A servo library for controlling servos without using PWM. Can be used on any pin.
Dependents: ServoTest ServoTest Nucleo_coffee NervousPuppySprintOne ... more
Servo Class Reference
Class to control a servo on any pin, without using pwm. More...
#include <Servo.h>
Public Member Functions | |
Servo (PinName Pin) | |
Create a new Servo object on any mbed pin. | |
void | SetPosition (int NewPos) |
Change the position of the servo. | |
void | Enable (int StartPos, int Period) |
Enable the servo. | |
void | Disable () |
Disable the servo. |
Detailed Description
Class to control a servo on any pin, without using pwm.
Example:
// Keep sweeping servo from left to right #include "mbed.h" #include "Servo.h" Servo Servo1(p20); Servo1.Enable(1500,20000); while(1) { for (int pos = 1000; pos < 2000; pos += 25) { Servo1.SetPosition(pos); wait_ms(20); } for (int pos = 2000; pos > 1000; pos -= 25) { Servo1.SetPosition(pos); wait_ms(20); } }
Definition at line 53 of file Servo.h.
Constructor & Destructor Documentation
Servo | ( | PinName | Pin ) |
Member Function Documentation
void Disable | ( | ) |
void Enable | ( | int | StartPos, |
int | Period | ||
) |
Generated on Tue Jul 12 2022 12:33:04 by
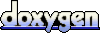