Basic library of routines to interface to a Microchip MCP23017 16-bit I/O expander using an I2C interface.
Dependents: Assignment_2_herpe Final_V1 ass2 ass2 ... more
MCP23017.h
00001 /* MCP23017 library for Arduino 00002 Copyright (C) 2009 David Pye <davidmpye@gmail.com 00003 Modified for use on the MBED ARM platform 00004 00005 This program is free software: you can redistribute it and/or modify 00006 it under the terms of the GNU General Public License as published by 00007 the Free Software Foundation, either version 3 of the License, or 00008 (at your option) any later version. 00009 00010 This program is distributed in the hope that it will be useful, 00011 but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00013 GNU General Public License for more details. 00014 00015 You should have received a copy of the GNU General Public License 00016 along with this program. If not, see <http://www.gnu.org/licenses/>. 00017 */ 00018 00019 #ifndef MBED_MCP23017_H 00020 #define MBED_MCP23017_H 00021 00022 #include "mbed.h" 00023 00024 // 00025 // Register defines from data sheet - we set IOCON.BANK to 0 00026 // as it is easier to manage the registers sequentially. 00027 // 00028 #define IODIR 0x00 00029 #define IPOL 0x02 00030 #define GPINTEN 0x04 00031 #define DEFVAL 0x06 00032 #define INTCON 0x08 00033 #define IOCON 0x0A 00034 #define GPPU 0x0C 00035 #define INTF 0x0E 00036 #define INTCAP 0x10 00037 #define GPIO 0x12 00038 #define OLAT 0x14 00039 00040 #define I2C_BASE_ADDRESS 0x40 00041 00042 #define DIR_OUTPUT 0 00043 #define DIR_INPUT 1 00044 00045 /** MCP23017 class 00046 * 00047 * Allow access to an I2C connected MCP23017 16-bit I/O extender chip 00048 * Example: 00049 * @code 00050 * MCP23017 *par_port; 00051 * @endcode 00052 * 00053 */ 00054 class MCP23017 { 00055 public: 00056 /** Constructor for the MCP23017 connected to specified I2C pins at a specific address 00057 * 00058 * 16-bit I/O expander with I2C interface 00059 * 00060 * @param sda I2C data pin 00061 * @param scl I2C clock pin 00062 * @param i2cAddress I2C address 00063 */ 00064 MCP23017(PinName sda, PinName scl, int i2cAddress); 00065 00066 /** Reset MCP23017 device to its power-on state 00067 */ 00068 void reset(void); 00069 00070 /** Write a 0/1 value to an output bit 00071 * 00072 * @param value 0 or 1 00073 * @param bit_number bit number range 0 --> 15 00074 */ 00075 void write_bit(int value, int bit_number); 00076 00077 /** Write a masked 16-bit value to the device 00078 * 00079 * @param data 16-bit data value 00080 * @param mask 16-bit mask value 00081 */ 00082 void write_mask(unsigned short data, unsigned short mask); 00083 00084 /** Read a 0/1 value from an input bit 00085 * 00086 * @param bit_number bit number range 0 --> 15 00087 * @return 0/1 value read 00088 */ 00089 int read_bit(int bit_number); 00090 00091 /** Read a 16-bit value from the device and apply mask 00092 * 00093 * @param mask 16-bit mask value 00094 * @return 16-bit data with mask applied 00095 */ 00096 int read_mask(unsigned short mask); 00097 00098 /** Configure an MCP23017 device 00099 * 00100 * @param dir_config data direction value (1 = input, 0 = output) 00101 * @param pullup_config 100k pullup value (1 = enabled, 0 = disabled) 00102 * @param polarity_config polarity value (1 = flip, 0 = normal) 00103 */ 00104 void config(unsigned short dir_config, unsigned short pullup_config, unsigned short polarity_config); 00105 00106 void writeRegister(int regAddress, unsigned char val); 00107 void writeRegister(int regAddress, unsigned short val); 00108 int readRegister(int regAddress); 00109 00110 /*----------------------------------------------------------------------------- 00111 * pinmode 00112 * Set units to sequential, bank0 mode 00113 */ 00114 void pinMode(int pin, int mode); 00115 void digitalWrite(int pin, int val); 00116 int digitalRead(int pin); 00117 00118 // These provide a more advanced mapping of the chip functionality 00119 // See the data sheet for more information on what they do 00120 00121 //Returns a word with the current pin states (ie contents of the GPIO register) 00122 unsigned short digitalWordRead(); 00123 // Allows you to write a word to the GPIO register 00124 void digitalWordWrite(unsigned short w); 00125 // Sets up the polarity mask that the MCP23017 supports 00126 // if set to 1, it will flip the actual pin value. 00127 void inputPolarityMask(unsigned short mask); 00128 //Sets which pins are inputs or outputs (1 = input, 0 = output) NB Opposite to arduino's 00129 //definition for these 00130 void inputOutputMask(unsigned short mask); 00131 // Allows enabling of the internal 100k pullup resisters (1 = enabled, 0 = disabled) 00132 void internalPullupMask(unsigned short mask); 00133 int read(void); 00134 void write(int data); 00135 00136 protected: 00137 I2C _i2c; 00138 int MCP23017_i2cAddress; // physical I2C address 00139 unsigned short shadow_GPIO, shadow_IODIR, shadow_GPPU, shadow_IPOL; // Cached copies of the register values 00140 00141 }; 00142 00143 #endif
Generated on Mon Jul 18 2022 21:09:52 by
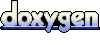