ZumoにFRDM KL-25Zを搭載した時のモーターコントロールライブラリです。 PWM用の端子が互換していないので、割り込みでパルスを作っています。 デフォルトは100Hzの10段階になっています。
Embed:
(wiki syntax)
Show/hide line numbers
ZumoControl.h
00001 /* mbed Zumo motor control Library 00002 * Copyright (c) 2010-2013 jksoft 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef ZUMOCONTROL_H 00024 #define ZUMOCONTROL_H 00025 00026 #include "mbed.h" 00027 00028 #define PERIOD 100.0f // Hz 00029 #define MINPULSWIDTH 1000.0f // us 00030 00031 /** Zumo motor control class 00032 * 00033 * Example: 00034 * @code 00035 * // Drive the Zumo forward, turn left, back, turn right, at half speed for half a second 00036 00037 #include "mbed.h" 00038 #include "ZumoControl.h" 00039 00040 ZumoControl zumo_ctrl; 00041 00042 int main() { 00043 00044 wait(0.5); 00045 00046 zumo_ctrl.forward(0.5); 00047 wait (0.5); 00048 zumo_ctrl.left(0.5); 00049 wait (0.5); 00050 zumo_ctrl.backward(0.5); 00051 wait (0.5); 00052 zumo_ctrl.right(0.5); 00053 wait (0.5); 00054 00055 zumo_ctrl.stop(); 00056 00057 } 00058 * @endcode 00059 */ 00060 class ZumoControl { 00061 00062 // Public functions 00063 public: 00064 00065 /** Create the Zumo object connected to the default pins 00066 * 00067 * @param rc GPIO pin used for Right motor control. Default is PTC9 00068 * @param rp GPIO pin used for Right motor PWM. Default is PTD5 00069 * @param lc GPIO pin used for Left motor control. Default is PTA13 00070 * @param lp GPIO pin used for Left motor PWM. Default is PTD0 00071 */ 00072 ZumoControl(); 00073 00074 00075 /** Create the Zumo object connected to specific pins 00076 * 00077 */ 00078 ZumoControl(PinName rc,PinName rp,PinName lc,PinName lp); 00079 00080 /** Directly control the speed and direction of the left motor 00081 * 00082 * @param speed A normalised number -1.0 - 1.0 represents the full range. 00083 */ 00084 void left_motor (float speed); 00085 00086 /** Directly control the speed and direction of the right motor 00087 * 00088 * @param speed A normalised number -1.0 - 1.0 represents the full range. 00089 */ 00090 void right_motor (float speed); 00091 00092 /** Drive both motors forward as the same speed 00093 * 00094 * @param speed A normalised number 0 - 1.0 represents the full range. 00095 */ 00096 void forward (float speed); 00097 00098 /** Drive both motors backward as the same speed 00099 * 00100 * @param speed A normalised number 0 - 1.0 represents the full range. 00101 */ 00102 void backward (float speed); 00103 00104 /** Drive left motor backwards and right motor forwards at the same speed to turn on the spot 00105 * 00106 * @param speed A normalised number 0 - 1.0 represents the full range. 00107 */ 00108 void left (float speed); 00109 00110 /** Drive left motor forward and right motor backwards at the same speed to turn on the spot 00111 * @param speed A normalised number 0 - 1.0 represents the full range. 00112 */ 00113 void right (float speed); 00114 00115 /** Stop both motors 00116 * 00117 */ 00118 void stop (void); 00119 00120 00121 private : 00122 00123 void cycle(void); 00124 00125 DigitalOut _rc; 00126 DigitalOut _rp; 00127 DigitalOut _lc; 00128 DigitalOut _lp; 00129 00130 int pwm_set_r,pwm_set_l; 00131 int pwm_count,pwm_count_max; 00132 int value_r,value_l; 00133 00134 Ticker _pwm; 00135 }; 00136 00137 #endif
Generated on Thu Jul 14 2022 10:25:10 by
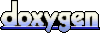