Subdirectory provided by Embedded Artists
Dependencies: DM_FATFileSystem DM_HttpServer DM_USBHost EthernetInterface USBDevice mbed-rpc mbed-rtos mbed-src
Dependents: lpc4088_displaymodule_hello_world_Sept_2018
Fork of DMSupport by
DMBoard.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "DMBoard.h" 00019 00020 #if defined(DM_BOARD_USE_DISPLAY) 00021 #include "BiosDisplay.h" 00022 #endif 00023 #if defined(DM_BOARD_USE_TOUCH) 00024 #include "BiosTouch.h" 00025 #endif 00026 00027 #if defined(DM_BOARD_ENABLE_MEASSURING_PINS) 00028 #include "meas.h" 00029 #endif 00030 00031 /****************************************************************************** 00032 * Configuration Compatibility Control 00033 *****************************************************************************/ 00034 00035 #if defined(DM_BOARD_USE_USB_DEVICE) && defined(DM_BOARD_USE_USB_HOST) 00036 #error The hardware supports either USB Device or USB Host - not both at the same time 00037 #endif 00038 00039 #if defined(DM_BOARD_USE_USBSERIAL_IN_RTOSLOG) && !defined(DM_BOARD_USE_USB_DEVICE) 00040 #error Cannot use USBSerial in RtosLog without DM_BOARD_USE_USB_DEVICE 00041 #endif 00042 00043 #if defined(DM_BOARD_USE_TOUCH) && !defined(DM_BOARD_USE_DISPLAY) 00044 #error Cannot have touch controller without a display! 00045 #endif 00046 00047 /****************************************************************************** 00048 * Defines and typedefs 00049 *****************************************************************************/ 00050 00051 #if defined(DM_BOARD_DISABLE_STANDARD_PRINTF) 00052 class DevNull : public Stream { 00053 00054 public: 00055 DevNull(const char *name=NULL) : Stream(name) {} 00056 00057 protected: 00058 virtual int _getc() {return 0;} 00059 virtual int _putc(int c) {return c;} 00060 }; 00061 00062 static DevNull null("null"); 00063 #endif 00064 00065 00066 /****************************************************************************** 00067 * Local variables 00068 *****************************************************************************/ 00069 00070 /****************************************************************************** 00071 * Private Functions 00072 *****************************************************************************/ 00073 00074 DMBoard::DMBoard() : 00075 _initialized(false), 00076 #if defined(DM_BOARD_USE_MCI_FS) 00077 _mcifs("mci", P4_16), 00078 #endif 00079 #if defined(DM_BOARD_USE_QSPI_FS) 00080 _qspifs("qspi"), 00081 #endif 00082 _buzzer(P1_5), 00083 _button(P2_10), 00084 _led1(LED1), 00085 _led2(LED2), 00086 _led3(LED3), 00087 _led4(LED4) 00088 { 00089 } 00090 00091 DMBoard::~DMBoard() 00092 { 00093 } 00094 00095 /****************************************************************************** 00096 * Public Functions 00097 *****************************************************************************/ 00098 00099 DMBoard::BoardError DMBoard::init() 00100 { 00101 BoardError err = Ok; 00102 if (!_initialized) { 00103 do { 00104 // Turn off the buzzer 00105 _buzzer.period_ms(1); 00106 _buzzer = 0; 00107 00108 // Make sure the button is configured correctly 00109 _button.mode(PullUp); 00110 00111 // Turn off all LEDs 00112 _led1 = 1; 00113 _led2 = 1; 00114 _led3 = 0; 00115 _led4 = 0; 00116 00117 // Make sure that the logger is always initialized even if 00118 // other initialization tasks fail 00119 _logger.init(); 00120 00121 #if defined(DM_BOARD_ENABLE_MEASSURING_PINS) 00122 _INTERNAL_INIT_MEAS(); 00123 #endif 00124 00125 #if defined(DM_BOARD_DISABLE_STANDARD_PRINTF) 00126 // Kill all ouput of calls to printf() so that there is no 00127 // simultaneous calls into the non-thread-safe standard libraries. 00128 // User code should use the RtosLogger anyway. 00129 freopen("/null", "w", stdout); 00130 #endif 00131 00132 #if defined(DM_BOARD_USE_QSPI) || defined(DM_BOARD_USE_QSPI_FS) 00133 if (SPIFI::instance().init() != SPIFI::Ok) { 00134 err = SpifiError; 00135 break; 00136 } 00137 #endif 00138 00139 #if defined(DM_BOARD_USE_DISPLAY) 00140 if (BiosDisplay::instance().init() != Display::DisplayError_Ok) { 00141 err = DisplayError; 00142 break; 00143 } 00144 #endif 00145 00146 #if defined(DM_BOARD_USE_TOUCH) 00147 if (BiosTouch::instance().init() != TouchPanel::TouchError_Ok) { 00148 err = TouchError; 00149 break; 00150 } 00151 #endif 00152 00153 #if defined(DM_BOARD_USE_REGISTRY) 00154 if (Registry::instance().load() != Registry::Ok) { 00155 err = RegistryError; 00156 break; 00157 } 00158 #endif 00159 _initialized = true; 00160 } while(0); 00161 } 00162 return err; 00163 } 00164 00165 void DMBoard::setLED(Leds led, bool on) 00166 { 00167 switch(led) { 00168 case Led1: 00169 _led1 = (on ? 0 : 1); 00170 break; 00171 case Led2: 00172 _led2 = (on ? 0 : 1); 00173 break; 00174 case Led3: 00175 _led3 = (on ? 1 : 0); 00176 break; 00177 case Led4: 00178 _led4 = (on ? 1 : 0); 00179 break; 00180 } 00181 } 00182 00183 void DMBoard::buzzer(int frequency, int duration_ms) 00184 { 00185 if (frequency <= 0) { 00186 _buzzer = 0; 00187 } else { 00188 _buzzer.period_us(1000000/frequency); 00189 _buzzer = 0.5; 00190 } 00191 if (duration_ms > 0) { 00192 Thread::wait(duration_ms); 00193 _buzzer = 0; 00194 } 00195 } 00196 00197 bool DMBoard::buttonPressed() 00198 { 00199 return _button.read() == 0; 00200 } 00201 00202 TouchPanel* DMBoard::touchPanel() 00203 { 00204 #if defined(DM_BOARD_USE_TOUCH) 00205 if (BiosTouch::instance().isTouchSupported()) { 00206 return &BiosTouch::instance(); 00207 } 00208 #endif 00209 return NULL; 00210 } 00211 00212 Display* DMBoard::display() 00213 { 00214 #if defined(DM_BOARD_USE_DISPLAY) 00215 return &BiosDisplay::instance(); 00216 #else 00217 return NULL; 00218 #endif 00219 }
Generated on Wed Jul 13 2022 02:57:23 by
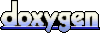