (x,y) \cdot (x - \bar{x} )^j \cdot (y - \bar{y} )^i \right )\f] 00815 00816 where \f$(\bar{x}, \bar{y})\f$ is the mass center: 00817 00818 \f[\bar{x} = \frac{\texttt{m}_{10}}{\texttt{m}_{00}} , \; \bar{y} = \frac{\texttt{m}_{01}}{\texttt{m}_{00}}\f] 00819 00820 The normalized central moments \f$\texttt{Moments::nu}_{ij}\f$ are computed as: 00821 00822 \f[\texttt{nu} _{ji}= \frac{\texttt{mu}_{ji}}{\texttt{m}_{00}^{(i+j)/2+1}} .\f] 00823 00824 @note 00825 \f$\texttt{mu}_{00}=\texttt{m}_{00}\f$, \f$\texttt{nu}_{00}=1\f$ 00826 \f$\texttt{nu}_{10}=\texttt{mu}_{10}=\texttt{mu}_{01}=\texttt{mu}_{10}=0\f$ , hence the values are not 00827 stored. 00828 00829 The moments of a contour are defined in the same way but computed using the Green's formula (see 00830 <http://en.wikipedia.org/wiki/Green_theorem>). So, due to a limited raster resolution, the moments 00831 computed for a contour are slightly different from the moments computed for the same rasterized 00832 contour. 00833 00834 @note 00835 Since the contour moments are computed using Green formula, you may get seemingly odd results for 00836 contours with self-intersections, e.g. a zero area (m00) for butterfly-shaped contours. 00837 */ 00838 class CV_EXPORTS_W_MAP Moments 00839 { 00840 public: 00841 //! the default constructor 00842 Moments(); 00843 //! the full constructor 00844 Moments(double m00, double m10, double m01, double m20, double m11, 00845 double m02, double m30, double m21, double m12, double m03 ); 00846 ////! the conversion from CvMoments 00847 //Moments( const CvMoments& moments ); 00848 ////! the conversion to CvMoments 00849 //operator CvMoments() const; 00850 00851 //! @name spatial moments 00852 //! @{ 00853 CV_PROP_RW double m00, m10, m01, m20, m11, m02, m30, m21, m12, m03; 00854 //! @} 00855 00856 //! @name central moments 00857 //! @{ 00858 CV_PROP_RW double mu20, mu11, mu02, mu30, mu21, mu12, mu03; 00859 //! @} 00860 00861 //! @name central normalized moments 00862 //! @{ 00863 CV_PROP_RW double nu20, nu11, nu02, nu30, nu21, nu12, nu03; 00864 //! @} 00865 }; 00866 00867 template<> class DataType<Moments> 00868 { 00869 public: 00870 typedef Moments value_type; 00871 typedef double work_type; 00872 typedef double channel_type; 00873 00874 enum { generic_type = 0, 00875 depth = DataType<channel_type>::depth, 00876 channels = (int)(sizeof(value_type)/sizeof(channel_type)), // 24 00877 fmt = DataType<channel_type>::fmt + ((channels - 1) << 8), 00878 type = CV_MAKETYPE(depth, channels) 00879 }; 00880 00881 typedef Vec<channel_type, channels> vec_type; 00882 }; 00883 00884 //! @} imgproc_shape 00885 00886 //! @cond IGNORED 00887 00888 ///////////////////////////////////////////////////////////////////////// 00889 ///////////////////////////// Implementation //////////////////////////// 00890 ///////////////////////////////////////////////////////////////////////// 00891 00892 //////////////////////////////// Complex //////////////////////////////// 00893 00894 template<typename _Tp> inline 00895 Complex<_Tp>::Complex() 00896 : re(0), im(0) {} 00897 00898 template<typename _Tp> inline 00899 Complex<_Tp>::Complex( _Tp _re, _Tp _im ) 00900 : re(_re), im(_im) {} 00901 00902 template<typename _Tp> template<typename T2> inline 00903 Complex<_Tp>::operator Complex<T2>() const 00904 { 00905 return Complex<T2>(saturate_cast<T2>(re), saturate_cast<T2>(im)); 00906 } 00907 00908 template<typename _Tp> inline 00909 Complex<_Tp> Complex<_Tp>::conj() const 00910 { 00911 return Complex<_Tp>(re, -im); 00912 } 00913 00914 00915 template<typename _Tp> static inline 00916 bool operator == (const Complex<_Tp>& a, const Complex<_Tp>& b) 00917 { 00918 return a.re == b.re && a.im == b.im; 00919 } 00920 00921 template<typename _Tp> static inline 00922 bool operator != (const Complex<_Tp>& a, const Complex<_Tp>& b) 00923 { 00924 return a.re != b.re || a.im != b.im; 00925 } 00926 00927 template<typename _Tp> static inline 00928 Complex<_Tp> operator + (const Complex<_Tp>& a, const Complex<_Tp>& b) 00929 { 00930 return Complex<_Tp>( a.re + b.re, a.im + b.im ); 00931 } 00932 00933 template<typename _Tp> static inline 00934 Complex<_Tp>& operator += (Complex<_Tp>& a, const Complex<_Tp>& b) 00935 { 00936 a.re += b.re; a.im += b.im; 00937 return a; 00938 } 00939 00940 template<typename _Tp> static inline 00941 Complex<_Tp> operator - (const Complex<_Tp>& a, const Complex<_Tp>& b) 00942 { 00943 return Complex<_Tp>( a.re - b.re, a.im - b.im ); 00944 } 00945 00946 template<typename _Tp> static inline 00947 Complex<_Tp>& operator -= (Complex<_Tp>& a, const Complex<_Tp>& b) 00948 { 00949 a.re -= b.re; a.im -= b.im; 00950 return a; 00951 } 00952 00953 template<typename _Tp> static inline 00954 Complex<_Tp> operator - (const Complex<_Tp>& a) 00955 { 00956 return Complex<_Tp>(-a.re, -a.im); 00957 } 00958 00959 template<typename _Tp> static inline 00960 Complex<_Tp> operator * (const Complex<_Tp>& a, const Complex<_Tp>& b) 00961 { 00962 return Complex<_Tp>( a.re*b.re - a.im*b.im, a.re*b.im + a.im*b.re ); 00963 } 00964 00965 template<typename _Tp> static inline 00966 Complex<_Tp> operator * (const Complex<_Tp>& a, _Tp b) 00967 { 00968 return Complex<_Tp>( a.re*b, a.im*b ); 00969 } 00970 00971 template<typename _Tp> static inline 00972 Complex<_Tp> operator * (_Tp b, const Complex<_Tp>& a) 00973 { 00974 return Complex<_Tp>( a.re*b, a.im*b ); 00975 } 00976 00977 template<typename _Tp> static inline 00978 Complex<_Tp> operator + (const Complex<_Tp>& a, _Tp b) 00979 { 00980 return Complex<_Tp>( a.re + b, a.im ); 00981 } 00982 00983 template<typename _Tp> static inline 00984 Complex<_Tp> operator - (const Complex<_Tp>& a, _Tp b) 00985 { return Complex<_Tp>( a.re - b, a.im ); } 00986 00987 template<typename _Tp> static inline 00988 Complex<_Tp> operator + (_Tp b, const Complex<_Tp>& a) 00989 { 00990 return Complex<_Tp>( a.re + b, a.im ); 00991 } 00992 00993 template<typename _Tp> static inline 00994 Complex<_Tp> operator - (_Tp b, const Complex<_Tp>& a) 00995 { 00996 return Complex<_Tp>( b - a.re, -a.im ); 00997 } 00998 00999 template<typename _Tp> static inline 01000 Complex<_Tp>& operator += (Complex<_Tp>& a, _Tp b) 01001 { 01002 a.re += b; return a; 01003 } 01004 01005 template<typename _Tp> static inline 01006 Complex<_Tp>& operator -= (Complex<_Tp>& a, _Tp b) 01007 { 01008 a.re -= b; return a; 01009 } 01010 01011 template<typename _Tp> static inline 01012 Complex<_Tp>& operator *= (Complex<_Tp>& a, _Tp b) 01013 { 01014 a.re *= b; a.im *= b; return a; 01015 } 01016 01017 template<typename _Tp> static inline 01018 double abs(const Complex<_Tp>& a) 01019 { 01020 return std::sqrt( (double)a.re*a.re + (double)a.im*a.im); 01021 } 01022 01023 template<typename _Tp> static inline 01024 Complex<_Tp> operator / (const Complex<_Tp>& a, const Complex<_Tp>& b) 01025 { 01026 double t = 1./((double)b.re*b.re + (double)b.im*b.im); 01027 return Complex<_Tp>( (_Tp)((a.re*b.re + a.im*b.im)*t), 01028 (_Tp)((-a.re*b.im + a.im*b.re)*t) ); 01029 } 01030 01031 template<typename _Tp> static inline 01032 Complex<_Tp>& operator /= (Complex<_Tp>& a, const Complex<_Tp>& b) 01033 { 01034 return (a = a / b); 01035 } 01036 01037 template<typename _Tp> static inline 01038 Complex<_Tp> operator / (const Complex<_Tp>& a, _Tp b) 01039 { 01040 _Tp t = (_Tp)1/b; 01041 return Complex<_Tp>( a.re*t, a.im*t ); 01042 } 01043 01044 template<typename _Tp> static inline 01045 Complex<_Tp> operator / (_Tp b, const Complex<_Tp>& a) 01046 { 01047 return Complex<_Tp>(b)/a; 01048 } 01049 01050 template<typename _Tp> static inline 01051 Complex<_Tp> operator /= (const Complex<_Tp>& a, _Tp b) 01052 { 01053 _Tp t = (_Tp)1/b; 01054 a.re *= t; a.im *= t; return a; 01055 } 01056 01057 01058 01059 //////////////////////////////// 2D Point /////////////////////////////// 01060 01061 template<typename _Tp> inline 01062 Point_<_Tp>::Point_() 01063 : x(0), y(0) {} 01064 01065 template<typename _Tp> inline 01066 Point_<_Tp>::Point_(_Tp _x, _Tp _y) 01067 : x(_x), y(_y) {} 01068 01069 template<typename _Tp> inline 01070 Point_<_Tp>::Point_(const Point_& pt) 01071 : x(pt.x), y(pt.y) {} 01072 01073 template<typename _Tp> inline 01074 Point_<_Tp>::Point_(const Size_<_Tp>& sz) 01075 : x(sz.width), y(sz.height) {} 01076 01077 template<typename _Tp> inline 01078 Point_<_Tp>::Point_(const Vec<_Tp,2>& v) 01079 : x(v[0]), y(v[1]) {} 01080 01081 template<typename _Tp> inline 01082 Point_<_Tp>& Point_<_Tp>::operator = (const Point_& pt) 01083 { 01084 x = pt.x; y = pt.y; 01085 return *this; 01086 } 01087 01088 template<typename _Tp> template<typename _Tp2> inline 01089 Point_<_Tp>::operator Point_<_Tp2>() const 01090 { 01091 return Point_<_Tp2>(saturate_cast<_Tp2>(x), saturate_cast<_Tp2>(y)); 01092 } 01093 01094 template<typename _Tp> inline 01095 Point_<_Tp>::operator Vec<_Tp, 2>() const 01096 { 01097 return Vec<_Tp, 2>(x, y); 01098 } 01099 01100 template<typename _Tp> inline 01101 _Tp Point_<_Tp>::dot(const Point_& pt) const 01102 { 01103 return saturate_cast<_Tp>(x*pt.x + y*pt.y); 01104 } 01105 01106 template<typename _Tp> inline 01107 double Point_<_Tp>::ddot(const Point_& pt) const 01108 { 01109 return (double)x*pt.x + (double)y*pt.y; 01110 } 01111 01112 template<typename _Tp> inline 01113 double Point_<_Tp>::cross(const Point_& pt) const 01114 { 01115 return (double)x*pt.y - (double)y*pt.x; 01116 } 01117 01118 template<typename _Tp> inline bool 01119 Point_<_Tp>::inside( const Rect_<_Tp>& r ) const 01120 { 01121 return r.contains(*this); 01122 } 01123 01124 01125 template<typename _Tp> static inline 01126 Point_<_Tp>& operator += (Point_<_Tp>& a, const Point_<_Tp>& b) 01127 { 01128 a.x += b.x; 01129 a.y += b.y; 01130 return a; 01131 } 01132 01133 template<typename _Tp> static inline 01134 Point_<_Tp>& operator -= (Point_<_Tp>& a, const Point_<_Tp>& b) 01135 { 01136 a.x -= b.x; 01137 a.y -= b.y; 01138 return a; 01139 } 01140 01141 template<typename _Tp> static inline 01142 Point_<_Tp>& operator *= (Point_<_Tp>& a, int b) 01143 { 01144 a.x = saturate_cast<_Tp>(a.x * b); 01145 a.y = saturate_cast<_Tp>(a.y * b); 01146 return a; 01147 } 01148 01149 template<typename _Tp> static inline 01150 Point_<_Tp>& operator *= (Point_<_Tp>& a, float b) 01151 { 01152 a.x = saturate_cast<_Tp>(a.x * b); 01153 a.y = saturate_cast<_Tp>(a.y * b); 01154 return a; 01155 } 01156 01157 template<typename _Tp> static inline 01158 Point_<_Tp>& operator *= (Point_<_Tp>& a, double b) 01159 { 01160 a.x = saturate_cast<_Tp>(a.x * b); 01161 a.y = saturate_cast<_Tp>(a.y * b); 01162 return a; 01163 } 01164 01165 template<typename _Tp> static inline 01166 Point_<_Tp>& operator /= (Point_<_Tp>& a, int b) 01167 { 01168 a.x = saturate_cast<_Tp>(a.x / b); 01169 a.y = saturate_cast<_Tp>(a.y / b); 01170 return a; 01171 } 01172 01173 template<typename _Tp> static inline 01174 Point_<_Tp>& operator /= (Point_<_Tp>& a, float b) 01175 { 01176 a.x = saturate_cast<_Tp>(a.x / b); 01177 a.y = saturate_cast<_Tp>(a.y / b); 01178 return a; 01179 } 01180 01181 template<typename _Tp> static inline 01182 Point_<_Tp>& operator /= (Point_<_Tp>& a, double b) 01183 { 01184 a.x = saturate_cast<_Tp>(a.x / b); 01185 a.y = saturate_cast<_Tp>(a.y / b); 01186 return a; 01187 } 01188 01189 template<typename _Tp> static inline 01190 double norm(const Point_<_Tp>& pt) 01191 { 01192 return std::sqrt((double)pt.x*pt.x + (double)pt.y*pt.y); 01193 } 01194 01195 template<typename _Tp> static inline 01196 bool operator == (const Point_<_Tp>& a, const Point_<_Tp>& b) 01197 { 01198 return a.x == b.x && a.y == b.y; 01199 } 01200 01201 template<typename _Tp> static inline 01202 bool operator != (const Point_<_Tp>& a, const Point_<_Tp>& b) 01203 { 01204 return a.x != b.x || a.y != b.y; 01205 } 01206 01207 template<typename _Tp> static inline 01208 Point_<_Tp> operator + (const Point_<_Tp>& a, const Point_<_Tp>& b) 01209 { 01210 return Point_<_Tp>( saturate_cast<_Tp>(a.x + b.x), saturate_cast<_Tp>(a.y + b.y) ); 01211 } 01212 01213 template<typename _Tp> static inline 01214 Point_<_Tp> operator - (const Point_<_Tp>& a, const Point_<_Tp>& b) 01215 { 01216 return Point_<_Tp>( saturate_cast<_Tp>(a.x - b.x), saturate_cast<_Tp>(a.y - b.y) ); 01217 } 01218 01219 template<typename _Tp> static inline 01220 Point_<_Tp> operator - (const Point_<_Tp>& a) 01221 { 01222 return Point_<_Tp>( saturate_cast<_Tp>(-a.x), saturate_cast<_Tp>(-a.y) ); 01223 } 01224 01225 template<typename _Tp> static inline 01226 Point_<_Tp> operator * (const Point_<_Tp>& a, int b) 01227 { 01228 return Point_<_Tp>( saturate_cast<_Tp>(a.x*b), saturate_cast<_Tp>(a.y*b) ); 01229 } 01230 01231 template<typename _Tp> static inline 01232 Point_<_Tp> operator * (int a, const Point_<_Tp>& b) 01233 { 01234 return Point_<_Tp>( saturate_cast<_Tp>(b.x*a), saturate_cast<_Tp>(b.y*a) ); 01235 } 01236 01237 template<typename _Tp> static inline 01238 Point_<_Tp> operator * (const Point_<_Tp>& a, float b) 01239 { 01240 return Point_<_Tp>( saturate_cast<_Tp>(a.x*b), saturate_cast<_Tp>(a.y*b) ); 01241 } 01242 01243 template<typename _Tp> static inline 01244 Point_<_Tp> operator * (float a, const Point_<_Tp>& b) 01245 { 01246 return Point_<_Tp>( saturate_cast<_Tp>(b.x*a), saturate_cast<_Tp>(b.y*a) ); 01247 } 01248 01249 template<typename _Tp> static inline 01250 Point_<_Tp> operator * (const Point_<_Tp>& a, double b) 01251 { 01252 return Point_<_Tp>( saturate_cast<_Tp>(a.x*b), saturate_cast<_Tp>(a.y*b) ); 01253 } 01254 01255 template<typename _Tp> static inline 01256 Point_<_Tp> operator * (double a, const Point_<_Tp>& b) 01257 { 01258 return Point_<_Tp>( saturate_cast<_Tp>(b.x*a), saturate_cast<_Tp>(b.y*a) ); 01259 } 01260 01261 template<typename _Tp> static inline 01262 Point_<_Tp> operator * (const Matx<_Tp, 2, 2>& a, const Point_<_Tp>& b) 01263 { 01264 Matx<_Tp, 2, 1> tmp = a * Vec<_Tp,2>(b.x, b.y); 01265 return Point_<_Tp>(tmp.val[0], tmp.val[1]); 01266 } 01267 01268 template<typename _Tp> static inline 01269 Point3_<_Tp> operator * (const Matx<_Tp, 3, 3>& a, const Point_<_Tp>& b) 01270 { 01271 Matx<_Tp, 3, 1> tmp = a * Vec<_Tp,3>(b.x, b.y, 1); 01272 return Point3_<_Tp>(tmp.val[0], tmp.val[1], tmp.val[2]); 01273 } 01274 01275 template<typename _Tp> static inline 01276 Point_<_Tp> operator / (const Point_<_Tp>& a, int b) 01277 { 01278 Point_<_Tp> tmp(a); 01279 tmp /= b; 01280 return tmp; 01281 } 01282 01283 template<typename _Tp> static inline 01284 Point_<_Tp> operator / (const Point_<_Tp>& a, float b) 01285 { 01286 Point_<_Tp> tmp(a); 01287 tmp /= b; 01288 return tmp; 01289 } 01290 01291 template<typename _Tp> static inline 01292 Point_<_Tp> operator / (const Point_<_Tp>& a, double b) 01293 { 01294 Point_<_Tp> tmp(a); 01295 tmp /= b; 01296 return tmp; 01297 } 01298 01299 01300 01301 //////////////////////////////// 3D Point /////////////////////////////// 01302 01303 template<typename _Tp> inline 01304 Point3_<_Tp>::Point3_() 01305 : x(0), y(0), z(0) {} 01306 01307 template<typename _Tp> inline 01308 Point3_<_Tp>::Point3_(_Tp _x, _Tp _y, _Tp _z) 01309 : x(_x), y(_y), z(_z) {} 01310 01311 template<typename _Tp> inline 01312 Point3_<_Tp>::Point3_(const Point3_& pt) 01313 : x(pt.x), y(pt.y), z(pt.z) {} 01314 01315 template<typename _Tp> inline 01316 Point3_<_Tp>::Point3_(const Point_<_Tp>& pt) 01317 : x(pt.x), y(pt.y), z(_Tp()) {} 01318 01319 template<typename _Tp> inline 01320 Point3_<_Tp>::Point3_(const Vec<_Tp, 3>& v) 01321 : x(v[0]), y(v[1]), z(v[2]) {} 01322 01323 template<typename _Tp> template<typename _Tp2> inline 01324 Point3_<_Tp>::operator Point3_<_Tp2>() const 01325 { 01326 return Point3_<_Tp2>(saturate_cast<_Tp2>(x), saturate_cast<_Tp2>(y), saturate_cast<_Tp2>(z)); 01327 } 01328 01329 template<typename _Tp> inline 01330 Point3_<_Tp>::operator Vec<_Tp, 3>() const 01331 { 01332 return Vec<_Tp, 3>(x, y, z); 01333 } 01334 01335 template<typename _Tp> inline 01336 Point3_<_Tp>& Point3_<_Tp>::operator = (const Point3_& pt) 01337 { 01338 x = pt.x; y = pt.y; z = pt.z; 01339 return *this; 01340 } 01341 01342 template<typename _Tp> inline 01343 _Tp Point3_<_Tp>::dot(const Point3_& pt) const 01344 { 01345 return saturate_cast<_Tp>(x*pt.x + y*pt.y + z*pt.z); 01346 } 01347 01348 template<typename _Tp> inline 01349 double Point3_<_Tp>::ddot(const Point3_& pt) const 01350 { 01351 return (double)x*pt.x + (double)y*pt.y + (double)z*pt.z; 01352 } 01353 01354 template<typename _Tp> inline 01355 Point3_<_Tp> Point3_<_Tp>::cross(const Point3_<_Tp>& pt) const 01356 { 01357 return Point3_<_Tp>(y*pt.z - z*pt.y, z*pt.x - x*pt.z, x*pt.y - y*pt.x); 01358 } 01359 01360 01361 template<typename _Tp> static inline 01362 Point3_<_Tp>& operator += (Point3_<_Tp>& a, const Point3_<_Tp>& b) 01363 { 01364 a.x += b.x; 01365 a.y += b.y; 01366 a.z += b.z; 01367 return a; 01368 } 01369 01370 template<typename _Tp> static inline 01371 Point3_<_Tp>& operator -= (Point3_<_Tp>& a, const Point3_<_Tp>& b) 01372 { 01373 a.x -= b.x; 01374 a.y -= b.y; 01375 a.z -= b.z; 01376 return a; 01377 } 01378 01379 template<typename _Tp> static inline 01380 Point3_<_Tp>& operator *= (Point3_<_Tp>& a, int b) 01381 { 01382 a.x = saturate_cast<_Tp>(a.x * b); 01383 a.y = saturate_cast<_Tp>(a.y * b); 01384 a.z = saturate_cast<_Tp>(a.z * b); 01385 return a; 01386 } 01387 01388 template<typename _Tp> static inline 01389 Point3_<_Tp>& operator *= (Point3_<_Tp>& a, float b) 01390 { 01391 a.x = saturate_cast<_Tp>(a.x * b); 01392 a.y = saturate_cast<_Tp>(a.y * b); 01393 a.z = saturate_cast<_Tp>(a.z * b); 01394 return a; 01395 } 01396 01397 template<typename _Tp> static inline 01398 Point3_<_Tp>& operator *= (Point3_<_Tp>& a, double b) 01399 { 01400 a.x = saturate_cast<_Tp>(a.x * b); 01401 a.y = saturate_cast<_Tp>(a.y * b); 01402 a.z = saturate_cast<_Tp>(a.z * b); 01403 return a; 01404 } 01405 01406 template<typename _Tp> static inline 01407 Point3_<_Tp>& operator /= (Point3_<_Tp>& a, int b) 01408 { 01409 a.x = saturate_cast<_Tp>(a.x / b); 01410 a.y = saturate_cast<_Tp>(a.y / b); 01411 a.z = saturate_cast<_Tp>(a.z / b); 01412 return a; 01413 } 01414 01415 template<typename _Tp> static inline 01416 Point3_<_Tp>& operator /= (Point3_<_Tp>& a, float b) 01417 { 01418 a.x = saturate_cast<_Tp>(a.x / b); 01419 a.y = saturate_cast<_Tp>(a.y / b); 01420 a.z = saturate_cast<_Tp>(a.z / b); 01421 return a; 01422 } 01423 01424 template<typename _Tp> static inline 01425 Point3_<_Tp>& operator /= (Point3_<_Tp>& a, double b) 01426 { 01427 a.x = saturate_cast<_Tp>(a.x / b); 01428 a.y = saturate_cast<_Tp>(a.y / b); 01429 a.z = saturate_cast<_Tp>(a.z / b); 01430 return a; 01431 } 01432 01433 template<typename _Tp> static inline 01434 double norm(const Point3_<_Tp>& pt) 01435 { 01436 return std::sqrt((double)pt.x*pt.x + (double)pt.y*pt.y + (double)pt.z*pt.z); 01437 } 01438 01439 template<typename _Tp> static inline 01440 bool operator == (const Point3_<_Tp>& a, const Point3_<_Tp>& b) 01441 { 01442 return a.x == b.x && a.y == b.y && a.z == b.z; 01443 } 01444 01445 template<typename _Tp> static inline 01446 bool operator != (const Point3_<_Tp>& a, const Point3_<_Tp>& b) 01447 { 01448 return a.x != b.x || a.y != b.y || a.z != b.z; 01449 } 01450 01451 template<typename _Tp> static inline 01452 Point3_<_Tp> operator + (const Point3_<_Tp>& a, const Point3_<_Tp>& b) 01453 { 01454 return Point3_<_Tp>( saturate_cast<_Tp>(a.x + b.x), saturate_cast<_Tp>(a.y + b.y), saturate_cast<_Tp>(a.z + b.z)); 01455 } 01456 01457 template<typename _Tp> static inline 01458 Point3_<_Tp> operator - (const Point3_<_Tp>& a, const Point3_<_Tp>& b) 01459 { 01460 return Point3_<_Tp>( saturate_cast<_Tp>(a.x - b.x), saturate_cast<_Tp>(a.y - b.y), saturate_cast<_Tp>(a.z - b.z)); 01461 } 01462 01463 template<typename _Tp> static inline 01464 Point3_<_Tp> operator - (const Point3_<_Tp>& a) 01465 { 01466 return Point3_<_Tp>( saturate_cast<_Tp>(-a.x), saturate_cast<_Tp>(-a.y), saturate_cast<_Tp>(-a.z) ); 01467 } 01468 01469 template<typename _Tp> static inline 01470 Point3_<_Tp> operator * (const Point3_<_Tp>& a, int b) 01471 { 01472 return Point3_<_Tp>( saturate_cast<_Tp>(a.x*b), saturate_cast<_Tp>(a.y*b), saturate_cast<_Tp>(a.z*b) ); 01473 } 01474 01475 template<typename _Tp> static inline 01476 Point3_<_Tp> operator * (int a, const Point3_<_Tp>& b) 01477 { 01478 return Point3_<_Tp>( saturate_cast<_Tp>(b.x * a), saturate_cast<_Tp>(b.y * a), saturate_cast<_Tp>(b.z * a) ); 01479 } 01480 01481 template<typename _Tp> static inline 01482 Point3_<_Tp> operator * (const Point3_<_Tp>& a, float b) 01483 { 01484 return Point3_<_Tp>( saturate_cast<_Tp>(a.x * b), saturate_cast<_Tp>(a.y * b), saturate_cast<_Tp>(a.z * b) ); 01485 } 01486 01487 template<typename _Tp> static inline 01488 Point3_<_Tp> operator * (float a, const Point3_<_Tp>& b) 01489 { 01490 return Point3_<_Tp>( saturate_cast<_Tp>(b.x * a), saturate_cast<_Tp>(b.y * a), saturate_cast<_Tp>(b.z * a) ); 01491 } 01492 01493 template<typename _Tp> static inline 01494 Point3_<_Tp> operator * (const Point3_<_Tp>& a, double b) 01495 { 01496 return Point3_<_Tp>( saturate_cast<_Tp>(a.x * b), saturate_cast<_Tp>(a.y * b), saturate_cast<_Tp>(a.z * b) ); 01497 } 01498 01499 template<typename _Tp> static inline 01500 Point3_<_Tp> operator * (double a, const Point3_<_Tp>& b) 01501 { 01502 return Point3_<_Tp>( saturate_cast<_Tp>(b.x * a), saturate_cast<_Tp>(b.y * a), saturate_cast<_Tp>(b.z * a) ); 01503 } 01504 01505 template<typename _Tp> static inline 01506 Point3_<_Tp> operator * (const Matx<_Tp, 3, 3>& a, const Point3_<_Tp>& b) 01507 { 01508 Matx<_Tp, 3, 1> tmp = a * Vec<_Tp,3>(b.x, b.y, b.z); 01509 return Point3_<_Tp>(tmp.val[0], tmp.val[1], tmp.val[2]); 01510 } 01511 01512 template<typename _Tp> static inline 01513 Matx<_Tp, 4, 1> operator * (const Matx<_Tp, 4, 4>& a, const Point3_<_Tp>& b) 01514 { 01515 return a * Matx<_Tp, 4, 1>(b.x, b.y, b.z, 1); 01516 } 01517 01518 template<typename _Tp> static inline 01519 Point3_<_Tp> operator / (const Point3_<_Tp>& a, int b) 01520 { 01521 Point3_<_Tp> tmp(a); 01522 tmp /= b; 01523 return tmp; 01524 } 01525 01526 template<typename _Tp> static inline 01527 Point3_<_Tp> operator / (const Point3_<_Tp>& a, float b) 01528 { 01529 Point3_<_Tp> tmp(a); 01530 tmp /= b; 01531 return tmp; 01532 } 01533 01534 template<typename _Tp> static inline 01535 Point3_<_Tp> operator / (const Point3_<_Tp>& a, double b) 01536 { 01537 Point3_<_Tp> tmp(a); 01538 tmp /= b; 01539 return tmp; 01540 } 01541 01542 01543 01544 ////////////////////////////////// Size ///////////////////////////////// 01545 01546 template<typename _Tp> inline 01547 Size_<_Tp>::Size_() 01548 : width(0), height(0) {} 01549 01550 template<typename _Tp> inline 01551 Size_<_Tp>::Size_(_Tp _width, _Tp _height) 01552 : width(_width), height(_height) {} 01553 01554 template<typename _Tp> inline 01555 Size_<_Tp>::Size_(const Size_& sz) 01556 : width(sz.width), height(sz.height) {} 01557 01558 template<typename _Tp> inline 01559 Size_<_Tp>::Size_(const Point_<_Tp>& pt) 01560 : width(pt.x), height(pt.y) {} 01561 01562 template<typename _Tp> template<typename _Tp2> inline 01563 Size_<_Tp>::operator Size_<_Tp2>() const 01564 { 01565 return Size_<_Tp2>(saturate_cast<_Tp2>(width), saturate_cast<_Tp2>(height)); 01566 } 01567 01568 template<typename _Tp> inline 01569 Size_<_Tp>& Size_<_Tp>::operator = (const Size_<_Tp>& sz) 01570 { 01571 width = sz.width; height = sz.height; 01572 return *this; 01573 } 01574 01575 template<typename _Tp> inline 01576 _Tp Size_<_Tp>::area() const 01577 { 01578 return width * height; 01579 } 01580 01581 template<typename _Tp> static inline 01582 Size_<_Tp>& operator *= (Size_<_Tp>& a, _Tp b) 01583 { 01584 a.width *= b; 01585 a.height *= b; 01586 return a; 01587 } 01588 01589 template<typename _Tp> static inline 01590 Size_<_Tp> operator * (const Size_<_Tp>& a, _Tp b) 01591 { 01592 Size_<_Tp> tmp(a); 01593 tmp *= b; 01594 return tmp; 01595 } 01596 01597 template<typename _Tp> static inline 01598 Size_<_Tp>& operator /= (Size_<_Tp>& a, _Tp b) 01599 { 01600 a.width /= b; 01601 a.height /= b; 01602 return a; 01603 } 01604 01605 template<typename _Tp> static inline 01606 Size_<_Tp> operator / (const Size_<_Tp>& a, _Tp b) 01607 { 01608 Size_<_Tp> tmp(a); 01609 tmp /= b; 01610 return tmp; 01611 } 01612 01613 template<typename _Tp> static inline 01614 Size_<_Tp>& operator += (Size_<_Tp>& a, const Size_<_Tp>& b) 01615 { 01616 a.width += b.width; 01617 a.height += b.height; 01618 return a; 01619 } 01620 01621 template<typename _Tp> static inline 01622 Size_<_Tp> operator + (const Size_<_Tp>& a, const Size_<_Tp>& b) 01623 { 01624 Size_<_Tp> tmp(a); 01625 tmp += b; 01626 return tmp; 01627 } 01628 01629 template<typename _Tp> static inline 01630 Size_<_Tp>& operator -= (Size_<_Tp>& a, const Size_<_Tp>& b) 01631 { 01632 a.width -= b.width; 01633 a.height -= b.height; 01634 return a; 01635 } 01636 01637 template<typename _Tp> static inline 01638 Size_<_Tp> operator - (const Size_<_Tp>& a, const Size_<_Tp>& b) 01639 { 01640 Size_<_Tp> tmp(a); 01641 tmp -= b; 01642 return tmp; 01643 } 01644 01645 template<typename _Tp> static inline 01646 bool operator == (const Size_<_Tp>& a, const Size_<_Tp>& b) 01647 { 01648 return a.width == b.width && a.height == b.height; 01649 } 01650 01651 template<typename _Tp> static inline 01652 bool operator != (const Size_<_Tp>& a, const Size_<_Tp>& b) 01653 { 01654 return !(a == b); 01655 } 01656 01657 01658 01659 ////////////////////////////////// Rect ///////////////////////////////// 01660 01661 template<typename _Tp> inline 01662 Rect_<_Tp>::Rect_() 01663 : x(0), y(0), width(0), height(0) {} 01664 01665 template<typename _Tp> inline 01666 Rect_<_Tp>::Rect_(_Tp _x, _Tp _y, _Tp _width, _Tp _height) 01667 : x(_x), y(_y), width(_width), height(_height) {} 01668 01669 template<typename _Tp> inline 01670 Rect_<_Tp>::Rect_(const Rect_<_Tp>& r) 01671 : x(r.x), y(r.y), width(r.width), height(r.height) {} 01672 01673 template<typename _Tp> inline 01674 Rect_<_Tp>::Rect_(const Point_<_Tp>& org, const Size_<_Tp>& sz) 01675 : x(org.x), y(org.y), width(sz.width), height(sz.height) {} 01676 01677 template<typename _Tp> inline 01678 Rect_<_Tp>::Rect_(const Point_<_Tp>& pt1, const Point_<_Tp>& pt2) 01679 { 01680 x = std::min(pt1.x, pt2.x); 01681 y = std::min(pt1.y, pt2.y); 01682 width = std::max(pt1.x, pt2.x) - x; 01683 height = std::max(pt1.y, pt2.y) - y; 01684 } 01685 01686 template<typename _Tp> inline 01687 Rect_<_Tp>& Rect_<_Tp>::operator = ( const Rect_<_Tp>& r ) 01688 { 01689 x = r.x; 01690 y = r.y; 01691 width = r.width; 01692 height = r.height; 01693 return *this; 01694 } 01695 01696 template<typename _Tp> inline 01697 Point_<_Tp> Rect_<_Tp>::tl() const 01698 { 01699 return Point_<_Tp>(x,y); 01700 } 01701 01702 template<typename _Tp> inline 01703 Point_<_Tp> Rect_<_Tp>::br() const 01704 { 01705 return Point_<_Tp>(x + width, y + height); 01706 } 01707 01708 template<typename _Tp> inline 01709 Size_<_Tp> Rect_<_Tp>::size() const 01710 { 01711 return Size_<_Tp>(width, height); 01712 } 01713 01714 template<typename _Tp> inline 01715 _Tp Rect_<_Tp>::area() const 01716 { 01717 return width * height; 01718 } 01719 01720 template<typename _Tp> template<typename _Tp2> inline 01721 Rect_<_Tp>::operator Rect_<_Tp2>() const 01722 { 01723 return Rect_<_Tp2>(saturate_cast<_Tp2>(x), saturate_cast<_Tp2>(y), saturate_cast<_Tp2>(width), saturate_cast<_Tp2>(height)); 01724 } 01725 01726 template<typename _Tp> inline 01727 bool Rect_<_Tp>::contains(const Point_<_Tp>& pt) const 01728 { 01729 return x <= pt.x && pt.x < x + width && y <= pt.y && pt.y < y + height; 01730 } 01731 01732 01733 template<typename _Tp> static inline 01734 Rect_<_Tp>& operator += ( Rect_<_Tp>& a, const Point_<_Tp>& b ) 01735 { 01736 a.x += b.x; 01737 a.y += b.y; 01738 return a; 01739 } 01740 01741 template<typename _Tp> static inline 01742 Rect_<_Tp>& operator -= ( Rect_<_Tp>& a, const Point_<_Tp>& b ) 01743 { 01744 a.x -= b.x; 01745 a.y -= b.y; 01746 return a; 01747 } 01748 01749 template<typename _Tp> static inline 01750 Rect_<_Tp>& operator += ( Rect_<_Tp>& a, const Size_<_Tp>& b ) 01751 { 01752 a.width += b.width; 01753 a.height += b.height; 01754 return a; 01755 } 01756 01757 template<typename _Tp> static inline 01758 Rect_<_Tp>& operator -= ( Rect_<_Tp>& a, const Size_<_Tp>& b ) 01759 { 01760 a.width -= b.width; 01761 a.height -= b.height; 01762 return a; 01763 } 01764 01765 template<typename _Tp> static inline 01766 Rect_<_Tp>& operator &= ( Rect_<_Tp>& a, const Rect_<_Tp>& b ) 01767 { 01768 _Tp x1 = std::max(a.x, b.x); 01769 _Tp y1 = std::max(a.y, b.y); 01770 a.width = std::min(a.x + a.width, b.x + b.width) - x1; 01771 a.height = std::min(a.y + a.height, b.y + b.height) - y1; 01772 a.x = x1; 01773 a.y = y1; 01774 if( a.width <= 0 || a.height <= 0 ) 01775 a = Rect(); 01776 return a; 01777 } 01778 01779 template<typename _Tp> static inline 01780 Rect_<_Tp>& operator |= ( Rect_<_Tp>& a, const Rect_<_Tp>& b ) 01781 { 01782 _Tp x1 = std::min(a.x, b.x); 01783 _Tp y1 = std::min(a.y, b.y); 01784 a.width = std::max(a.x + a.width, b.x + b.width) - x1; 01785 a.height = std::max(a.y + a.height, b.y + b.height) - y1; 01786 a.x = x1; 01787 a.y = y1; 01788 return a; 01789 } 01790 01791 template<typename _Tp> static inline 01792 bool operator == (const Rect_<_Tp>& a, const Rect_<_Tp>& b) 01793 { 01794 return a.x == b.x && a.y == b.y && a.width == b.width && a.height == b.height; 01795 } 01796 01797 template<typename _Tp> static inline 01798 bool operator != (const Rect_<_Tp>& a, const Rect_<_Tp>& b) 01799 { 01800 return a.x != b.x || a.y != b.y || a.width != b.width || a.height != b.height; 01801 } 01802 01803 template<typename _Tp> static inline 01804 Rect_<_Tp> operator + (const Rect_<_Tp>& a, const Point_<_Tp>& b) 01805 { 01806 return Rect_<_Tp>( a.x + b.x, a.y + b.y, a.width, a.height ); 01807 } 01808 01809 template<typename _Tp> static inline 01810 Rect_<_Tp> operator - (const Rect_<_Tp>& a, const Point_<_Tp>& b) 01811 { 01812 return Rect_<_Tp>( a.x - b.x, a.y - b.y, a.width, a.height ); 01813 } 01814 01815 template<typename _Tp> static inline 01816 Rect_<_Tp> operator + (const Rect_<_Tp>& a, const Size_<_Tp>& b) 01817 { 01818 return Rect_<_Tp>( a.x, a.y, a.width + b.width, a.height + b.height ); 01819 } 01820 01821 template<typename _Tp> static inline 01822 Rect_<_Tp> operator & (const Rect_<_Tp>& a, const Rect_<_Tp>& b) 01823 { 01824 Rect_<_Tp> c = a; 01825 return c &= b; 01826 } 01827 01828 template<typename _Tp> static inline 01829 Rect_<_Tp> operator | (const Rect_<_Tp>& a, const Rect_<_Tp>& b) 01830 { 01831 Rect_<_Tp> c = a; 01832 return c |= b; 01833 } 01834 01835 01836 01837 ////////////////////////////// RotatedRect ////////////////////////////// 01838 01839 inline 01840 RotatedRect::RotatedRect() 01841 : center(), size(), angle(0) {} 01842 01843 inline 01844 RotatedRect::RotatedRect(const Point2f& _center, const Size2f& _size, float _angle) 01845 : center(_center), size(_size), angle(_angle) {} 01846 01847 01848 01849 ///////////////////////////////// Range ///////////////////////////////// 01850 01851 inline 01852 Range::Range() 01853 : start(0), end(0) {} 01854 01855 inline 01856 Range::Range(int _start, int _end) 01857 : start(_start), end(_end) {} 01858 01859 inline 01860 int Range::size() const 01861 { 01862 return end - start; 01863 } 01864 01865 inline 01866 bool Range::empty() const 01867 { 01868 return start == end; 01869 } 01870 01871 inline 01872 Range Range::all() 01873 { 01874 return Range(INT_MIN, INT_MAX); 01875 } 01876 01877 01878 static inline 01879 bool operator == (const Range& r1, const Range& r2) 01880 { 01881 return r1.start == r2.start && r1.end == r2.end; 01882 } 01883 01884 static inline 01885 bool operator != (const Range& r1, const Range& r2) 01886 { 01887 return !(r1 == r2); 01888 } 01889 01890 static inline 01891 bool operator !(const Range& r) 01892 { 01893 return r.start == r.end; 01894 } 01895 01896 static inline 01897 Range operator & (const Range& r1, const Range& r2) 01898 { 01899 Range r(std::max(r1.start, r2.start), std::min(r1.end, r2.end)); 01900 r.end = std::max(r.end, r.start); 01901 return r; 01902 } 01903 01904 static inline 01905 Range& operator &= (Range& r1, const Range& r2) 01906 { 01907 r1 = r1 & r2; 01908 return r1; 01909 } 01910 01911 static inline 01912 Range operator + (const Range& r1, int delta) 01913 { 01914 return Range(r1.start + delta, r1.end + delta); 01915 } 01916 01917 static inline 01918 Range operator + (int delta, const Range& r1) 01919 { 01920 return Range(r1.start + delta, r1.end + delta); 01921 } 01922 01923 static inline 01924 Range operator - (const Range& r1, int delta) 01925 { 01926 return r1 + (-delta); 01927 } 01928 01929 01930 01931 ///////////////////////////////// Scalar //////////////////////////////// 01932 01933 template<typename _Tp> inline 01934 Scalar_<_Tp>::Scalar_() 01935 { 01936 this->val[0] = this->val[1] = this->val[2] = this->val[3] = 0; 01937 } 01938 01939 template<typename _Tp> inline 01940 Scalar_<_Tp>::Scalar_(_Tp v0, _Tp v1, _Tp v2, _Tp v3) 01941 { 01942 this->val[0] = v0; 01943 this->val[1] = v1; 01944 this->val[2] = v2; 01945 this->val[3] = v3; 01946 } 01947 01948 template<typename _Tp> template<typename _Tp2, int cn> inline 01949 Scalar_<_Tp>::Scalar_(const Vec<_Tp2, cn>& v) 01950 { 01951 int i; 01952 for( i = 0; i < (cn < 4 ? cn : 4); i++ ) 01953 this->val[i] = cv::saturate_cast<_Tp>(v.val[i]); 01954 for( ; i < 4; i++ ) 01955 this->val[i] = 0; 01956 } 01957 01958 template<typename _Tp> inline 01959 Scalar_<_Tp>::Scalar_(_Tp v0) 01960 { 01961 this->val[0] = v0; 01962 this->val[1] = this->val[2] = this->val[3] = 0; 01963 } 01964 01965 template<typename _Tp> inline 01966 Scalar_<_Tp> Scalar_<_Tp>::all(_Tp v0) 01967 { 01968 return Scalar_<_Tp>(v0, v0, v0, v0); 01969 } 01970 01971 01972 template<typename _Tp> inline 01973 Scalar_<_Tp> Scalar_<_Tp>::mul(const Scalar_<_Tp>& a, double scale ) const 01974 { 01975 return Scalar_<_Tp>(saturate_cast<_Tp>(this->val[0] * a.val[0] * scale), 01976 saturate_cast<_Tp>(this->val[1] * a.val[1] * scale), 01977 saturate_cast<_Tp>(this->val[2] * a.val[2] * scale), 01978 saturate_cast<_Tp>(this->val[3] * a.val[3] * scale)); 01979 } 01980 01981 template<typename _Tp> inline 01982 Scalar_<_Tp> Scalar_<_Tp>::conj() const 01983 { 01984 return Scalar_<_Tp>(saturate_cast<_Tp>( this->val[0]), 01985 saturate_cast<_Tp>(-this->val[1]), 01986 saturate_cast<_Tp>(-this->val[2]), 01987 saturate_cast<_Tp>(-this->val[3])); 01988 } 01989 01990 template<typename _Tp> inline 01991 bool Scalar_<_Tp>::isReal() const 01992 { 01993 return this->val[1] == 0 && this->val[2] == 0 && this->val[3] == 0; 01994 } 01995 01996 01997 template<typename _Tp> template<typename T2> inline 01998 Scalar_<_Tp>::operator Scalar_<T2>() const 01999 { 02000 return Scalar_<T2>(saturate_cast<T2>(this->val[0]), 02001 saturate_cast<T2>(this->val[1]), 02002 saturate_cast<T2>(this->val[2]), 02003 saturate_cast<T2>(this->val[3])); 02004 } 02005 02006 02007 template<typename _Tp> static inline 02008 Scalar_<_Tp>& operator += (Scalar_<_Tp>& a, const Scalar_<_Tp>& b) 02009 { 02010 a.val[0] += b.val[0]; 02011 a.val[1] += b.val[1]; 02012 a.val[2] += b.val[2]; 02013 a.val[3] += b.val[3]; 02014 return a; 02015 } 02016 02017 template<typename _Tp> static inline 02018 Scalar_<_Tp>& operator -= (Scalar_<_Tp>& a, const Scalar_<_Tp>& b) 02019 { 02020 a.val[0] -= b.val[0]; 02021 a.val[1] -= b.val[1]; 02022 a.val[2] -= b.val[2]; 02023 a.val[3] -= b.val[3]; 02024 return a; 02025 } 02026 02027 template<typename _Tp> static inline 02028 Scalar_<_Tp>& operator *= ( Scalar_<_Tp>& a, _Tp v ) 02029 { 02030 a.val[0] *= v; 02031 a.val[1] *= v; 02032 a.val[2] *= v; 02033 a.val[3] *= v; 02034 return a; 02035 } 02036 02037 template<typename _Tp> static inline 02038 bool operator == ( const Scalar_<_Tp>& a, const Scalar_<_Tp>& b ) 02039 { 02040 return a.val[0] == b.val[0] && a.val[1] == b.val[1] && 02041 a.val[2] == b.val[2] && a.val[3] == b.val[3]; 02042 } 02043 02044 template<typename _Tp> static inline 02045 bool operator != ( const Scalar_<_Tp>& a, const Scalar_<_Tp>& b ) 02046 { 02047 return a.val[0] != b.val[0] || a.val[1] != b.val[1] || 02048 a.val[2] != b.val[2] || a.val[3] != b.val[3]; 02049 } 02050 02051 template<typename _Tp> static inline 02052 Scalar_<_Tp> operator + (const Scalar_<_Tp>& a, const Scalar_<_Tp>& b) 02053 { 02054 return Scalar_<_Tp>(a.val[0] + b.val[0], 02055 a.val[1] + b.val[1], 02056 a.val[2] + b.val[2], 02057 a.val[3] + b.val[3]); 02058 } 02059 02060 template<typename _Tp> static inline 02061 Scalar_<_Tp> operator - (const Scalar_<_Tp>& a, const Scalar_<_Tp>& b) 02062 { 02063 return Scalar_<_Tp>(saturate_cast<_Tp>(a.val[0] - b.val[0]), 02064 saturate_cast<_Tp>(a.val[1] - b.val[1]), 02065 saturate_cast<_Tp>(a.val[2] - b.val[2]), 02066 saturate_cast<_Tp>(a.val[3] - b.val[3])); 02067 } 02068 02069 template<typename _Tp> static inline 02070 Scalar_<_Tp> operator * (const Scalar_<_Tp>& a, _Tp alpha) 02071 { 02072 return Scalar_<_Tp>(a.val[0] * alpha, 02073 a.val[1] * alpha, 02074 a.val[2] * alpha, 02075 a.val[3] * alpha); 02076 } 02077 02078 template<typename _Tp> static inline 02079 Scalar_<_Tp> operator * (_Tp alpha, const Scalar_<_Tp>& a) 02080 { 02081 return a*alpha; 02082 } 02083 02084 template<typename _Tp> static inline 02085 Scalar_<_Tp> operator - (const Scalar_<_Tp>& a) 02086 { 02087 return Scalar_<_Tp>(saturate_cast<_Tp>(-a.val[0]), 02088 saturate_cast<_Tp>(-a.val[1]), 02089 saturate_cast<_Tp>(-a.val[2]), 02090 saturate_cast<_Tp>(-a.val[3])); 02091 } 02092 02093 02094 template<typename _Tp> static inline 02095 Scalar_<_Tp> operator * (const Scalar_<_Tp>& a, const Scalar_<_Tp>& b) 02096 { 02097 return Scalar_<_Tp>(saturate_cast<_Tp>(a[0]*b[0] - a[1]*b[1] - a[2]*b[2] - a[3]*b[3]), 02098 saturate_cast<_Tp>(a[0]*b[1] + a[1]*b[0] + a[2]*b[3] - a[3]*b[2]), 02099 saturate_cast<_Tp>(a[0]*b[2] - a[1]*b[3] + a[2]*b[0] + a[3]*b[1]), 02100 saturate_cast<_Tp>(a[0]*b[3] + a[1]*b[2] - a[2]*b[1] + a[3]*b[0])); 02101 } 02102 02103 template<typename _Tp> static inline 02104 Scalar_<_Tp>& operator *= (Scalar_<_Tp>& a, const Scalar_<_Tp>& b) 02105 { 02106 a = a * b; 02107 return a; 02108 } 02109 02110 template<typename _Tp> static inline 02111 Scalar_<_Tp> operator / (const Scalar_<_Tp>& a, _Tp alpha) 02112 { 02113 return Scalar_<_Tp>(a.val[0] / alpha, 02114 a.val[1] / alpha, 02115 a.val[2] / alpha, 02116 a.val[3] / alpha); 02117 } 02118 02119 template<typename _Tp> static inline 02120 Scalar_<float> operator / (const Scalar_<float>& a, float alpha) 02121 { 02122 float s = 1 / alpha; 02123 return Scalar_<float>(a.val[0] * s, a.val[1] * s, a.val[2] * s, a.val[3] * s); 02124 } 02125 02126 template<typename _Tp> static inline 02127 Scalar_<double> operator / (const Scalar_<double>& a, double alpha) 02128 { 02129 double s = 1 / alpha; 02130 return Scalar_<double>(a.val[0] * s, a.val[1] * s, a.val[2] * s, a.val[3] * s); 02131 } 02132 02133 template<typename _Tp> static inline 02134 Scalar_<_Tp>& operator /= (Scalar_<_Tp>& a, _Tp alpha) 02135 { 02136 a = a / alpha; 02137 return a; 02138 } 02139 02140 template<typename _Tp> static inline 02141 Scalar_<_Tp> operator / (_Tp a, const Scalar_<_Tp>& b) 02142 { 02143 _Tp s = a / (b[0]*b[0] + b[1]*b[1] + b[2]*b[2] + b[3]*b[3]); 02144 return b.conj() * s; 02145 } 02146 02147 template<typename _Tp> static inline 02148 Scalar_<_Tp> operator / (const Scalar_<_Tp>& a, const Scalar_<_Tp>& b) 02149 { 02150 return a * ((_Tp)1 / b); 02151 } 02152 02153 template<typename _Tp> static inline 02154 Scalar_<_Tp>& operator /= (Scalar_<_Tp>& a, const Scalar_<_Tp>& b) 02155 { 02156 a = a / b; 02157 return a; 02158 } 02159 02160 template<typename _Tp> static inline 02161 Scalar operator * (const Matx<_Tp, 4, 4>& a, const Scalar& b) 02162 { 02163 Matx<double, 4, 1> c((Matx<double, 4, 4>)a, b, Matx_MatMulOp()); 02164 return reinterpret_cast<const Scalar&>(c); 02165 } 02166 02167 template<> inline 02168 Scalar operator * (const Matx<double, 4, 4>& a, const Scalar& b) 02169 { 02170 Matx<double, 4, 1> c(a, b, Matx_MatMulOp()); 02171 return reinterpret_cast<const Scalar&>(c); 02172 } 02173 02174 02175 02176 //////////////////////////////// KeyPoint /////////////////////////////// 02177 02178 inline 02179 KeyPoint::KeyPoint() 02180 : pt(0,0), size(0), angle(-1), response(0), octave(0), class_id(-1) {} 02181 02182 inline 02183 KeyPoint::KeyPoint(Point2f _pt, float _size, float _angle, float _response, int _octave, int _class_id) 02184 : pt(_pt), size(_size), angle(_angle), response(_response), octave(_octave), class_id(_class_id) {} 02185 02186 inline 02187 KeyPoint::KeyPoint(float x, float y, float _size, float _angle, float _response, int _octave, int _class_id) 02188 : pt(x, y), size(_size), angle(_angle), response(_response), octave(_octave), class_id(_class_id) {} 02189 02190 02191 02192 ///////////////////////////////// DMatch //////////////////////////////// 02193 02194 inline 02195 DMatch::DMatch() 02196 : queryIdx(-1), trainIdx(-1), imgIdx(-1), distance(FLT_MAX) {} 02197 02198 inline 02199 DMatch::DMatch(int _queryIdx, int _trainIdx, float _distance) 02200 : queryIdx(_queryIdx), trainIdx(_trainIdx), imgIdx(-1), distance(_distance) {} 02201 02202 inline 02203 DMatch::DMatch(int _queryIdx, int _trainIdx, int _imgIdx, float _distance) 02204 : queryIdx(_queryIdx), trainIdx(_trainIdx), imgIdx(_imgIdx), distance(_distance) {} 02205 02206 inline 02207 bool DMatch::operator < (const DMatch &m) const 02208 { 02209 return distance < m.distance; 02210 } 02211 02212 02213 02214 ////////////////////////////// TermCriteria ///////////////////////////// 02215 02216 inline 02217 TermCriteria::TermCriteria() 02218 : type(0), maxCount(0), epsilon(0) {} 02219 02220 inline 02221 TermCriteria::TermCriteria(int _type, int _maxCount, double _epsilon) 02222 : type(_type), maxCount(_maxCount), epsilon(_epsilon) {} 02223 02224 //! @endcond 02225 02226 } // cv 02227 02228 #endif //__OPENCV_CORE_TYPES_HPP__ 02229
Generated on Tue Jul 12 2022 16:42:40 by
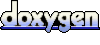