added debugging
Fork of BLE_nRF8001 by
Embed:
(wiki syntax)
Show/hide line numbers
aci_setup.cpp
00001 /* Copyright (c) 2014, Nordic Semiconductor ASA 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy 00004 * of this software and associated documentation files (the "Software"), to deal 00005 * in the Software without restriction, including without limitation the rights 00006 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00007 * copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all 00011 * copies or substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00014 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00015 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00016 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00017 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00018 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00019 * SOFTWARE. 00020 */ 00021 00022 #include <lib_aci.h> 00023 #include "aci_setup.h" 00024 00025 00026 // aci_struct that will contain 00027 // total initial credits 00028 // current credit 00029 // current state of the aci (setup/standby/active/sleep) 00030 // open remote pipe pending 00031 // close remote pipe pending 00032 // Current pipe available bitmap 00033 // Current pipe closed bitmap 00034 // Current connection interval, slave latency and link supervision timeout 00035 // Current State of the the GATT client (Service Discovery status) 00036 00037 00038 extern hal_aci_data_t msg_to_send; 00039 00040 00041 00042 /************************************************************************** */ 00043 /* Utility function to fill the the ACI command queue */ 00044 /* aci_stat Pointer to the ACI state */ 00045 /* num_cmd_offset(in/out) Offset in the Setup message array to start from */ 00046 /* offset is updated to the new index after the queue is filled */ 00047 /* or the last message us placed in the queue */ 00048 /* Returns true if at least one message was transferred */ 00049 /***************************************************************************/ 00050 static bool aci_setup_fill(aci_state_t *aci_stat, uint8_t *num_cmd_offset) 00051 { 00052 bool ret_val = false; 00053 00054 while (*num_cmd_offset < aci_stat->aci_setup_info.num_setup_msgs) 00055 { 00056 //Board dependent defines 00057 memcpy(&msg_to_send, &(aci_stat->aci_setup_info.setup_msgs[*num_cmd_offset]), 00058 (aci_stat->aci_setup_info.setup_msgs[*num_cmd_offset].buffer[0]+2)); 00059 00060 //Put the Setup ACI message in the command queue 00061 if (!hal_aci_tl_send(&msg_to_send)) 00062 { 00063 //ACI Command Queue is full 00064 // *num_cmd_offset is now pointing to the index of the Setup command that did not get sent 00065 return ret_val; 00066 } 00067 00068 ret_val = true; 00069 00070 (*num_cmd_offset)++; 00071 } 00072 00073 return ret_val; 00074 } 00075 00076 uint8_t do_aci_setup(aci_state_t *aci_stat) 00077 { 00078 uint8_t setup_offset = 0; 00079 uint32_t i = 0x0000; 00080 aci_evt_t * aci_evt = NULL; 00081 aci_status_code_t cmd_status = ACI_STATUS_ERROR_CRC_MISMATCH; 00082 00083 /* 00084 We are using the same buffer since we are copying the contents of the buffer 00085 when queuing and immediately processing the buffer when receiving 00086 */ 00087 hal_aci_evt_t *aci_data = (hal_aci_evt_t *)&msg_to_send; 00088 00089 /* Messages in the outgoing queue must be handled before the Setup routine can run. 00090 * If it is non-empty we return. The user should then process the messages before calling 00091 * do_aci_setup() again. 00092 */ 00093 if (!lib_aci_command_queue_empty()) 00094 { 00095 return SETUP_FAIL_COMMAND_QUEUE_NOT_EMPTY; 00096 } 00097 00098 /* If there are events pending from the device that are not relevant to setup, we return false 00099 * so that the user can handle them. At this point we don't care what the event is, 00100 * as any event is an error. 00101 */ 00102 if (lib_aci_event_peek(aci_data)) 00103 { 00104 return SETUP_FAIL_EVENT_QUEUE_NOT_EMPTY; 00105 } 00106 00107 /* Fill the ACI command queue with as many Setup messages as it will hold. */ 00108 aci_setup_fill(aci_stat, &setup_offset); 00109 00110 while (cmd_status != ACI_STATUS_TRANSACTION_COMPLETE) 00111 { 00112 /* This counter is used to ensure that this function does not loop forever. When the device 00113 * returns a valid response, we reset the counter. 00114 */ 00115 if (i++ > 0xFFFFE) 00116 { 00117 return SETUP_FAIL_TIMEOUT; 00118 } 00119 00120 if (lib_aci_event_peek(aci_data)) 00121 { 00122 aci_evt = &(aci_data->evt); 00123 00124 if (ACI_EVT_CMD_RSP != aci_evt->evt_opcode) 00125 { 00126 //Receiving something other than a Command Response Event is an error. 00127 return SETUP_FAIL_NOT_COMMAND_RESPONSE; 00128 } 00129 00130 cmd_status = (aci_status_code_t) aci_evt->params.cmd_rsp.cmd_status; 00131 switch (cmd_status) 00132 { 00133 case ACI_STATUS_TRANSACTION_CONTINUE: 00134 //As the device is responding, reset guard counter 00135 i = 0; 00136 00137 /* As the device has processed the Setup messages we put in the command queue earlier, 00138 * we can proceed to fill the queue with new messages 00139 */ 00140 aci_setup_fill(aci_stat, &setup_offset); 00141 break; 00142 00143 case ACI_STATUS_TRANSACTION_COMPLETE: 00144 //Break out of the while loop when this status code appears 00145 break; 00146 00147 default: 00148 //An event with any other status code should be handled by the application 00149 return SETUP_FAIL_NOT_SETUP_EVENT; 00150 } 00151 00152 /* If we haven't returned at this point, the event was either ACI_STATUS_TRANSACTION_CONTINUE 00153 * or ACI_STATUS_TRANSACTION_COMPLETE. We don't need the event itself, so we simply 00154 * remove it from the queue. 00155 */ 00156 lib_aci_event_get (aci_stat, aci_data); 00157 } 00158 } 00159 00160 return SETUP_SUCCESS; 00161 } 00162
Generated on Tue Jul 12 2022 15:15:45 by
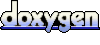