
this is fork and i will modify for STM32
Fork of AWS-test by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2017, CATIE, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include "mbed.h" 00018 //#include "EthernetInterface.h" 00019 00020 #include "aws_iot_config.h" 00021 #include "aws_iot_mqtt_client_interface.h" 00022 00023 namespace { 00024 #define READ_DURATION 500 00025 #define DEMO_PAYLOAD "Hello, world!" 00026 #define PUBLISH_TOPIC "sdkTest/sub" 00027 #define PUBLISH_TOPIC_LEN 11 00028 } 00029 00030 void subscribe_callback(AWS_IoT_Client *client, char *topic_name, 00031 uint16_t topic_name_length, IoT_Publish_Message_Params *params, 00032 void *data) 00033 { 00034 printf("Subscribe callback"); 00035 printf("%.*s\t%.*s", topic_name_length, topic_name, (int)params->payloadLen, (char *)params->payload); 00036 } 00037 00038 void disconnect_handler(AWS_IoT_Client *client, void *data) 00039 { 00040 printf("MQTT Disconnect"); 00041 IoT_Error_t rc = IOT_FAILURE; 00042 00043 if (!client) { 00044 return; 00045 } 00046 00047 if (aws_iot_is_autoreconnect_enabled(client)) { 00048 printf("Auto Reconnect is enabled, Reconnecting attempt will start now"); 00049 } else { 00050 printf("Auto Reconnect not enabled. Starting manual reconnect..."); 00051 rc = aws_iot_mqtt_attempt_reconnect(client); 00052 if (NETWORK_RECONNECTED == rc) { 00053 printf("Manual Reconnect Successful"); 00054 } else { 00055 printf("Manual Reconnect Failed - %d", rc); 00056 } 00057 } 00058 } 00059 00060 //EthernetInterface net; 00061 00062 int main() 00063 { 00064 //net.connect(); 00065 AWS_IoT_Client client; 00066 00067 /* 00068 * MQTT Init 00069 */ 00070 00071 IoT_Client_Init_Params mqtt_init_params = iotClientInitParamsDefault; 00072 mqtt_init_params.enableAutoReconnect = false; // We enable this later below 00073 char mqtt_host[] = AWS_IOT_MQTT_HOST; 00074 mqtt_init_params.pHostURL = mqtt_host; 00075 mqtt_init_params.port = AWS_IOT_MQTT_PORT; 00076 mqtt_init_params.disconnectHandler = disconnect_handler; 00077 mqtt_init_params.disconnectHandlerData = NULL; 00078 00079 IoT_Error_t mqtt_init_status = aws_iot_mqtt_init(&client, &mqtt_init_params); 00080 if (mqtt_init_status != IOT_SUCCESS) { 00081 printf("aws_iot_mqtt_init returned error : %d ", mqtt_init_status); 00082 while (true) { Thread::wait(1000); } 00083 } 00084 00085 /* 00086 * MQTT Connect 00087 */ 00088 00089 IoT_Client_Connect_Params connect_params = iotClientConnectParamsDefault; 00090 00091 connect_params.keepAliveIntervalInSec = 10; 00092 connect_params.isCleanSession = true; 00093 connect_params.MQTTVersion = MQTT_3_1_1; 00094 char client_id[] = AWS_IOT_MQTT_CLIENT_ID; 00095 connect_params.pClientID = client_id; 00096 connect_params.clientIDLen = (uint16_t)strlen(AWS_IOT_MQTT_CLIENT_ID); 00097 connect_params.isWillMsgPresent = false; 00098 00099 IoT_Error_t mqtt_connect_status = aws_iot_mqtt_connect(&client, &connect_params); 00100 if (mqtt_connect_status != IOT_SUCCESS) { 00101 printf("Error(%d) connecting to %s:%d", mqtt_connect_status, 00102 mqtt_init_params.pHostURL, mqtt_init_params.port); 00103 while (true) { Thread::wait(1000); } 00104 } 00105 00106 /* 00107 * Set MQTT autoreconnect 00108 */ 00109 00110 IoT_Error_t mqtt_autoreconnect_status = aws_iot_mqtt_autoreconnect_set_status(&client, true); 00111 if (mqtt_autoreconnect_status != IOT_SUCCESS) { 00112 printf("Unable to set Auto Reconnect to true - %d", mqtt_autoreconnect_status); 00113 while (true) { Thread::wait(1000); } 00114 } 00115 00116 /* 00117 * MQTT Subscribe 00118 */ 00119 00120 IoT_Error_t mqtt_subscribe_status = aws_iot_mqtt_subscribe( 00121 &client, "sdkTest/sub", 11, QOS0, subscribe_callback, NULL); 00122 if (mqtt_subscribe_status != IOT_SUCCESS) { 00123 printf("Error subscribing: %d ", mqtt_subscribe_status); 00124 while (true) { Thread::wait(1000); } 00125 } 00126 00127 /* 00128 * MQTT Publish 00129 */ 00130 00131 IoT_Publish_Message_Params mqtt_publish_params; 00132 mqtt_publish_params.qos = QOS0; 00133 char payload[] = DEMO_PAYLOAD; 00134 mqtt_publish_params.payload = payload; 00135 mqtt_publish_params.payloadLen = strlen(payload); 00136 mqtt_publish_params.isRetained = 0; 00137 00138 // Main loop 00139 IoT_Error_t mqtt_status; 00140 while (true) { 00141 //Max time the yield function will wait for read messages 00142 mqtt_status = aws_iot_mqtt_yield(&client, READ_DURATION); 00143 if (mqtt_status == NETWORK_ATTEMPTING_RECONNECT) { 00144 // If the client is attempting to reconnect we will skip the rest of the loop. 00145 continue; 00146 } 00147 00148 mqtt_status = aws_iot_mqtt_publish( 00149 &client, PUBLISH_TOPIC, PUBLISH_TOPIC_LEN, &mqtt_publish_params); 00150 if (mqtt_status != IOT_SUCCESS) { 00151 printf("Error publishing: %d", mqtt_status); 00152 } 00153 } 00154 }
Generated on Tue Jul 12 2022 11:16:38 by
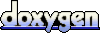