C++ class for controlling DC motor with encoder feedback. Dependencies include LS7366LIB, MotCon, and PID.
Dependencies: LS7366LIB MotCon2 PID
Axis.h
00001 00002 00003 #ifndef MBED_AXIS_H 00004 #define MBED_AXIS_H 00005 00006 #include "mbed.h" 00007 #include "PID.h" //library for software routine PID controller 00008 #include "LS7366.h" //library for quadrature encoder interface IC's 00009 #include "MotCon.h" //simple motor control routines 00010 00011 class Axis{ 00012 public: 00013 Axis(SPI& _spi, PinName _cs, PinName _pwm, PinName _dir, PinName _analog, int* limit); 00014 void paramUpdate(void); 00015 void center(void); 00016 void init(void); 00017 void moveTrapezoid(float position, float time); 00018 void moveUpdate(void); 00019 float readCurrent(void); 00020 void axisOff(void); 00021 void axisOn(void); 00022 void zero(void); 00023 void writeEncoderValue(long value); 00024 void updatePIDgains(float P, float I, float D); 00025 00026 long enc; //used to return the data from the LS7366 encoder chip 00027 float co; // = 0.0; 00028 float Tdelay; // = .01; 00029 float Pk; // 120.0 for scorbot 00030 float Ik; // 55.0 for scorbot 00031 float Dk; 00032 float set_point;// = 0.0; 00033 float set_point_last; 00034 float pos, vel, acc; //calculated position, velocity, and acceleration 00035 int stat; //overall axis status 00036 float pos_last, vel_last, acc_last; //history variables used to calculate motion 00037 float pos_cmd, vel_cmd, vel_avg_cmd, acc_cmd; 00038 float vel_max, acc_max; 00039 float vel_accum; 00040 float moveTime; 00041 float p_higher, p_lower; 00042 int moveStatus; 00043 int moveState; 00044 int debug; 00045 int *ptr_limit; 00046 float motI; //motor current read from readCurrent() function 00047 volatile float motI_last; 00048 float mot_I_lim; //max current limit 00049 float dIdT; 00050 float mot_I_max, mot_I_max_last; 00051 int axisState; 00052 int motInvert; 00053 char dataFormat; //'r'=radians (default), 'd'=degrees, 'e'=encoder counts 00054 float pos_rad, vel_rad; //current position measurement in radians 00055 float pos_deg, vel_deg; //current position measurement in degrees 00056 float ctsPerDeg; 00057 int busyflag; 00058 00059 Ticker update; 00060 Ticker moveProfile; 00061 Timer t; 00062 PID *pid; 00063 LS7366 *ls7366; 00064 MotCon *motcon; 00065 //AnalogIn *motCurrent; 00066 00067 private: 00068 SPI _spi; 00069 DigitalOut _cs; 00070 PwmOut _pwm; 00071 DigitalOut _dir; 00072 AnalogIn _analog; 00073 }; 00074 00075 #endif 00076 00077
Generated on Tue Jul 26 2022 19:48:09 by
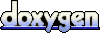