added debugging
Fork of BLE_nRF8001 by
Embed:
(wiki syntax)
Show/hide line numbers
hal_aci_tl.h
Go to the documentation of this file.
00001 /* Copyright (c) 2014, Nordic Semiconductor ASA 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy 00004 * of this software and associated documentation files (the "Software"), to deal 00005 * in the Software without restriction, including without limitation the rights 00006 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00007 * copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all 00011 * copies or substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00014 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00015 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00016 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00017 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00018 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00019 * SOFTWARE. 00020 */ 00021 00022 /** @file 00023 * @brief Interface for hal_aci_tl. 00024 */ 00025 00026 /** @defgroup hal_aci_tl hal_aci_tl 00027 @{ 00028 @ingroup hal 00029 00030 @brief Module for the ACI Transport Layer interface 00031 @details This module is responsible for sending and receiving messages over the ACI interface of the nRF8001 chip. 00032 The hal_aci_tl_send_cmd() can be called directly to send ACI commands. 00033 00034 00035 The RDYN line is hooked to an interrupt on the MCU when the level is low. 00036 The SPI master clocks in the interrupt context. 00037 The ACI Command is taken from the head of the command queue is sent over the SPI 00038 and the received ACI event is placed in the tail of the event queue. 00039 00040 */ 00041 00042 #ifndef HAL_ACI_TL_H__ 00043 #define HAL_ACI_TL_H__ 00044 00045 #include "hal_platform.h " 00046 #include "aci.h " 00047 #include "boards.h" 00048 00049 #ifndef HAL_ACI_MAX_LENGTH 00050 #define HAL_ACI_MAX_LENGTH 31 00051 #endif 00052 00053 /************************************************************************/ 00054 /* Unused nRF8001 pin */ 00055 /************************************************************************/ 00056 #define UNUSED 255 00057 00058 /** Data type for ACI commands and events */ 00059 typedef struct { 00060 uint8_t status_byte; 00061 uint8_t buffer[HAL_ACI_MAX_LENGTH+1]; 00062 } _aci_packed_ hal_aci_data_t; 00063 00064 ACI_ASSERT_SIZE(hal_aci_data_t, HAL_ACI_MAX_LENGTH + 2); 00065 00066 /** Datatype for ACI pins and interface (polling/interrupt)*/ 00067 typedef struct aci_pins_t 00068 { 00069 uint8_t board_name; //Optional : Use BOARD_DEFAULT if you do not know. See boards.h 00070 DigitalInOut *reqn_pin; //Required 00071 DigitalInOut *rdyn_pin; //Required 00072 00073 DigitalInOut *reset_pin; //Recommended but optional - Set it to UNUSED when not connected 00074 DigitalInOut *active_pin; //Optional - Set it to UNUSED when not connected 00075 00076 bool interface_is_interrupt; //Required - true = Uses interrupt on RDYN pin. false - Uses polling on RDYN pin 00077 00078 uint8_t interrupt_number; //Required when using interrupts, otherwise ignored 00079 } aci_pins_t; 00080 00081 /** @brief ACI Transport Layer initialization. 00082 * @details 00083 * This function initializes the transport layer, including configuring the SPI, creating 00084 * message queues for Commands and Events and setting up interrupt if required. 00085 * @param a_pins Pins on the MCU used to connect to the nRF8001 00086 * @param bool True if debug printing should be enabled on the Serial. 00087 */ 00088 void hal_aci_tl_init(aci_pins_t *a_pins, bool debug); 00089 00090 /** @brief Sends an ACI command to the radio. 00091 * @details 00092 * This function sends an ACI command to the radio. This queue up the message to send and 00093 * lower the request line. When the device lowers the ready line, @ref m_aci_spi_transfer() 00094 * will send the data. 00095 * @param aci_buffer Pointer to the message to send. 00096 * @return True if the data was successfully queued for sending, 00097 * false if there is no more space to store messages to send. 00098 */ 00099 bool hal_aci_tl_send(hal_aci_data_t *aci_buffer); 00100 00101 /** @brief Process pending transactions. 00102 * @details 00103 * The library code takes care of calling this function to check if the nRF8001 RDYN line indicates a 00104 * pending transaction. It will send a pending message if there is one and return any receive message 00105 * that was pending. 00106 * @return Points to data buffer for received data. Length byte in buffer is 0 if no data received. 00107 */ 00108 hal_aci_data_t * hal_aci_tl_poll_get(void); 00109 00110 /** @brief Get an ACI event from the event queue 00111 * @details 00112 * Call this function from the main context to get an event from the ACI event queue 00113 * This is called by lib_aci_event_get 00114 */ 00115 bool hal_aci_tl_event_get(hal_aci_data_t *p_aci_data); 00116 00117 /** @brief Peek an ACI event from the event queue 00118 * @details 00119 * Call this function from the main context to peek an event from the ACI event queue. 00120 * This is called by lib_aci_event_peek 00121 */ 00122 bool hal_aci_tl_event_peek(hal_aci_data_t *p_aci_data); 00123 00124 /** @brief Enable debug printing of all ACI commands sent and ACI events received 00125 * @details 00126 * when the enable parameter is true. The debug printing is enabled on the Serial. 00127 * When the enable parameter is false. The debug printing is disabled on the Serial. 00128 * By default the debug printing is disabled. 00129 */ 00130 void hal_aci_tl_debug_print(bool enable); 00131 00132 00133 /** @brief Pin reset the nRF8001 00134 * @details 00135 * The reset line of the nF8001 needs to kept low for 200 ns. 00136 * Redbearlab shield v1.1 and v2012.07 are exceptions as they 00137 * have a Power ON Reset circuit that works differently. 00138 * The function handles the exceptions based on the board_name in aci_pins_t 00139 */ 00140 void hal_aci_tl_pin_reset(void); 00141 00142 /** @brief Return full status of transmit queue 00143 * @details 00144 * 00145 */ 00146 bool hal_aci_tl_rx_q_full(void); 00147 00148 /** @brief Return empty status of receive queue 00149 * @details 00150 * 00151 */ 00152 bool hal_aci_tl_rx_q_empty(void); 00153 00154 /** @brief Return full status of receive queue 00155 * @details 00156 * 00157 */ 00158 bool hal_aci_tl_tx_q_full(void); 00159 00160 /** @brief Return empty status of transmit queue 00161 * @details 00162 * 00163 */ 00164 bool hal_aci_tl_tx_q_empty(void); 00165 00166 /** @brief Flush the ACI command Queue and the ACI Event Queue 00167 * @details 00168 * Call this function in the main thread 00169 */ 00170 void hal_aci_tl_q_flush(void); 00171 00172 #endif // HAL_ACI_TL_H__ 00173 /** @} */
Generated on Tue Jul 12 2022 15:15:46 by
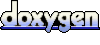