
this is fork and i will modify for STM32
Fork of AWS-test by
Embed:
(wiki syntax)
Show/hide line numbers
aws_iot_mqtt_client_interface.h
00001 /* 00002 * Copyright 2015-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 // Based on Eclipse Paho. 00017 /******************************************************************************* 00018 * Copyright (c) 2014 IBM Corp. 00019 * 00020 * All rights reserved. This program and the accompanying materials 00021 * are made available under the terms of the Eclipse Public License v1.0 00022 * and Eclipse Distribution License v1.0 which accompany this distribution. 00023 * 00024 * The Eclipse Public License is available at 00025 * http://www.eclipse.org/legal/epl-v10.html 00026 * and the Eclipse Distribution License is available at 00027 * http://www.eclipse.org/org/documents/edl-v10.php. 00028 * 00029 * Contributors: 00030 * Ian Craggs - initial API and implementation and/or initial documentation 00031 * Xiang Rong - 442039 Add makefile to Embedded C client 00032 *******************************************************************************/ 00033 00034 /** 00035 * @file aws_iot_mqtt_interface.h 00036 * @brief Interface definition for MQTT client. 00037 */ 00038 00039 #ifndef AWS_IOT_SDK_SRC_IOT_MQTT_INTERFACE_H 00040 #define AWS_IOT_SDK_SRC_IOT_MQTT_INTERFACE_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Library Header files */ 00047 #include "stdio.h" 00048 #include "stdbool.h" 00049 #include "stdint.h" 00050 #include "stddef.h" 00051 00052 /* AWS Specific header files */ 00053 #include "aws_iot_error.h" 00054 #include "aws_iot_config.h" 00055 #include "aws_iot_mqtt_client.h" 00056 00057 /* Platform specific implementation header files */ 00058 #include "network_interface.h" 00059 #include "timer_interface.h" 00060 00061 /** 00062 * @brief MQTT Client Initialization Function 00063 * 00064 * Called to initialize the MQTT Client 00065 * 00066 * @param pClient Reference to the IoT Client 00067 * @param pInitParams Pointer to MQTT connection parameters 00068 * 00069 * @return IoT_Error_t Type defining successful/failed API call 00070 */ 00071 IoT_Error_t aws_iot_mqtt_init(AWS_IoT_Client *pClient, IoT_Client_Init_Params *pInitParams); 00072 00073 /** 00074 * @brief MQTT Connection Function 00075 * 00076 * Called to establish an MQTT connection with the AWS IoT Service 00077 * 00078 * @param pClient Reference to the IoT Client 00079 * @param pConnectParams Pointer to MQTT connection parameters 00080 * 00081 * @return An IoT Error Type defining successful/failed connection 00082 */ 00083 IoT_Error_t aws_iot_mqtt_connect(AWS_IoT_Client *pClient, IoT_Client_Connect_Params *pConnectParams); 00084 00085 /** 00086 * @brief Publish an MQTT message on a topic 00087 * 00088 * Called to publish an MQTT message on a topic. 00089 * @note Call is blocking. In the case of a QoS 0 message the function returns 00090 * after the message was successfully passed to the TLS layer. In the case of QoS 1 00091 * the function returns after the receipt of the PUBACK control packet. 00092 * 00093 * @param pClient Reference to the IoT Client 00094 * @param pTopicName Topic Name to publish to 00095 * @param topicNameLen Length of the topic name 00096 * @param pParams Pointer to Publish Message parameters 00097 * 00098 * @return An IoT Error Type defining successful/failed publish 00099 */ 00100 IoT_Error_t aws_iot_mqtt_publish(AWS_IoT_Client *pClient, const char *pTopicName, uint16_t topicNameLen, 00101 IoT_Publish_Message_Params *pParams); 00102 00103 /** 00104 * @brief Subscribe to an MQTT topic. 00105 * 00106 * Called to send a subscribe message to the broker requesting a subscription 00107 * to an MQTT topic. 00108 * @note Call is blocking. The call returns after the receipt of the SUBACK control packet. 00109 * 00110 * @param pClient Reference to the IoT Client 00111 * @param pTopicName Topic Name to publish to 00112 * @param topicNameLen Length of the topic name 00113 * @param pApplicationHandler_t Reference to the handler function for this subscription 00114 * @param pApplicationHandlerData Data to be passed as argument to the application handler callback 00115 * 00116 * @return An IoT Error Type defining successful/failed subscription 00117 */ 00118 IoT_Error_t aws_iot_mqtt_subscribe(AWS_IoT_Client *pClient, const char *pTopicName, uint16_t topicNameLen, 00119 QoS qos, pApplicationHandler_t pApplicationHandler, void *pApplicationHandlerData); 00120 00121 /** 00122 * @brief Subscribe to an MQTT topic. 00123 * 00124 * Called to resubscribe to the topics that the client has active subscriptions on. 00125 * Internally called when autoreconnect is enabled 00126 * 00127 * @note Call is blocking. The call returns after the receipt of the SUBACK control packet. 00128 * 00129 * @param pClient Reference to the IoT Client 00130 * 00131 * @return An IoT Error Type defining successful/failed subscription 00132 */ 00133 IoT_Error_t aws_iot_mqtt_resubscribe(AWS_IoT_Client *pClient); 00134 00135 /** 00136 * @brief Unsubscribe to an MQTT topic. 00137 * 00138 * Called to send an unsubscribe message to the broker requesting removal of a subscription 00139 * to an MQTT topic. 00140 * @note Call is blocking. The call returns after the receipt of the UNSUBACK control packet. 00141 * 00142 * @param pClient Reference to the IoT Client 00143 * @param pTopicName Topic Name to publish to 00144 * @param topicNameLen Length of the topic name 00145 * 00146 * @return An IoT Error Type defining successful/failed unsubscribe call 00147 */ 00148 IoT_Error_t aws_iot_mqtt_unsubscribe(AWS_IoT_Client *pClient, const char *pTopicFilter, uint16_t topicFilterLen); 00149 00150 /** 00151 * @brief Disconnect an MQTT Connection 00152 * 00153 * Called to send a disconnect message to the broker. 00154 * 00155 * @param pClient Reference to the IoT Client 00156 * 00157 * @return An IoT Error Type defining successful/failed send of the disconnect control packet. 00158 */ 00159 IoT_Error_t aws_iot_mqtt_disconnect(AWS_IoT_Client *pClient); 00160 00161 /** 00162 * @brief Yield to the MQTT client 00163 * 00164 * Called to yield the current thread to the underlying MQTT client. This time is used by 00165 * the MQTT client to manage PING requests to monitor the health of the TCP connection as 00166 * well as periodically check the socket receive buffer for subscribe messages. Yield() 00167 * must be called at a rate faster than the keepalive interval. It must also be called 00168 * at a rate faster than the incoming message rate as this is the only way the client receives 00169 * processing time to manage incoming messages. 00170 * 00171 * @param pClient Reference to the IoT Client 00172 * @param timeout_ms Maximum number of milliseconds to pass thread execution to the client. 00173 * 00174 * @return An IoT Error Type defining successful/failed client processing. 00175 * If this call results in an error it is likely the MQTT connection has dropped. 00176 * iot_is_mqtt_connected can be called to confirm. 00177 */ 00178 IoT_Error_t aws_iot_mqtt_yield(AWS_IoT_Client *pClient, uint32_t timeout_ms); 00179 00180 /** 00181 * @brief MQTT Manual Re-Connection Function 00182 * 00183 * Called to establish an MQTT connection with the AWS IoT Service 00184 * using parameters from the last time a connection was attempted 00185 * Use after disconnect to start the reconnect process manually 00186 * Makes only one reconnect attempt Sets the client state to 00187 * pending reconnect in case of failure 00188 * 00189 * @param pClient Reference to the IoT Client 00190 * 00191 * @return An IoT Error Type defining successful/failed connection 00192 */ 00193 IoT_Error_t aws_iot_mqtt_attempt_reconnect(AWS_IoT_Client *pClient); 00194 00195 #ifdef __cplusplus 00196 } 00197 #endif 00198 00199 #endif
Generated on Tue Jul 12 2022 11:16:37 by
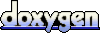